# validator.js
[![NPM version][npm-image]][npm-url]
[![CI][ci-image]][ci-url]
[![Coverage][codecov-image]][codecov-url]
[![Downloads][downloads-image]][npm-url]
[](#backers)
[](#sponsors)
[![Gitter][gitter-image]][gitter-url]
[![Disclose a vulnerability][huntr-image]][huntr-url]
A library of string validators and sanitizers.
## Strings only
**This library validates and sanitizes strings only.**
If you're not sure if your input is a string, coerce it using `input + ''`.
Passing anything other than a string will result in an error.
## Installation and Usage
### Server-side usage
Install the library with `npm install validator`
#### No ES6
```javascript
var validator = require('validator');
validator.isEmail('foo@bar.com'); //=> true
```
#### ES6
```javascript
import validator from 'validator';
```
Or, import only a subset of the library:
```javascript
import isEmail from 'validator/lib/isEmail';
```
#### Tree-shakeable ES imports
```javascript
import isEmail from 'validator/es/lib/isEmail';
```
### Client-side usage
The library can be loaded either as a standalone script, or through an [AMD][amd]-compatible loader
```html
<script type="text/javascript" src="validator.min.js"></script>
<script type="text/javascript">
validator.isEmail('foo@bar.com'); //=> true
</script>
```
The library can also be installed through [bower][bower]
```bash
$ bower install validator-js
```
CDN
```html
<script src="https://unpkg.com/validator@latest/validator.min.js"></script>
```
## Contributors
[Become a backer](https://opencollective.com/validatorjs#backer)
[Become a sponsor](https://opencollective.com/validatorjs#sponsor)
Thank you to the people who have already contributed:
<a href="https://github.com/validatorjs/validator.js/graphs/contributors"><img src="https://opencollective.com/validatorjs/contributors.svg?width=890" /></a>
## Validators
Here is a list of the validators currently available.
Validator | Description
--------------------------------------- | --------------------------------------
**contains(str, seed [, options])** | check if the string contains the seed.<br/><br/>`options` is an object that defaults to `{ ignoreCase: false, minOccurrences: 1 }`.<br />Options: <br/> `ignoreCase`: Ignore case when doing comparison, default false.<br/>`minOccurences`: Minimum number of occurrences for the seed in the string. Defaults to 1.
**equals(str, comparison)** | check if the string matches the comparison.
**isAfter(str [, options])** | check if the string is a date that is after the specified date.<br/><br/>`options` is an object that defaults to `{ comparisonDate: Date().toString() }`.<br/>**Options:**<br/>`comparisonDate`: Date to compare to. Defaults to `Date().toString()` (now).
**isAlpha(str [, locale, options])** | check if the string contains only letters (a-zA-Z).<br/><br/>`locale` is one of `['ar', 'ar-AE', 'ar-BH', 'ar-DZ', 'ar-EG', 'ar-IQ', 'ar-JO', 'ar-KW', 'ar-LB', 'ar-LY', 'ar-MA', 'ar-QA', 'ar-QM', 'ar-SA', 'ar-SD', 'ar-SY', 'ar-TN', 'ar-YE', 'bg-BG', 'bn', 'cs-CZ', 'da-DK', 'de-DE', 'el-GR', 'en-AU', 'en-GB', 'en-HK', 'en-IN', 'en-NZ', 'en-US', 'en-ZA', 'en-ZM', 'es-ES', 'fa-IR', 'fi-FI', 'fr-CA', 'fr-FR', 'he', 'hi-IN', 'hu-HU', 'it-IT', 'kk-KZ', 'ko-KR', 'ja-JP', 'ku-IQ', 'nb-NO', 'nl-NL', 'nn-NO', 'pl-PL', 'pt-BR', 'pt-PT', 'ru-RU', 'si-LK', 'sl-SI', 'sk-SK', 'sr-RS', 'sr-RS@latin', 'sv-SE', 'th-TH', 'tr-TR', 'uk-UA']` and defaults to `en-US`. Locale list is `validator.isAlphaLocales`. `options` is an optional object that can be supplied with the following key(s): `ignore` which can either be a String or RegExp of characters to be ignored e.g. " -" will ignore spaces and -'s.
