# TA-Lib

This is a Python wrapper for [TA-LIB](http://ta-lib.org) based on Cython
instead of SWIG. From the homepage:
> TA-Lib is widely used by trading software developers requiring to perform
> technical analysis of financial market data.
>
> * Includes 150+ indicators such as ADX, MACD, RSI, Stochastic, Bollinger
> Bands, etc.
> * Candlestick pattern recognition
> * Open-source API for C/C++, Java, Perl, Python and 100% Managed .NET
The original Python bindings included with TA-Lib use
[SWIG](http://swig.org) which unfortunately are difficult to install and
aren't as efficient as they could be. Therefore this project uses
[Cython](https://cython.org) and [Numpy](https://numpy.org) to efficiently
and cleanly bind to TA-Lib -- producing results 2-4 times faster than the
SWIG interface.
In addition, this project also supports the use of the
[Polars](https://www.pola.rs) and [Pandas](https://pandas.pydata.org)
libraries.
## Installation
You can install from PyPI:
```
$ python -m pip install TA-Lib
```
Or checkout the sources and run ``setup.py`` yourself:
```
$ python setup.py install
```
It also appears possible to install via
[Conda Forge](https://anaconda.org/conda-forge/ta-lib):
```
$ conda install -c conda-forge ta-lib
```
### Dependencies
To use TA-Lib for python, you need to have the
[TA-Lib](http://ta-lib.org/hdr_dw.html) already installed. You should
probably follow their installation directions for your platform, but some
suggestions are included below for reference.
> Some Conda Forge users have reported success installing the underlying TA-Lib C
> library using [the libta-lib package](https://anaconda.org/conda-forge/libta-lib):
>
> ``$ conda install -c conda-forge libta-lib``
##### Mac OS X
You can simply install using Homebrew:
```
$ brew install ta-lib
```
If you are using Apple Silicon, such as the M1 processors, and building mixed
architecture Homebrew projects, you might want to make sure it's being built
for your architecture:
```
$ arch -arm64 brew install ta-lib
```
And perhaps you can set these before installing with ``pip``:
```
$ export TA_INCLUDE_PATH="$(brew --prefix ta-lib)/include"
$ export TA_LIBRARY_PATH="$(brew --prefix ta-lib)/lib"
```
You might also find this helpful, particularly if you have tried several
different installations without success:
```
$ your-arm64-python -m pip install --no-cache-dir ta-lib
```
##### Windows
Download [ta-lib-0.4.0-msvc.zip](https://sourceforge.net/projects/ta-lib/files/ta-lib/0.4.0/ta-lib-0.4.0-msvc.zip/download)
and unzip to ``C:\ta-lib``.
> This is a 32-bit binary release. If you want to use 64-bit Python, you will
> need to build a 64-bit version of the library. Some unofficial instructions
> for building on 64-bit Windows 10 or Windows 11, here for reference:
>
> 1. Download and Unzip ``ta-lib-0.4.0-msvc.zip``
> 2. Move the Unzipped Folder ``ta-lib`` to ``C:\``
> 3. Download and Install Visual Studio Community (2015 or later)
> * Remember to Select ``[Visual C++]`` Feature
> 4. Build TA-Lib Library
> * From Windows Start Menu, Start ``[VS2015 x64 Native Tools Command
> Prompt]``
> * Move to ``C:\ta-lib\c\make\cdr\win32\msvc``
> * Build the Library ``nmake``
You might also try these unofficial windows binary wheels for both 32-bit
and 64-bit:
https://github.com/cgohlke/talib-build/
##### Linux
Download
[ta-lib-0.4.0-src.tar.gz](https://sourceforge.net/projects/ta-lib/files/ta-lib/0.4.0/ta-lib-0.4.0-src.tar.gz/download)
and:
```
$ tar -xzf ta-lib-0.4.0-src.tar.gz
$ cd ta-lib/
$ ./configure --prefix=/usr
$ make
$ sudo make install
```
> If you build ``TA-Lib`` using ``make -jX`` it will fail but that's OK!
