# Async.js
[](https://travis-ci.org/caolan/async)
[](https://www.npmjs.org/package/async)
[](https://coveralls.io/r/caolan/async?branch=master)
[](https://gitter.im/caolan/async?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
Async is a utility module which provides straight-forward, powerful functions
for working with asynchronous JavaScript. Although originally designed for
use with [Node.js](http://nodejs.org) and installable via `npm install async`,
it can also be used directly in the browser.
Async is also installable via:
- [bower](http://bower.io/): `bower install async`
- [component](https://github.com/component/component): `component install
caolan/async`
- [jam](http://jamjs.org/): `jam install async`
- [spm](http://spmjs.io/): `spm install async`
Async provides around 20 functions that include the usual 'functional'
suspects (`map`, `reduce`, `filter`, `each`…) as well as some common patterns
for asynchronous control flow (`parallel`, `series`, `waterfall`…). All these
functions assume you follow the Node.js convention of providing a single
callback as the last argument of your `async` function.
## Quick Examples
```javascript
async.map(['file1','file2','file3'], fs.stat, function(err, results){
// results is now an array of stats for each file
});
async.filter(['file1','file2','file3'], fs.exists, function(results){
// results now equals an array of the existing files
});
async.parallel([
function(){ ... },
function(){ ... }
], callback);
async.series([
function(){ ... },
function(){ ... }
]);
```
There are many more functions available so take a look at the docs below for a
full list. This module aims to be comprehensive, so if you feel anything is
missing please create a GitHub issue for it.
## Common Pitfalls <sub>[(StackOverflow)](http://stackoverflow.com/questions/tagged/async.js)</sub>
### Synchronous iteration functions
If you get an error like `RangeError: Maximum call stack size exceeded.` or other stack overflow issues when using async, you are likely using a synchronous iterator. By *synchronous* we mean a function that calls its callback on the same tick in the javascript event loop, without doing any I/O or using any timers. Calling many callbacks iteratively will quickly overflow the stack. If you run into this issue, just defer your callback with `async.setImmediate` to start a new call stack on the next tick of the event loop.
This can also arise by accident if you callback early in certain cases:
```js
async.eachSeries(hugeArray, function iterator(item, callback) {
if (inCache(item)) {
callback(null, cache[item]); // if many items are cached, you'll overflow
} else {
doSomeIO(item, callback);
}
}, function done() {
//...
});
```
Just change it to:
```js
async.eachSeries(hugeArray, function iterator(item, callback) {
if (inCache(item)) {
async.setImmediate(function () {
callback(null, cache[item]);
});
} else {
doSomeIO(item, callback);
//...
```
Async guards against synchronous functions in some, but not all, cases. If you are still running into stack overflows, you can defer as suggested above, or wrap functions with [`async.ensureAsync`](#ensureAsync) Functions that are asynchronous by their nature do not have this problem and don't need the extra callback deferral.
If JavaScript's event loop is still a bit nebulous, check out [this article](http://blog.carbonfive.com/2013/10/27/the-javascript-event-loop-explained/) or [this talk](http://2014.jsconf.eu/speakers/philip-roberts-what-the-heck-is-the-event-loop-anyway.html) for more detailed information about how it works.
### Multiple callbacks
Make sure to always `return` when calling a callback early, otherwise you will cause multiple callbacks and unpredictable behavior in many cases.
```js
async.waterfall([
function (callback) {
getSomething(options, function (err, result) {
if (err) {
callback(new Error("failed getting something:" + err.message));
// we should return here
}
// since we did not return, this callback still will be called and
// `processData` will be called twice
callback(null, result);
});
},
processData
], done)
```
It is always good practice to `return callback(err, result)` whenever a callback call is not the last statement of a function.
