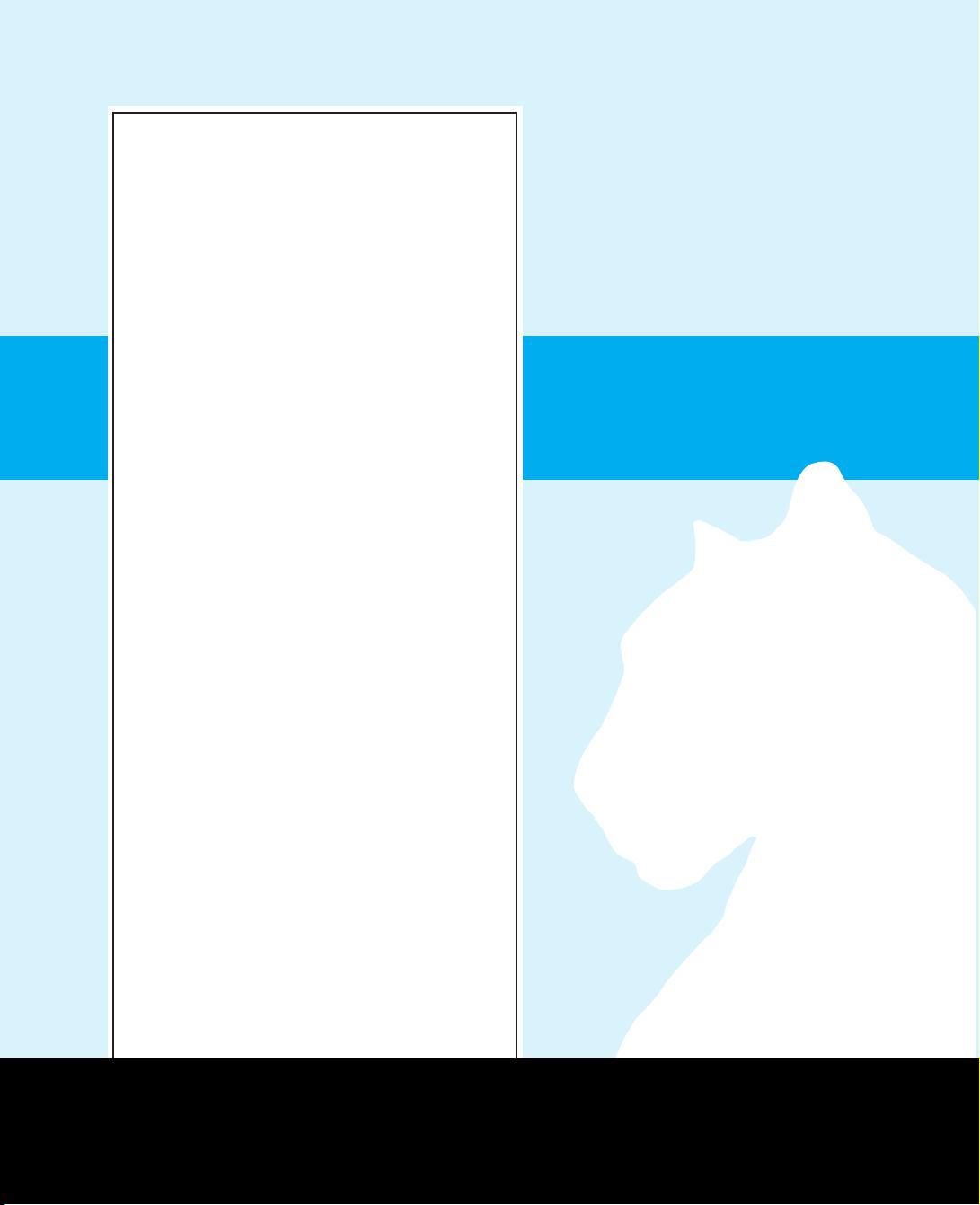
1
Getting Started
1.1 Introduction to Java 2
Origins of the Java Language
★
2
Objects and Methods 3
Applets
★
4
A Sample Java Application Program 5
Byte-Code and the Java Virtual Machine 8
Class Loader
★
10
Compiling a Java Program or Class 10
Running a Java Program 11
1.2 Expressions and Assignment
Statements 13
Identifiers 13
Variables 15
Assignment Statements 16
More Assignment Statements
★
19
Assignment Compatibility 20
Constants 21
Arithmetic Operators and Expressions 23
Parentheses and Precedence Rules
★
24
Integer and Floating-Point Division 26
