import sys
import os
import random
from PyQt5 import QtWidgets, QtGui, QtCore
class DeskPet(QtWidgets.QLabel):
def __init__(self):
super().__init__()
self.initUI()
self.childPets = []
self.isDragging = False
self.isMoving = False
self.change = False
def initUI(self):
self.setWindowFlags(QtCore.Qt.FramelessWindowHint | QtCore.Qt.WindowStaysOnTopHint)
self.setAttribute(QtCore.Qt.WA_TranslucentBackground)
self.setGeometry(500, 500, 130, 130)
self.currentAction = self.startIdle
self.timer = QtCore.QTimer(self)
self.timer.timeout.connect(self.updateAnimation)
self.changeDirectionTimer = QtCore.QTimer(self) # 添加定时器
self.changeDirectionTimer.timeout.connect(self.changeDirection) # 定时器触发时调用changeDirection方法
self.startIdle()
self.setContextMenuPolicy(QtCore.Qt.CustomContextMenu)
self.customContextMenuRequested.connect(self.showMenu)
self.setMouseTracking(True)
self.dragging = False
def loadImages(self, path):
return [QtGui.QPixmap(os.path.join(path, f)) for f in os.listdir(path) if f.endswith('.png')]
def startIdle(self):
self.setFixedSize(130, 130)
self.currentAction = self.startIdle
self.images = self.loadImages("Deskpet/resource/xianzhi")
self.currentImage = 0
self.timer.start(100)
self.moveSpeed = 0
self.movingDirection = 0
if self.changeDirectionTimer.isActive():
self.changeDirectionTimer.stop() # 停止方向改变的定时器
def startWalk(self):
self.setFixedSize(130, 130)
if not self.isDragging:
self.currentAction = self.startWalk
direction = random.choice(["zuo", "you"])
self.images = self.loadImages(f"Deskpet/resource/sanbu/{direction}")