### Binding a context to an iterator
This section is really about `bind`, not about `async`. If you are wondering how to
make `async` execute your iterators in a given context, or are confused as to why
a method of another library isn't working as an iterator, study this example:
```js
// Here is a simple object with an (unnecessarily roundabout) squaring method
var AsyncSquaringLibrary = {
squareExponent: 2,
square: function(number, callback){
var result = Math.pow(number, this.squareExponent);
setTimeout(function(){
callback(null, result);
}, 200);
}
};
async.map([1, 2, 3], AsyncSquaringLibrary.square, function(err, result){
// result is [NaN, NaN, NaN]
// This fails because the `this.squareExponent` expression in the square
// function is not evaluated in the context of AsyncSquaringLibrary, and is
// therefore undefined.
});
async.map([1, 2, 3], AsyncSquaringLibrary.square.bind(AsyncSquaringLibrary), function(err, result){
// result is [1, 4, 9]
// With the help of bind we can attach a context to the iterator before
// passing it to async. Now the square function will be executed in its
// 'home' AsyncSquaringLibrary context and the value of `this.squareExponent`
// will be as expected.
});
```
## Download
The source is available for download from
[GitHub](https://github.com/caolan/async/blob/master/lib/async.js).
Alternatively, you can install using Node Package Manager (`npm`):
