package com.util;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Random;
import java.util.Set;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/*import dao.CommDAO;*/
import jxl.Workbook;
import jxl.write.Label;
import jxl.write.WritableSheet;
import jxl.write.WritableWorkbook;
import jxl.write.WriteException;
import jxl.write.biff.RowsExceededException;
public class Info {
//public static String popheight = "alliframe.height=document.body.clientHeight+";
public static String popheight = "alliframe.style.height=document.body.scrollHeight+";
public static HashMap getUser(HttpServletRequest request)
{
HashMap map = (HashMap)(request.getSession().getAttribute("admin")==null?request.getSession().getAttribute("user"):request.getSession().getAttribute("admin"));
return map;
}
public static int getBetweenDayNumber(String dateA, String dateB) {
long dayNumber = 0;
//1小时=60分钟=3600秒=3600000
long mins = 60L * 1000L;
//long day= 24L * 60L * 60L * 1000L;计算天数之差
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd HH:mm");
try {
java.util.Date d1 = df.parse(dateA);
java.util.Date d2 = df.parse(dateB);
dayNumber = (d2.getTime() - d1.getTime()) / mins;
} catch (Exception e) {
e.printStackTrace();
}
return (int) dayNumber;
}
public static void main(String[] g )
{
System.out.print(Info.getBetweenDayNumber("2012-11-11 11:19", "2012-11-11 11:11"));
}
public static String getselect(String name,String tablename,String zdname)
{
String select = "<select name=\""+name+"\" id=\""+name+"\" >";
/*for(HashMap permap:new CommDAO().select("select * from "+tablename+" order by id desc")){
select+="<option value=\""+permap.get(zdname)+"\">"+permap.get(zdname)+"</option>";