self.currentImage = 0
self.movingDirection = -1 if direction == "zuo" else 1
self.moveSpeed = 10
self.timer.start(100)
self.changeDirectionTimer.start(3000) # 启动定时器
def movePet(self):
screen = QtWidgets.QDesktopWidget().screenGeometry()
new_x = self.x() + self.movingDirection * self.moveSpeed
if new_x < 10:
new_x = 10
if self.currentAction == self.startWalk:
self.movingDirection *= -1
# 停止加载原先的图片
self.timer.stop()
self.images = [] # 清空当前图片列表
if self.movingDirection == -1: # 向左移动
self.images = self.loadImages("Deskpet/resource/sanbu/zuo")
else: # 向右移动
self.images = self.loadImages("Deskpet/resource/sanbu/you")
self.currentImage = 0
self.timer.start(100)
elif new_x > screen.width() - self.width() - 10:
new_x = screen.width() - self.width() - 10
if self.currentAction == self.startWalk:
self.movingDirection *= -1
# 停止加载原先的图片
self.timer.stop()
self.images = [] # 清空当前图片列表
# 根据移动方向加载对应的图片
if self.movingDirection == -1: # 向左移动
self.images = self.loadImages("Deskpet/resource/sanbu/zuo")
else: # 向右移动
self.images = self.loadImages("Deskpet/resource/sanbu/you")
self.currentImage = 0
self.timer.start(100)
self.deskpet_rect = self.geometry()
for child in self.childPets:
if isinstance(child, XiaobaiWindow):
self.xiaobai_rect = child.geometry()
if self.deskpet_rect.intersects(self.xiaobai_rect):
child.close()
self.startMeet()
self.move(new_x, self.y())
def startMeet(self):
self.setFixedSize(150, 150)
self.currentAction = self.startMeet
self.images = self.loadImages("Deskpet/resource/meet")
self.currentImage = 0
self.moveSpeed = 0
