from langchain.llms.base import LLM
from langchain.callbacks.manager import CallbackManagerForLLMRun
from langchain.callbacks.streaming_stdout import StreamingStdOutCallbackHandler
from functools import partial
from typing import List, Optional, Mapping, Any
import openai
# 基于vllm推理
class LL:
def __init__(self, model, api_base=None, api_key=None):
self.model = model
self.api_base = 'http://192.168.204.120:7000/v1'
self.api_key = 'EMPTY'
def stream_chat(
self,
prompt,
history=[],
max_length=3096,
top_p=0.7,
temperature=0.1
):
openai.api_key = self.api_key
openai.api_base = self.api_base
completion = openai.Completion.create(
model=self.model,
prompt=prompt,
stream=False,
stop=["</s>", '<|im_end|>', '<|endoftext|>'],
temperature=temperature,
use_beam_search=False, n=1, frequency_penalty=1.0, best_of=1,
presence_penalty=1.0, top_p=top_p, top_k=1, max_tokens=max_length
)
response = completion['choices'][0]['text']
history.append([prompt, response])
yield response, history
def chat(self, prompt,
history=[],
max_length=3096,
top_p=0.7,
temperature=0.1
):
openai.api_key = self.api_key
openai.api_base = self.api_base
completion = openai.Completion.create(
model=self.model,
prompt=prompt,
stream=False,
stop=["</s>", '<|im_end|>', '<|endoftext|>'],
temperature=temperature,
use_beam_search=False, n=1, frequency_penalty=1.0, best_of=1,
presence_penalty=1.0, top_p=top_p, top_k=1, max_tokens=max_length
)
respose = completion['choices'][0]['text']
history.append([prompt, respose])
return respose, history
class LLMs(LLM):
model_path: str
max_length: int = 2048
temperature: float = 0.1
top_p: float = 0.7
history: List = []
streaming: bool = True
model: object = None
@property
def _llm_type(self) -> str:
return "llm"
@property
def _identifying_params(self) -> Mapping[str, Any]:
"""Get the identifying parameters."""
return {
"model_path": self.model_path,
"max_length": self.max_length,
"temperature": self.temperature,
"top_p": self.top_p,
"history": [],
"streaming": self.streaming
}
def _call(
self,
prompt: str,
stop: Optional[List[str]] = None,
run_manager: Optional[CallbackManagerForLLMRun] = None,
add_history: bool = False
) -> str:
if self.model is None:
raise RuntimeError("Must call `load_model()` to load model and tokenizer!")
if self.streaming:
text_callback = partial(StreamingStdOutCallbackHandler().on_llm_new_token, verbose=True)
resp = self.generate_resp(prompt, text_callback, add_history=add_history)
else:
resp = self.generate_resp(self, prompt, add_history=add_history)
return resp
def generate_resp(self, prompt, text_callback=None, add_history=True):
resp = ""
index = 0
if text_callback:
for i, (resp, _) in enumerate(self.model.stream_chat(
prompt,
self.history,
max_length=self.max_length,
top_p=self.top_p,
temperature=self.temperature
)):
if add_history:
if i == 0:
self.history += [[prompt, resp]]
else:
self.history[-1] = [prompt, resp]
text_callback(resp[index:])
index = len(resp)
else:
resp, _ = self.model.chat(
prompt,
self.history,
max_length=self.max_length,
top_p=self.top_p,
temperature=self.temperature
)
if add_history:
self.history += [[prompt, resp]]
return resp
def load_model(self):
if self.model is not None:
return
self.model = LL(self.model_path)
def set_params(self, **kwargs):
for k, v in kwargs.items():
if k in self._identifying_params:
self.k = v
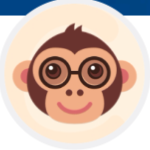
onnx
- 粉丝: 1w+
- 资源: 5626
最新资源
- 基于matlab的传统滤波、Butterworth滤波、FIR、移动平均滤波、中值滤波、现代滤波、维纳滤波、自适应滤波、小波变,七种滤波方法,可替自己的数据进行滤波,程序已调通,可直接运行
- 基于Java语言开发的ASR+TTS+声纹识别功能的智能聊天小程序设计源码
- 含风电-光伏-光热电站电力系统N-k安全优化调度模型 关键词:N-K安全约束 光热电站 优化调度 参考文档:参考《光热电站促进风电消纳的电力系统优化调度》光热电站模型; 仿真软件: matlab+y
- 基于TypeScript和JavaScript的每日饮食与运动记录工具设计源码
- 基于JavaScript的仪器预约系统设计源码
- 基于Vue的依沫一站式内容资源变现博客设计源码
- 基于SSM框架与微信小程序的宠物管理系统源码设计
- 基于宝塔Linux面板7.9.0免费版的7.9.2兼容CSS美化设计源码
- 基于ActiveReports的C#报表控件设计源码
- 基于C#与Shell语言的SangServerTool服务器DDNS与SSL证书申请工具设计源码
- 基于SpringBoot+Vue的智能停车场管理系统设计源码
- 基于Shell、Python、PHP、HTML的zzxia-op-super-invincible-lollipop代码构建部署运维工具箱设计源码
- 华为FusionCompute 8.0.1 集成设计指导书
- 基于C语言实现的新型疫苗接种管理系统设计源码
- 基于JavaScript和微信小程序的抖音本地生活团购系统源码搭建与部署方案
- 电力电子boost升压电路MATLAB仿真,pi控制闭环(15r)滑模控制改进版(29r)24升48V,电压可修改 基于反激变器的升压电路,降压电路boost buck的MATLAB仿真,PLECS也
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


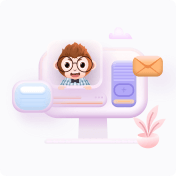