npm install async
As well as using Bower:
bower install async
__Development:__ [async.js](https://github.com/caolan/async/raw/master/lib/async.js) - 29.6kb Uncompressed
## In the Browser
So far it's been tested in IE6, IE7, IE8, FF3.6 and Chrome 5.
Usage:
```html
<script type="text/javascript" src="async.js"></script>
<script type="text/javascript">
async.map(data, asyncProcess, function(err, results){
alert(results);
});
</script>
```
## Documentation
Some functions are also available in the following forms:
* `<name>Series` - the same as `<name>` but runs only a single async operation at a time
* `<name>Limit` - the same as `<name>` but runs a maximum of `limit` async operations at a time
### Collections
* [`each`](#each), `eachSeries`, `eachLimit`
* [`forEachOf`](#forEachOf), `forEachOfSeries`, `forEachOfLimit`
* [`map`](#map), `mapSeries`, `mapLimit`
* [`filter`](#filter), `filterSeries`, `filterLimit`
* [`reject`](#reject), `rejectSeries`, `rejectLimit`
* [`reduce`](#reduce), [`reduceRight`](#reduceRight)
* [`detect`](#detect), `detectSeries`, `detectLimit`
* [`sortBy`](#sortBy)
* [`some`](#some), `someLimit`
* [`every`](#every), `everyLimit`
* [`concat`](#concat), `concatSeries`
### Control Flow
* [`series`](#seriestasks-callback)
* [`parallel`](#parallel), `parallelLimit`
* [`whilst`](#whilst), [`doWhilst`](#doWhilst)
* [`until`](#until), [`doUntil`](#doUntil)
