package com.controller;
import java.text.SimpleDateFormat;
import com.alibaba.fastjson.JSONObject;
import java.util.*;
import com.entity.*;
import com.service.*;
import org.springframework.beans.BeanUtils;
import javax.servlet.http.HttpServletRequest;
import org.springframework.web.context.ContextLoader;
import javax.servlet.ServletContext;
import com.utils.StringUtil;
import java.lang.reflect.InvocationTargetException;
import org.apache.commons.lang3.StringUtils;
import com.annotation.IgnoreAuth;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.baomidou.mybatisplus.mapper.Wrapper;
import com.entity.view.GoodsOrderView;
import com.utils.PageUtils;
import com.utils.R;
/**
* 采购调度表
* 后端接口
* @author
* @email
* @date 2021-04-09
*/
@RestController
@Controller
@RequestMapping("/goodsOrder")
public class GoodsOrderController {
private static final Logger logger = LoggerFactory.getLogger(GoodsOrderController.class);
@Autowired
private GoodsOrderService goodsOrderService;
@Autowired
private TokenService tokenService;
@Autowired
private DictionaryService dictionaryService;
//级联表service
@Autowired
private FendianService fendianService;
@Autowired
private GongyingshangService gongyingshangService;
// 列表详情的表级联service
@Autowired
private GoodsOrderListService goodsOrderListService;
@Autowired
private YonghuService yonghuService;
@Autowired
private GoodsService goodsService;
@Autowired
private FendianGoodsService fendianGoodsService;
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params, HttpServletRequest request){
logger.debug("page方法:,,Controller:{},,params:{}",this.getClass().getName(),JSONObject.toJSONString(params));
String role = String.valueOf(request.getSession().getAttribute("role"));
