Documentation for class Archive_Tar
===================================
Last update : 2001-08-15
Overview :
----------
The Archive_Tar class helps in creating and managing GNU TAR format
files compressed by GNU ZIP or not.
The class offers basic functions like creating an archive, adding
files in the archive, extracting files from the archive and listing
the archive content.
It also provide advanced functions that allow the adding and
extraction of files with path manipulation.
Sample :
--------
// ----- Creating the object (uncompressed archive)
$tar_object = new Archive_Tar("tarname.tar");
$tar_object->setErrorHandling(PEAR_ERROR_PRINT);
// ----- Creating the archive
$v_list[0]="file.txt";
$v_list[1]="data/";
$v_list[2]="file.log";
$tar_object->create($v_list);
// ----- Adding files
$v_list[0]="dev/file.txt";
$v_list[1]="dev/data/";
$v_list[2]="log/file.log";
$tar_object->add($v_list);
// ----- Adding more files
$tar_object->add("release/newfile.log release/readme.txt");
// ----- Listing the content
if (($v_list = $tar_object->listContent()) != 0)
for ($i=0; $i<sizeof($v_list); $i++)
{
echo "Filename :'".$v_list[$i][filename]."'<br>";
echo " .size :'".$v_list[$i][size]."'<br>";
echo " .mtime :'".$v_list[$i][mtime]."' (".date("l dS of F Y h:i:s A", $v_list[$i][mtime]).")<br>";
echo " .mode :'".$v_list[$i][mode]."'<br>";
echo " .uid :'".$v_list[$i][uid]."'<br>";
echo " .gid :'".$v_list[$i][gid]."'<br>";
echo " .typeflag :'".$v_list[$i][typeflag]."'<br>";
}
// ----- Extracting the archive in directory "install"
$tar_object->extract("install");
Public arguments :
------------------
None
Public Methods :
----------------
Method : Archive_Tar($p_tarname, $compress = null)
Description :
Archive_Tar Class constructor. This flavour of the constructor only
declare a new Archive_Tar object, identifying it by the name of the
tar file.
If the compress argument is set the tar will be read or created as a
gzip or bz2 compressed TAR file.
Arguments :
$p_tarname : A valid filename for the tar archive file.
$p_compress : can be null, 'gz' or 'bz2'. For
compatibility reason it can also be true. This
parameter indicates if gzip or bz2 compression
is required.
Return value :
The Archive_Tar object.
Sample :
$tar_object = new Archive_Tar("tarname.tar");
$tar_object_compressed = new Archive_Tar("tarname.tgz", true);
How it works :
Initialize the object.
Method : create($p_filelist)
Description :
This method creates the archive file and add the files / directories
that are listed in $p_filelist.
If the file already exists and is writable, it is replaced by the
new tar. It is a create and not an add. If the file exists and is
read-only or is a directory it is not replaced. The method return
false and a PEAR error text.
The $p_filelist parameter can be an array of string, each string
representing a filename or a directory name with their path if
needed. It can also be a single string with names separated by a
single blank.
See also createModify() method for more details.
Arguments :
$p_filelist : An array of filenames and directory names, or a single
string with names separated by a single blank space.
Return value :
true on success, false on error.
Sample 1 :
$tar_object = new Archive_Tar("tarname.tar");
$tar_object->setErrorHandling(PEAR_ERROR_PRINT); // Optional error handling
$v_list[0]="file.txt";
$v_list[1]="data/"; (Optional '/' at the end)
$v_list[2]="file.log";
$tar_object->create($v_list);
Sample 2 :
$tar_object = new Archive_Tar("tarname.tar");
$tar_object->setErrorHandling(PEAR_ERROR_PRINT); // Optional error handling
$tar_object->create("file.txt data/ file.log");
How it works :
Just calling the createModify() method with the right parameters.
Method : createModify($p_filelist, $p_add_dir, $p_remove_dir = "")
Description :
This method creates the archive file and add the files / directories
that are listed in $p_filelist.
If the file already exists and is writable, it is replaced by the
new tar. It is a create and not an add. If the file exists and is
read-only or is a directory it is not replaced. The method return
false and a PEAR error text.