* [`during`](#during), [`doDuring`](#doDuring)
* [`forever`](#forever)
* [`waterfall`](#waterfall)
* [`compose`](#compose)
* [`seq`](#seq)
* [`applyEach`](#applyEach), `applyEachSeries`
* [`queue`](#queue), [`priorityQueue`](#priorityQueue)
* [`cargo`](#cargo)
* [`auto`](#auto)
* [`retry`](#retry)
* [`iterator`](#iterator)
* [`times`](#times), `timesSeri
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于Node.js的艺恩票房数据可视化系统源码+sql数据库+使用说明.zip 【资源说明】 该项目是个人毕设项目源码,评审分达到95分,都经过严格调试,确保可以运行!放心下载使用。 该项目资源主要针对计算机、自动化等相关专业的学生或从业者下载使用,也可作为期末课程设计、课程大作业、毕业设计等。 具有较高的学习借鉴价值!基础能力强的可以在此基础上修改调整,以实现类似其他功能。 利用Python爬取电影数据,将数据存入MySQL,Node调用MySQL模块,操作SQL对数据进行存取;封装SQL函数,提高复用率;数据分类,以满足Echarts对数据格式的要求;使用Element-ui构建页面骨架,Vue渲染排行榜内容,渲染Echarts图表。 ## 项目演示 [http://119.29.141.196](http://119.29.141.196:3020) ## 快速上手 ### 环境安装 #### Nodejs安装: 官网:https://nodejs.org/en/ #### vue-cli安装: ``` npm install -g @vue/cli ``` ### Node服务 ``` node app.js ``` ### Vue启动 ``` npm run serve
资源推荐
资源详情
资源评论
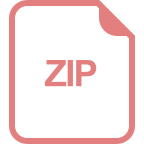
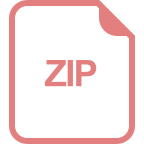
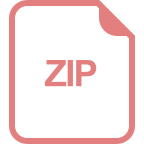
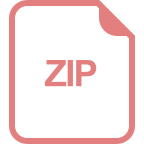
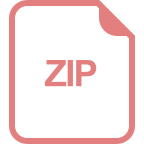
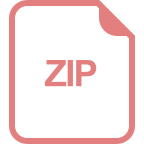
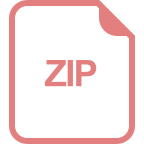
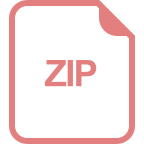
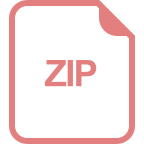
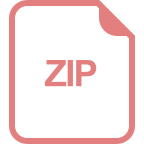
