package cn.vesns.sunweather.activity;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import androidx.core.content.ContextCompat;
import androidx.drawerlayout.widget.DrawerLayout;
import androidx.fragment.app.Fragment;
import androidx.fragment.app.FragmentPagerAdapter;
import androidx.swiperefreshlayout.widget.SwipeRefreshLayout;
import androidx.viewpager.widget.ViewPager;
import androidx.viewpager2.widget.ViewPager2;
import android.Manifest;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.os.Build;
import android.os.Bundle;
import android.util.Log;
import android.view.Gravity;
import android.view.LayoutInflater;
import android.view.MotionEvent;
import android.view.View;
import android.view.WindowManager;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.LinearLayout;
import android.widget.ProgressBar;
import android.widget.ScrollView;
import android.widget.TextView;
import com.bumptech.glide.Glide;
import com.google.gson.Gson;
import com.qweather.sdk.bean.IndicesBean;
import com.qweather.sdk.bean.air.AirNowBean;
import com.qweather.sdk.bean.base.Code;
import com.qweather.sdk.bean.base.IndicesType;
import com.qweather.sdk.bean.base.Lang;
import com.qweather.sdk.bean.geo.GeoBean;
import com.qweather.sdk.bean.weather.WeatherDailyBean;
import com.qweather.sdk.bean.weather.WeatherHourlyBean;
import com.qweather.sdk.bean.weather.WeatherNowBean;
import com.qweather.sdk.view.QWeather;
import java.io.IOException;
import java.util.AbstractList;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import cn.vesns.sunweather.R;
import cn.vesns.sunweather.adapter.ViewPagerAdapter;
import cn.vesns.sunweather.entity.City;
import cn.vesns.sunweather.entity.CityList;
import cn.vesns.sunweather.fragment.OneFragment;
import cn.vesns.sunweather.util.CommonUtil;
import cn.vesns.sunweather.util.DisplayUtil;
import cn.vesns.sunweather.util.HttpUtil;
import cn.vesns.sunweather.util.SpUtils;
import cn.vesns.sunweather.util.WeekUtil;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.Response;
/**
* created by vesns on 2021/06/06.
*/
public class WeatherActivity extends AppCompatActivity {
private static final String TAG = "WeatherActivity.class";
private final int REQUEST_GPS = 1;
private int mNum = 0;
private List<Fragment> fragments;
CityList cityList = new CityList();
private List<String> locaitons;
private List<String> cityIds;
private LinearLayout llRound;
public SwipeRefreshLayout swipeRefresh;
private ImageView navButton;
private TextView titleCity;
private TextView degreeText;
private TextView weatherInfoText;
private TextView cloudageText;
private TextView humidityText;
private LinearLayout forecastLayout;
private TextView comfortText;
private TextView carWashText;
private TextView sportText;
private ImageView backBingImg;
private ProgressBar progressBar;
private TextView progressBar_text;
private TextView gradle_air_text;
private TextView traffic_text;
private TextView fish_text;
private TextView travel_text;
private LinearLayout linearLayout;
private int[] image = {R.mipmap.cloudy, R.mipmap.fine, R.mipmap.rain, R.mipmap.thunder, R.mipmap.dawu, R.mipmap.haze, R.mipmap.fog};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_weather);
getWindow().addFlags(WindowManager.LayoutParams.FLAG_TRANSLUCENT_STATUS);
init();
// initFragment();
loadBingPic();
ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.ACCESS_FINE_LOCATION}, REQUEST_GPS);
swipeRefresh.setColorSchemeResources(R.color.colorPrimary);
requestWeather(CommonUtil.CITY_ID);
swipeRefresh.setOnRefreshListener(() -> {
requestWeather(CommonUtil.CITY_ID);
loadBingPic();
});
navButton.setOnClickListener(v->{
if (v.getId() == R.id.nav_button) {
startActivity(new Intent(this,SearchActivity.class));
}
});
}
public void init() {
swipeRefresh = findViewById(R.id.swipe_refresh);
titleCity = findViewById(R.id.title_city);
degreeText = findViewById(R.id.degree_text);
weatherInfoText = findViewById(R.id.weather_info_text);
cloudageText = findViewById(R.id.cloudage_text);
humidityText = findViewById(R.id.humidity_text);
forecastLayout = findViewById(R.id.forecast_layout);
comfortText = findViewById(R.id.comfort_text);
carWashText = findViewById(R.id.car_wash_text);
sportText = findViewById(R.id.sport_text);
backBingImg = findViewById(R.id.bing_pic_img);
swipeRefresh = findViewById(R.id.swipe_refresh);
swipeRefresh.setColorSchemeResources(R.color.colorPrimary);
// drawerLayout = findViewById(R.id.drawer_layout);
navButton = findViewById(R.id.nav_button);
progressBar = findViewById(R.id.progressBar);
progressBar_text = findViewById(R.id.progressBar_text);
gradle_air_text = findViewById(R.id.gradle_air);
linearLayout = findViewById(R.id.linear);
travel_text = findViewById(R.id.travel_text);
fish_text = findViewById(R.id.fish_text);
traffic_text = findViewById(R.id.traffic_text);
navButton = findViewById(R.id.nav_button);
llRound = findViewById(R.id.ll_round);
}
private void initFragment() {
cityList = SpUtils.getBean(WeatherActivity.this, "cityBean", CityList.class);
CityList cityBean = SpUtils.getBean(WeatherActivity.this, "cityBean", CityList.class);
locaitons = new ArrayList<>();
if (cityBean != null) {
for (City city : cityBean.getCityBeans()) {
String cityName = city.getCityName();
locaitons.add(cityName);
}
}
cityIds = new ArrayList<>();
fragments = new ArrayList<>();
}
/**
* 根据天气id差城市天气信息
*
* @param weatherId
*/
public void requestWeather(final String weatherId) {
/**
* 实况天气数据
* @param location 所查询的地区,可通过该地区名称、ID、IP和经纬度进行查询经纬度格式:经度,纬度
* (英文,分隔,十进制格式,北纬东经为正,南纬西经为负)
* @param lang (选填)多语言,可以不使用该参数,默认为简体中文
* @param unit (选填)单位选择,公制(m)或英制(i),默认为公制单位
* @param listener 网络访问结果回调
*/
QWeather.getGeoCityLookup(WeatherActivity.this, CommonUtil.CITY_INITIAL, new QWeather.OnResultGeoListener() {
@Override
public void onError(Throwable throwable) {
runOnUiThread(() -> {
// Toast.makeText(WeatherActivity.this, CommonUtil.CITY_INITIAL, Toast.LENGTH_SHORT).show();
swipeRefresh.setRefreshing(false);
});
Log.i(TAG, "getGeoCityLookup onError: " + throwable);
}
@Override
public void onSuccess(GeoBean geoBean) {
Log.i(TAG, "getGeoCityLookup onSuccess: " + new Gson().toJson(geoBean));
if (Code.OK == geoBean.getCode()) {
List<GeoBean.LocationBean> locationBean = geoBean.getLocationBean();
List<City> cityBeans = new ArrayList<>();
City cityBean = new City();
for (GeoBean.LocationBean bean : locationBean) {
cityBean.setCityName(bean.getName())
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
新开发项目_Android毕设基于Android开发的天气预报app系统java源码+项目说明 该项目主要针对计算机相关专业的正在做毕设的学生和需要项目实战的Java学习者。 也可作为课程设计、期末大作业。包含:项目源码、数据库脚本、项目说明等,该项目可以直接作为毕设使用,也可以参考学习借鉴! 【特别强调】 1、csdn上资源保证是完整最新,会不定期更新优化; 2、请用自己的账号在csdn官网下载,若通过第三方代下,博主不对您下载的资源作任何保证,且不提供任何形式的技术支持和答疑!!!
