from django.shortcuts import render
from django.shortcuts import HttpResponse
from django.utils import timezone
import json
import traceback
from hoimsystem.models.roleUser import *
from hoimsystem.models.adminOp import *
from hoimsystem.models.doctorOp import *
from hoimsystem.models.roleUser import *
from hoimsystem.models.patientOp import *
# Create your views here.
# 测试api
def test(request):
response = {"code": 200, "msg": 'success'}
return HttpResponse(json.dumps(response))
# 医生注册
def add_doctor(request):
received_data = json.loads(request.body.decode())
username = received_data.get('username')
if users.objects.filter(username=username).exists():
response = {"code": 500, "msg": '已存在相同用户'}
return HttpResponse(json.dumps(response))
password = received_data.get('password')
name = received_data.get('name')
title = received_data.get('title')
if received_data.get('sex') == '女':
sex = 0
else:
sex = 1
phone = received_data.get('phone')
department_in = department.objects.get(department_id=received_data.get('department'))
permission = received_data.get('permission')
education = received_data.get('education')
try:
# 判断是否为科室主任
if permission == 'director':
users.objects.create(username=username, password=password, user_role="director")
else:
users.objects.create(username=username, password=password, user_role="doctor")
user_id = users.objects.get(username=username)
# 在医生表中插入记录
doctor.objects.create(name=name, sex=sex, title=title, education=education, phone=phone, permission=permission, department_id=department_in, user_id=user_id)
response = {"code": 200, "msg": 'success'}
except:
traceback.print_exc()
response = {"code": 500, "msg": '医生注册失败'}
return HttpResponse(json.dumps(response))
# 医生排班
def doctor_schedule_register(request):
received_data = json.loads(request.body.decode())
schedule_list = received_data.get('schedule')
specialist = received_data.get('specialist')
number = received_data.get('number')
doctor_obj = doctor.objects.get(doctor_id=received_data.get('doctor'))
for i in schedule_list:
week = i[0:3]
time = i[3:5]
if doctor_schedule.objects.filter(week=week, time=time, doctor_id=doctor_obj).exists():
doctor_schedule_obj = doctor_schedule.objects.get(week=week, time=time, doctor_id=doctor_obj)
doctor_schedule_obj.number = number
doctor_schedule_obj.specialist = specialist
doctor_schedule_obj.save()
else:
doctor_schedule.objects.create(week=week, time=time, number=number, specialist=specialist, doctor_id=doctor_obj)
response = {"code": 200, "msg": 'success'}
return HttpResponse(json.dumps(response))
# 医生排班信息查看
def doctor_schedule_getlist(request):
token = users.objects.get(username=request.META.get('HTTP_ACCESSTOKEN')).user_role
data = []
if token == 'admin' or token == 'patient':
schedule_list = doctor_schedule.objects.all()
doctor_list = doctor.objects.all()
for i in doctor_list:
schedule_list = doctor_schedule.objects.filter(doctor_id=i.doctor_id)
schedule = []
for j in schedule_list:
schedule.append(j.week[2] + j.time[0])
data.append({
'id': i.doctor_id,
'name': i.name,
'schedule': schedule,
'number': j.number,
'specialist': j.specialist
})
elif token == 'doctor' or token == 'director':
token = users.objects.get(username=request.META.get('HTTP_ACCESSTOKEN')).user_role
doctor_obj = doctor.objects.get(user_id=users.objects.get(username=request.META.get('HTTP_ACCESSTOKEN')))
schedule_list = doctor_schedule.objects.filter(doctor_id=doctor_obj.doctor_id)
schedule = []
for i in schedule_list:
schedule.append(i.week[2] + i.time[0])
data.append({
'id': doctor_obj.doctor_id,
'name': doctor_obj.name,
'schedule': schedule
})
response = {"code": 200, "msg": 'success', 'data': data}
return HttpResponse(json.dumps(response))
# 药品注册
def pharmaceutical_register(request):
received_data = json.loads(request.body.decode())
name = received_data.get('name')
stock = received_data.get('stock')
price = float(received_data.get('price'))
expireddate = received_data.get('expireddate')
purchasing_time = timezone.now()
supplier = received_data.get('supplier')
remark = received_data.get('remark')
pharmaceutical.objects.create(name=name, stock=stock, price=price, expireddate=expireddate, purchasing_time=purchasing_time, supplier=supplier, remark=remark)
response = {"code": 200, "msg": 'success'}
return HttpResponse(json.dumps(response))
# 药品信息
def get_pharmaceutical_list(request):
pharmaceutical_list = pharmaceutical.objects.all()
data = []
for item in pharmaceutical_list:
data.append({
'id': item.pharmaceutical_id,
'name': item.name,
'stock': item.stock,
'price': item.price,
'expireddate': str(item.expireddate),
'purchasing_time': str(item.purchasing_time),
'supplier': item.supplier,
'remark': item.remark
})
response = {"code": 200, "msg": 'success', "data": data}
return HttpResponse(json.dumps(response))
# 药品处方查询
def pharmaceutical_stock_query(request):
received_data = json.loads(request.body.decode())
pharmaceutical_id = received_data.get('id')
stock_status = pharmaceutical.objects.get(pharmaceutical_id=pharmaceutical_id).stock
data = {"stock": stock_status}
response = {"code": 200, "msg": 'success', "data": data}
return HttpResponse(json.dumps(response))
# 处方注册
def prescription_register(request):
received_data = json.loads(request.body.decode())
doctor_uid = users.objects.get(username=request.META.get('HTTP_ACCESSTOKEN')).user_id
doctor_obj = doctor.objects.get(user_id=doctor_uid)
patient_id = received_data.get('patient')
patient_obj = patient.objects.get(patient_id=patient_id)
pre = prescription.objects.create(patient_id=patient_obj, doctor_id=doctor_obj)
prescription_obj = prescription.objects.get(prescription_id=pre.prescription_id)
phas = received_data.get('phas')
amount = 0
for item in phas:
pharmaceutical_obj = pharmaceutical.objects.get(pharmaceutical_id=item['id'])