if(StringUtil.isNotEmpty(role) && "用户".equals(role)){
params.put("yonghuId",request.getSession().getAttribute("userId"));
}
params.put("orderBy","id");
PageUtils page = goodsOrderService.queryPage(params);
//字典表数据转换
List<GoodsOrderView> list =(List<GoodsOrderView>)page.getList();
for(GoodsOrderView c:list){
//修改对应字典表字段
dictionaryService.dictionaryConvert(c);
}
return R.ok().put("data", page);
}
/**
* 后端详情
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") Long id){
logger.debug("info方法:,,Controller:{},,id:{}",this.getClass().getName(),id);
GoodsOrderEntity goodsOrder = goodsOrderService.selectById(id);
if(goodsOrder !=null){
//entity转view
GoodsOrderView view = new GoodsOrderView();
BeanUtils.copyProperties( goodsOrder , view );//把实体数据重构到view中
//级联表
FendianEntity fendian = fendianService.selectById(goodsOrder.getFendianId());
if(fendian != null){
BeanUtils.copyProperties( fendian , view ,new String[]{ "id", "createDate"});//把级联的数据添加到view中,并排除id和创建时间字段
view.setFendianId(fendian.getId());
}
//级联表
GongyingshangEntity gongyingshang = gongyingshangService.selectById(goodsOrder.getGongyingshangId());
if(gongyingshang != null){
BeanUtils.copyProperties( gongyingshang , view ,new String[]{ "id", "createDate"});//把级联的数据添加到view中,并排除id和创建时间字段
view.setGongyingshangId(gongyingshang.getId());
}
//修改对应字典表字段
dictionaryService.dictionaryConvert(view);
return R.ok().put("data", view);
}else {
return R.error(511,"查不到数据");
}
}
/**
* 后端保存
*/
@RequestMapping("/save")
public R save(@RequestBody GoodsOrderEntity goodsOrder, HttpServletRequest request){
logger.debug("save方法:,,Controller:{},,goodsOrder:{}",this.getClass().getName(),goodsOrder.toString());
goodsOrder.setInsertTime(new Date());