Type Casting 29
Increment and Decrement Operators 30
1.3 The Class
String
33
String Constants and Variables 33
Concatenation of Strings 34
Classes 35
String Methods 37
Escape Sequences 42
String Processing 43
The Unicode Character Set
★
43
1.4 Program Style 46
Naming Constants 46
Java Spelling Conventions 48
Comments 49
Indenting 50
Chapter Summary 51
Answers to Self-Test Exercises 52
Programming Projects 54
ch01_v2.fm Page 1 Saturday, February 10, 2007 1:13 PM
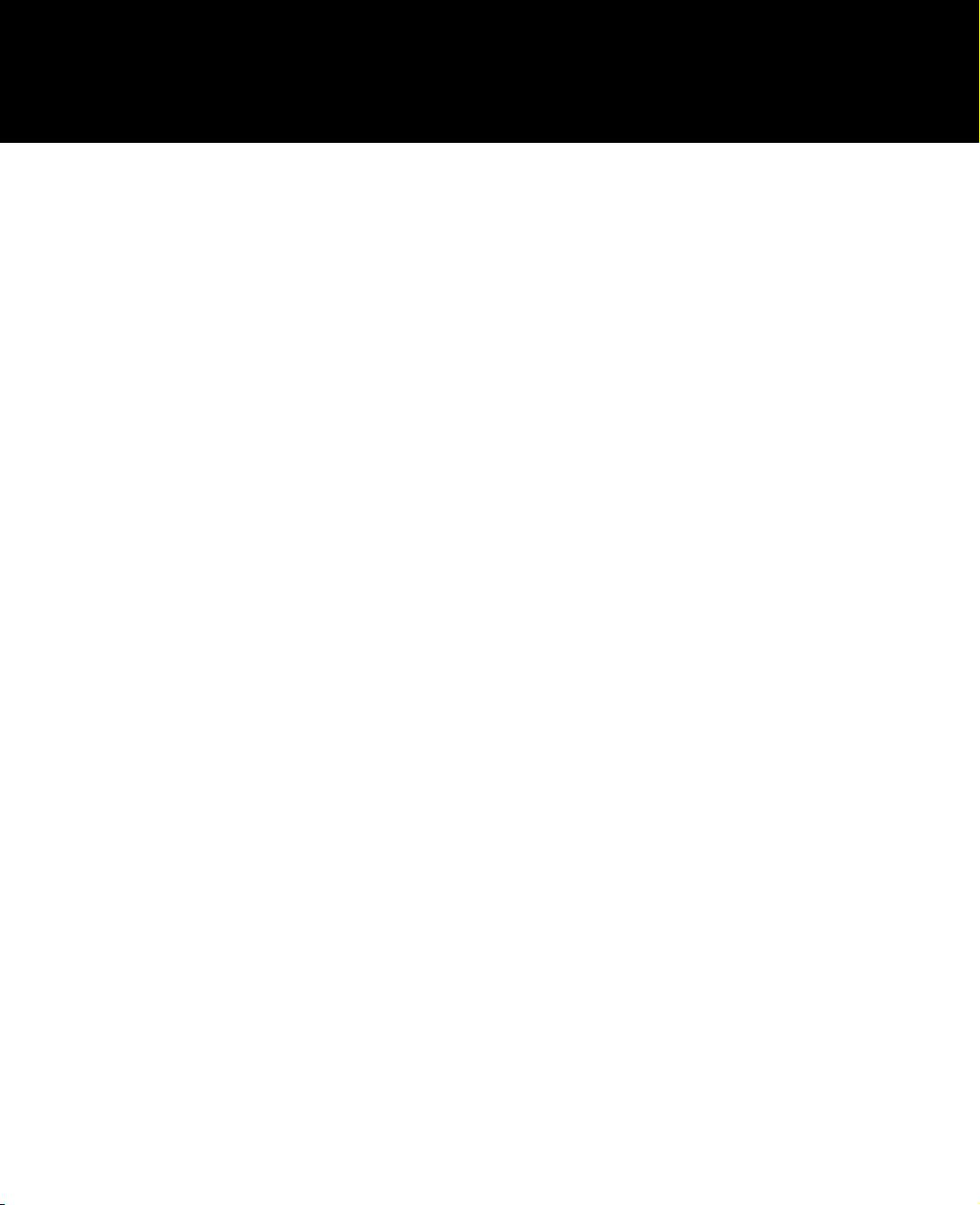
1
Getting Started
She starts—she moves—she seems to feel
The thrill of life along her keel.