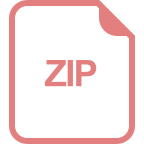
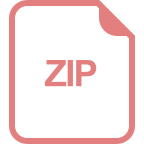
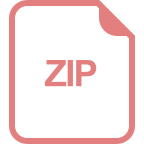
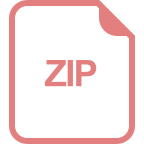
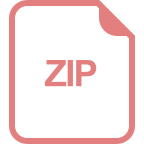
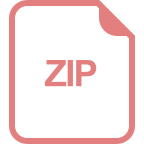
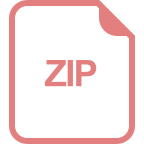
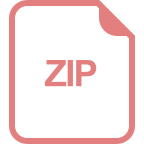
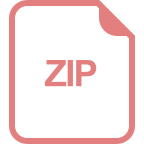
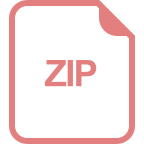
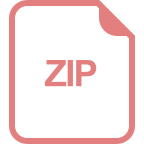
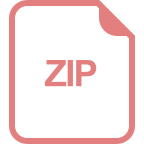
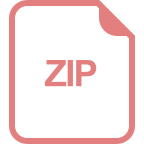
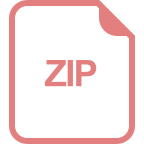
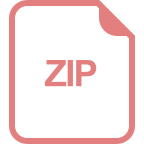
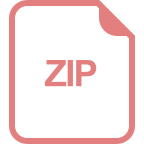
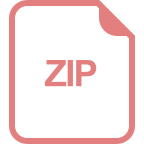
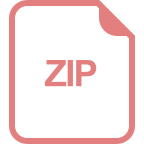
收起资源包目录

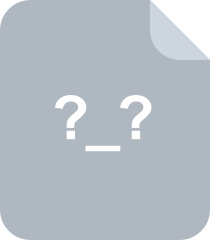
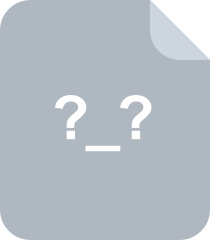
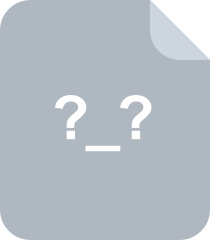
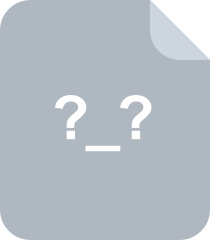
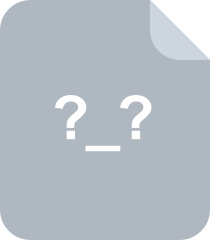
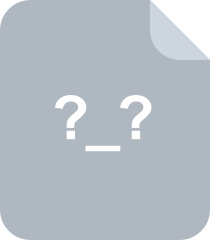
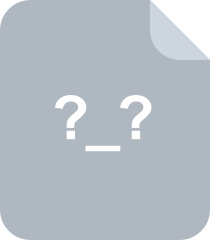
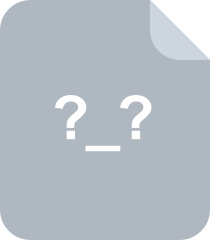
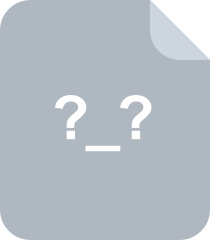
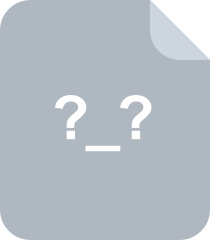
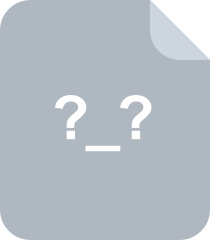
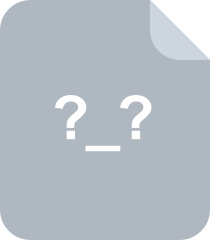
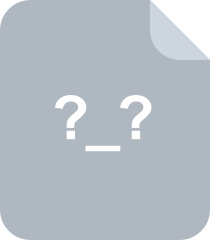
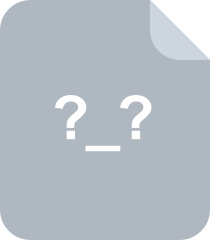
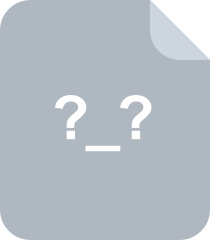

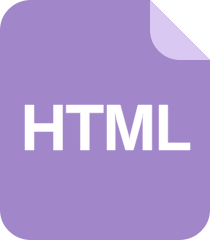