goodsOrder.setCreateTime(new Date());
goodsOrderService.insert(goodsOrder);
return R.ok();
}
/**
* 后端修改
*/
@RequestMapping("/update")
public R update(@RequestBody GoodsOrderEntity goodsOrder, HttpServletRequest request){
logger.debug("update方法:,,Controller:{},,goodsOrder:{}",this.getClass().getName(),goodsOrder.toString());
goodsOrderService.updateById(goodsOrder);//根据id更新
return R.ok();
}
/**
* 调度
*/
@RequestMapping("/outGoodsOrderList")
public R outGoodsOrderList(@RequestBody Map<String, Object> params,HttpServletRequest request){
logger.debug("outGoodsOrderList方法:,,Controller:{},,params:{}",this.getClass().getName(),JSONObject.toJSONString(params));
Date date = new Date();//当前时间
Integer userId = (Integer) request.getSession().getAttribute("userId");//当前登录人的id
String tableName = (String) request.getSession().getAttribute("tableName");//当前登录人的表名
YonghuEntity yonghuEntity = yonghuService.selectById(userId);//当前登录人的信息
//当前表的表级联查询
// String gongyingshangId = (String) params.get("gongyingshangId"); //供应商
String fendianId = (String) params.get("fendianId"); //分店
if(fendianId == "" || fendianId== null || fendianId == "null"){
return R.error("请选择分店");
}
String goodsOrderName = (String) params.get("goodsOrderName"); // 订单名
HashMap<String, Integer> map = (HashMap<String, Integer>) params.get("map");// id 和 数量
if(map == null || map.size() ==0){
return R.error("添加不能为空");
}else{
Set<String> ids = map.keySet(); // 获取添加数据的ids
List<GoodsEntity> goodsList = goodsService.selectList(new EntityWrapper<GoodsEntity>().in("id", ids));
if(goodsList == null || goodsList.size() ==0 || map.size() != goodsList.size() ){
return R.error("查询物资为空 或者 采购的物资和数据库中不一致,请去列表中查找确认");
}else{