}
select+="</select>";*/
return select;
}
public static String getselect(String name,String tablename,String zdname,String where)
{
String select = "<select name=\""+name+"\" id=\""+name+"\" >";
select+="<option value=\"\">不限</option>";
/*for(HashMap permap:new CommDAO().select("select * from "+tablename+" where "+where+" order by id desc")){
String optionstr = "";
if(zdname.split(";").length==1){
optionstr=permap.get(zdname.split("~")[0]).toString();
}else{
for(String str:zdname.split(";"))
{
String zdstr = str.split("~")[0];
String zdnamestr = str.split("~")[1].equals("无名")?"":(str.split("~")[1]+":");
optionstr+=zdnamestr+permap.get(zdstr)+" - ";
}
}
if(optionstr.indexOf(" - ")>-1)optionstr=optionstr.substring(0,optionstr.length()-3);
select+="<option value=\""+optionstr+"\">"+optionstr+"</option>";
}
select+="</select>";*/
return select;
}
public static String getradio(String name,String tablename,String zdname,String where)
{
String radio="";
int dxii = 0;
/*for(HashMap permap:new CommDAO().select("select * from "+tablename+" where "+where+" order by id desc")){
String check="";
if(dxii==0)check="checked=checked";
String optionstr = "";
for(String str:zdname.split(";"))
{
String zdstr = str.split("~")[0];
String zdnamestr = str.split("~")[1].equals("无名")?"":(str.split("~")[1]+":");
optionstr+=zdnamestr+permap.get(zdstr)+" - ";
}
if(optionstr.length()>0)optionstr=optionstr.substring(0,optionstr.length()-3);
radio+="<label><input type='radio' name='"+name+"' "+check+" value=\""+optionstr+"\">"+optionstr+"</label>\n";
dxii++;
}*/
return radio;
}
public static void writeExcel(String fileName,String prosstr,java.util.List<List> plist,HttpServletRequest request, HttpServletResponse response){