HENRY WADSWORTH LONGFELLOW,
The Building of the Ship
Introduction
This chapter introduces you to the Java language and gives you enough details to allow
you to write simple programs involving expressions, assignments, and console output.
The details about assignments and expressions are similar to that of most other high-
level languages. Every language has its own way of handling strings and console out-
put, so even the experienced programmer should look at that material. Even if you are
already an experienced programmer in some language other than Java, you should read
at least the subsection entitled “A Sample Java Application Program” in Section 1.1
and preferably all of Section 1.2, and you should read all of Section 1.3 on strings and
at least skim Section 1.4 to find out about Java defined constants and comments.
Prerequisites
This book is self-contained and requires no preparation other than some simple high
school algebra.
1.1
Introduction to Java
Eliminating the middle man is not necessarily a good idea.
Found in my old economics class notes
In this section we give you an overview of the Java programming language.
Origins of the Java Language
★
Java is well-known as a programming language for Internet applications. However, this
book, and many other books and programmers, view Java as a general-purpose program-
ming language that is suitable for most any application whether it involves the Internet
or not. The first version of Java was neither of these things, but it evolved into both of
these things.
In 1991, James Gosling led a team at Sun Microsystems that developed the first
version of Java (which was not yet called
Java
). This first version of the language was
ch01_v2.fm Page 2 Saturday, February 10, 2007 1:13 PM
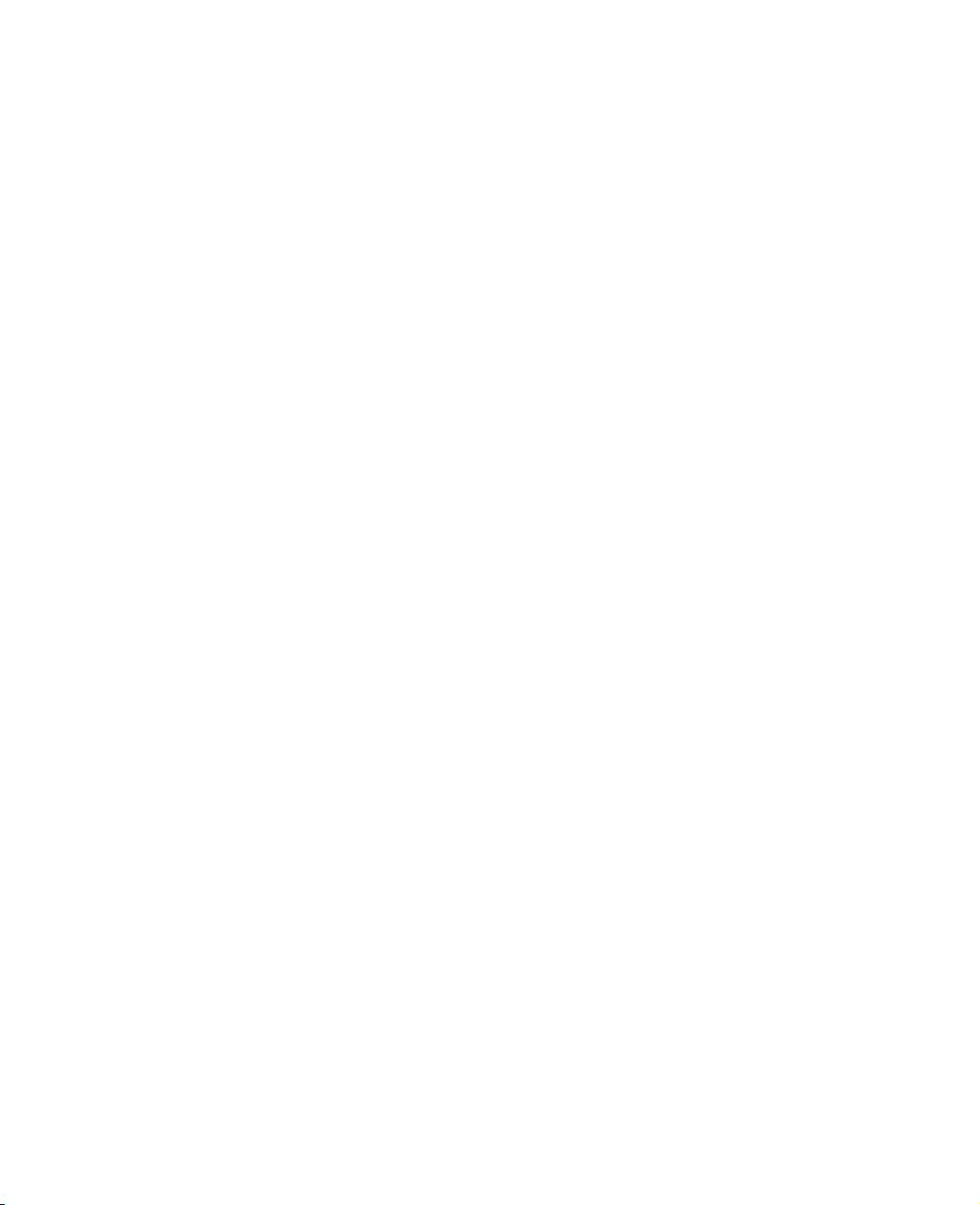
Introduction to Java
3
designed for programming home appliances, such as washing machines and televi-
sion sets. Although that may not be a very glamorous application area, it is no easy
task to design such a language. Home appliances are controlled by a wide variety of
computer processors (chips). The language that Gosling was designing needed to
work on all these different processors. Moreover, a home appliance is typically an
inexpensive item, so the manufacturer would be unwilling to invest large amounts
of money into developing complicated compilers. (A compiler is a program that
