<div align="center">
<img src="https://github.com/eserie/wax-ml/blob/main/docs/_static/wax_logo.png" alt="logo" width="40%"></img>
</div>
# WAX-ML: A Python library for machine-learning and feedback loops on streaming data

[](https://wax-ml.readthedocs.io/en/latest/)
[](https://badge.fury.io/py/wax-ml)
[](https://codecov.io/gh/eserie/wax-ml)
[](https://github.com/ambv/black)
[**Quickstart**](#quickstart-colab-in-the-cloud)
| [**Install guide**](#installation)
| [**Change logs**](https://wax-ml.readthedocs.io/en/latest/changelog.html)
| [**Reference docs**](https://wax-ml.readthedocs.io/en/latest/)
## Introduction
ð Wax is what you put on a surfboard to avoid slipping. It is an essential tool to go
surfing ... ð
WAX-ML is a research-oriented [Python](https://www.python.org/) library
providing tools to design powerful machine learning algorithms and feedback loops
working on streaming data.
It strives to complement [JAX](https://jax.readthedocs.io/en/latest/)
with tools dedicated to time series.
WAX-ML makes JAX-based programs easy to use for end-users working
with
[pandas](https://pandas.pydata.org/) and [xarray](http://xarray.pydata.org/en/stable/)
for data manipulation.
WAX-ML provides a simple mechanism for implementing feedback loops, allows the implementation of
reinforcement learning algorithms with functions, and makes them easy to integrate by
end-users working with the object-oriented reinforcement learning framework from the
[Gym](https://gym.openai.com/) library.
To learn more, you can read our [article on ArXiv](http://arxiv.org/abs/2106.06524)
or simply access the code in this repository.
## WAX-ML Goal
WAX-ML's goal is to expose "traditional" algorithms that are often difficult to find in standard
Python ecosystem and are related to time-series and more generally to streaming data.
It aims to make it easy to work with algorithms from very various computational domains such as
machine learning, online learning, reinforcement learning, optimal control, time-series analysis,
optimization, statistical modeling.
For now, WAX-ML focuses on **time-series** algorithms as this is one of the areas of machine learning
that lacks the most dedicated tools. Working with time series is notoriously known to be difficult
and often requires very specific algorithms (statistical modeling, filtering, optimal control).
Even though some of the modern machine learning methods such as RNN, LSTM, or reinforcement learning
can do an excellent job on some specific time-series problems, most of the problems require using
more traditional algorithms such as linear and non-linear filters, FFT,
the eigendecomposition of matrices (e.g. [[7]](#references)),
principal component analysis (PCA) (e.g. [[8]](#references)), Riccati solvers for
optimal control and filtering, ...
By adopting a functional approach, inherited from JAX, WAX-ML aims to be an efficient tool to
combine modern machine learning approaches with more traditional ones.
Some work has been done in this direction in [[2] in References](#references) where transformer encoder
architectures are massively accelerated, with limited accuracy costs, by replacing the
self-attention sublayers with a standard, non-parameterized Fast Fourier Transform (FFT).
WAX-ML may also be useful for developing research ideas in areas such as online machine learning
(see [[1] in References](#references)) and development of control, reinforcement learning,
and online optimization methods.
## What does WAX-ML do?
Well, building WAX-ML, we have some pretty ambitious design and implementation goals.
To do things right, we decided to start small and in an open-source design from the beginning.
For now, WAX-ML contains:
- transformation tools that we call "unroll" transformations allowing us to
apply any transformation, possibly stateful, on sequential data. It generalizes the RNN
architecture to any stateful transformation allowing the implementation of any kind of "filter".
- a "stream" module, described in [ð Streaming Data ð](#-streaming-data-), permitting us to
synchronize data streams with different time resolutions.
- some general pandas and xarray "accessors" permitting the application of any
JAX-functions on pandas and xarray data containers:
`DataFrame`, `Series`, `Dataset`, and `DataArray`.
- ready-to-use exponential moving average filter that we exposed with two APIs:
- one for JAX users: as Haiku modules (`EWMA`, ... see the complete list in our
[API documentation](https://wax-ml.readthedocs.io/en/latest/wax.modules.html)
).