**isAlphanumeric(str [, locale, options])** | check if the string contains only letters and numbers (a-zA-Z0-9).<br/><br/>`locale` is one of `['ar', 'ar-AE', 'ar-BH', 'ar-DZ', 'ar-EG', 'ar-IQ', 'ar-JO', 'ar-KW', 'ar-LB', 'ar-LY', 'ar-MA', 'ar-QA', 'ar-QM', 'ar-SA', 'ar-SD', 'ar-SY', 'ar-TN', 'ar-YE', 'bn', 'bg-BG', 'cs-CZ', 'da-DK', 'de-DE', 'el-GR', 'en-AU', 'en-GB', 'en-HK', 'en-IN', 'en-NZ', 'en-US', 'en-ZA', 'en-ZM', 'es-ES', 'fa-IR', 'fi-FI', 'fr-CA', 'fr-FR', 'he', 'hi-IN', 'hu-HU', 'it-IT', 'kk-KZ', 'ko-KR', 'ja-JP','ku-IQ', 'nb-NO', 'nl-NL', 'nn-NO', 'pl-PL', 'pt-BR', 'pt-PT', 'ru-RU', 'si-LK', 'sl-SI', 'sk-SK', 'sr-RS', 'sr-RS@latin', 'sv-SE', 'th-TH', 'tr-TR', 'uk-UA']`) and defaults to `en-US`. Locale list is `validator.isAlphanumericLocales`. `options` is an optional object that can be supplied with the following key(s): `ignore` which can either be a String or RegExp of characters to be ignored e.g. " -" will ignore spaces and -'s.
**isAscii(str)** | check if the string contains ASCII chars only.
**isBase32(str [, options])** | check if the string is base32 encoded. `options` is optional and defaults to `{ crockford: false }`.<br/> When `crockford` is true it tests the given base32 encoded string using [Crockford's base32 alternative][Crockford Base32].
**isBase58(str)** | check if the string is base58 encoded.
**isBase64(str [, options])** | check if the string is base64 encoded. `options` is optional and defaults to `{ urlSafe: false }`<br/> when `urlSafe` is true it tests the given base64 encoded string is [url safe][Base64 URL Safe].
**isBefore(str [, date])** | check if the string is a date that is before the specified date.
**isBIC(str)** | check if the string is a BIC (Bank Identification Code) or SWIFT code.
**isBoolean(str [, options])** | check if the string is a boolean.<br/>`options` is an object which defaults to `{ loose: false }`. If `loose` is is set to false, the validator will strictly match ['true', 'false', '0', '1']. If `loose` is set to true, the validator will also match 'yes', 'no', and will match a valid boolean string of any case. (e.g.: ['true', 'True', 'TRUE']).
**isBtcAddress(str)** | check if the string is a valid BTC address.
**isByteLength(str [, options])** | check if the string's length (in UTF-8 bytes) falls in a range.<br/><br/>`options` is an object which defaults to `{ min: 0, max: undefined }`.
**isCreditCard(str [, options])** | check if the string is a credit card number.<br/><br/> `options` is an optional object that can be supplied with the following key(s): `provider` is an optional key whose value should be a string, and defines the company issuing the credit card. Valid values include `['amex', 'dinersclub', 'discover', 'jcb', 'mastercard', 'unionpay', 'visa']` or blank will check for any provider.
**isCurrency(str [, options])** | check if the string is a valid currency amount.<br/><br/>`options` is an object which defaults to `{ symbol: '$', require_symbol: false, allow_space_after_symbol: false, symbol_after_digits: false, allow_negatives: true, parens_for_negatives: false, negative_sign_before_digits: false, negative_sign_after_digits: false, allow_negative_sign_placeholder: false, thousands_separator: ',', decimal_separator: '.', allow_decimal: true, require_decimal: false, digits_after_decimal: [2], allow_space_after_digits: false }`.<br/>**Note:** The array `digits_after_decimal` is filled with the exact number of digits allowed not a range, for example a range 1 to 3 will be given as [1, 2, 3].