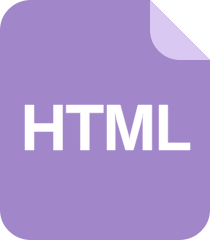
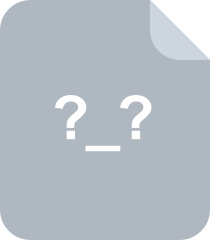
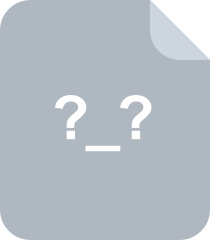
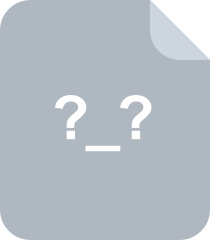
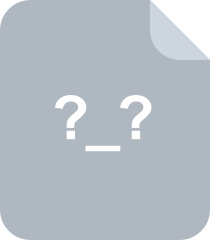
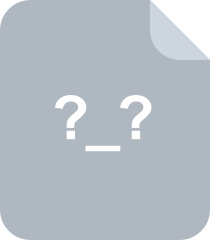
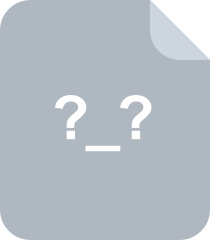
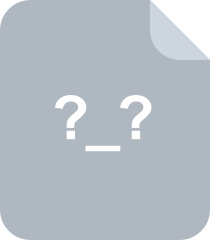
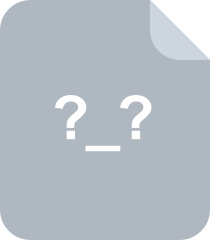
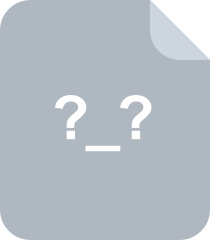
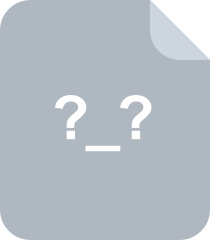
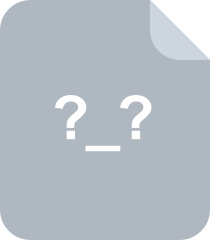
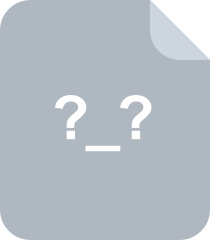
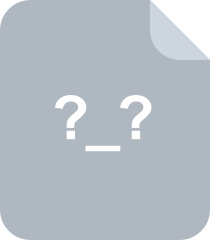
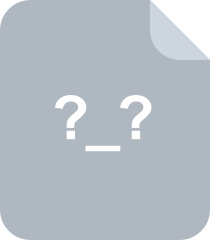
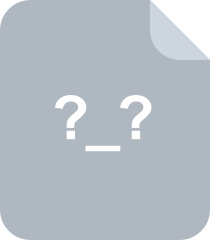
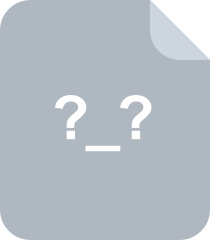
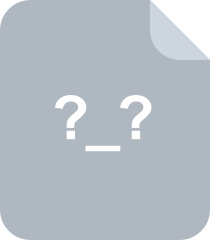
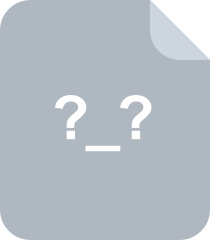
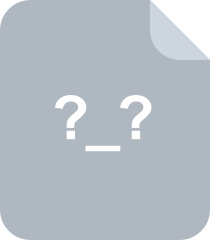
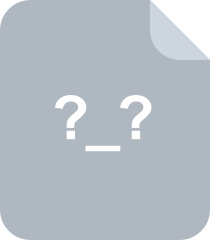
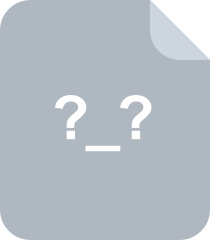
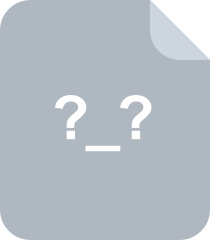
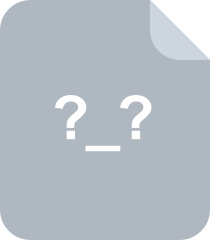
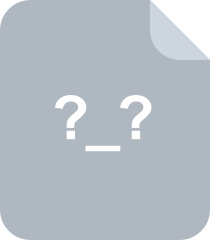
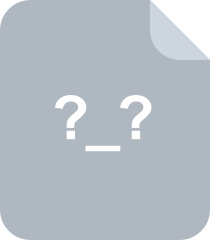
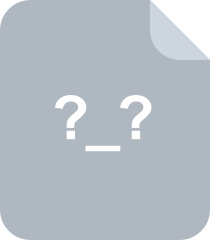
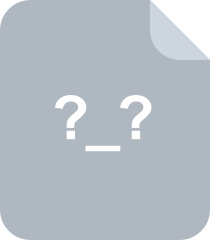
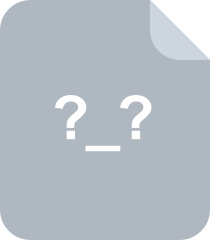
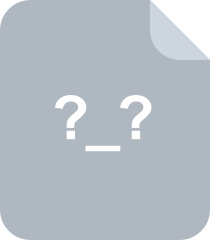
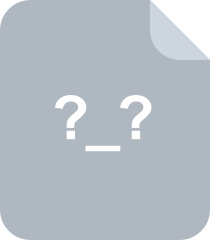
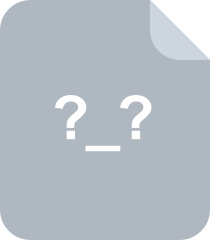
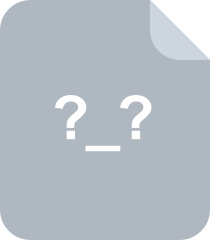
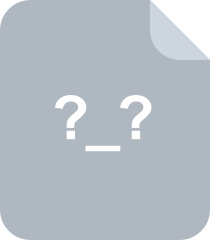