- a second one for pandas and xarray users: with drop-in replacement of pandas
`ewm` accessor.
- a simple module `OnlineSupervisedLearner` to implement online learning algorithms
for supervised machine learning problems.
- building blocks for designing feedback loops in reinforcement learning, and have
provided a module called `GymFeedback` allowing the implementation of feedback loop as the
introduced in the library [Gym](https://gym.openai.com/), and illustrated this figure:
<div align="center">
<img src="docs/tikz/gymfeedback.png" alt="logo" width="60%"></img>
</div>
### What is JAX?
JAX is a research-oriented computational system implemented in Python that leverages the
XLA optimization framework for machine learning computations. It makes XLA usable with
the NumPy API and some functional primitives for just-in-time compilation,
differentiation, vectorization, and parallelization. It allows building higher-level
transformations or "programs" in a functional programming approach.
See [JAX's page](https://github.com/google/jax) for more details.
## Why to use WAX-ML?
If you deal with time-series and are a pandas or xarray user, b
ut you want to use the impressive
tools of the JAX ecosystem, then WAX-ML might be the right tool for you,
as it implements pandas and
xarray accessors to apply JAX functions.
If you are a user of JAX, you may be interested in adding WAX-ML to your toolbox to address
time-series problems.
## Design
### Research oriented
WAX-ML is a research-oriented library. It relies on
[JAX](https://jax.readthedocs.io/en/latest/notebooks/quickstart.html) and
[Haiku](https://github.com/deepmind/dm-haiku) functional programming paradigm to ease the
development of research ideas.
WAX-ML is a bit like [Flux](https://fluxml.ai/Flux.jl/stable/)
in [Julia](https://julialang.org/) programming language.
### Functional programming
In WAX-ML, we pursue a functional programming approach inherited from JAX.
In this sense, WAX-ML is not a framework, as most object-oriented libraries offer. Instead, we
implement "functions" that must be pure to exploit the JAX ecosystem.
### Haiku modules
We use the "module" mechanism proposed by the Haiku library to easily generate pure function pairs,
called `init` and `apply` in Haiku, to implement programs that require the management of
parameters and/or state variables.
You can see
[the Haiku module API](https://dm-haiku.readthedocs.io/en/latest/api.html#modules-parameters-and-state)
and
[Haiku transformation functions](https://dm-haiku.readthedocs.io/en/latest/api.html#haiku-transforms)
for more details.
In this way, we can recover all the advantages of
object-oriented programming but exposed in the functional programming approach.
It permits to ease the development of robust and reusable features and to