The $p_filelist parameter can be an array of string, each string
representing a filename or a directory name with their path if
needed. It can also be a single string with names separated by a
single blank.
The path indicated in $p_remove_dir will be removed from the
memorized path of each file / directory listed when this path
exists. By default nothing is removed (empty path "")
The path indicated in $p_add_dir will be added at the beginning of
the memorized path of each file / directory listed. However it can
be set to empty "". The adding of a path is done after the removing
of path.
The path add/remove ability enables the user to prepare an archive
for extraction in a different path than the origin files are.
See also addModify() method for file adding properties.
Arguments :
$p_filelist : An array of filenames and directory names, or a single
string with names separated by a single blank space.
$p_add_dir : A string which contains a path to be added to the
memorized path of each element in the list.
$p_remove_dir : A string which contains a path to be removed from
the memorized path of each element in the list, when
relevant.
Return value :
true on success, false on error.
Sample 1 :
$tar_object = new Archive_Tar("tarname.tar");
$tar_object->setErrorHandling(PEAR_ERROR_PRINT); // Optional error handling
$v_list[0]="file.txt";
$v_list[1]="data/"; (Optional '/' at the end)
$v_list[2]="file.log";
$tar_object->createModify($v_list, "install");
// files are stored in the archive as :
// install/file.txt
// install/data
// install/data/file1.txt
// install/data/... all the files and sub-dirs of data/
// install/file.log
Sample 2 :
$tar_object = new Archive_Tar("tarname.tar");
$tar_object->setErrorHandling(PEAR_ERROR_PRINT); // Optional error handling
$v_list[0]="dev/file.txt";
$v_list[1]="dev/data/"; (Optional '/' at the end)
$v_list[2]="log/file.log";
$tar_object->createModify($v_list, "install", "dev");
// files are stored in the archive as :
// install/file.txt
// install/data
// install/data/file1.txt
// install/data/... all the files and sub-dirs of data/
// install/log/file.log
How it works :
Open the file in write mode (erasing the existing one if one),
call the _addList() method for adding the files in an empty archive,
add the tar footer (512 bytes block), close the tar file.
Method : addModify($p_filelist, $p_add_dir, $p_remove_dir="")
Description :
This method add the files / directories listed in $p_filelist at the
end of the existing archive. If the archive does not yet exists it
is created.
The $p_filelist parameter can be an array of string, each string
representing a filename or a directory name with their path if
needed. It can also be a single string with names separated by a
single blank.
The path indicated in $p_remove_dir will be removed from the
memorized path of each file / directory listed when this path
exists. By default nothing is removed (empty path "")