for(GoodsEntity goods : goodsList){
if(goods.getGoodsNumber()-map.get(String.valueOf(goods.getId())) <0){
return R.error(goods.getGoodsName()+"的出库数量小于大于库存数量,请重新确认出库数量");
}
goods.setGoodsNumber(goods.getGoodsNumber()-map.get(String.valueOf(goods.getId())));
}
}
// 查询当前分店的所有库存
List<FendianGoodsEntity> fendianGoodsEntities = fendianGoodsService.selectList(new EntityWrapper<FendianGoodsEntity>().eq("fendian_id",fendianId));
HashMap<Integer, FendianGoodsEntity> fendianGoodsMap = new HashMap<>();
for(FendianGoodsEntity fendianGoods :fendianGoodsEntities){
fendianGoodsMap.put(fendianGoods.getGoodsOnly(),fendianGoods);//以总店id作为key,以对象作为值,用于下面判断
}
List<FendianGoodsEntity> fendianGoodsEntityList = new ArrayList<>();//要更新的分店库存对象
for(GoodsEntity goods:goodsList){
FendianGoodsEntity fendianGoodsEntity = fendianGoodsMap.get(goods.getId());
if(fendianGoodsEntity != null){//�
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
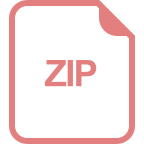
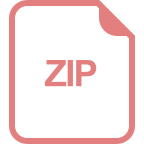
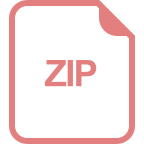
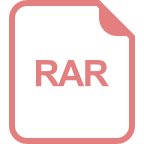
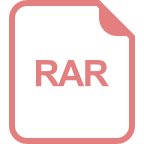
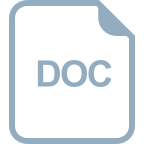
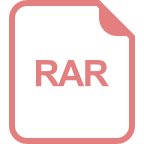
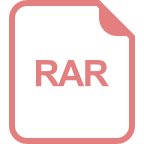
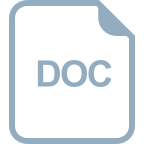
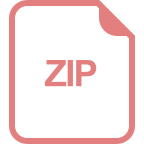
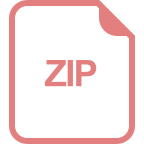
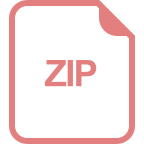
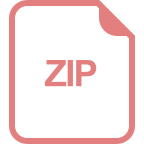
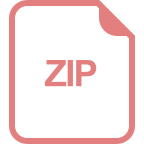
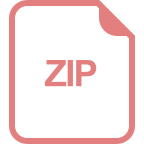
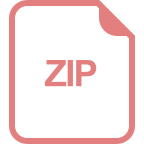
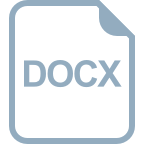
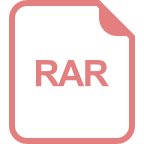
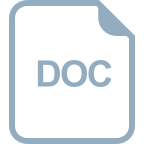