develop "mini-languages" tailored to specific scientific domains.
### WAX-ML works with other libraries
We want existing machine learning libraries to work well together while try
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
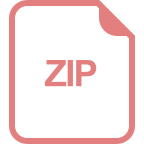
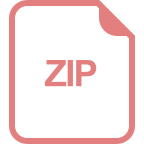
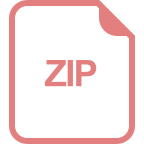
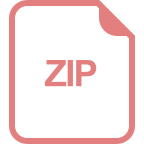
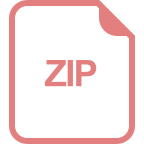
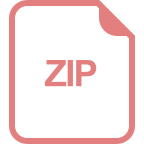
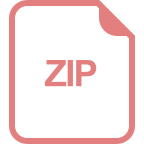
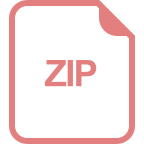
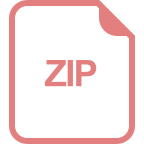
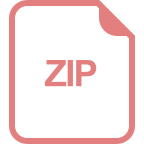
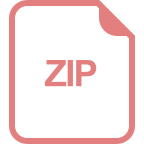
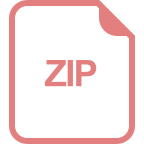
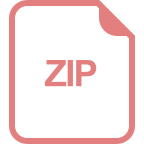
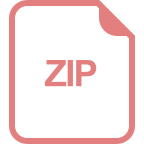
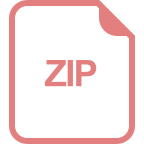
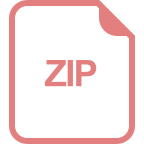
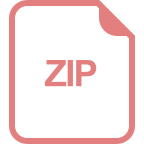
收起资源包目录

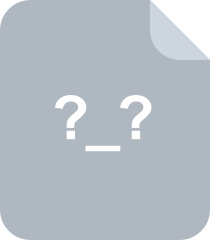
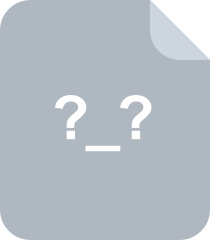
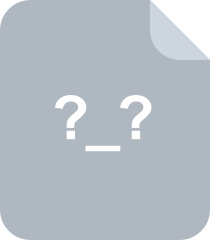
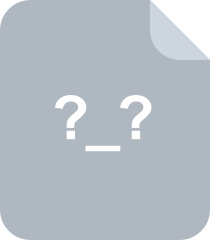
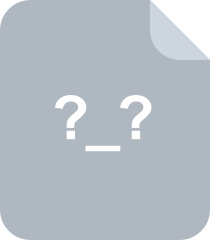

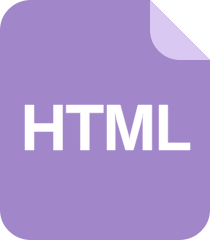
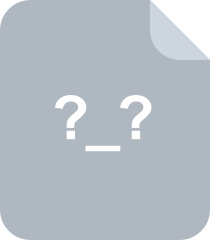
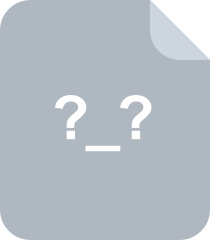
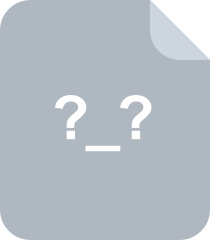
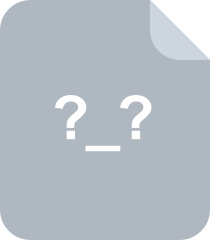
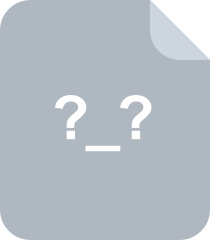
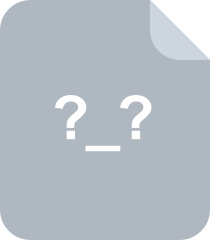