资源推荐
资源详情
资源评论
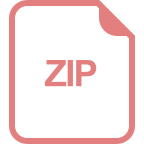
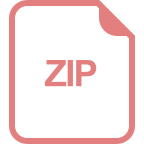
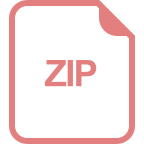
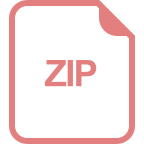
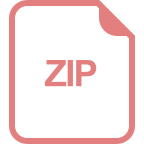
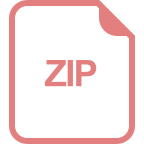
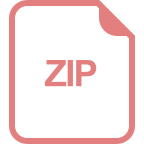
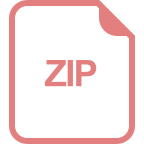
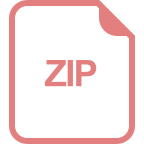
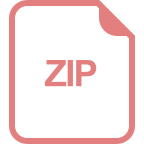
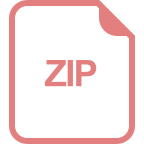
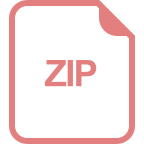
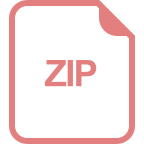
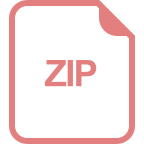
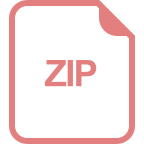
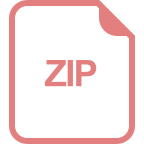
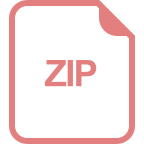
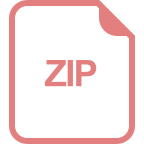
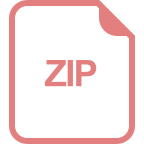
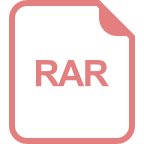
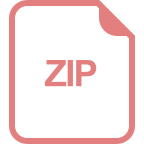
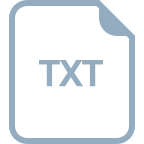
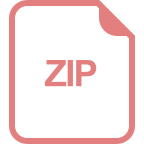
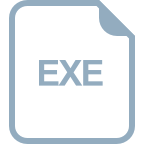
收起资源包目录

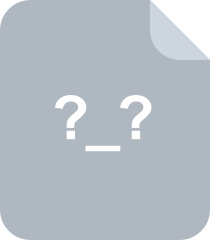
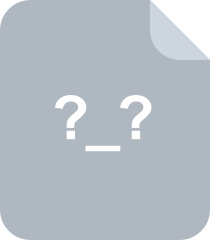