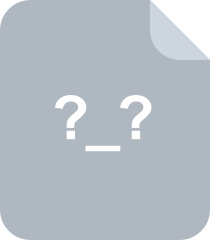
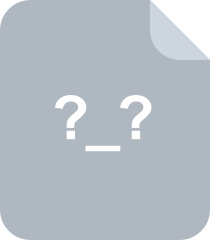
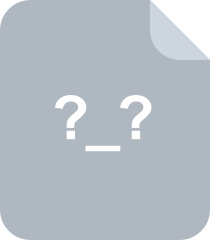
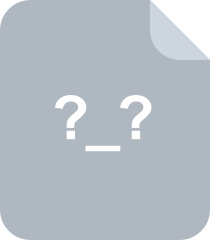
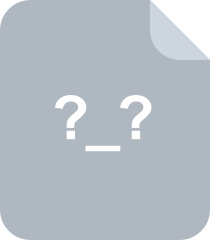
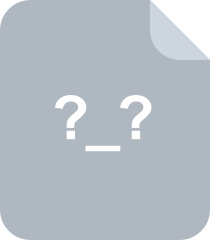
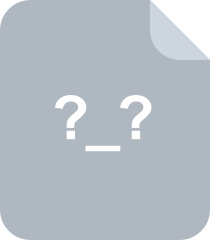
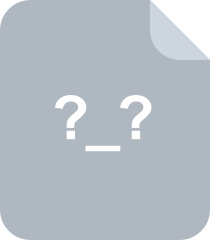
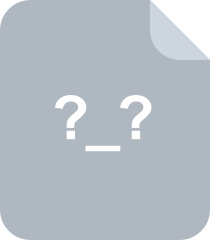
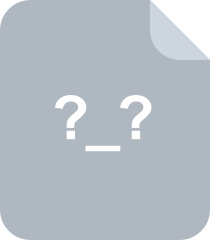
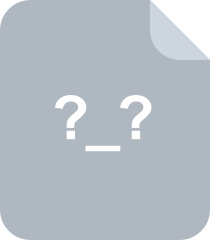
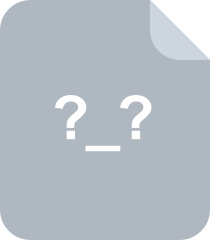
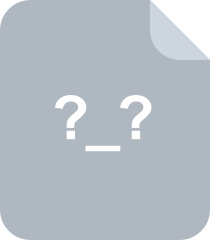
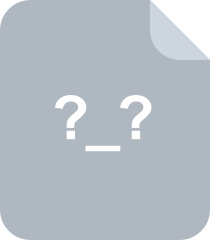
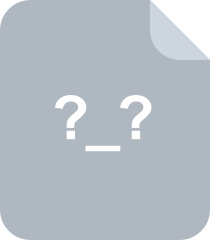
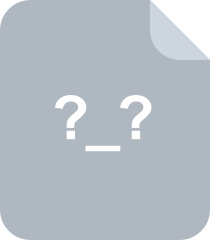
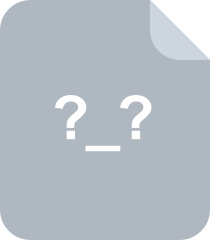
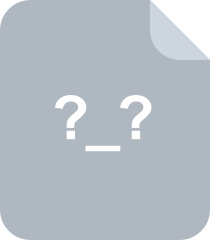
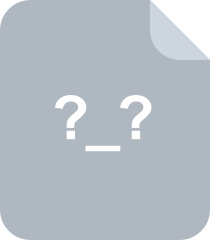
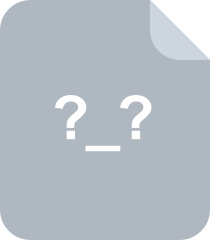
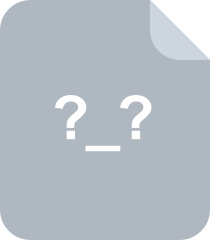
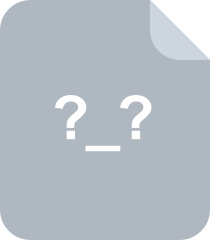
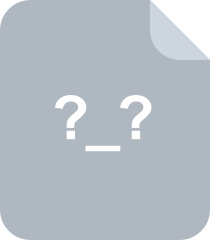
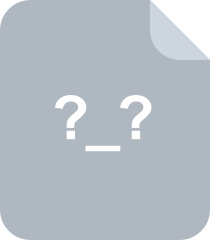
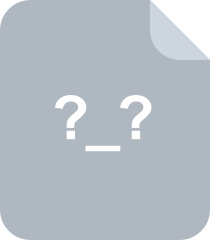
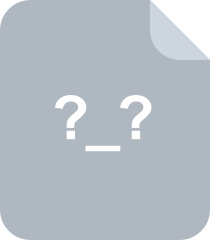
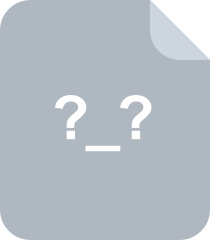
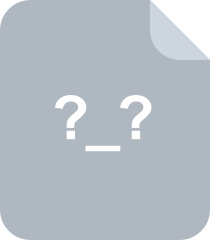
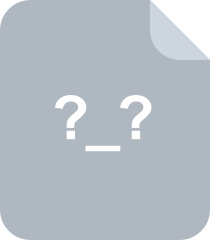
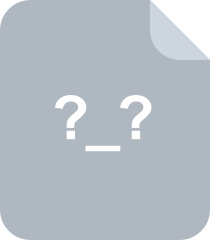
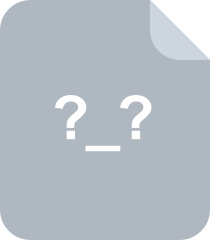
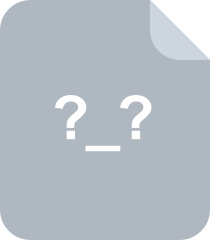
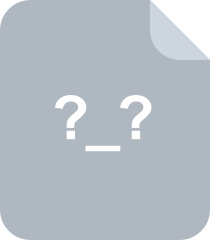
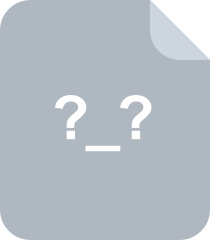