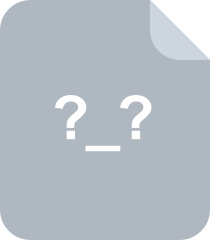
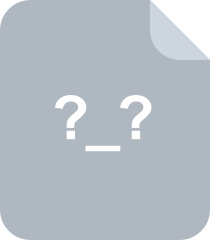
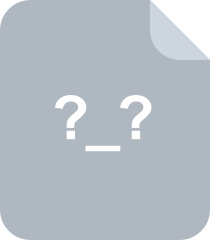
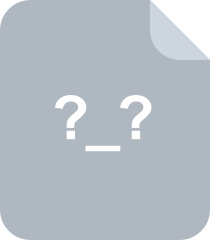
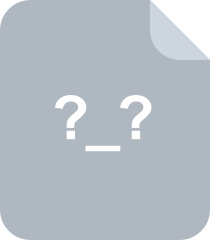
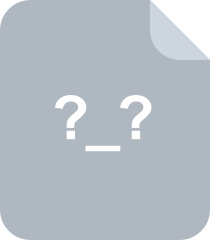
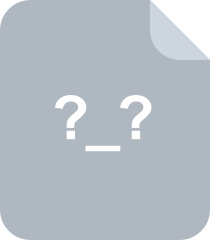
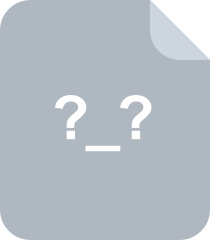
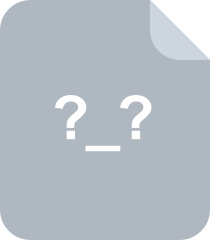
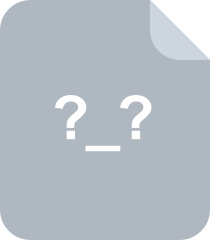
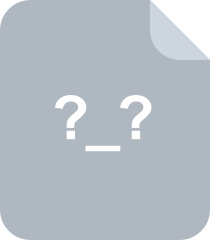
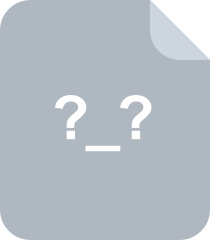
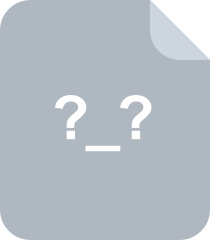
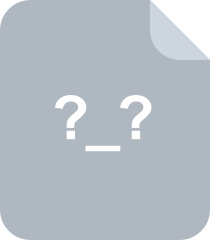
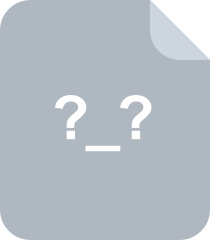
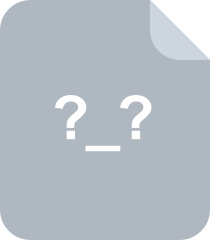
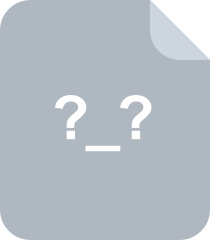
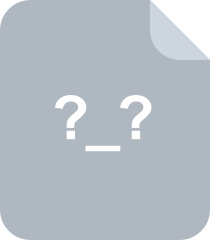
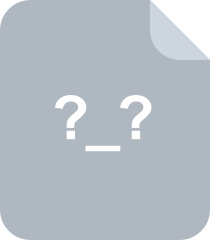
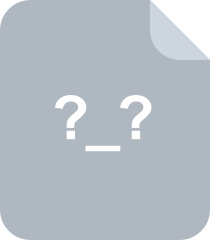
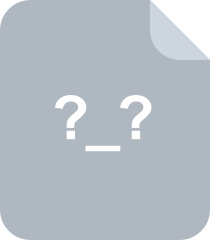
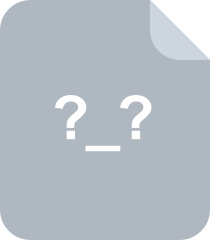
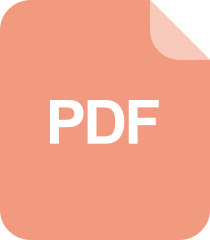
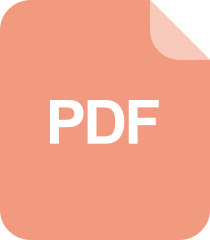
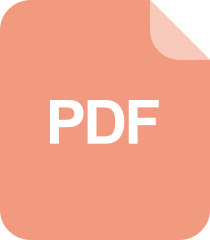
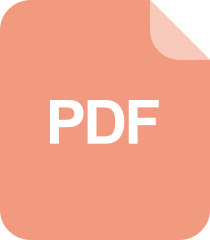
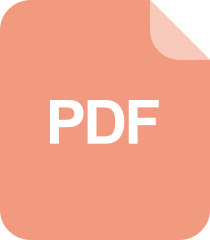
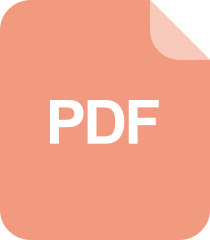
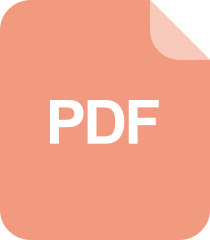
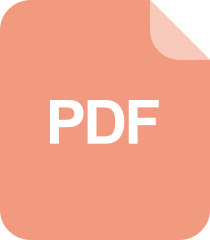
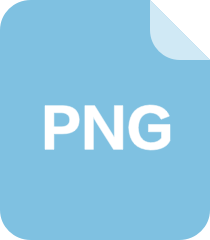
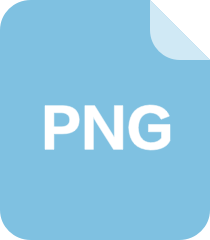
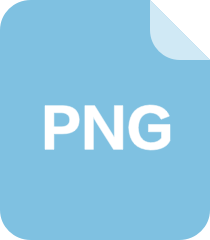
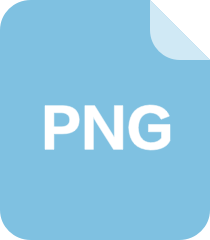