> Simply rerun ``make -jX`` followed by ``[sudo] make install``.
Note: if your directory path includes spaces, the installation will probably
fail with ``No such file or directory`` errors.
### Troubleshooting
If you get a warning that looks like this:
```
setup.py:79: UserWarning: Cannot find ta-lib library, installation may fail.
warnings.warn('Cannot find ta-lib library, installation may fail.')
```
This typically means ``setup.py`` can't find the underlying ``TA-Lib``
library, a dependency which needs to be installed.
---
If you installed the underlying ``TA-Lib`` library with a custom prefix
(e.g., with ``./configure --prefix=$PREFIX``), then when you go to install
this python wrapper you can specify additional search paths to find the
library and include files for the underlying ``TA-Lib`` library using the
``TA_LIBRARY_PATH`` and ``TA_INCLUDE_PATH`` environment variables:
```sh
$ export TA_LIBRARY_PATH=$PREFIX/lib
$ export TA_INCLUDE_PATH=$PREFIX/include
$ python setup.py install # or pip install ta-lib
```
---
Sometimes installation will produce build errors like this:
```
talib/_ta_lib.c:601:10: fatal error: ta-lib/ta_defs.h: No such file or directory
601 | #include "ta-lib/ta_defs.h"
| ^~~~~~~~~~~~~~~~~~
compilation terminated.
```
or:
```
common.obj : error LNK2001: unresolved external symbol TA_SetUnstablePeriod
common.obj : error LNK2001: unresolved external symbol TA_Shutdown
common.obj : error LNK2001: unresolved external symbol TA_Initialize
common.obj : error LNK2001: unresolved external symbol TA_GetUnstablePeriod
common.obj : error LNK2001: unresolved external symbol TA_GetVersionString
```
This typically means that it can't find the underlying ``TA-Lib`` library, a
dependency which needs to be installed. On Windows, this could be caused by
installing the 32-bit binary distribution of the underlying ``TA-Lib`` library,
but trying to use it with 64-bit Python.
---
Sometimes installation will fail with errors like this:
```
talib/common.c:8:22: fatal error: pyconfig.h: No such file or directory
#include "pyconfig.h"
^
compilation terminated.
error: command 'x86_64-linux-gnu-gcc' failed with exit status 1
```
This typically means that you need the Python headers, and should run
something like:
```
$ sudo apt-get install python3-dev
```
---
Sometimes building the underlying ``TA-Lib`` library has errors running
``make`` that look like this:
```
../libtool: line 1717: cd: .libs/libta_lib.lax/libta_abstract.a: No such file or directory
make[2]: *** [libta_lib.la] Error 1
make[1]: *** [all-recursive] Error 1
make: *** [all-recursive] Error 1
```
This might mean that the directory path to the underlying ``TA-Lib`` library
has spaces in the directory names. Try putting it in a path that does not have
any spaces and trying again.
---
Sometimes you might get this error running ``setup.py``:
```
/usr/include/limits.h:26:10: fatal error: bits/libc-header-start.h: No such file or directory
#include <bits/libc-header-start.h>
^~~~~~~~~~~~~~~~~~~~~~~~~~
```
This is likely an issue with trying to compile for 32-bit platform but
without the appropriate headers. You might find some success looking at the
first answer to [this question](https://stackoverflow.com/questions/54082459/fatal-error-bits-libc-header-start-h-no-such-file-or-directory-while-compili).
---
If you get an error on macOS like this:
```
code signature in <141BC883-189B-322C-AE90-CBF6B5206F67>
'python3.9/site-packages/talib/_ta_lib.cpython-39-darwin.so' not valid for
use in process: Trying to load an unsigned library)
```
You might look at [this question](https://stackoverflow.com/questions/69610572/how-can-i-solve-the-below-error-while-importing-nltk-package)
and use ``xcrun codesign`` to fix it.