pharmaceutical_obj.stock -= int(item['number'])
pharmaceutical_obj.save()
pre_pha.objects.create(prescription_id=pre.prescription_id, pharmaceutical_id=pharmaceutical_obj, number=item['number'])
price = pharmaceutical.objects.get(pharmaceutical_id=item['id']).price
amount = amount + float(price) * int(item['number'])
print(amount)
charge.objects.create(charge_time=timezone.now(), time="1970-1-1", prescription_id=prescription_obj, amount=amount, status=0)
response = {"code": 200, "msg": 'success'}
return HttpResponse(json.dumps(response))
# 获取处方信息
def get_prescription_list(request):
role = users.objects.get(username=request.META.get('HTTP_ACCESSTOKEN')).user_role
username = users.objects.get(username=request.META.get('HTTP_ACCESSTOKEN')).username
data = []
if role == 'admin':
prescriptions = prescription.objects.all()
for item in prescriptions:
doctor_id = item.doctor_id.doctor_id
doctor_name = item.doctor_id.name
patient_id = item.patient_id.patient_id
patient_name = item.patient_id.name
phas = []
phas_raw = p
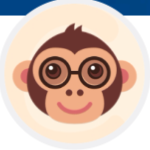
onnx
- 粉丝: 1w+
- 资源: 5627
最新资源
- 锅炉控制体系:西门子PLC与昆仑触摸屏的集成管理与CAD电气图纸指导下的精准调控,锅炉控制系统,西门子200smartPLC程序,昆仑触摸屏程序,带CAD电气图纸 ,核心关键词:锅炉控制系统; 西门子
- Maxwell电机模型:完整设计可运行,峰值功率达120kw,损耗计算与温度场分析功能强大,Maxwell电机模型,电机设计,电机设计,模型完整可以运行,峰值功率120kw,额定功率80kw,可以计算
- FLUENT模拟仿真分析树形流道设计在质子交换膜燃料电池中的应用探究,FLUENT模拟仿真树形流道质子交膜燃料电池 ,核心关键词:FLUENT模拟; 树形流道; 质子交换膜; 燃料电池; 仿真 ,"F
- FPGA系统下的JPEG-LS图像压缩方案:可配置无损与有损压缩,含工程源码及仿真测试报告,FPGA实现JPEG-LS图像压缩 FPGA实现JPEG-LS图像压缩,有损无损可配置,提供工程源码和 本设
- 三相不平衡潮流计算Matlab程序:采用前推回代法模拟三相不平衡模型及互阻抗分析,三相不平衡潮流计算matlab 本程序采用前推回代法,考虑三相不平衡和互阻抗,可通过改变三相负荷和线路参数构建三相不平
- Matlab鲸鱼优化算法:含23目标函数模板,自定义数据灵活调整与应用,Matlab程序,鲸鱼优化算法(WOA),有23个目标函数,根据自己需求修改,修改自己数据即可使用 ,Matlab程序; 鲸
- 关于光伏并网中单相与三相逆变及lcl仿真对配电网继电保护影响的研究,光伏并网 单相 三相 逆变 lcl 仿真 光伏对配电网继电保护影响 ,核心关键词:光伏并网; 单相与三相; 逆变技术; LCL滤波
- 三菱PLC与组态王联合打造图书馆智能借还书控制系统,基于三菱PLC和组态王组态图书馆借还书的智能控制系统 ,基于三菱PLC;组态王组态;借还书;智能控制系统,基于三菱PLC与组态王智能控制的图书馆借还
- 光伏储能与三相并离网逆变切换运行模型:Boost、Buck-boost双向DCDC控制、PQ与VF控制策略及孤岛检测自动切换技术笔记,光伏储能+三相并离网逆变切运行模型含笔记 包含Boost、Bu
- 储能变流器:高效功率双向流动,含DCDC和DCAC两大功能模块,并网与离网皆可,灵活模型轻松拓展使用,储能变流器(双向) 包含dcdc?dcac两部分 功率双向流动 可并网,也可改为离网状态下带三相负
- 内置式MTPA控制模型:速度环输出转矩,两种求解dq给定电流方法(工程近似与求解MTPA方程),与id=0控制比较分析,该模型是内置式的MTPA控制,速度环的输出为给定转矩,然后方式1通过求解MTPA
- STM32F系列兼容西门子S7 200 PLC源码开发详解:基于STM32F103RCT6芯片与Keil MDK5开发环境,STM32F系列兼容西门子S7 200PLC源码 CPU:STM32F
- 模拟IC设计入门:SMIC 0.18um锁相环电路仿真及400MHz锁定频率VCO实践,模拟ic设计,smic0.18um的锁相环电路,较简单的结构,适合入门学习,可以直接仿真,输出结果较为理想,锁定
- IEEE69节点配电网Simulink模型:新能源设备与无功补偿设备集成应用探索,IEEE69节点配电网simulink模型,可以加入风机光伏等新能源设备,SVC等无功补偿设备 ,核心关键词:IEEE
- 拓展卡尔曼滤波算法的应用原理与优化实践-高精度数据处理的关键技术,扩展卡尔曼滤波算法 ,核心关键词:扩展卡尔曼滤波算法; 滤波; 估计; 状态; 算法优化; 动态系统; 噪声处理; 参数估计; 预测
- 同相载波层叠三电平逆变器窄脉冲剔除仿真研究:电路参数与波形图分析,同相载波层叠的三电平逆变器窄脉冲直接剔除仿真 已知电路参数,下图为仿真模型和窄脉冲剔除前后的输出波形图 ,同相载波层叠;三电平逆变器
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


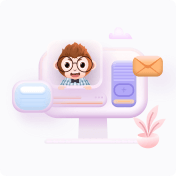