self.movingDirection = 0
self.timer.start(30)
def startLift(self):
self.setFixedSize(160, 160)
self.currentAction = self.startLift
self.images = self.loadImages("Deskpet/resource/linqi")
self.currentImage = 0
self.moveSpeed = 0
self.movingDirection = 0
self.timer.start(100)
def startFall(self):
self.setFixedSize(150, 150)
self.currentAction = self.startFall
self.images = self.loadImages("Deskpet/resource/xialuo")
self.currentImage = 0
self.movingDirection = 0
self.moveSpeed = 5
self.stopOtherActions()
self.timer.start(30)
def stopOtherActions(self):
self.timer.stop()
if self.currentAction == self.startWalk:
self.changeDirectionTimer.stop() # 停止方向判定定时器
self.startIdle()
elif self.currentAction == self.startLift:
self.startIdle()
elif self.currentAction == self.startFall:
pass
else:
self.startIdle()
def updateAnimation(self):
self.setPixmap(self.images[self.currentImage])
self.currentImage = (self.currentImage + 1) % len(self.images)
if hasattr(self, 'movingDirection'):
if self.currentAction == self.startFall:
self.fallPet()
else:
self.movePet()
def fallPet(self):
self.setFixedSize(130, 130)
screen = QtWidgets.QDesktopWidget().screenGeometry()
new_y = self.y() + self.moveSpeed
if new_y > screen.height() - self.height() - 10:
new_y = screen.height() - self.height() - 10
self.timer.stop()
self.startIdle()
self.move(self.x(), new_y)
def showMenu(self, position):
menu = QtWidgets.QMenu()
if self.currentAction == self.sleep:
menu.addAction("偷吃宵夜", self.Snack)
menu.addAction("唤醒", self.WakeUp)
menu.addSeparator()