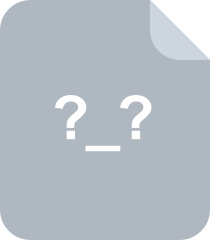
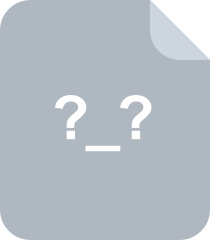
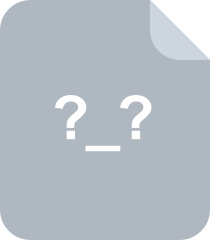
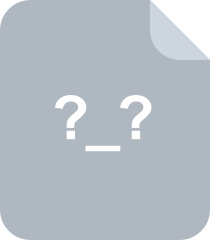
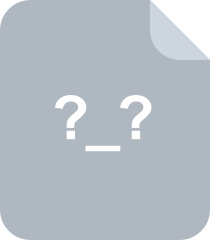
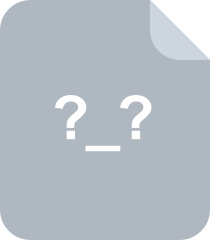
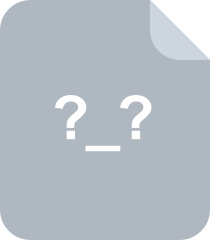
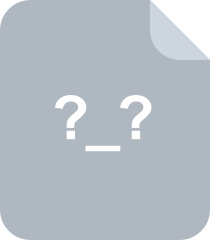
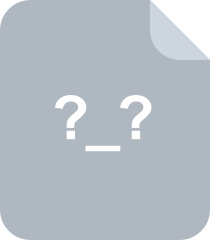
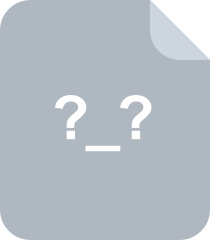
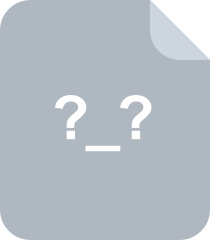
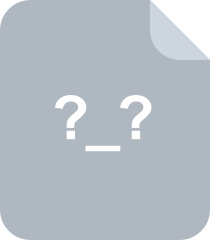
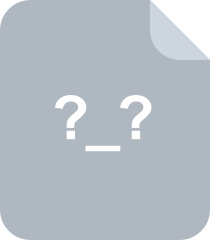
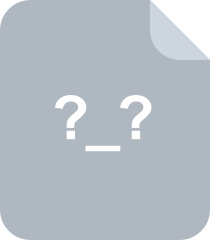
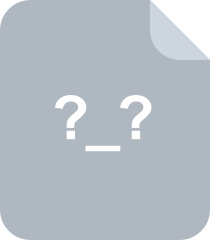
共 766 条
- 1
- 2
- 3
- 4
- 5
- 6
- 8
资源评论
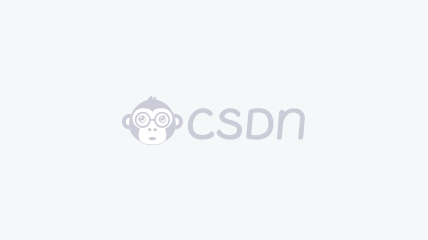
- ydjnxn06012024-04-27果断支持这个资源,资源解决了当前遇到的问题,给了新的灵感,感谢分享~
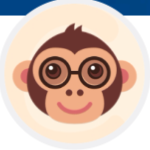
onnx
- 粉丝: 9640
- 资源: 5598
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

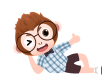
最新资源
- 技术资料分享TF卡资料很好的技术资料.zip
- 技术资料分享TF介绍很好的技术资料.zip
- 10、安徽省大学生学科和技能竞赛A、B类项目列表(2019年版).xlsx
- 9、教育主管部门公布学科竞赛(2015版)-方喻飞
- C语言-leetcode题解之83-remove-duplicates-from-sorted-list.c
- C语言-leetcode题解之79-word-search.c
- C语言-leetcode题解之78-subsets.c
- C语言-leetcode题解之75-sort-colors.c
- C语言-leetcode题解之74-search-a-2d-matrix.c
- C语言-leetcode题解之73-set-matrix-zeroes.c
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


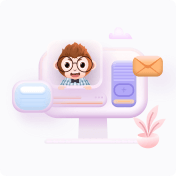
安全验证
文档复制为VIP权益,开通VIP直接复制