**isDataURI(str)** | check if the string is a [data uri format][Data URI Format].
**isDate(str [, options])** | check if the string is a valid date. e.g. [`2002-07-15`, new Date()].<br/><br/> `options` is an object which can contain the keys `format`, `strictMode` and/or `delimiters`.<br/><br/>`format` is a string and defaults to `YYYY/MM/DD`.<br/><br/>`strict
没有合适的资源?快使用搜索试试~ 我知道了~
前端最全表单校验JS,支持各种身份证号码、手机号码、固定电话、URL、类型等校验
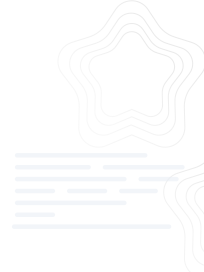
共344个文件
js:333个
json:4个
md:3个


温馨提示
前端最强表单校验JS文件,资源包已经做好打包,支持在线引入,webpack引入。 适用于各种证件号码,身份证号码,护照等校验,各地手机号码,固定电话校验,网址以及一些前端类型判断,非空判断等校验。 压缩包内有使用说明书,部分文档如下: ### Client-side usage The library can be loaded either as a standalone script, or through an [AMD][amd]-compatible loader ```html <script type="text/javascript" src="validator.min.js"></script> <script type="text/javascript"> validator.isEmail('foo@bar.com'); //=> true </script> ```
资源推荐
资源详情
资源评论
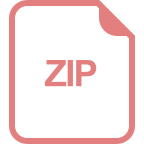
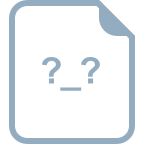
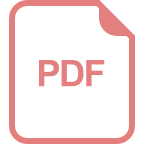
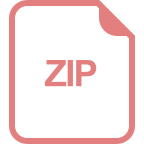