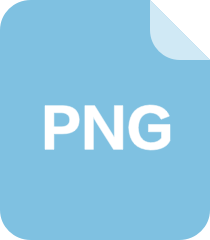
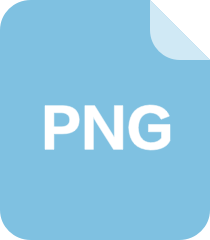
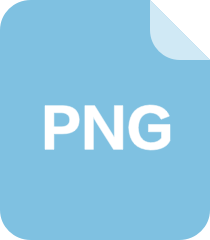
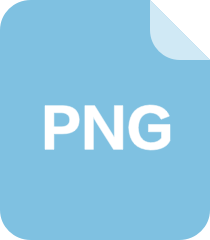
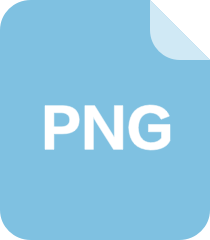
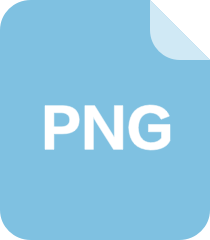
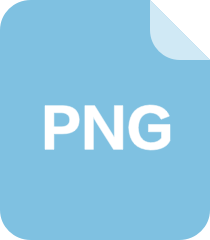
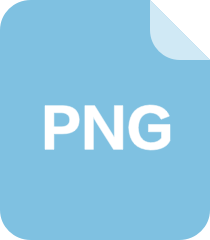
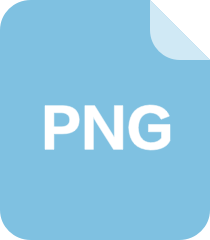
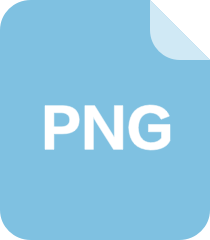
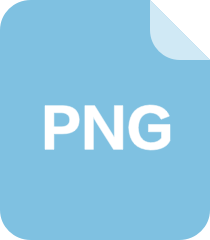
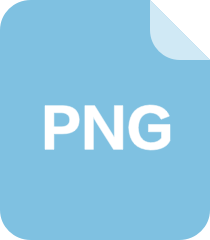
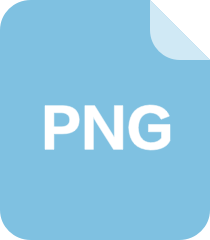
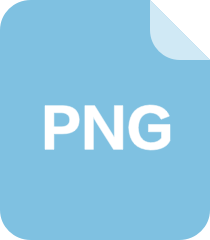
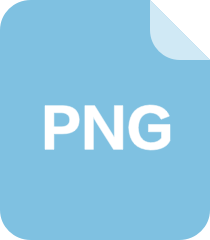
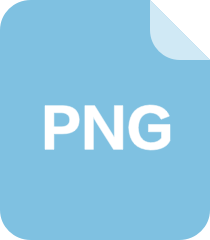
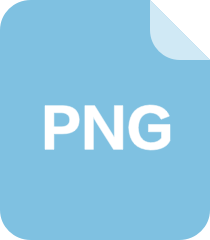
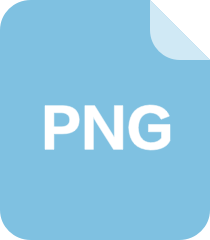
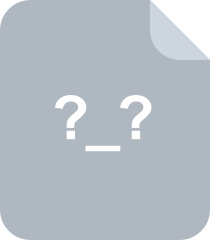
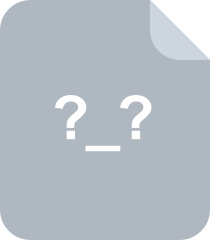
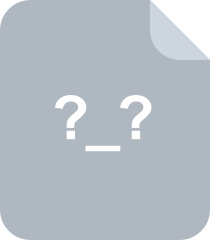
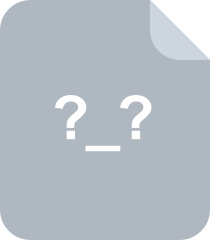
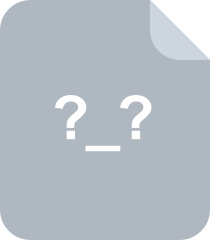
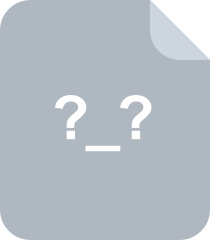
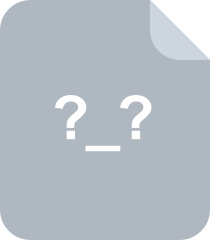
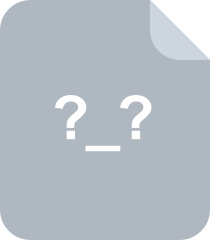
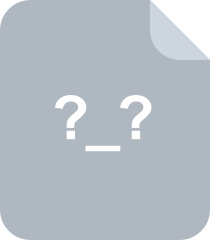
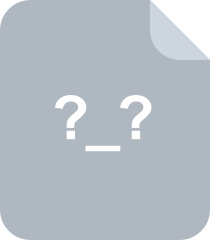
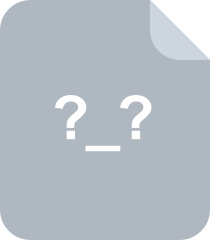
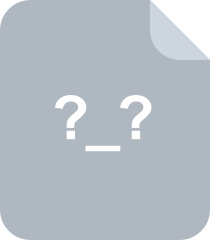
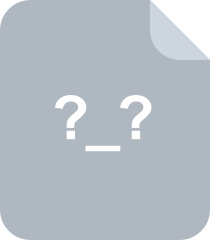
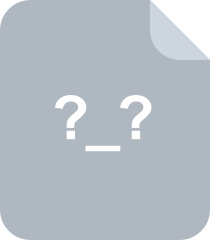
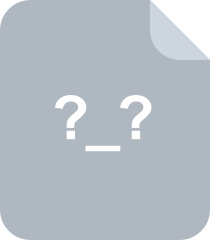
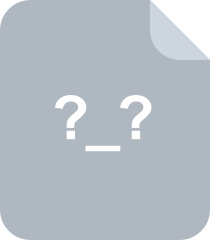