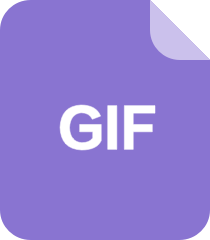
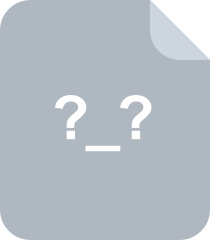
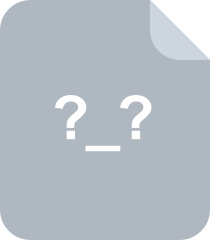
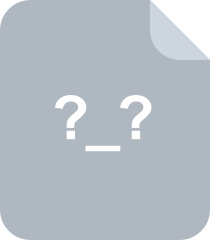
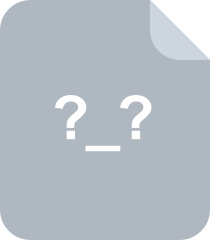
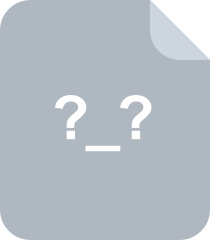
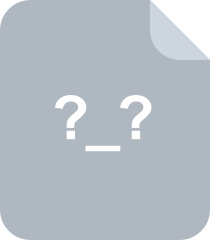
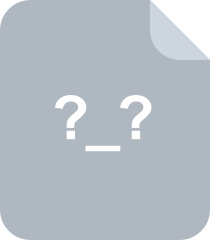
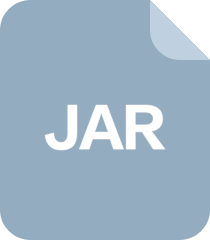
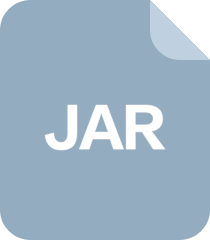
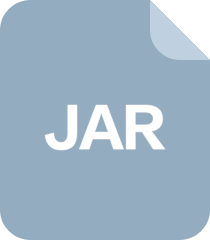
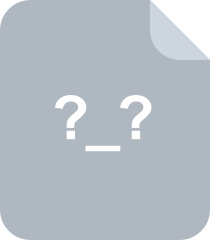
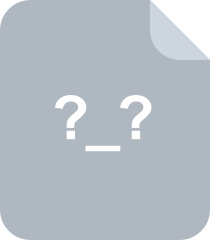
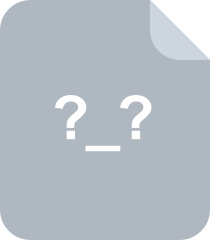
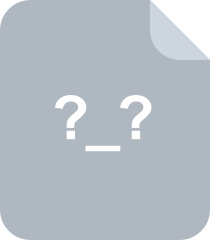
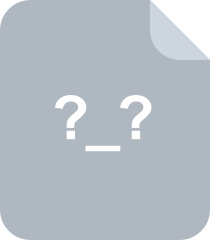
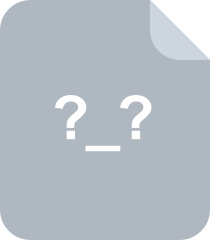
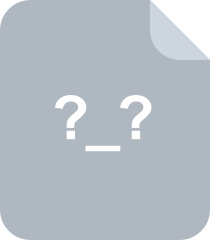
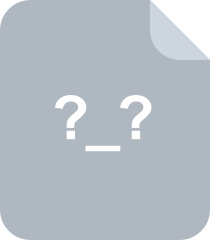
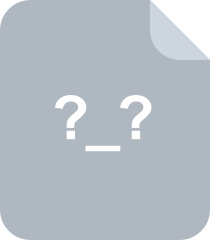
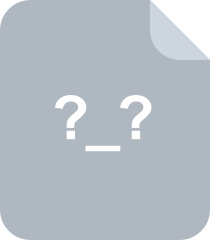
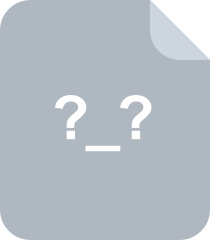
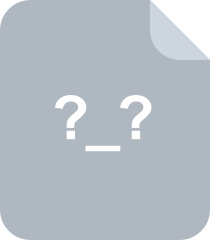
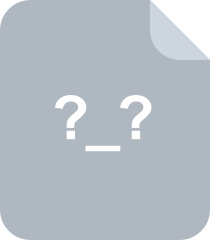
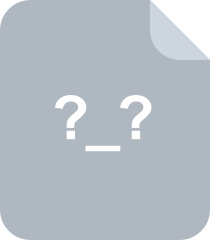
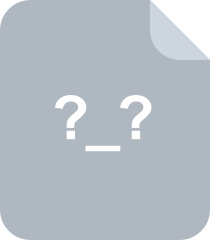
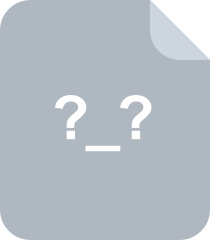