menu.addAction("隐藏", self.minimizeWindow)
menu.addAction("退出", self.close)
else:
menu.addAction("散步", self.startWalk)
menu.addAction("下落", self.startFall)
menu.addAction("运动", self.exercise)
menu.addAction("吃饭", self.eating)
menu.addAction("睡觉", self.sleep)
menu.addAction("屁屁舞", self.pipi)
menu.addAction("分身术", self.clonePet)
menu.addAction("动感光波!", self.transform)
menu.addAction("呼唤小白", self.summonXiaobai)
menu.addAction("测试", self.startMeet)
child_menu = menu.addMenu("小彩蛋")
child_menu.addAction("开发者的Q/A", self.starttalk)
child_menu.addAction("小游戏", self.transform)
menu.addSeparator()
menu.addAction("停止", self.startIdle)
menu.addAction("隐藏", self.minimizeWindow)
menu.addAction("退出", self.close)
menu.exec_(self.mapToGlobal(position))
def Snack(self):
self.setFixedSize(160, 130)
self.currentAction = self.sleep
self.images = self.loadImages("Deskpet/resource/snack")
self.currentImage = 0
self.timer.start(100)
self.moveSpeed = 0
self.movingDirection = 0
QtCore.QTimer.singleShot(len(self.images) * 100, self.sleep)
def transform(self):
self.setFixedSize(160, 130)
self.currentAction
没有合适的资源?快使用搜索试试~ 我知道了~
蜡笔小新桌面宠物(1).zip
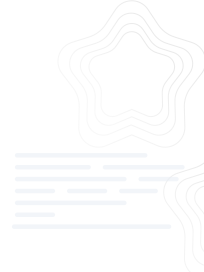
共865个文件
png:863个
py:1个
exe:1个

需积分: 0 0 下载量 87 浏览量
更新于2024-12-16
收藏 16.21MB ZIP 举报
蜡笔小新桌面宠物(1).zip
收起资源包目录

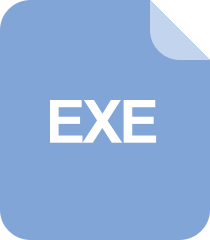
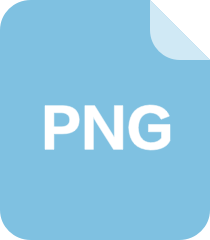
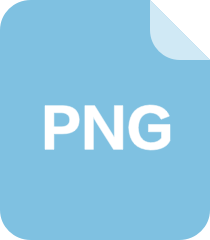
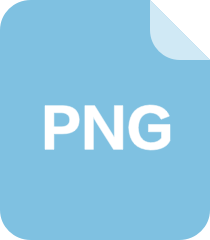
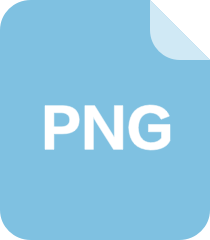
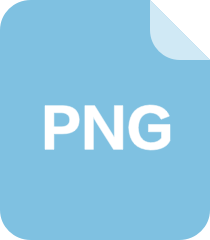
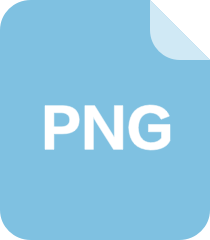