WritableWorkbook wwb = null;
String cols = "";
for(String str:prosstr.split("@"))
{
cols+=str.split("-")[0]+",";
}
cols = cols.substring(0,cols.length()-1);
String where = request.getAttribute("where")==null?"":request.getAttribute("where").toString();
/* List<List> mlist = new CommDAO().selectforlist("select "+cols+" from "+fileName+" "+where+" order by id desc");*/
fileName = request.getRealPath("/")+"/upfile/"+Info.generalFileName("a.xls");
String[] pros = prosstr.split("@");
try {
//首先要使用Workbook类的工厂方法创建一个可写入的工作薄(Workbook)对象
wwb = Workbook.createWorkbook(new File(fileName));
} catch (IOException e) {
e.printStackTrace();
}
if(wwb!=null){
//创建一个可写入的工作表
//Workbook的createSheet方法有两个参数,第一个是工作表的名称,第二个是工作表在工作薄中的位置
WritableSheet ws = wwb.createSheet("sheet1", 0);
ws.setColumnView(0,20);
ws.setColumnView(1,20);
ws.setColumnView(2,20);
ws.setColumnView(3,20);
ws.setColumnView(4,20);
ws.setColumnView(5,20);
try {
for(int i=0;i<pros.length;i++)
{
Label label1 = new Label(i, 0,"");
label1.setString(pros[i]);
ws.addCell(label1);
}
} catch (RowsExceededException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
} catch (WriteException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
//下面开始添加单元格
/*int i=1;*/
/* for(List t:mlist){
try {
Iterator it = t.iterator();
int jj=0;
while(it.hasNext())
{
Label label1 = new Label(jj, i,"");
String a = it.next().toString();
label1.setString(a);
ws.addCell(label1);
jj++;
}
i++;
} catch (RowsExceededException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
} catch (WriteException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
}
try {