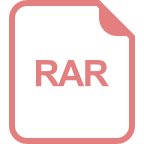
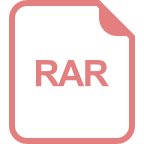
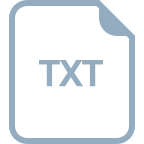
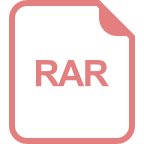
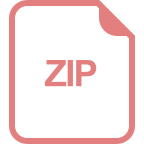
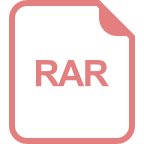
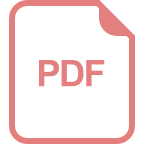
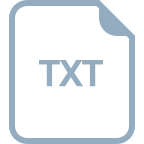
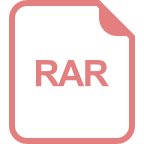
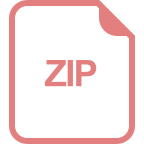
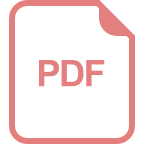
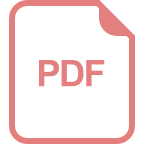
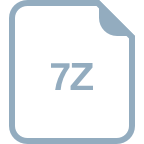
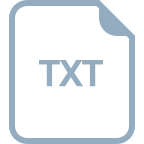
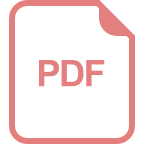
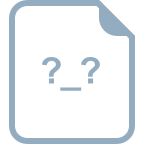
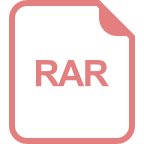
收起资源包目录

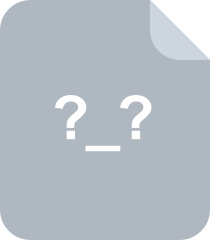
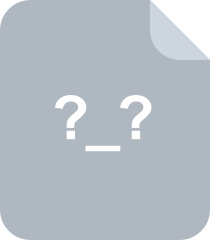
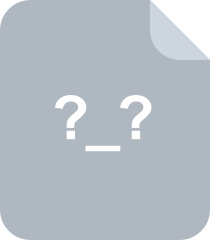
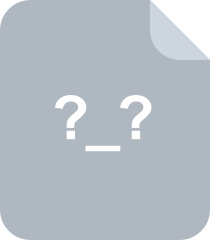
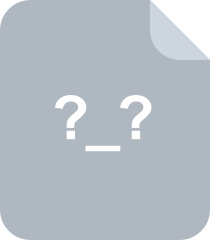
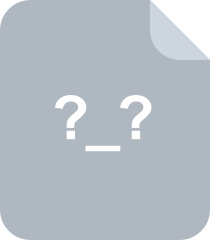
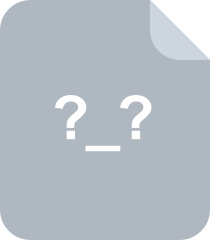
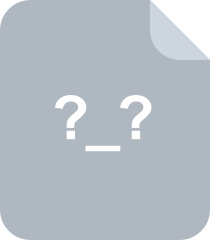
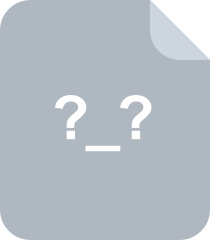
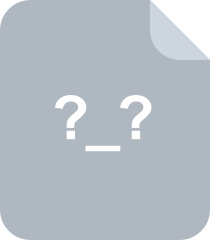