translates the program into a language the processor can understand.) To simplify
the tasks of writing compilers (translation programs) for each class of appliances, the
team used a two-step translation process. The programs are first translated into an
intermediate language
that is the same for all appliances (or all computers), then a
small, easy-to-write, and hence inexpensive, program translates this intermediate
language into the machine language for a particular appliance or computer. This
intermediate language is called
Java byte-code
or simply
byte-code
. Since there is
only one intermediate language, the hardest step of the two-step translation from
program to intermediate language to machine language is the same for all appliances
(or all computers), and hence, most of the cost of translating to multiple machine
languages was saved. The language for programming appliances never caught on
with appliance manufacturers, but the Java language into which it evolved has
become a widely used programming language.
Why call it
byte-code
? The word
code
is commonly used to mean a program or part
of a program. A byte is a small unit of storage (eight bits to be precise). Computer-
readable information is typically organized into bytes. So the term
byte-code
suggests a
program that is readable by a computer as opposed to a person.
In 1994, Patrick Naughton and Jonathan Payne at Sun Microsystems developed a
Web browser that could run (Java) programs over the Internet. That Web browser has
evolved into the browser known as HotJava. This was the start of Java’s connection to
the Internet. In the fall of 1995, Netscape Incorporated made its Web browser capable
of running Java programs. Other companies followed suit and have developed soft-
ware that accommodates Java programs.
Objects and Methods
Java is an
object-oriented programming
language, abbreviated
OOP
. What is OOP?
The world around us is made up of objects, such as people, automobiles, buildings,
streets, adding machines, papers, and so forth. Each of these objects has the ability to
perform certain actions, and each of these actions has some effect on some of the other
objects in the world. OOP is a programming methodology that views a program as
similarly consisting of objects that interact with each other by means of actions.