//从内存中写入文件中
wwb.write();
//关闭资源,释放内存
wwb.close();
} catch (IOException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
try {
response.sendRedirect("/pianotrainwebstie/upload?filename="+fileName.substring(fileName.lastIndexOf("/")+1));
} catch (IOException e) {
e.printStackTrace();*/
}
}
public static String getcheckbox(String name,String tablename,String zdname,String where)
{
String checkbox="";
/* f
没有合适的资源?快使用搜索试试~ 我知道了~
ssm_014_mysql_矿场仓储管理系统_.zip
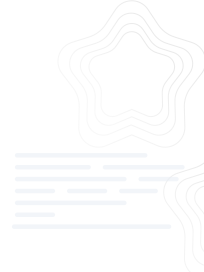
共336个文件
jar:78个
class:62个
java:62个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 107 浏览量
2024-08-08
21:23:11
上传
评论
收藏 23.22MB ZIP 举报
温馨提示
随着数字化的建设,根据当时的发展和用户的需求,选择使用矿产资源管理的信息都是可以用作示范。物质生活已经达到了人们的基本要求,人们追求生活层次越来越高,享受生活成为人们休闲娱乐的主要生活方式。人们在购买和销售中注重享受生活带来的舒适性和方便性等主观体验。因此,随着社会大潮的涌动,现状科技采用最新技术,对于矿场采用科技开发网站实现矿产矿物的提前性和高效性。客观角度上简化矿物的价格、信息、图片、时间、购买等关键步骤,解决矿场管理中出现的购买排队,购买时间,出错率高等现象,真正提高技术实现远程购买和销售的便捷性。使用JSP的开发平台实现基于WEB的矿产资源管理系统,使用户获取最新的矿物、矿产相关新闻等的矿产终端系统,实现了矿产业务的终端系统,体现了售票的鲁棒性和高效性;提供良好的人机交互页面,操作方便高效;系统反应快,维护方便,满足矿产业务的需求,提高矿物的效率。
资源推荐
资源详情
资源评论
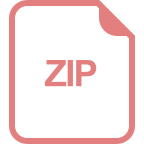
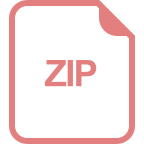
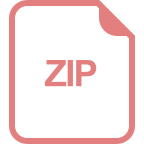
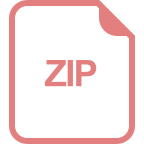
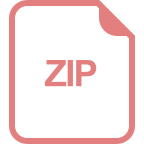
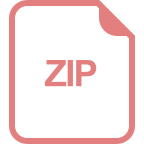
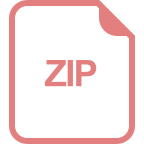
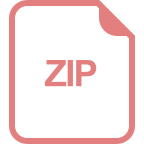
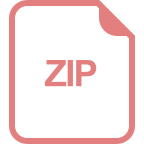
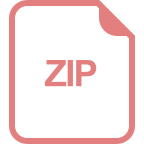
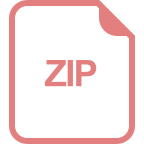
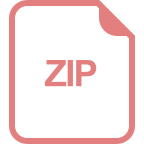
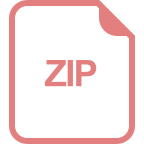
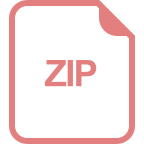
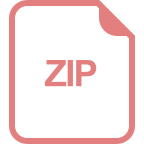