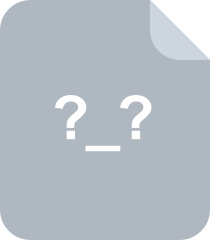
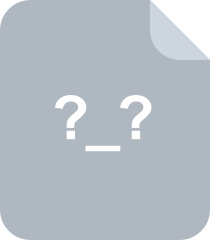
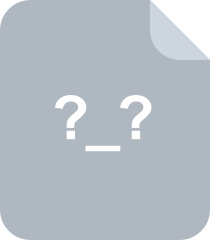
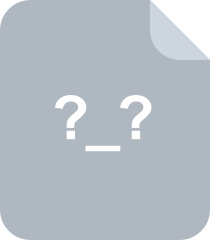
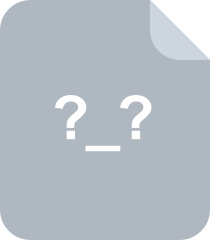
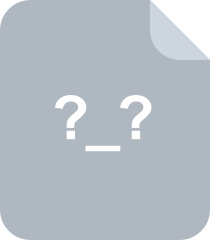
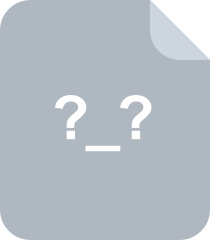
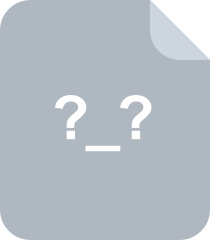
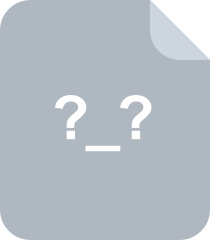
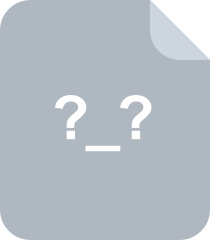
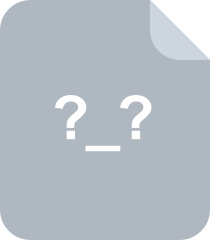
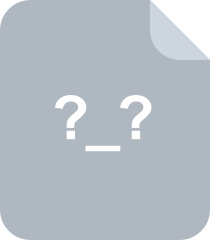
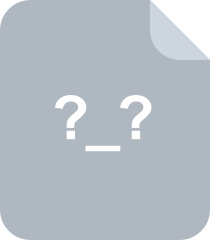
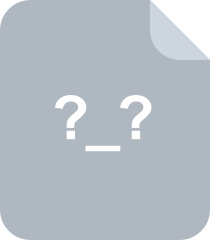
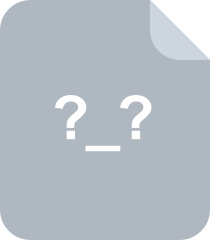
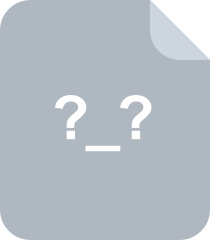
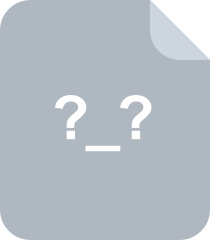
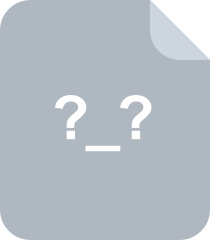
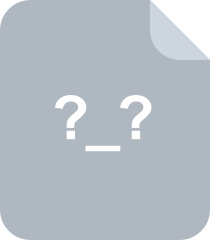
共 189 条
- 1
- 2
资源评论
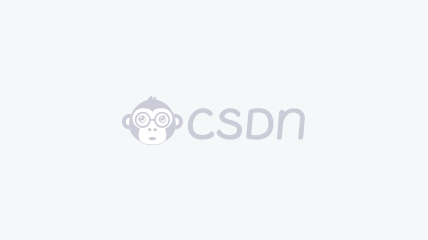

极客11
- 粉丝: 385
- 资源: 5519
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

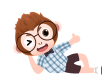
最新资源
- Chrome代理 switchyOmega
- GVC-全球价值链参与地位指数,基于ICIO表,(Wang等 2017a)计算方法
- 易语言ADS指纹浏览器管理工具
- 易语言奇易模块5.3.6
- cad定制家具平面图工具-(FG)门板覆盖柜体
- asp.net 原生js代码及HTML实现多文件分片上传功能(自定义上传文件大小、文件上传类型)
- whl@pip install pyaudio ERROR: Failed building wheel for pyaudio
- Constantsfd密钥和权限集合.kt
- 基于Java的财务报销管理系统后端开发源码
- 基于Python核心技术的cola项目设计源码介绍
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


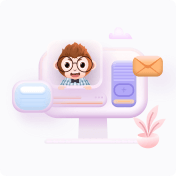
安全验证
文档复制为VIP权益,开通VIP直接复制