Object-oriented programming has its own specialized terminology. The objects are
called, appropriately enough,
objects
. The actions that an object can take are called
methods
. Objects of the same kind are said to have the same
type
or, more often, are
said to be in the same
class
. For example, in an airport simulation program, all the
intermediate
language
byte-code
code
OOP
object
method
class
ch01_v2.fm Page 3 Saturday, February 10, 2007 1:13 PM
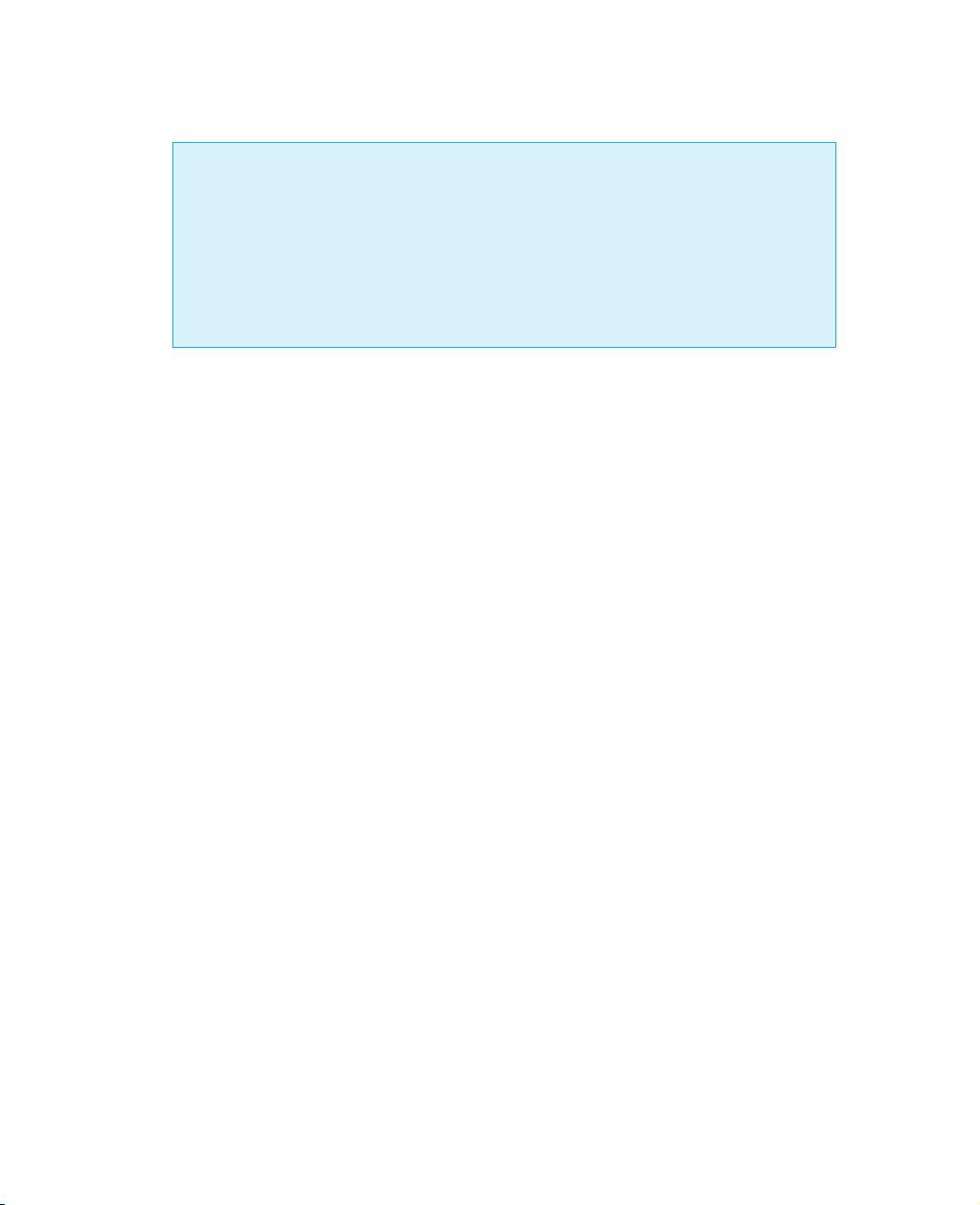
4
CHAPTER 1 Getting Started
simulated airplanes might belong to the same class, probably called the
Airplane
class.