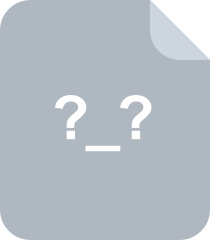
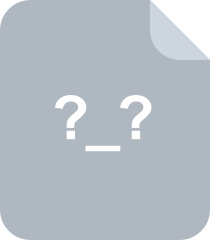
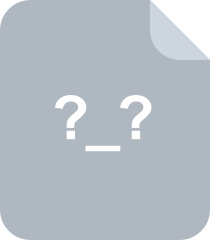
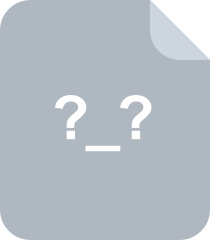
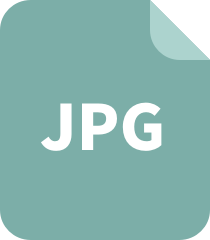
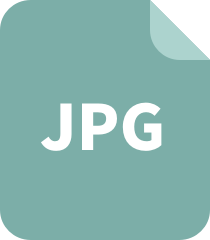
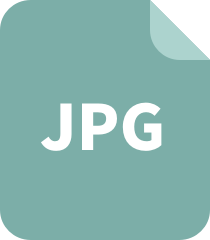
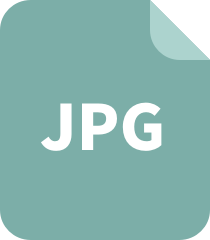
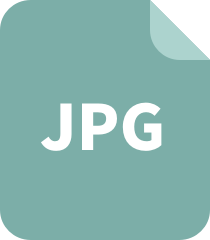
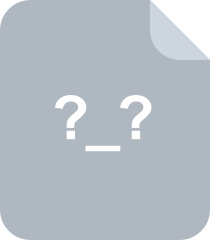
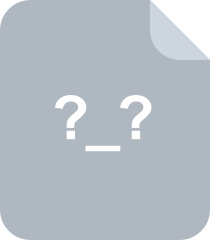
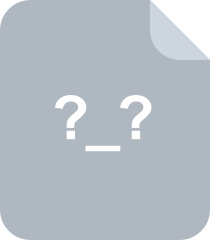
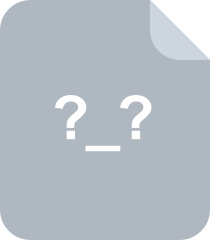
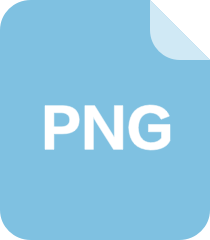
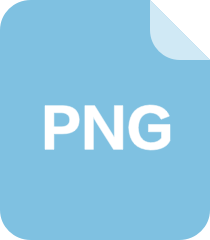
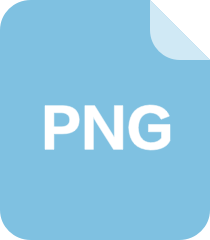
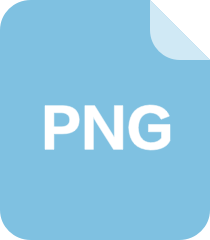
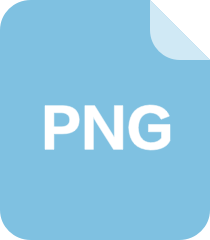
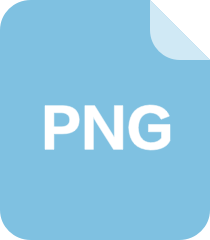
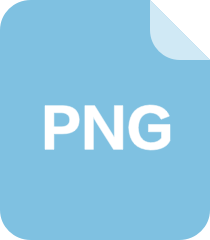
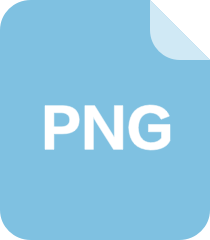
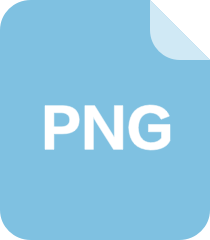
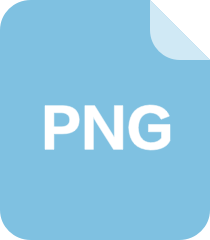
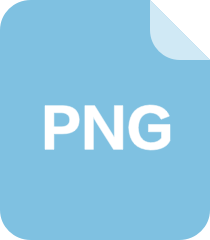
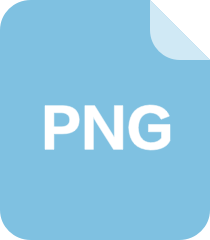
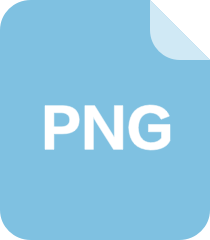