The path indicated in $p_add_dir will be added at the beginning of
the memorized path of each file / directory listed. However it can
be set to empty "". The adding of a path is done after the removing
of path.
The path add/remove ability enables the user to prepare an archive
没有合适的资源?快使用搜索试试~ 我知道了~
基于PHP实现的WEB图片共享系统(源代码)
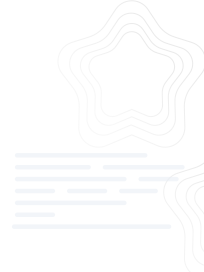
共1390个文件
js:306个
php:266个
gif:245个

需积分: 1 0 下载量 24 浏览量
2024-05-25
21:32:13
上传
评论
收藏 5.49MB RAR 举报
温馨提示
本系统主要从现代社会电脑化观念出发,通过对现有资料的分析、研究和整理,确定了在基于现存的WEB2.0模式下开发图片共享系统的可行性、紧迫性和必要性。在现阶段,国内基于WEB2.0的图片共享系统才刚起步,该市场还有很大的介入空间。其中,在国外,已经有了很成熟的图片共享平台。在WEB2.0时代,信息由以前的服务器发布变成了用户发布。也就是从以前的通过服务器搜集资源并且发布变成了通过用户提供资源,服务器进行整理,分类,发布的模式。而且这种模式对于一个网站的用户吸引度也远远高于传统模式。
资源推荐
资源详情
资源评论
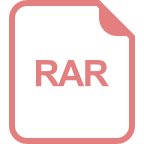
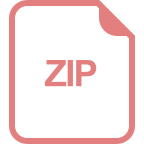
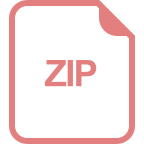
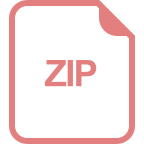
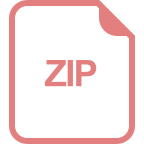
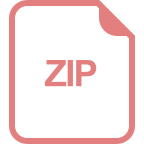
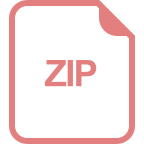
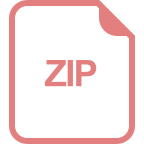
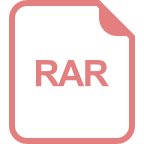
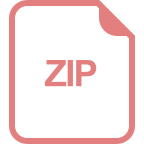
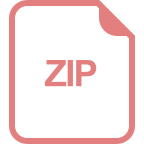
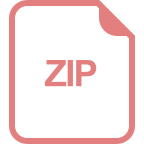
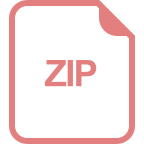
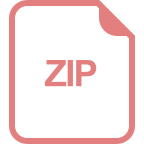
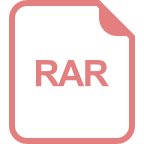
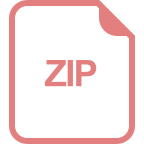
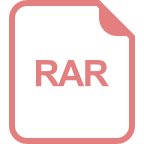