All objects within a class have the same methods. Thus, in a simulation program, all
airplanes have the same methods (or possible actions) such as taking off, flying to a
specific location, landing, and so forth. However, all simulated airplanes are not iden-
tical. They can have different characteristics, which are indicated in the program by
associating different data (that is, some different information) with each particular air-
plane object. For example, the data associated with an airplane object might be two
numbers for its speed and altitude.
If you have used some other programming language, it might help to explain Java
terminology in terms of the terminology used in other languages. Things that are
called
procedures,
methods,
functions,
or
subprograms
in other languages are all called
methods
in Java. In Java, all methods (and for that matter, any programming constructs
whatsoever) are part of a class. As we will see, a Java
application
program
is a class
with a method named
main
, and when you run the Java program, the run-time system
automatically invokes the method named
main
(that is, it automatically initiates the
main
action). An application program is a “regular” Java program; as we are about to
see, there is another kind of Java program known as an applet. Other Java terminology
is pretty much the same as the terminology in most other programming languages
and, in any case, will be explained when each concept is introduced.
Applets
★
There are two kinds of Java programs,
applets
and
applications
. An application or
application program is just a regular program. Although the name
applet
may sound
like it has something to do with apples, the name really means a
little Java application,
not a little apple. Applets and applications are almost identical. The difference is that
applications are meant to be run on your computer like any other program, whereas
an
applet
is meant to be run from a Web browser, and so can be sent to another loca-
tion on the Internet and run there. Applets always use a windowing interface, but not
all programs with a windowing interface are applets, as you will see in Chapters 16–
18.
Although applets were designed to be run from a Web browser, they can also be
run with a program known as an
applet viewer
. The applet viewer is really meant as
Why Is the Language Named “Java”?
The current custom is to name programming languages according to the whims of their
designers. Java is no exception. There are conflicting explanations of the origin of the name
“Java.” Despite these conflicting stories, one thing is clear: The word “Java” does not refer
to any property or serious history of the Java language. One believable story about where
the name “Java” came from is that the name was thought of when, after a fruitless meet-