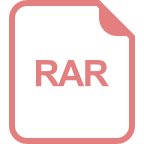
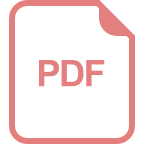
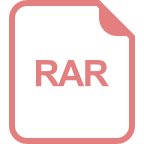
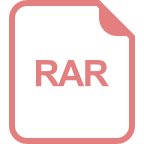
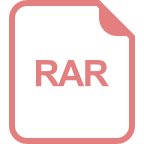
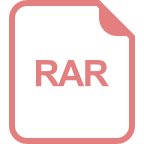
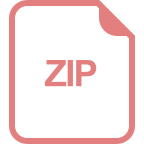
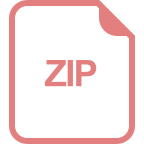
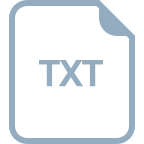
收起资源包目录

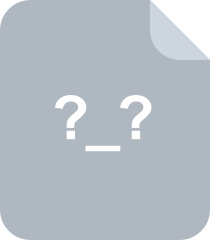
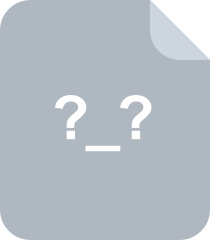
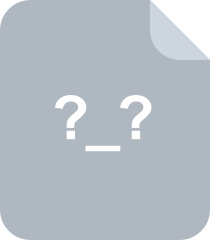
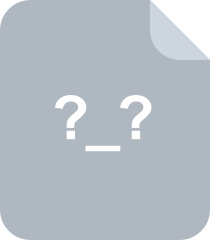
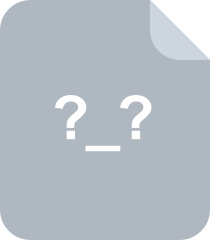
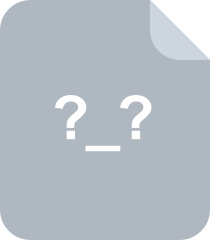
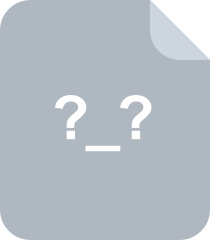
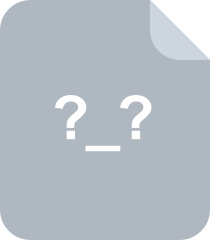
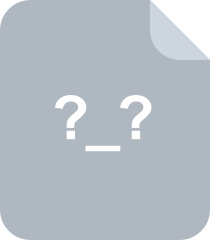
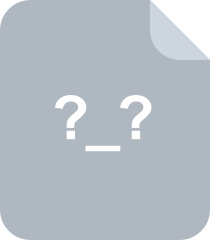
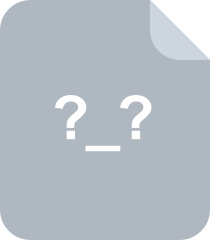
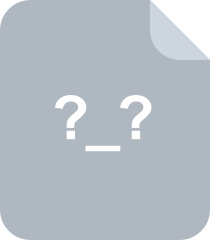
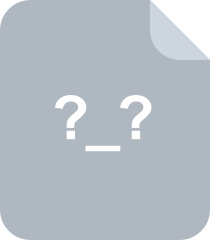
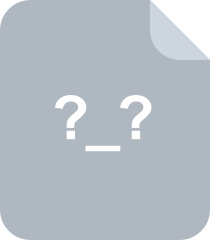
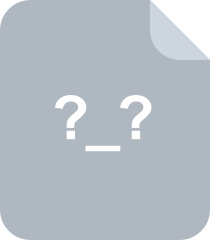
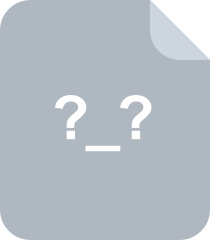
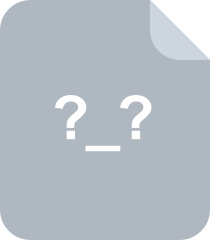
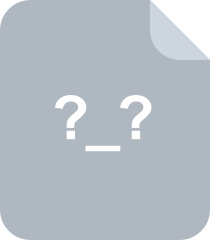
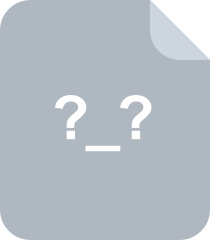
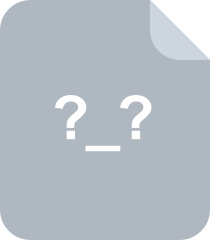
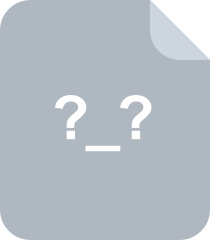
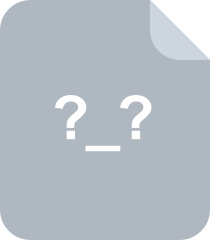
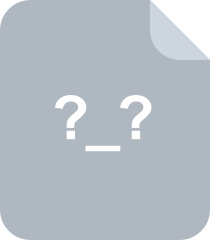
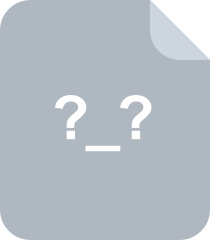
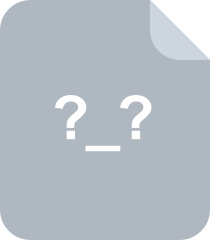
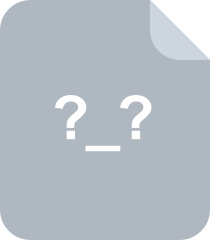
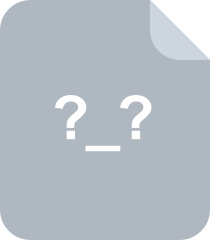
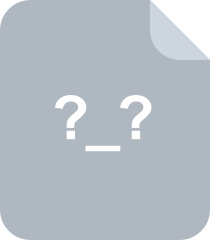
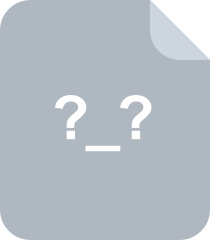
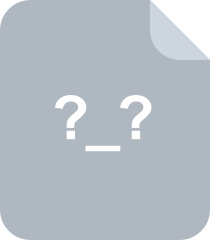
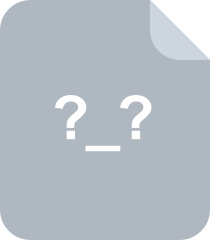
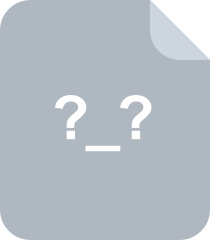
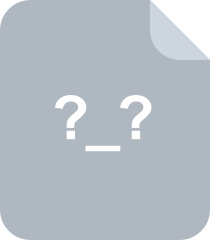