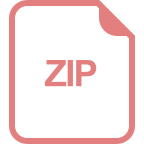
收起资源包目录

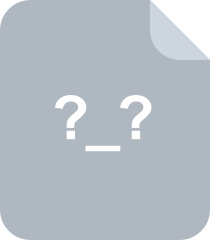
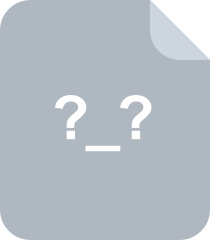
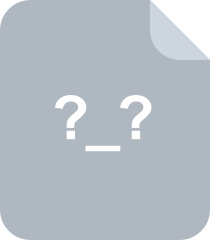
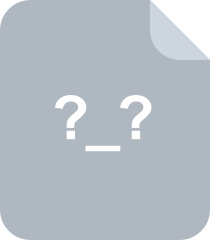
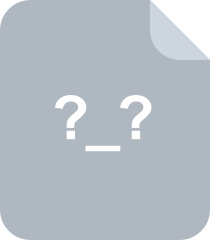
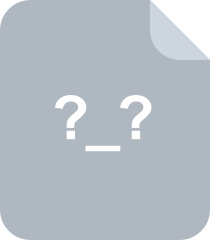
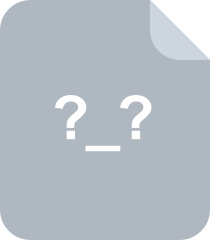
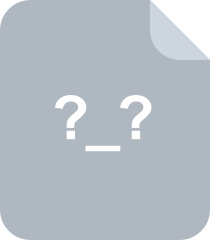
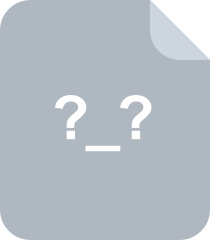
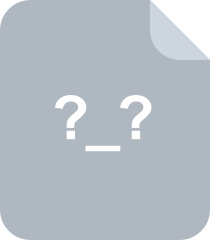
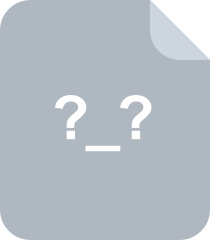
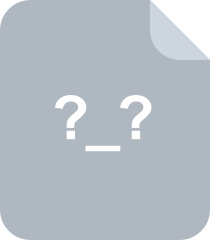
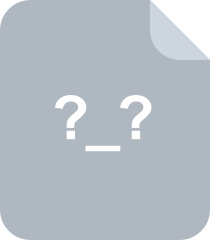
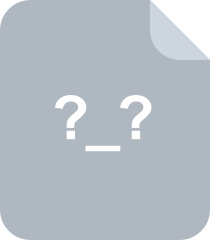
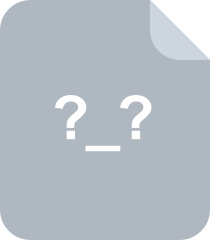
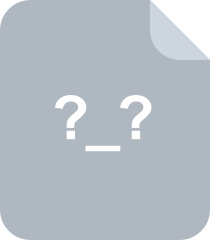
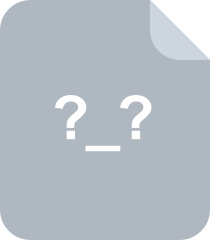
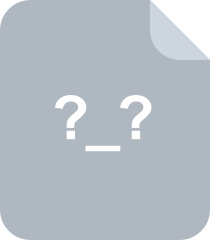
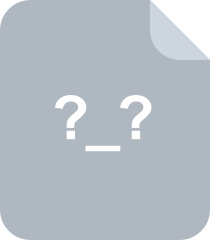
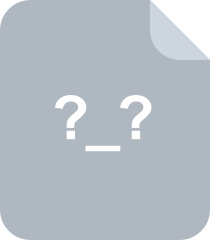
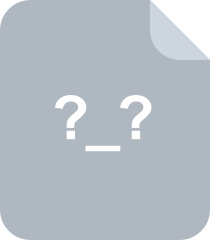
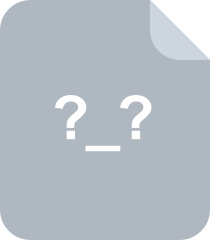
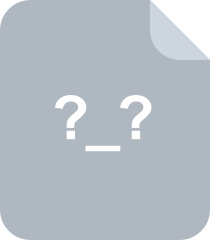
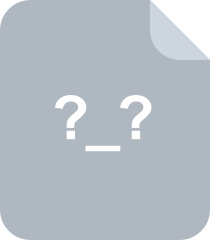
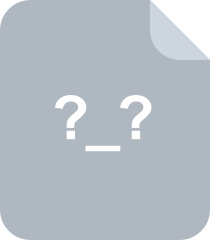
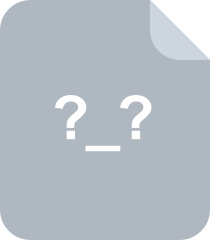
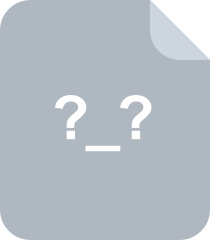
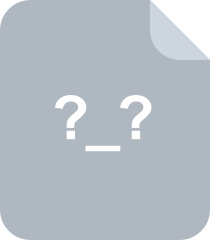
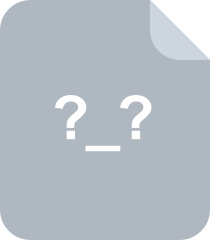
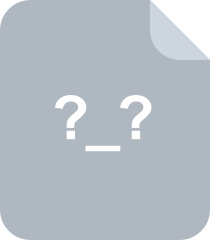
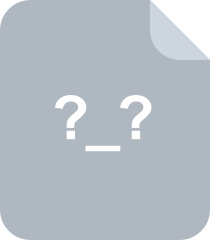
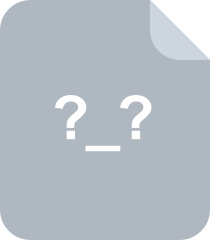
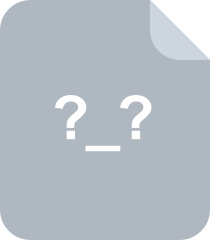
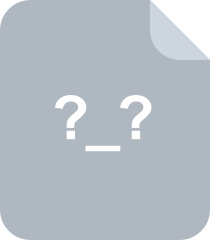
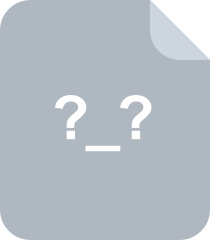