ing trying to come up with a new name for the language, the development team went out
for coffee, and hence the inspiration for the name “Java.”
application
program
applet
application
applet viewer
ch01_v2.fm Page 4 Saturday, February 10, 2007 1:13 PM
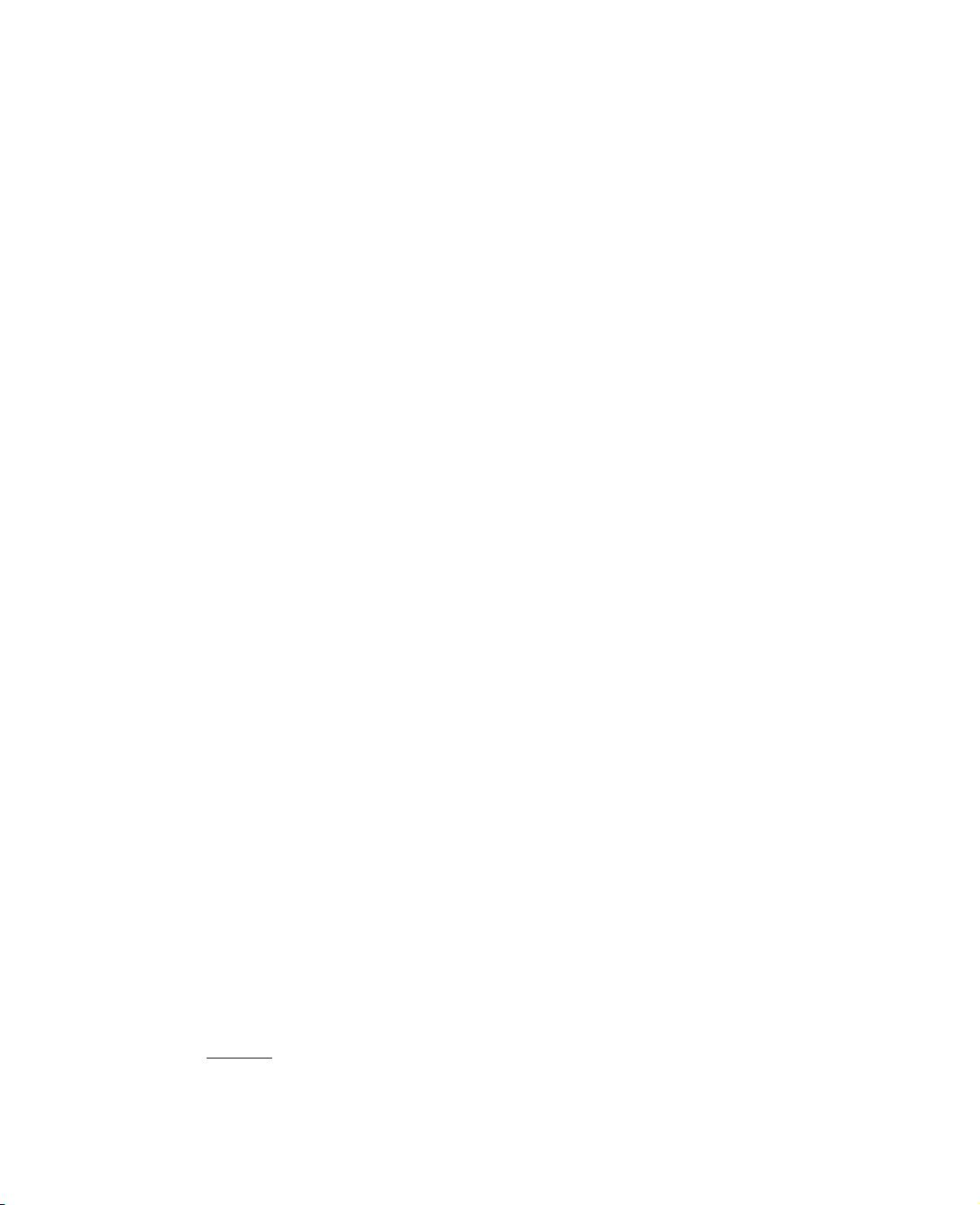
Introduction to Java
5
a debugging aid and not as the final environment to allow users to run applets.
Nonetheless, applets are now often run as stand-alone programs using an applet
viewer.
1
We find this to be a somewhat unfortunate accident of history. Java has multi-
ple libraries of software for designing windowing interfaces that run with no connec-
tion to a browser. We prefer to use these libraries, rather than applets, to write
windowing programs that will not be run from a Web browser. In this book we show
you how to do windowing interfaces as applets and as programs with no connection to
a Web browser. In fact, the two approaches have a large overlap of both techniques and
the Java libraries that they use. Once you know how to design and write either applets
or applications, it is easy to learn to write the other of these two kinds of programs.
An applet always has a windowing interface. An application program may have a
windowing interface or use simple console I/O. So as not to detract from the code
being studied, most of our example programs, particularly early in the book, use sim-
ple console I/O (that is, simple text I/O).
A Sample Java Application Program
Display 1.1 contains a simple Java program and the screen displays produced when it
is run. A Java program is really a class definition (whatever that is) with a method
named
main
. When the program is run, the method named
main
is invoked; that is,
the action specified by
main
is carried out. The body of the method
main
is enclosed
in braces,
{}
, so that when the program is run, the statements in the braces are exe-
cuted. (If you are not even vaguely familiar with the words
class
and
method,
they will
be explained. Read on.)
The following line says that this program is a class called
FirstProgram
:
public class FirstProgram
{
The next two lines, shown below, begin the definition of the
main
method:
public static void main(String[] args)
{
The details of exactly what a Java class is and what words like
public
,
static
,
void
,
and so forth mean will be explained in the next few chapters. Until then, you can
think of these opening lines, repeated below, as being a rather wordy way of saying
“Begin the program named
FirstProgram
.”
public class FirstProgram
{
public static void main(String[] args)
{
1
An applet viewer does indeed use a browser to run an applet, but the look and feel is that of a stand-
alone program with no interaction with a browser.
ch01_v2.fm Page 5 Saturday, February 10, 2007 1:13 PM
评论0