#include<iostream>
using namespace std;
class Date
{
public:
int GetMonthDay(int year, int month)
{
static int days[13]={0,31,28,31,30,31,30,31,31,30,31,30,31};
int day = days[month];
if((month == 2)&&(((year % 4 == 0)&&(year % 100 != 0))||(year%400 ==0)))
{
day+=1;
}
return day;
}
Date(int year = 2000, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
Date(const Date& d)//拷贝构造函数
{
_year = d._year;
_month =d._month;
_day = d._day;
}
Date& operator+=(int day)//+=运算符重载
{
if(day < 0)
{
return *this -= -day;
}
_day += day;
while(_day > GetMonthDay(_year,_month))
{
_day -=GetMonthDay(_year,_month);
_month++;
if(_month == 13)
{
_year++;
_month = 1;
}
}
return *this;
}
Date& operator-=(int day)//-=运算符重载
{
if(day < 0)
{
return *this += -day;
}
_day -= day;
while(_day < 0)
{
_month--;
if(_month == 0)
{
_year--;
_month = 12;
}
_day +=GetMonthDay(_year,_month);
}
return *this;
}
Date& operator=(const Date& d)//赋值运算符重载
{
if(this !=&d)
{
_year = d._year;
_month = d._month;
_day = d._day;
}
}
Date& operator++()//前置++运算符重载
{
*this+=1;
return *this;
}
Date operator++(int)//后置++运算符重载
{
Date ret(*this);
*this +=1;
return ret;
}
bool operator<(const Date& d)
{
if(_year < d._year)
{
return 1;
}
else if (_year == d._year)
{
if(_month < d._month)
{
return 1;
}
else if(_month == d._month)
{
if(_day < d._day)
{
return 1;
}
else
{
return 0;
}
}
else
{
return 0;
}
}
else
{
return 0;
}
}
int operator-(const Date&d)//-运算符重载,用于计算日期差
{
Date max = *this;
Date min = d;
if(*this < d)
{
max = d;
min = *this;
}
int day =0;
while(min <max)
{
++(min);
++day;
}
return day;
}
int _year;
int _month;
int _day;
};
int main()
{
//Date d1(2024,9,5);
int i,j,k;
cout <<"请输入初始日期的年"<<'\n';
cin>>i;
cout <<"请输入初始日期的月"<<'\n';
cin>>j;
cout <<"请输入初始日期的日"<<'\n';
cin>>k;
Date d1(i,j,k);
cout <<"您输入的日期为:"<<'\t'<< d1._year << '-' << d1._month << '-' << d1._day <<endl;
int select;
cout <<"请输入需要进行的操作"<<'\n';
cout <<"1.日期加运算"<<'\n';
cout <<"2.日期减运算"<<'\n';
cout <<"3.计算日期差"<<'\n';
cin >>select;
if(select == 1)
{
int day1;
cout <<"请输入需要加的天数"<<endl;
cin >> day1;
d1+=day1;
cout << d1._year << '-' << d1._month << '-' << d1._day <<endl;
}
else if (select ==2)
{
int day2;
cout <<"请输入需要减的天数"<<endl;
cin >> day2;
d1-=day2;
cout << d1._year << '-' << d1._month << '-' << d1._day <<endl;
}
else if(select == 3)
{
int i1,j1,k1;
cout <<"请输入目标日期年"<<'\n';
cin>>i1;
cout <<"请输入目标日期月"<<'\n';
cin>>j1;
cout <<"请输入目标日期日"<<'\n';
cin>>k1;
Date d2(i1,j1,k1);
cout <<"您输入的日期为:"<<'\t'<< d2._year << '-' << d2._month << '-' << d2._day <<endl;
int ret = d1-d2;
cout <<"两个日期只差为:"<<' ' << ret << "天" <<endl;
}
else
{
cout << "您的输入有误"<<endl;
}
return 0;
}


雪好像下整夜
- 粉丝: 156
- 资源: 2
最新资源
- 基于java的工作量统计系统的设计与实现.docx
- 基于java的工资信息管理系统的设计与实现.docx
- 与SecureCRT-Xshell变色方案文章配套使用
- 基于java的教师薪酬管理系统的设计与实现.docx
- 基于java的家教管理系统的设计与实现.docx
- 基于java的国产动漫网站的设计与实现.docx
- 基于java的教学辅助系统的设计与实现.docx
- 基于java的客户管理系统的设计与实现.docx
- 基于java的教学资源共享平台的设计与实现.docx
- 基于java的垃圾分类回收系统的设计与实现.docx
- 基于java的篮球联盟管理系统的设计与实现.docx
- 基于java的七彩云南文化旅游网站的设计与实现.docx
- 基于java的农业设备租赁系统的设计与实现.docx
- 基于java的美食信息推荐系统的设计与实现.docx
- 新型滑模扰动观测器+模型预测控制的永磁同步电机带载仿真模型 控制结合:转速环(新型滑模扰动观测器(NSMDO)+电流内环(MPCC模型预测控制 模型预测m代码附带详细注释 1转速环:采用新型滑模扰
- 基于java的人驾校预约管理系统的设计与实现.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


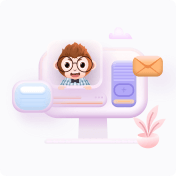