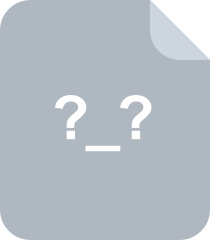
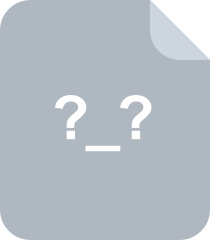
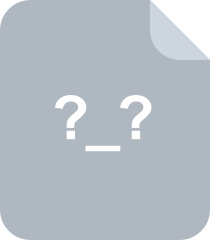
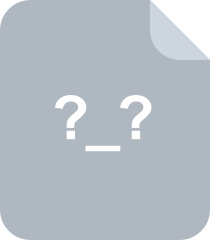
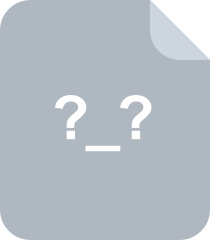
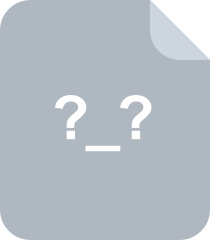
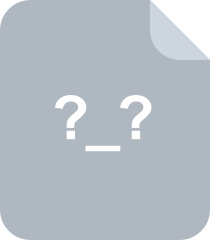
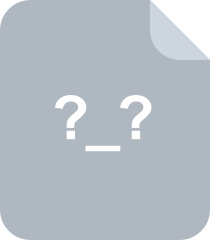
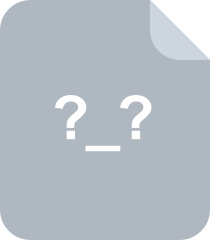
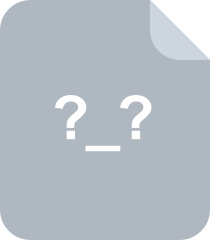
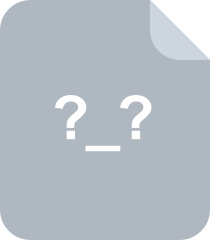
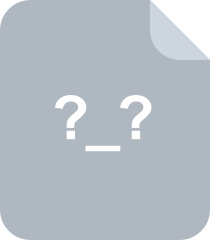
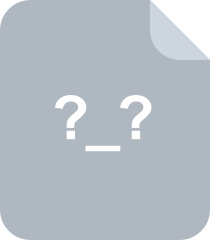
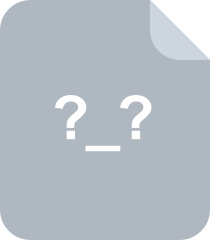
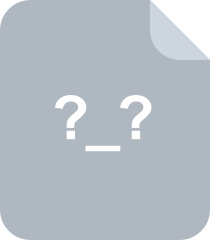
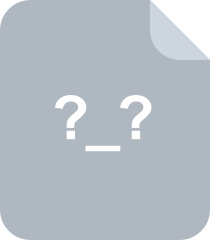
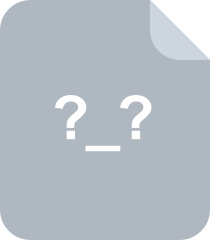
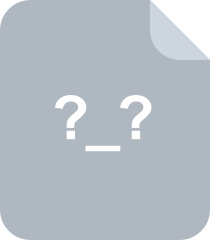
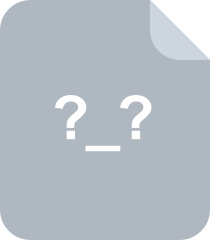
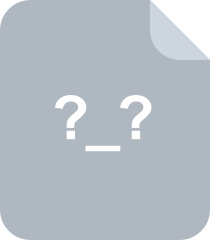
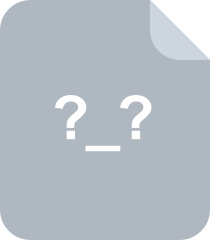
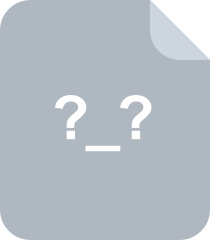
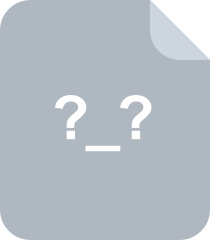
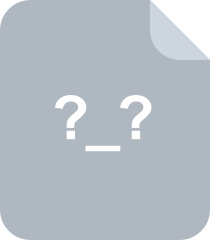
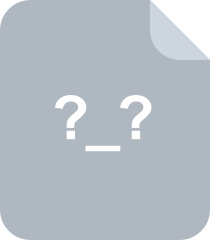
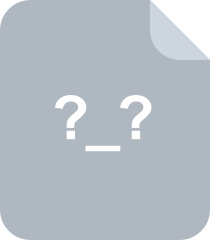
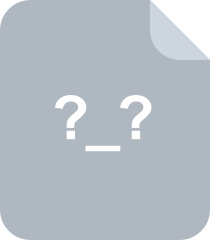
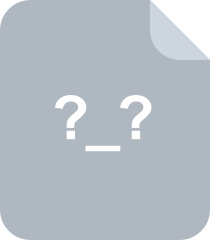
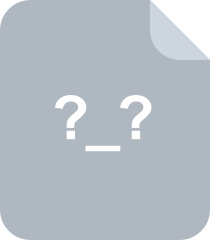
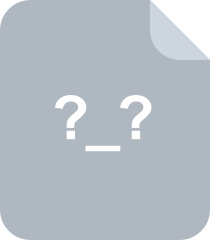
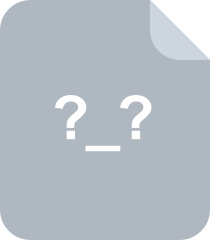
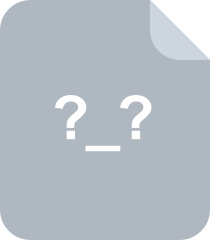
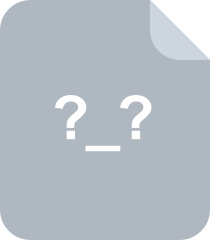
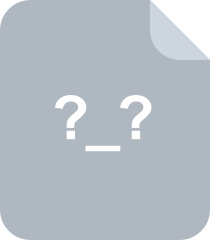
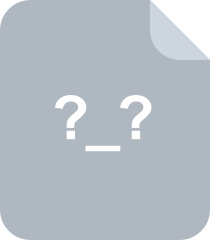