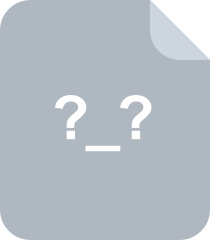
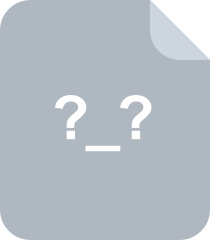
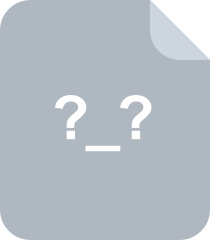
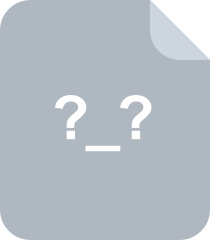
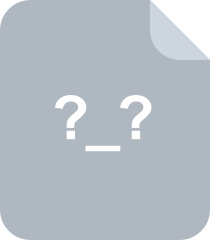
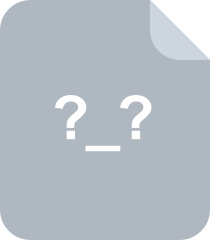
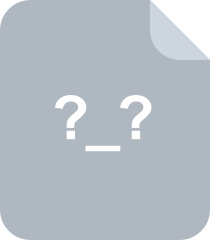
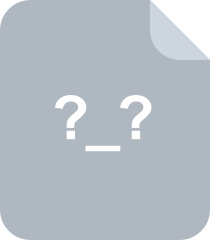
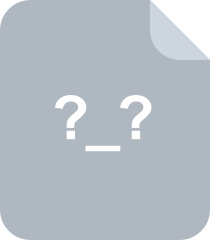
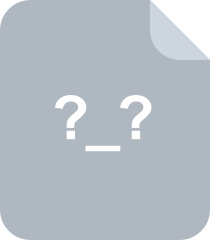
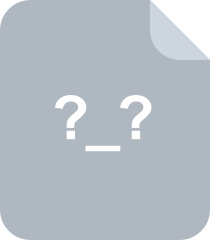
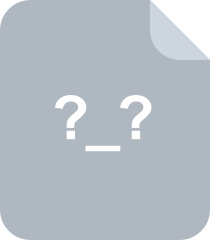
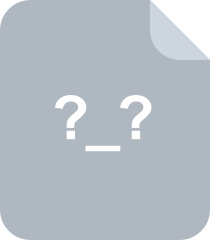
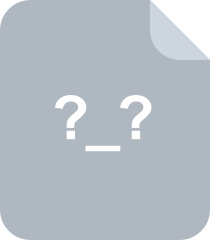
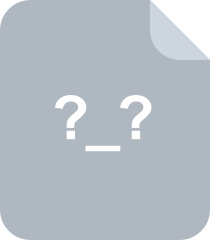
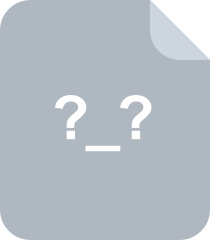
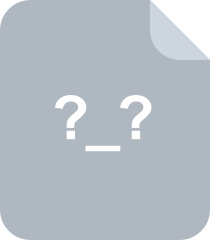
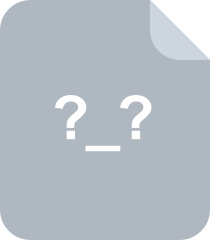
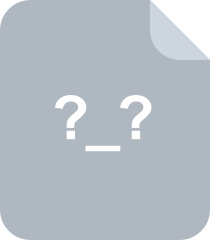
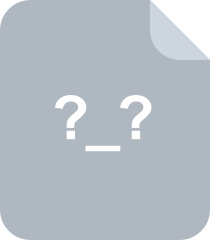
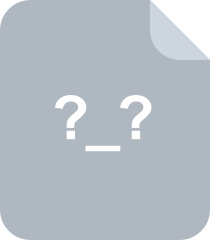
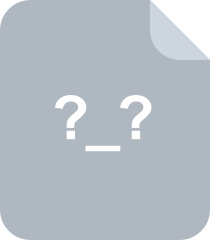
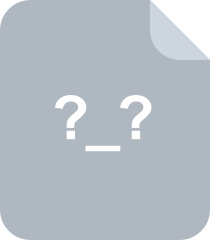
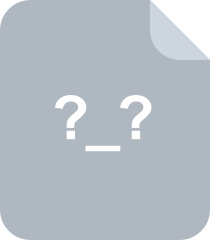
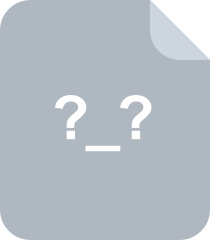
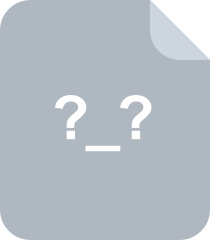