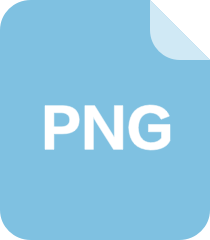
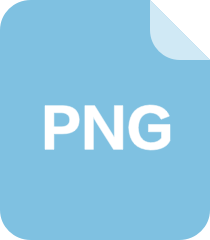
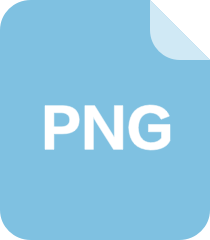
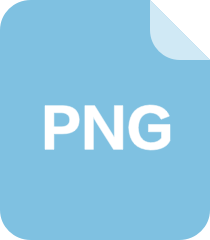
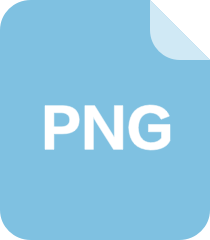
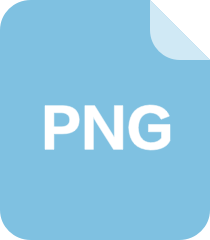
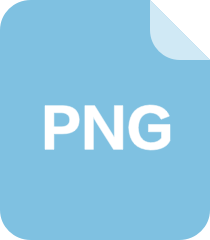
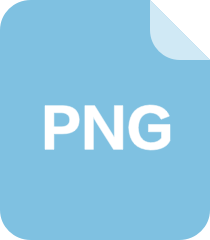
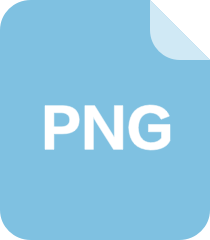
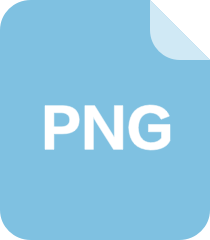
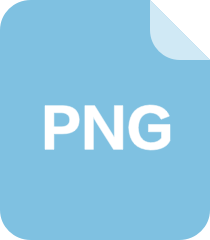
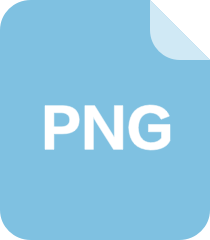
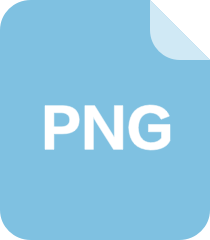
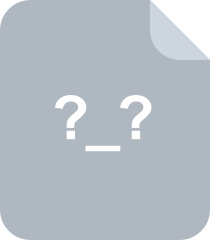
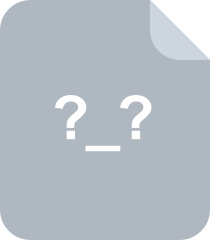
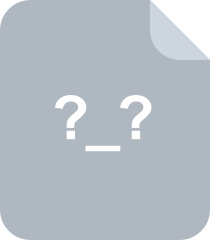
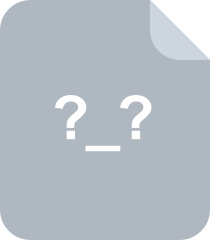
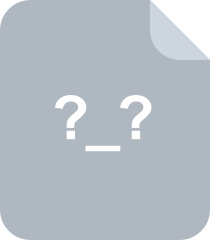
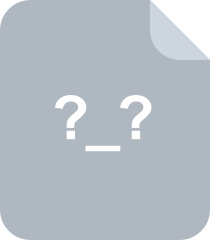
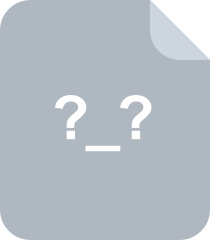
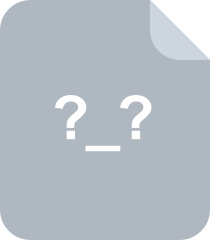
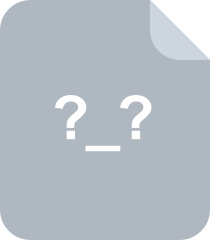
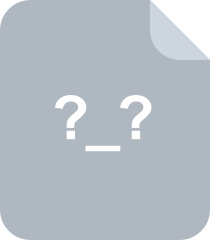
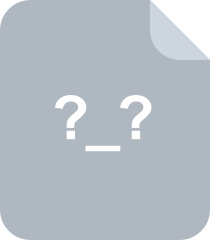
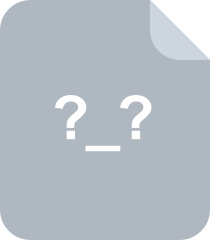
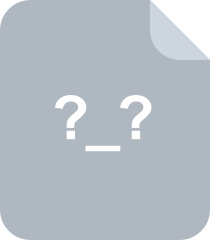
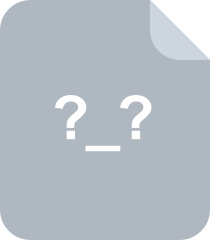