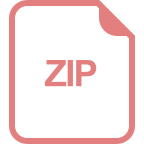
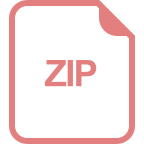
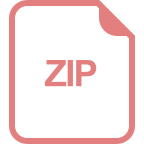
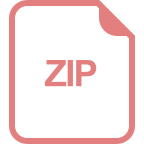
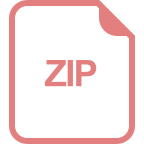
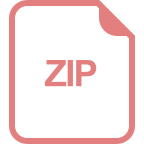
收起资源包目录

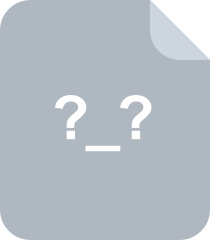
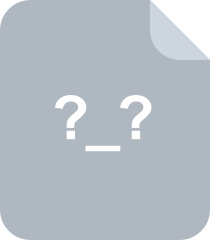
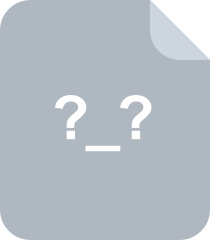
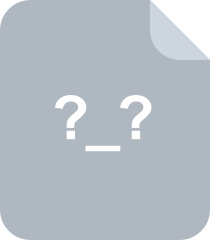
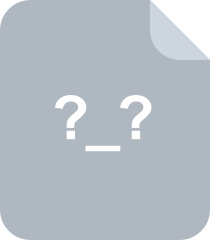
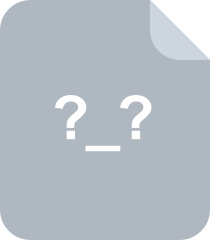
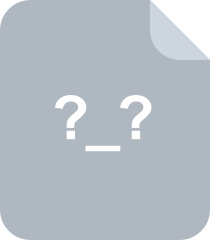
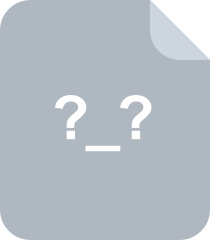
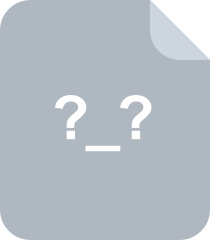
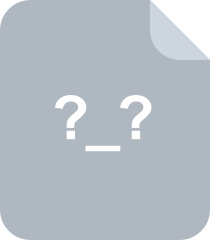
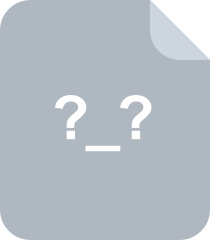
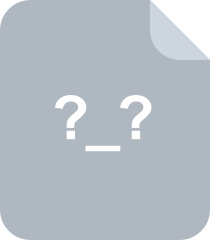
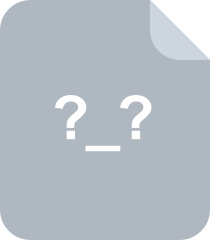
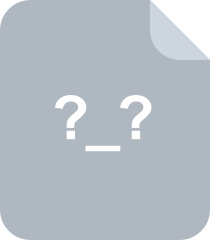
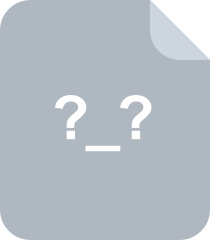
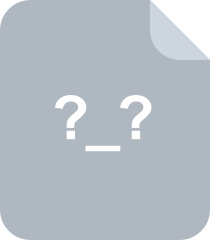
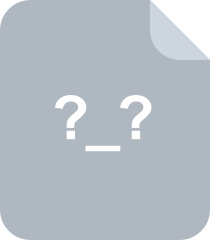
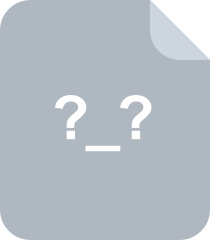
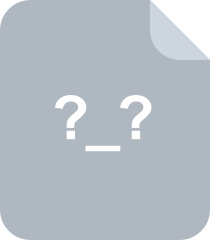
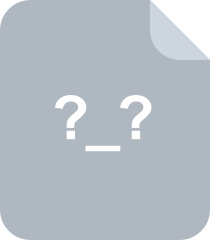
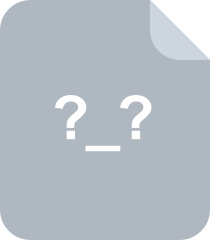
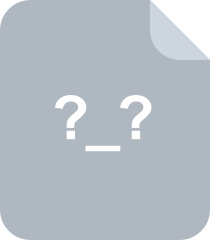
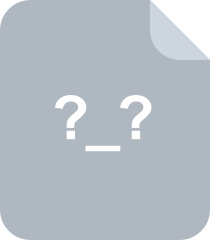
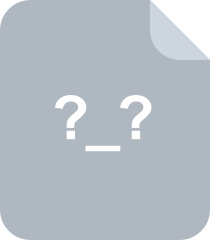
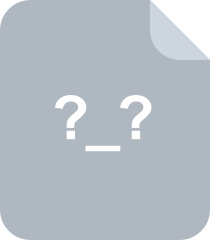
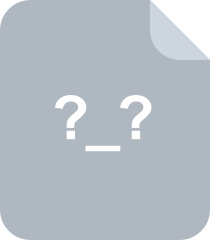
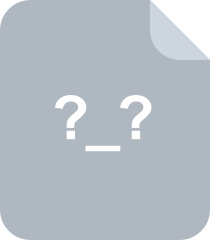
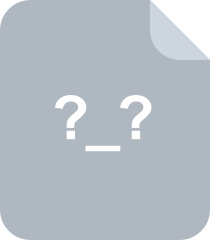
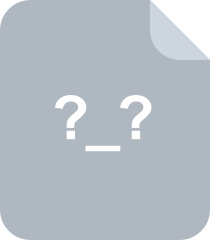
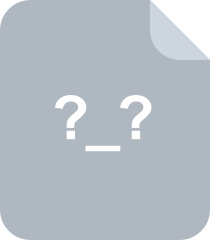
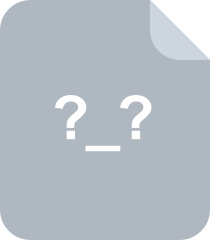
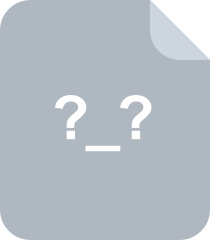
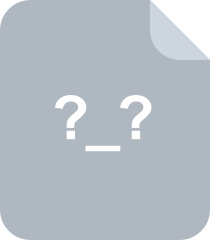
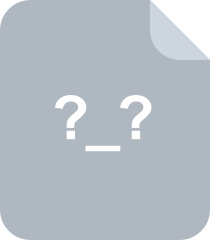
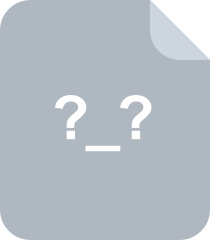