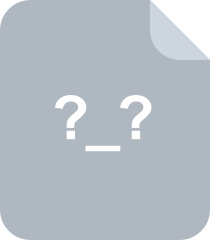
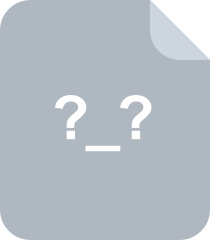
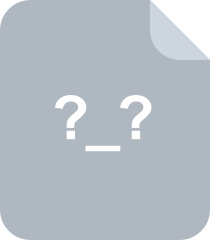
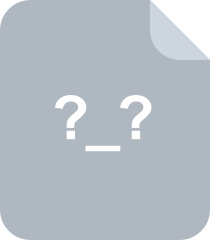
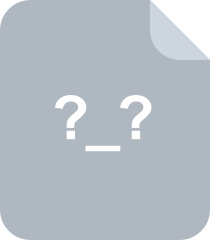
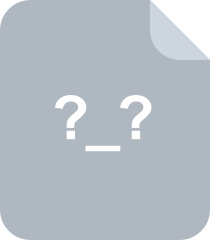
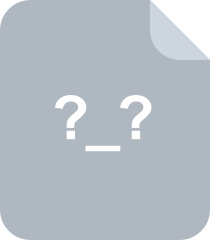
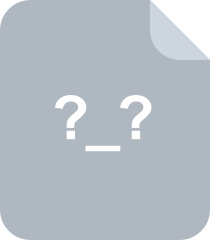
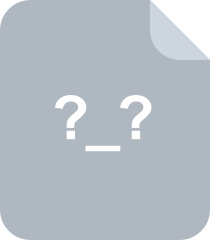
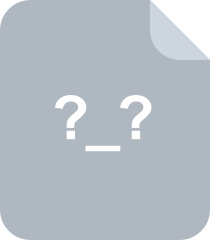
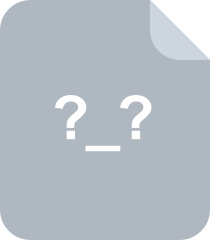
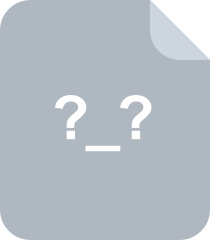
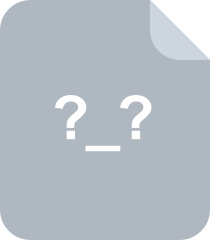
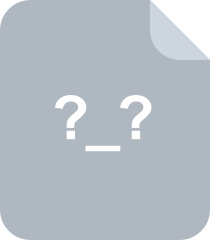
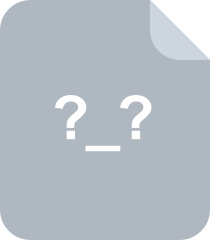
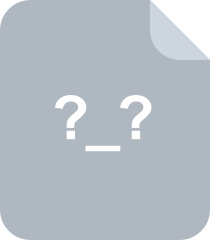
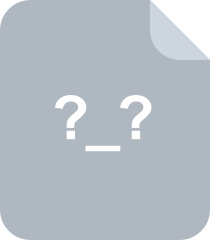
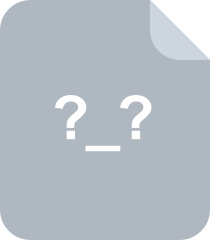
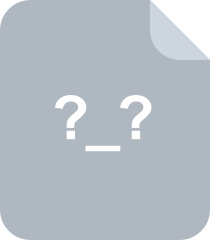
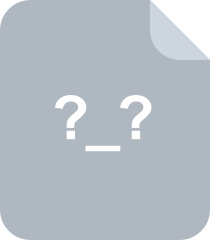
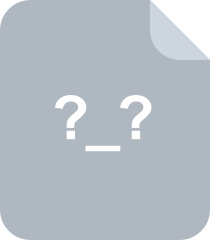
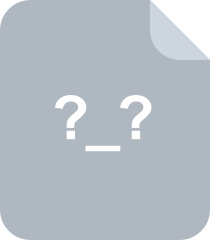
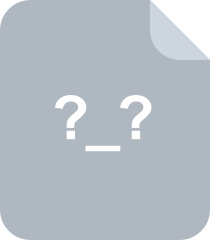
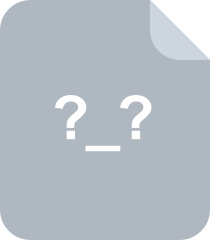
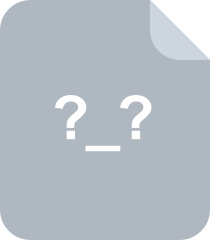
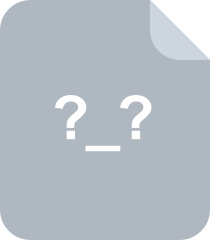
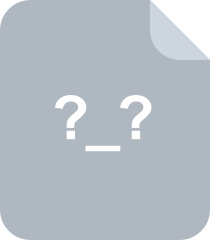