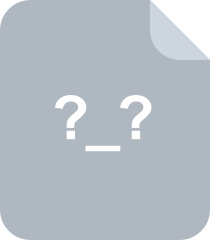
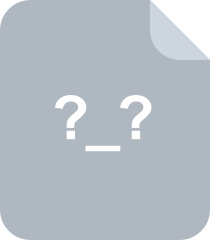
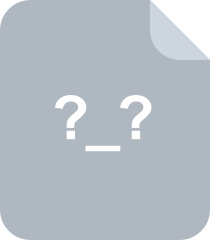
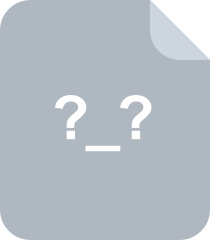
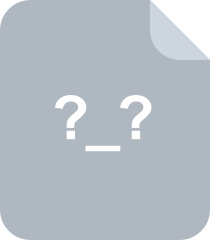
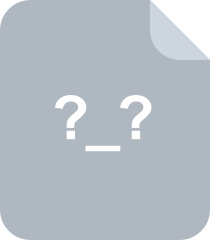
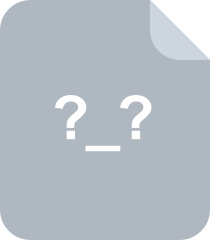
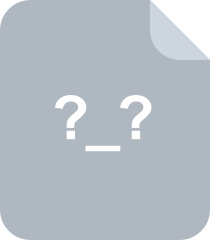
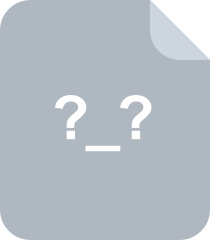
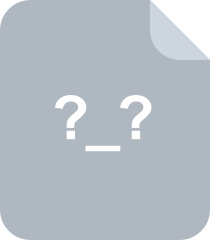
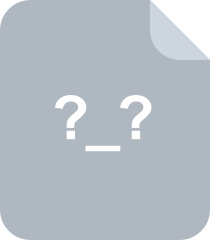
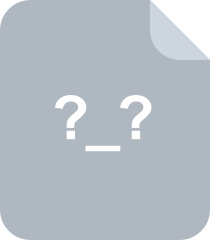
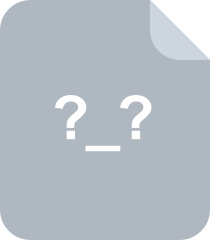
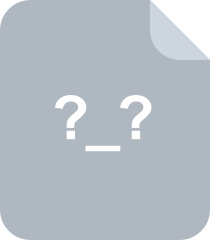
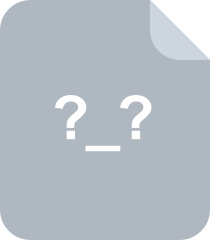
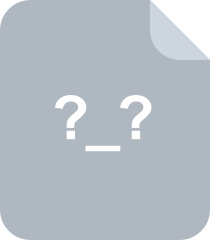
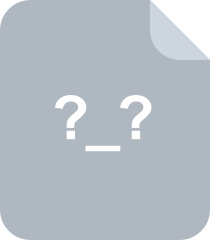
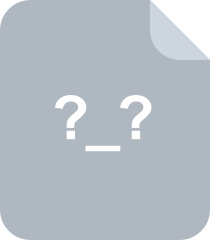
共 109 条
- 1
- 2
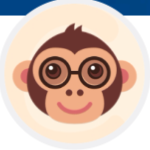
onnx
- 粉丝: 9625
- 资源: 5597
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

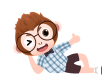
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


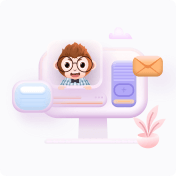
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
前往页