---
If you wonder why ``STOCHRSI`` gives you different results than you expect,
probably you want ``STOCH`` applied to ``RSI``, which is a little different
than the ``STOCHRSI`` which is ``STOCHF`` applied to ``RSI``:
```python
>>> import talib
>>> import numpy as np
>>> c = np.random.randn(100)
# this is the library function
>>> k, d = talib.STOCHRSI(c)
# this produces the same result, calling ST
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
安装方法 TA-Lib的安装方法因操作系统和编程语言的不同而有所差异。以下是在Windows系统上使用Python安装TA-Lib的示例步骤: 下载TA-Lib的源代码或预编译包,并解压到指定目录。 安装Visual Studio等必要的开发工具,并确保勾选了Visual C++功能。 使用Visual Studio的命令提示符工具,进入TA-Lib的源代码目录,并执行构建命令(如nmake)来生成TA-Lib的动态链接库(DLL)文件。 将生成的DLL文件复制到Python的库目录中,或者将其路径添加到系统的环境变量中。 使用pip命令安装TA-Lib的Python接口包(如pip install TA-Lib)。
资源推荐
资源详情
资源评论
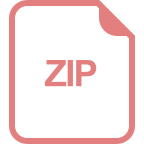
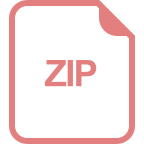
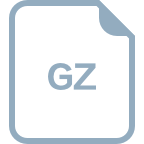
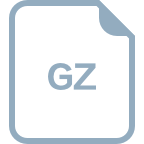
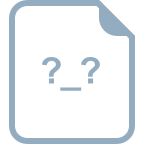
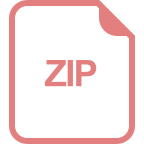
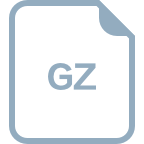
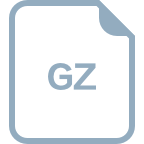
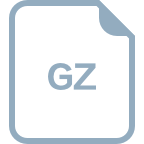
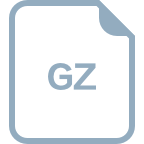
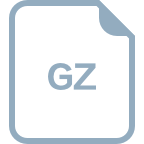
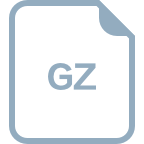
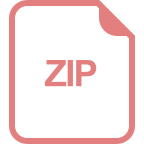
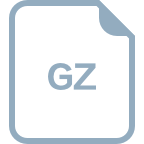