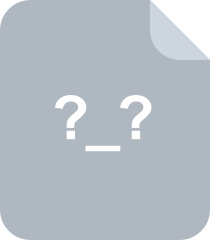
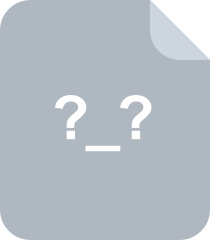
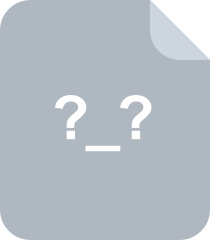
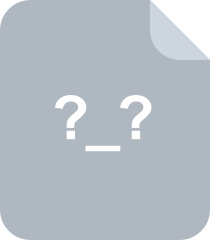
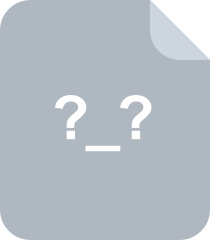
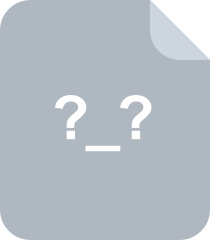
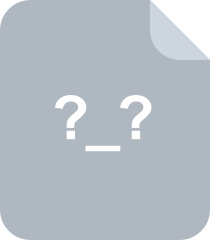
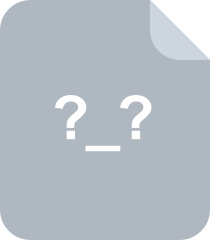
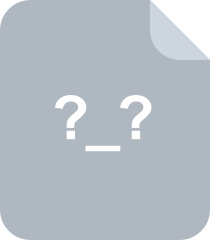
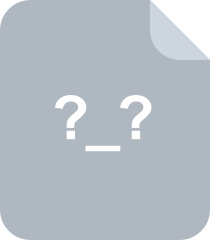
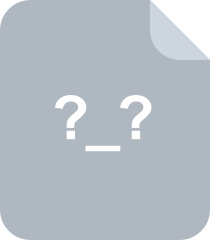
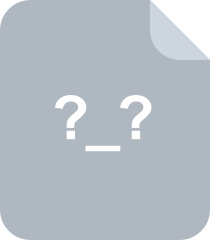
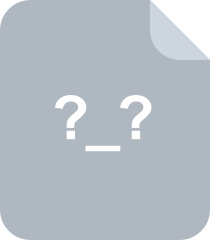
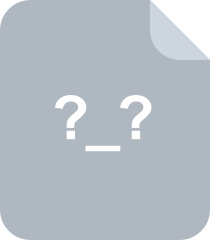
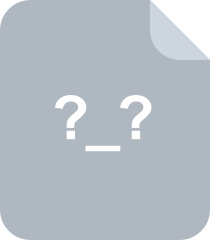
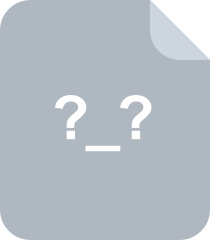
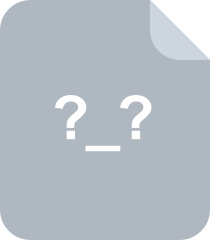
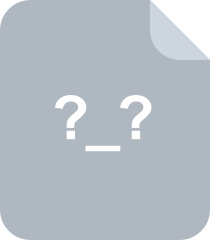
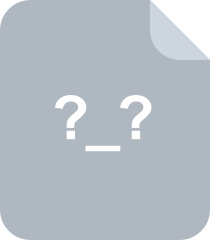
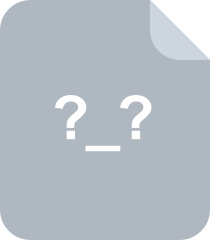
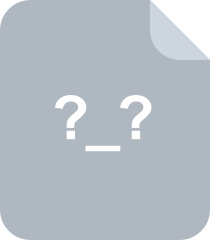
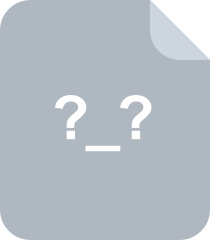
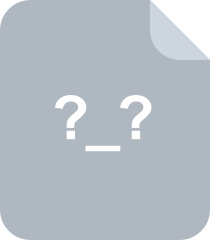
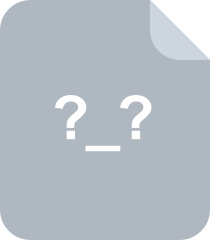
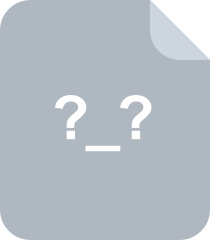
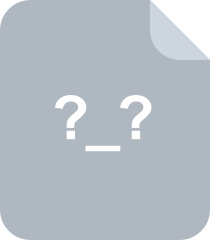
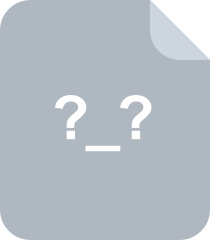