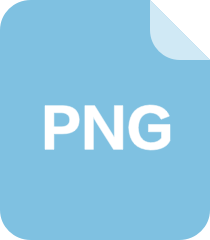
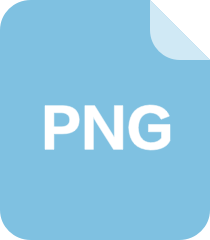
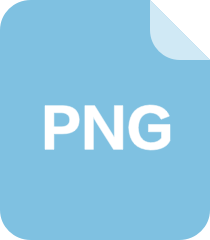
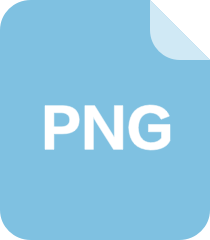
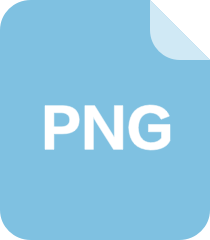
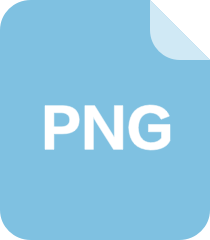
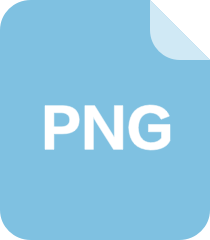
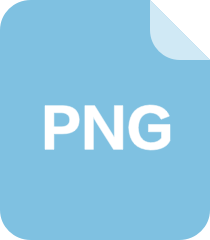
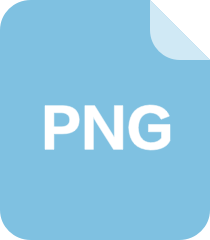
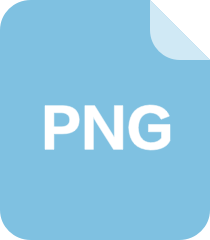
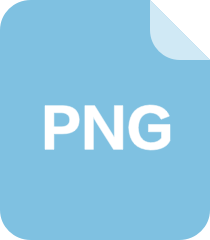
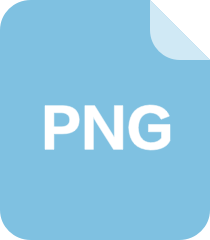
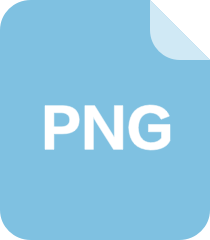
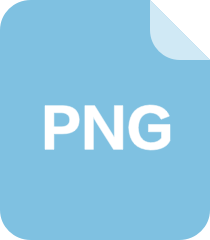
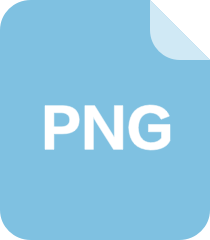
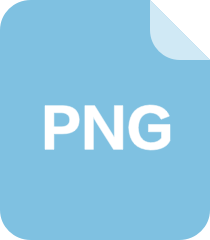
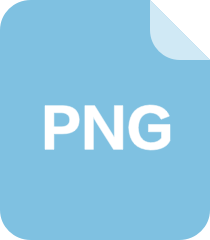
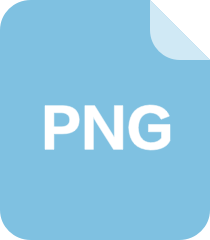
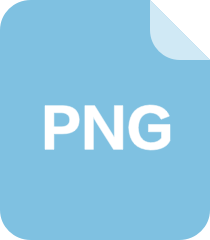
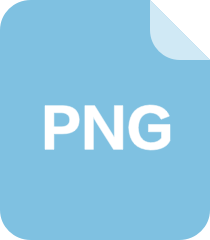
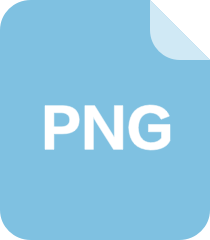
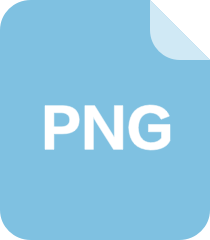
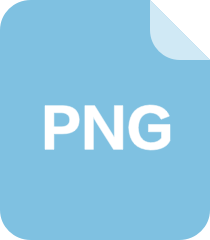
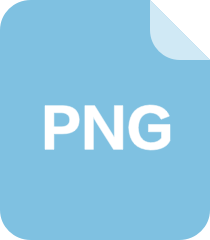
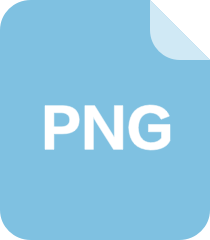
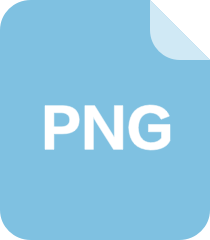
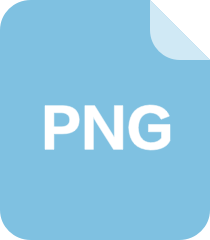
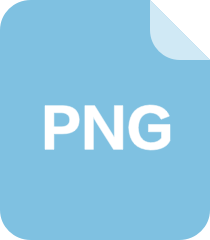
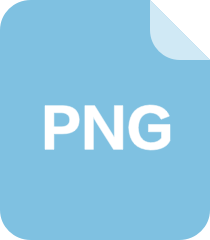
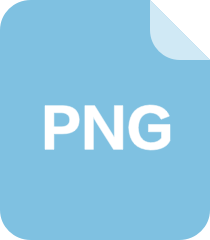
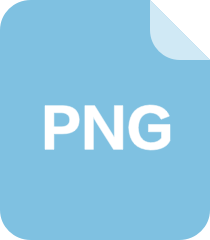
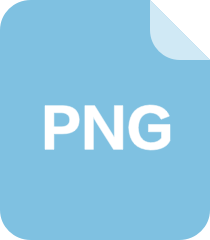
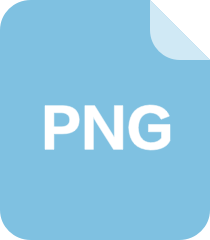
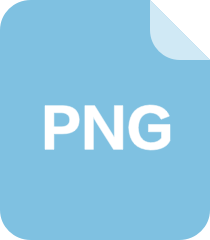
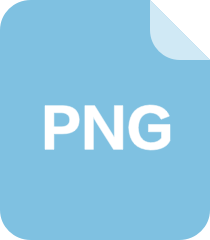
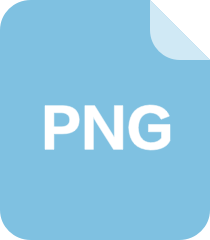
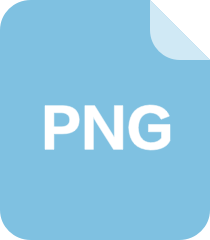