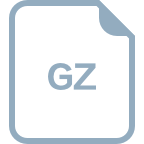
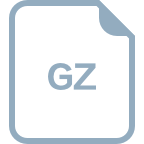
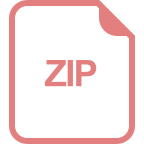
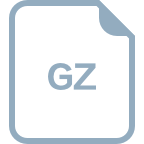
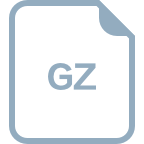
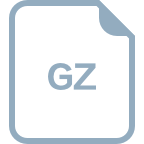
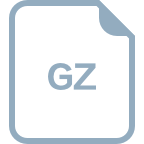
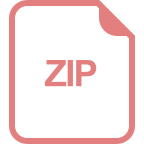
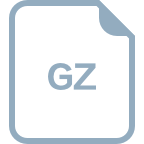
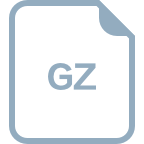
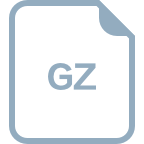
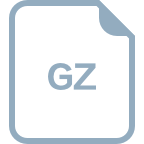
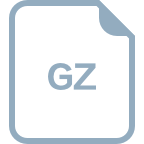
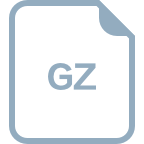
收起资源包目录


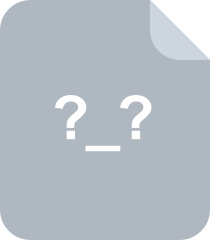
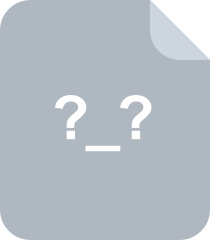
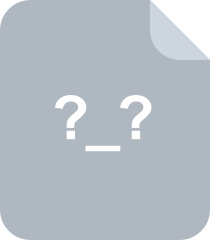
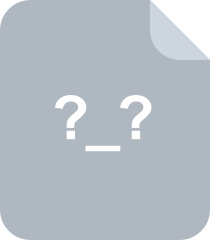
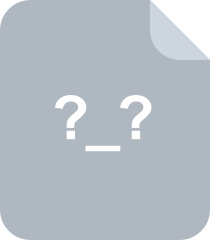

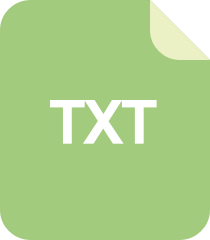
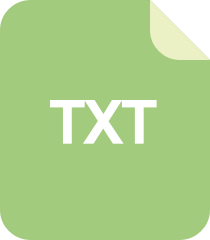
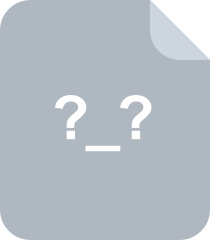
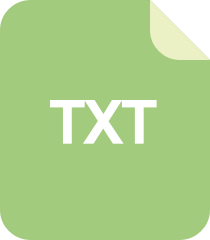
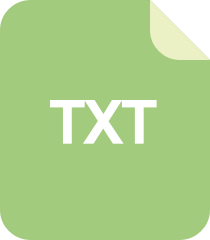

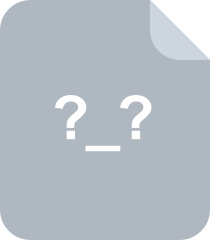
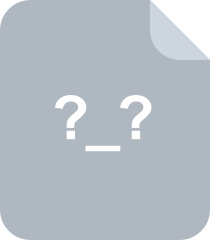
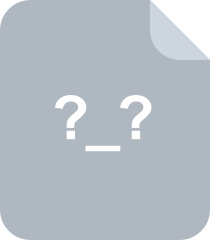
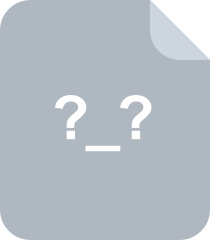
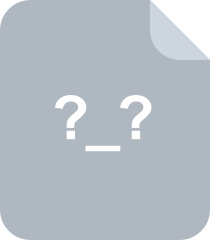
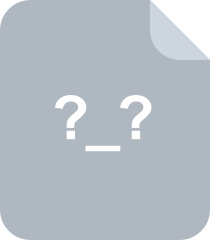
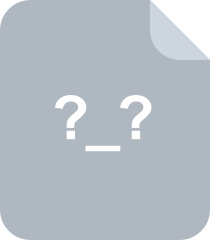
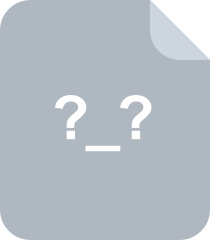