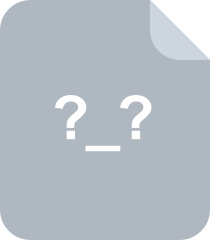
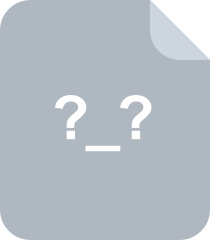
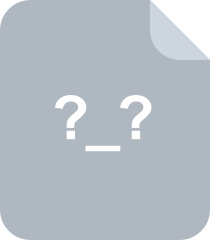
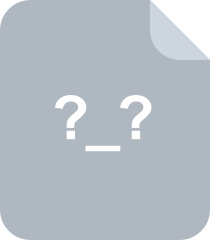
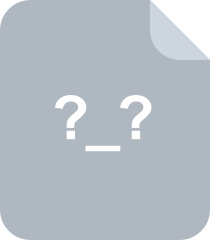
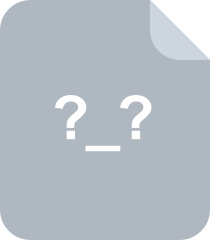
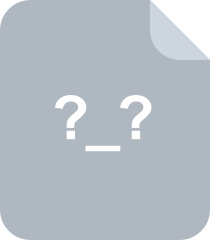
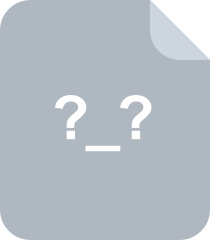
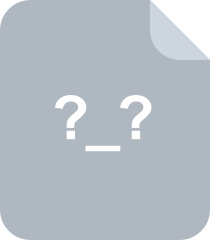
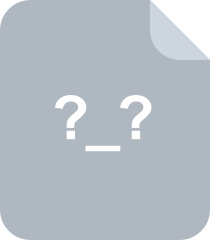
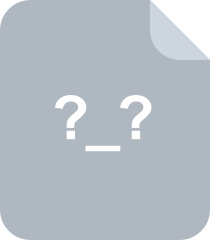
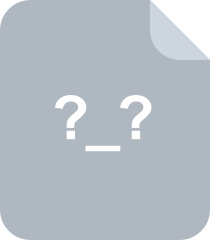
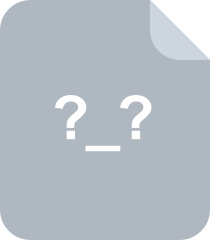
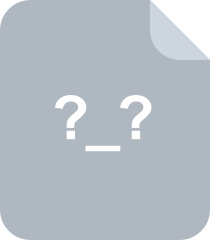
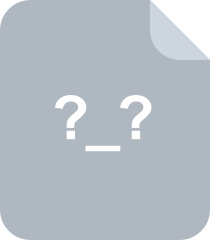
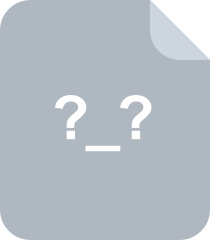
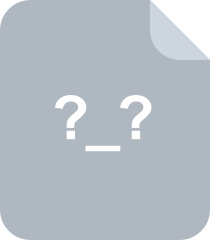
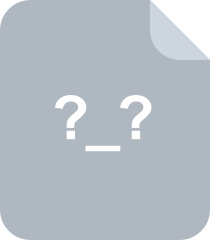
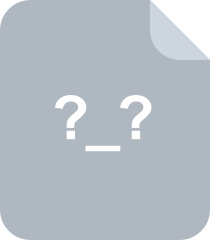
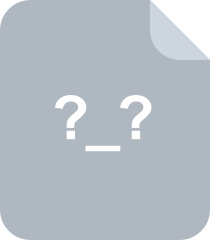
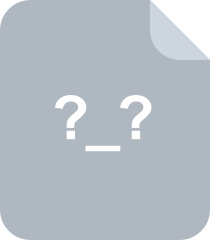
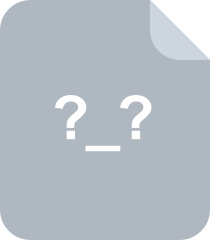
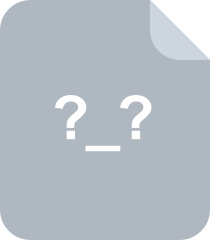
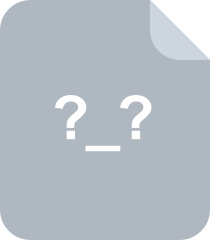
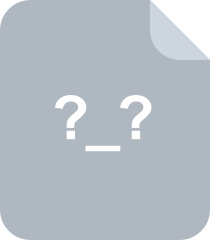
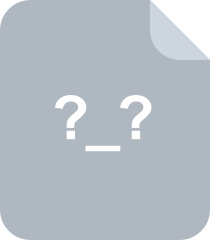
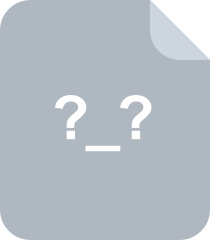
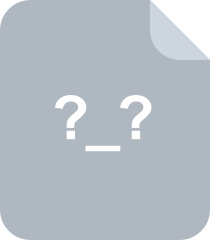
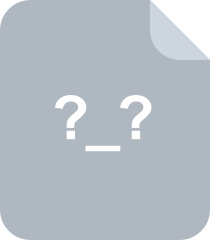
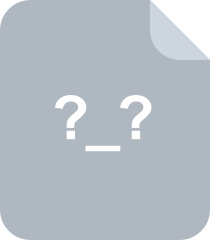
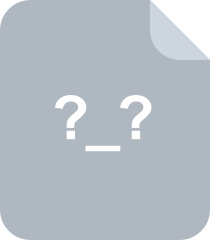
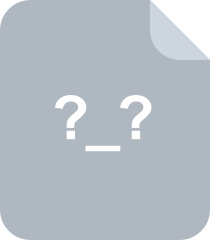
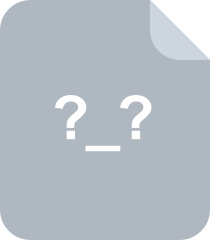
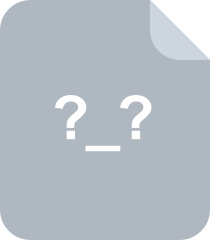
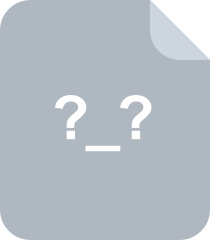
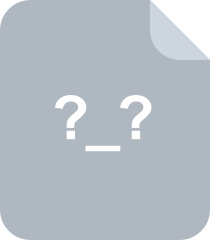
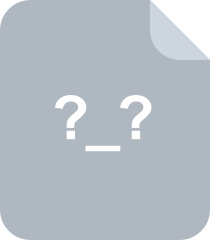
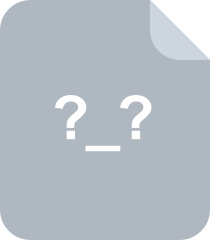
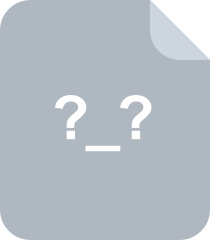
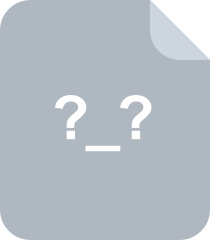
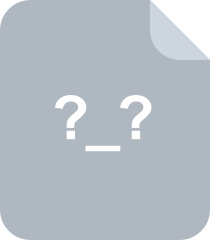