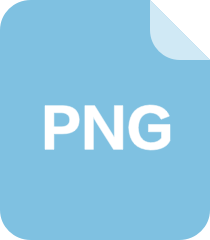
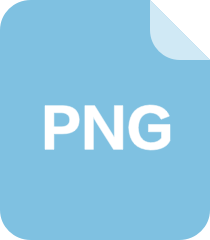
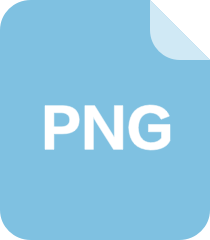
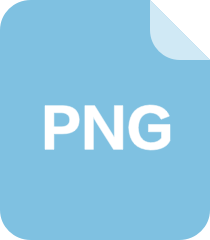
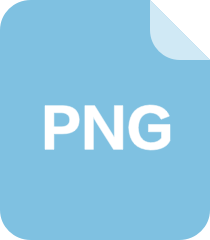
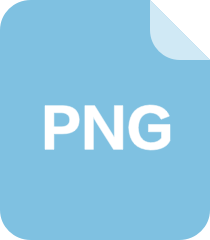
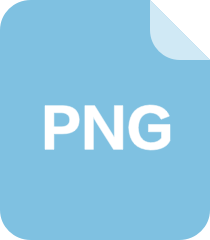
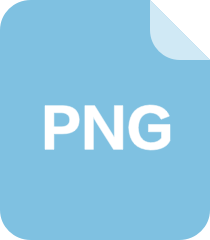
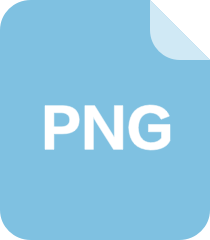
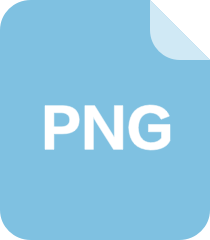
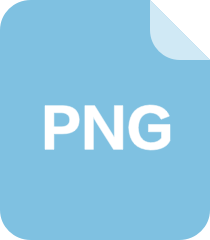
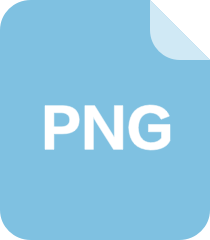
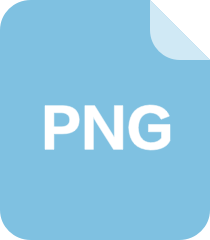
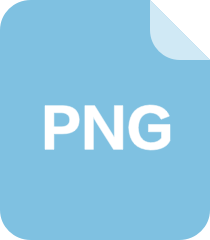
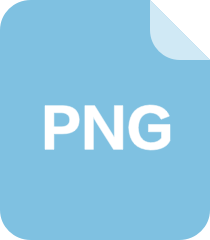
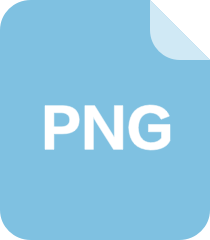
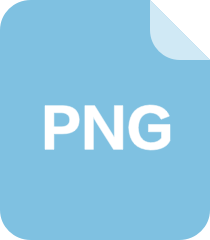
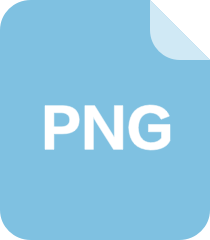
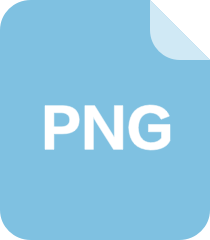
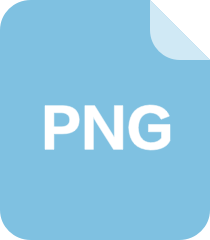
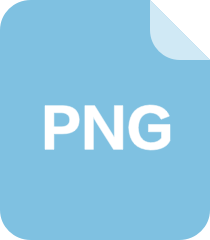
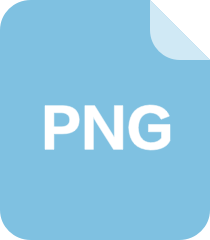
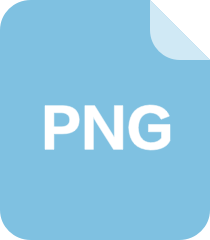
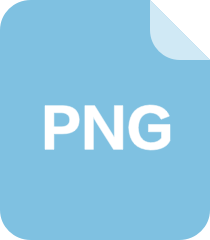
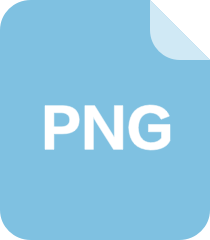
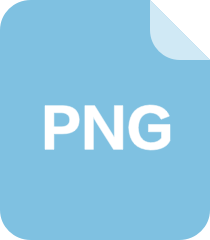
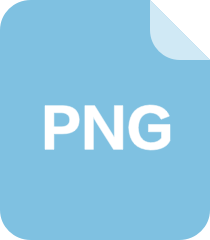
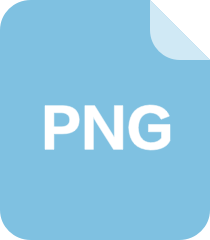
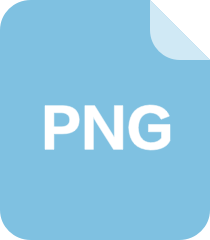
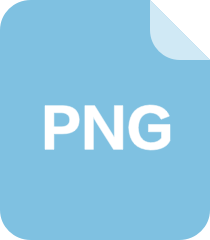
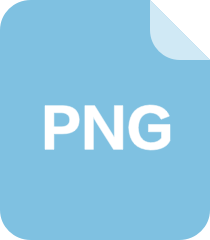
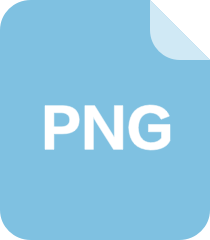
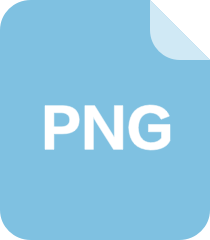
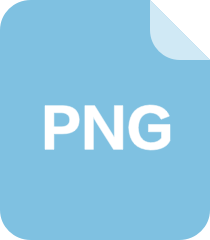
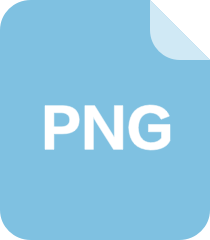
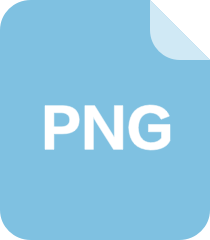
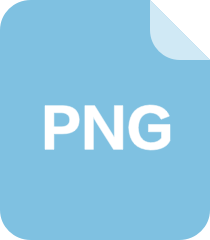
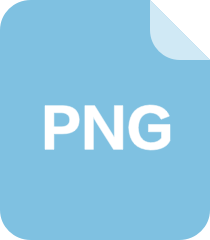