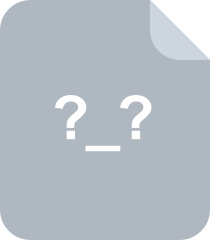

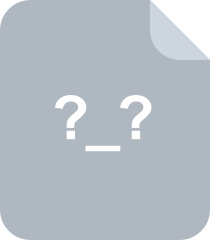
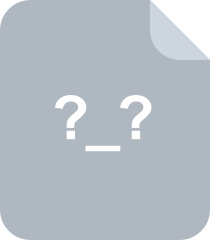
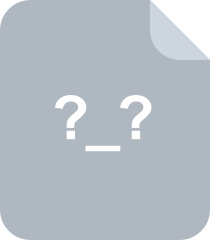
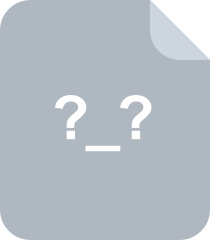
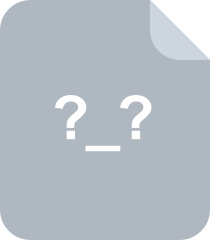
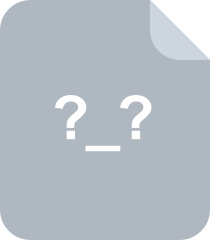
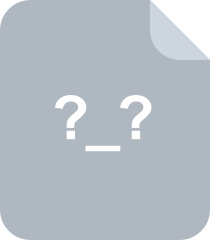
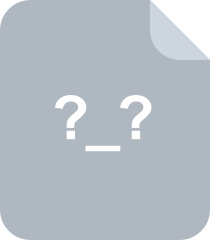
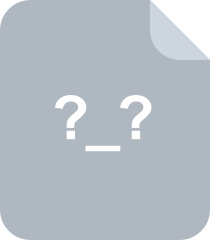
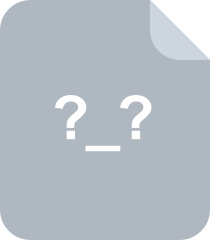
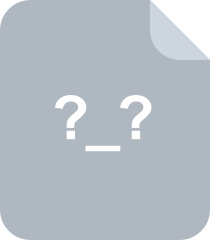
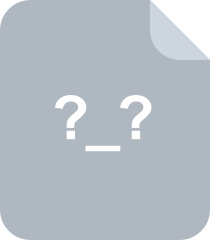
共 31 条
- 1
资源评论
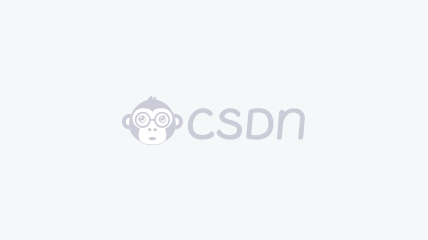
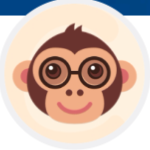
onnx
- 粉丝: 9782
- 资源: 5615
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

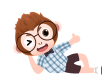
最新资源
- 基于微信小程序的医院挂号系统的开题报告.docx
- 基于微信小程序的在线课堂微信小程序的开题报告.docx
- 基于微信小程序的智能招聘小程序设计的开题报告.docx
- 基于微信小程序的在线选课系统springboot开题.docx
- 基于MLP、RNN、LSTM的锂电池寿命预测Python完整源码+数据集(高分项目)
- 基于微信小程序的懂球短视频微信小程序的开题报告.docx
- 基于微信小程序的电影院订票选座系统的开题报告.docx
- 基于微信小程序的便捷饭店点餐小程序的开题报告.docx
- 基于微信小程序的健身小程序的开题报告.docx
- 基于微信小程序的高校教师成果管理小程序的开题报告.docx
- 基于微信小程序的高校体育场管理系统的开题报告 =.docx
- 基于微信小程序的居住证申报系统的开题报告.docx
- 基于微信小程序的社区团购的开题报告 .docx
- 基于微信小程序的明星应援系统的开题报告.docx
- 基于微信小程序的宿舍管理系统的小程序开题.docx
- 基于微信小程序的实验室管理微信小程序的开题报告.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


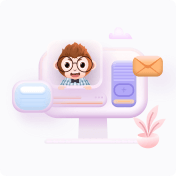
安全验证
文档复制为VIP权益,开通VIP直接复制