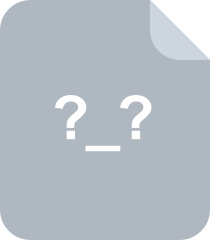
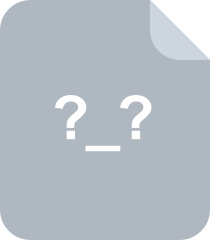
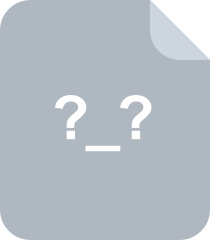
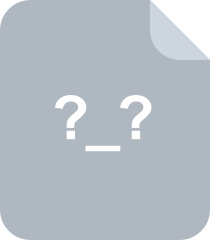
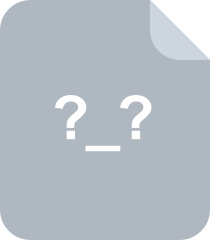
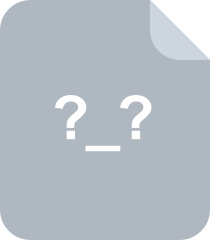
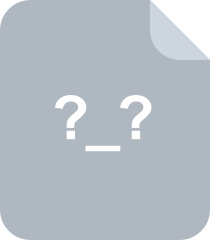
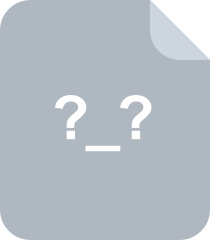
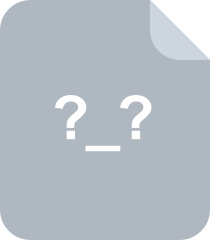
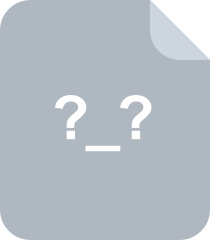
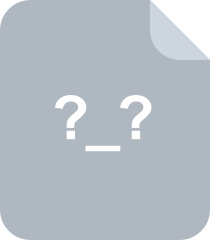
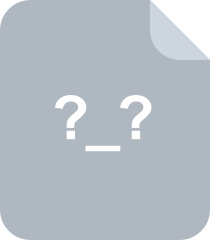
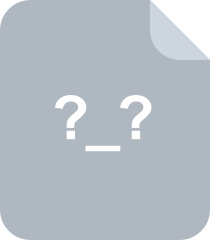
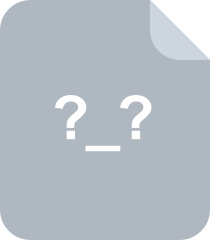
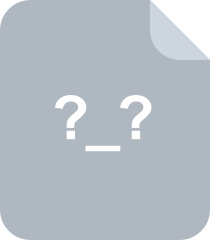
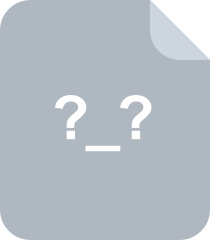
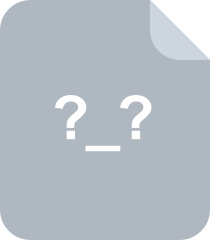
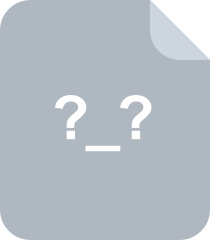
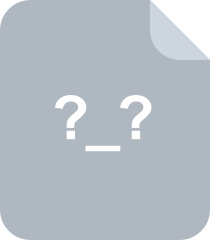
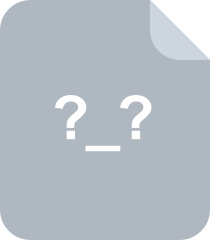
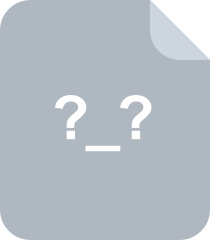
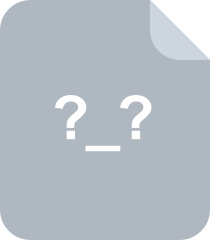
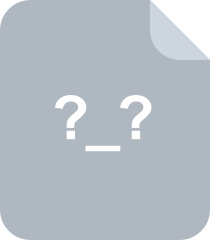
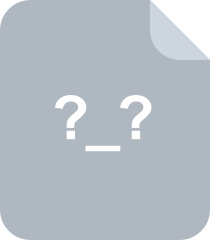
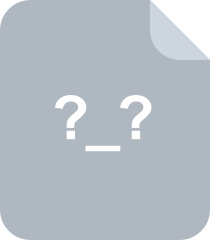
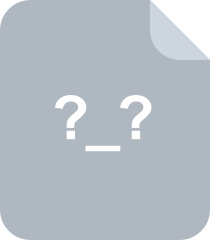
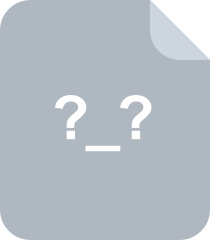
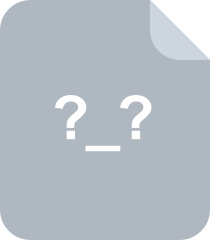
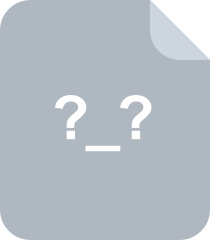
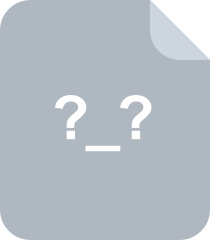
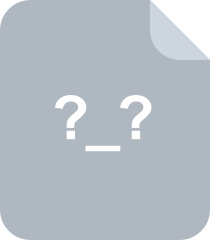
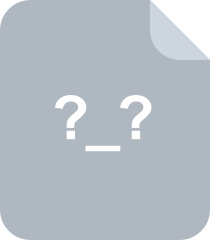
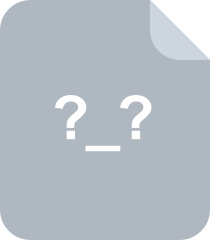
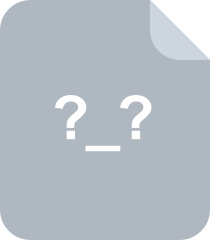
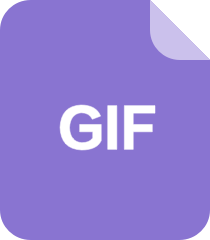
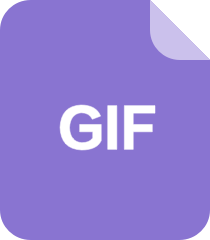
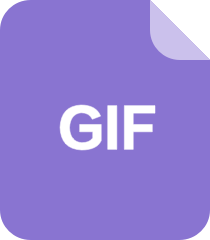
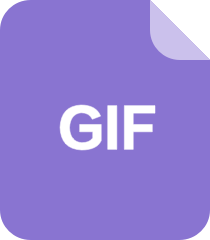
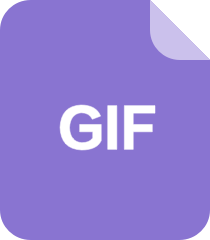
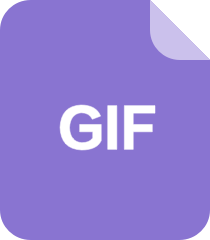