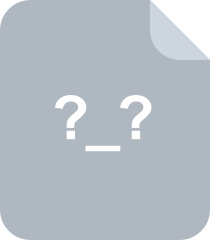
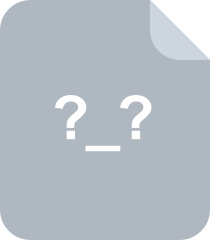
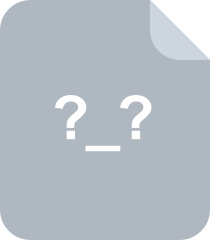
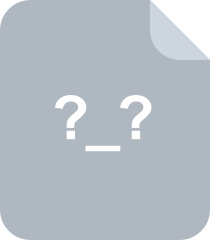
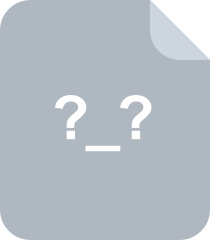
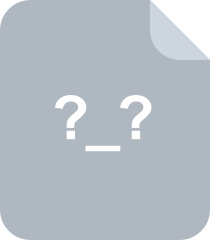
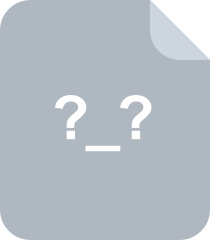
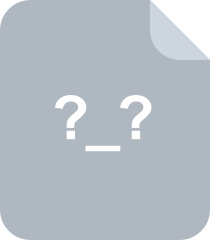
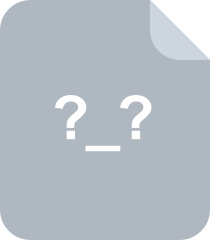
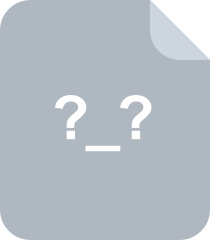
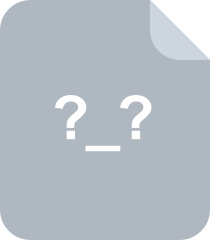
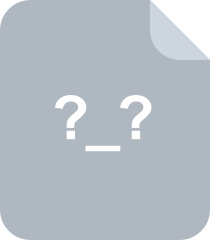
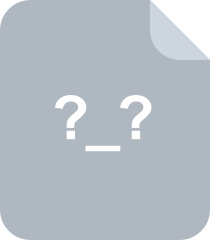
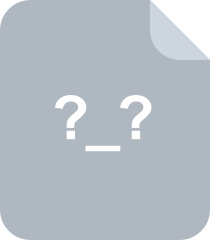
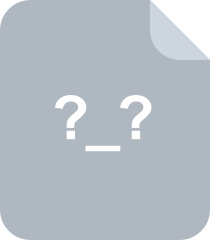
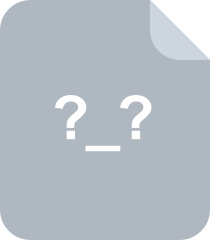
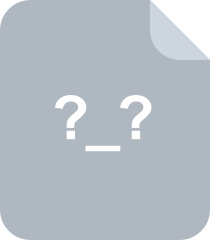
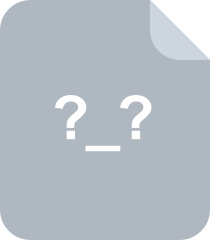
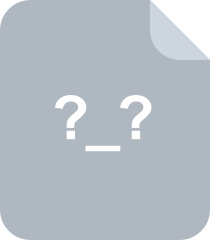
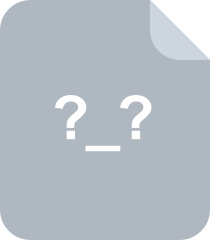
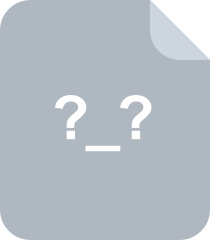
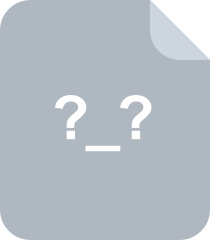
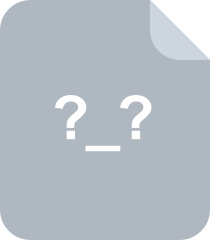
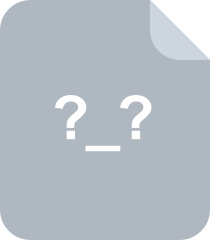
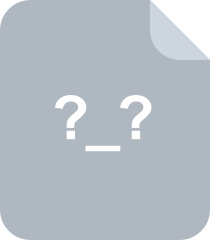
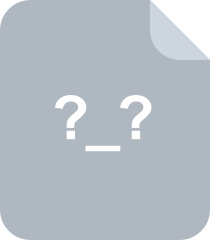
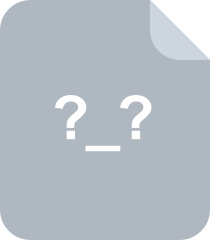
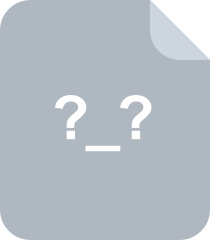
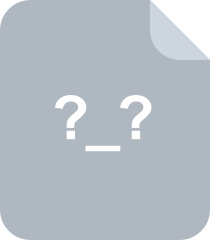
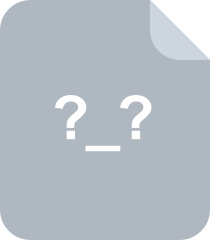
共 1390 条
- 1
- 2
- 3
- 4
- 5
- 6
- 14
资源评论
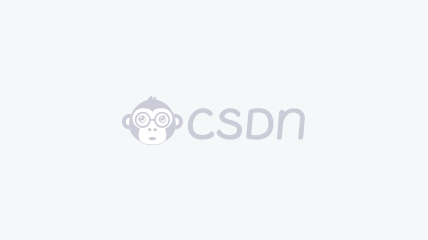

Java资深爱好者
- 粉丝: 397
- 资源: 143
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

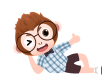
安全验证
文档复制为VIP权益,开通VIP直接复制