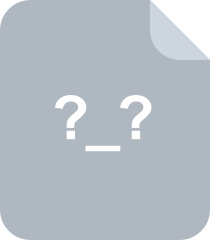
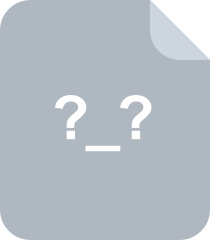
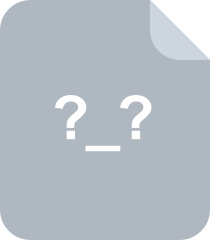
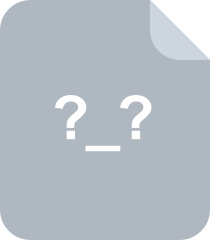
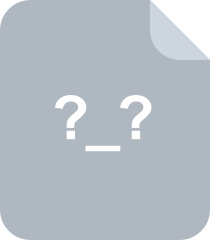
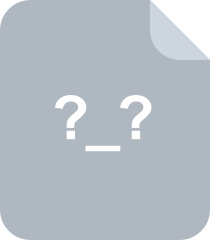
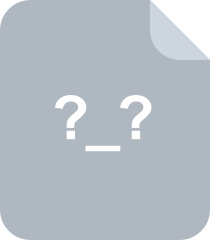
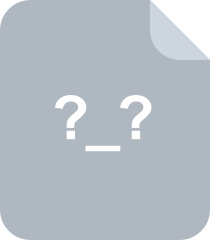
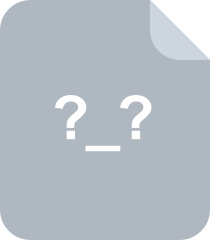
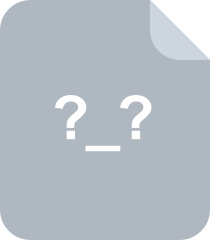
共 344 条
- 1
- 2
- 3
- 4
资源评论
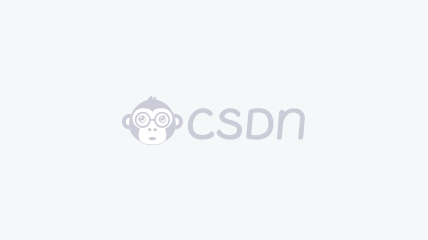
- m0_674022222024-02-10#完美解决问题

youmatech
- 粉丝: 1061
- 资源: 17
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

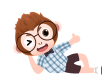
最新资源
- (18956428)STM32F103C8T6 小系统原理图 PCB
- (175828796)python全国疫情数据爬虫可视化分析系统(django)源码数据库演示.zip
- 记账本项目市场需求文档(MRD)
- (31687028)PID控制器matlab仿真.zip
- 基于SpringBoot的“在线答疑系统”的设计与实现(源码+数据库+文档+PPT).zip
- (11828838)进销存系统源码
- 记账本项目三大模块原型图
- fed54987-3a28-4a7a-9c89-52d3ac6bc048.vsidx
- (177367038)QT实现教务管理系统.zip
- (178041422)基于springboot网上书城系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


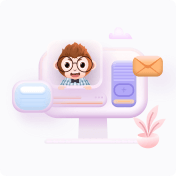
安全验证
文档复制为VIP权益,开通VIP直接复制