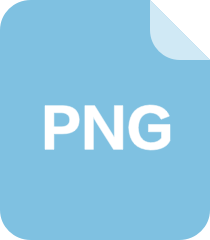
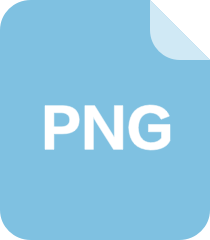
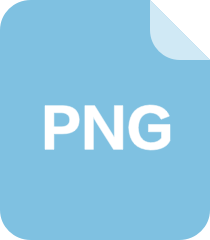
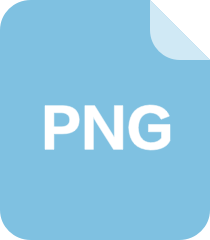
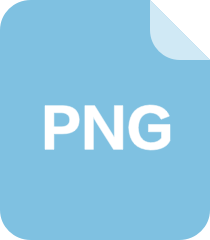
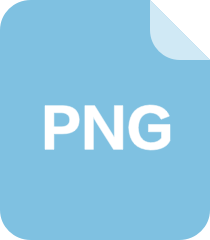
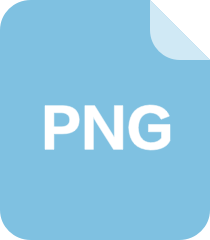
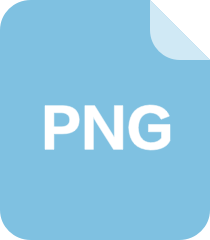
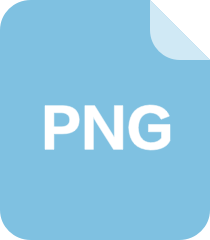
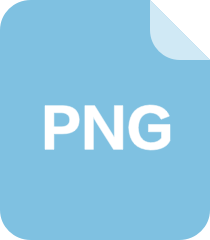
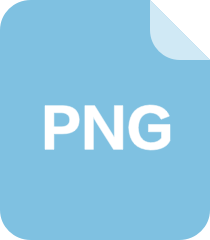
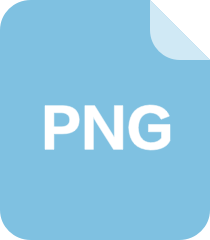
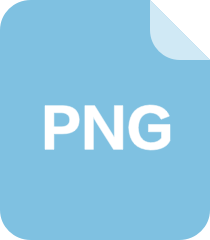
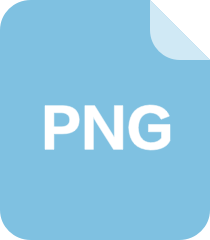
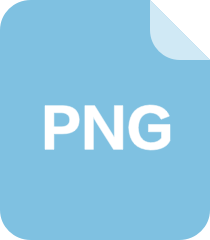
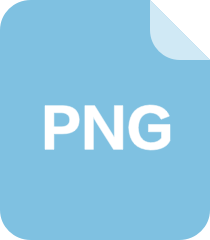
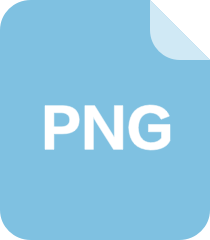
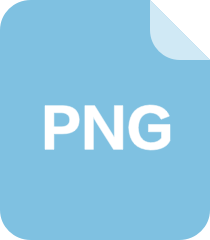
共 865 条
- 1
- 2
- 3
- 4
- 5
- 6
- 9
资源推荐
资源预览
资源评论
187 浏览量
180 浏览量
155 浏览量
137 浏览量
186 浏览量

176 浏览量


2021-08-30 上传
2023-01-01 上传
166 浏览量
195 浏览量

196 浏览量
2021-08-23 上传
2013-05-28 上传
2021-09-13 上传
2019-07-12 上传
125 浏览量
129 浏览量
177 浏览量
151 浏览量
2019-07-11 上传
资源评论
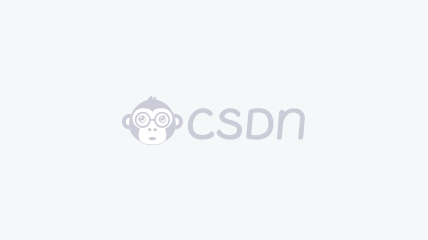

日富一日851
- 粉丝: 0
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

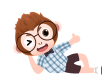
最新资源
- 免费SQL Server数据库监控工具
- 02-Kibana 构建高级可视化 包春喜 北京 20241214
- iview467-plugins-x64.zip
- 垃圾检测52-YOLO(v5至v8)、COCO、CreateML、Darknet、Paligemma数据集合集.rar
- 编写一个简单的个人记账本程序,可以记录收入和支出,并计算总余额
- JavaWeb环境下通过代理设置Firebase Admin SDK全攻略
- 编写一个Python程序,实现从文件中读取数据,并在数据末尾添加新内容后保存
- JavaScript锅打灰太狼小游戏项目源码
- C++嵌入式设备版opencv做图像模糊处理.zip
- 编写的一个简单的计算器程序,能够实现加、减、乘、除四种基本运算
安全验证
文档复制为VIP权益,开通VIP直接复制