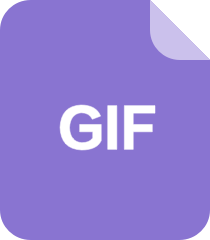
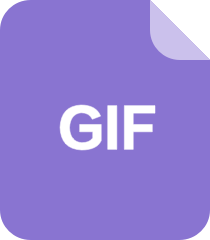
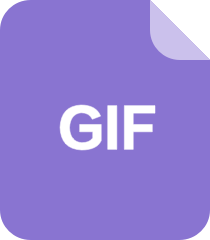
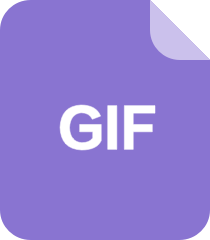
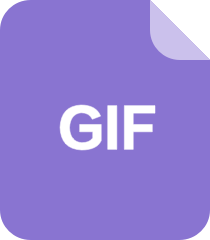
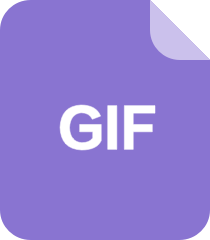
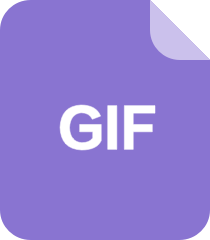
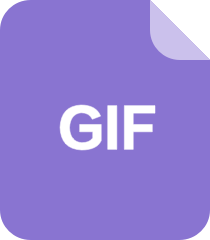
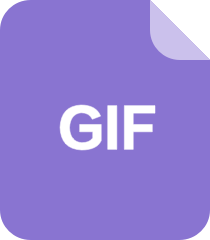
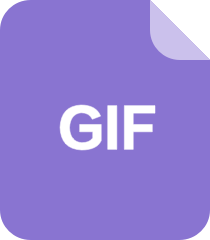
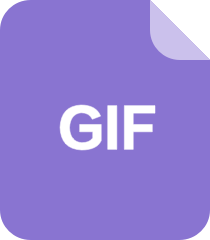
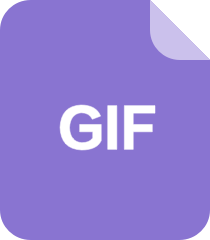
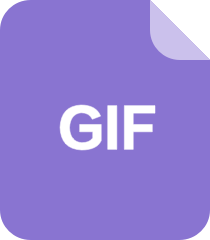
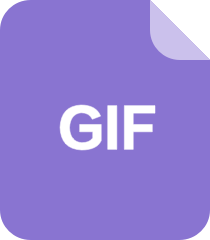
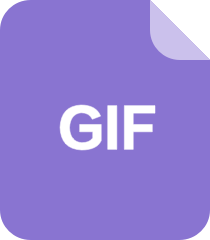
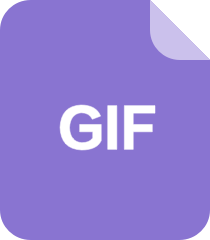
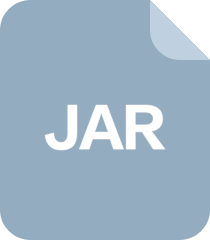
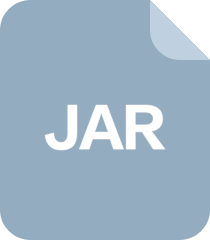
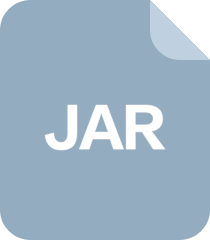
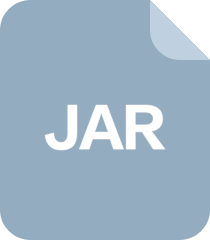
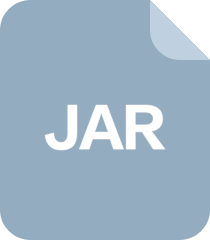
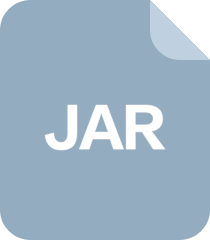
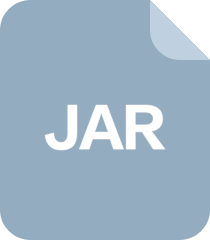
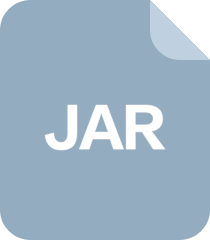
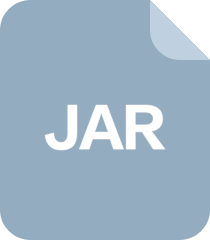
共 336 条
- 1
- 2
- 3
- 4
资源评论
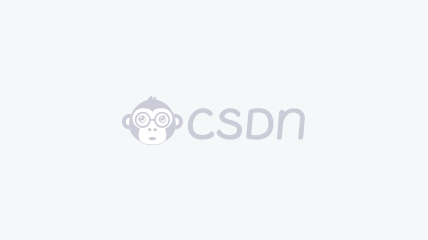

python资深爱好者
- 粉丝: 1720
- 资源: 2784
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

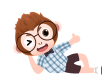
最新资源
- best-of-bits-Matlab工具箱使用资源
- TrainSimulationPlat-simulink仿真资源
- 实例分割-使用opencv+yolact实现实例分割算法-C++和python两种实现-附项目源码.zip
- numpy-numpy
- typora-typora
- riscv-pke-操作系统实验
- 迅睿CMS框架-mysql安装教程
- 实例分割-将SAM和YOLOv8结合实现开集实例分割+目标检测算法-附项目源码+流程教程-优质项目实战.zip
- 实例分割-基于TensorRT加速+YOLOv9的目标检测+实例分割算法实现-付项目源码+流程教程-优质项目实战.zip
- numpy作业-numpy
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


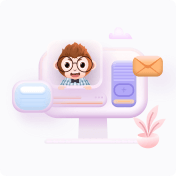
安全验证
文档复制为VIP权益,开通VIP直接复制