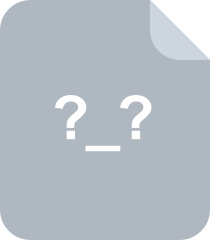
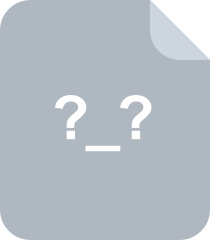
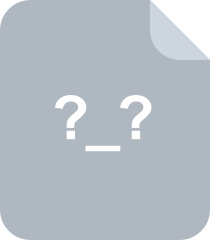
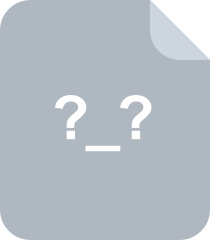
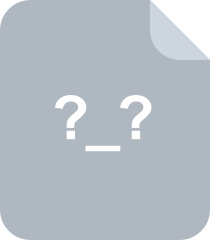
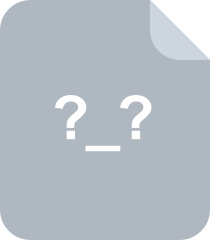
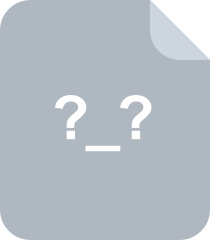
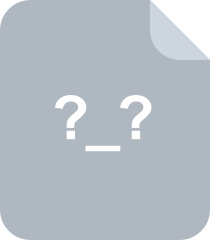
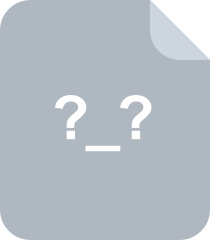
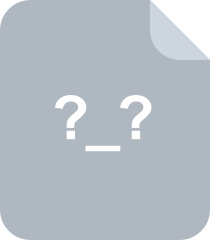
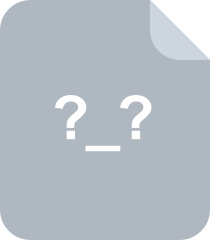
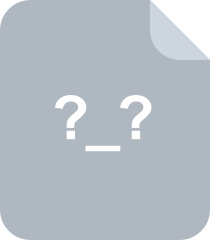
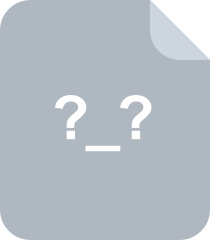
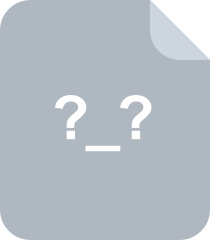
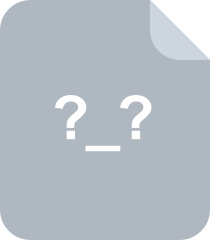
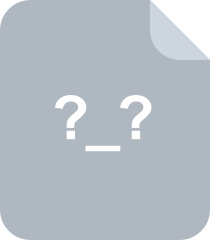
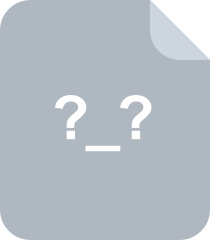
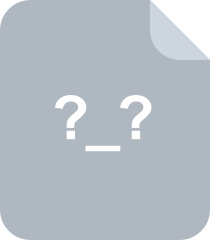
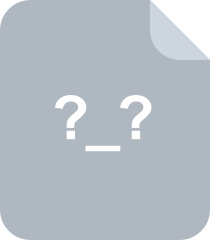
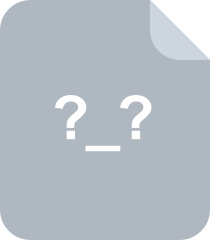
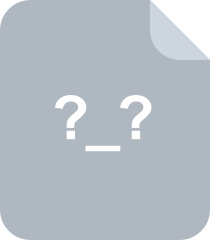
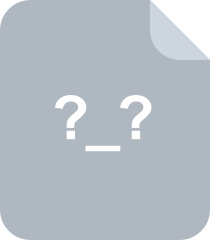
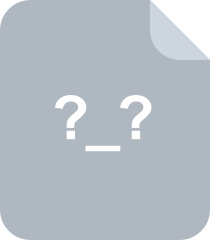
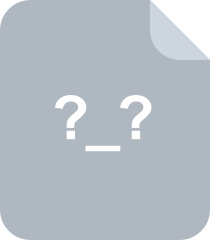
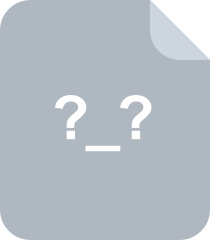
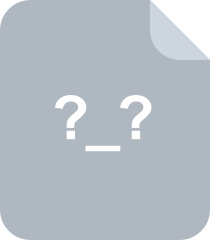
共 1047 条
- 1
- 2
- 3
- 4
- 5
- 6
- 11
资源评论
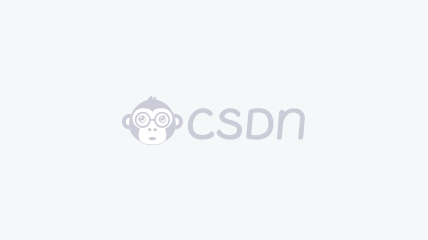

普通网友
- 粉丝: 1126
- 资源: 5294
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

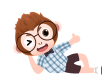
最新资源
- ouc2024秋攻防先导作业
- ouc2024秋攻防先导作业
- 算数优化算法AOA对BP的权值和阈值做优化,建立多个特征变量输入,单个因变量输出的拟合预测建模 程序内注释详细,可学习性强 直接替数据就可以用,可以直接导入excel数据 程序语言为matlab
- 精确符合规范反应谱的人工合成地震波
- LINUX最新版本算法库5.0.15(1).zip
- Matlab基于量子遗传算法的函数寻优方法 量子遗传算法QGA是量子计算与遗传算法相结合的产物,是一种新发展起来的概率进化算法 代码可正常运行
- 驾校预约学习系统 源码+数据库+论文(JAVA+SpringBoot+Vue.JS+MySQL).zip
- 基于ssm+mysql图书仓储管理系统源码数据库.docx
- springboot3+vue3
- CREO7装配与产品设计-钟日铭-配套操作文件
- 基于SpringBoot+Vue.JS开发的校园志愿者管理系统 JAVA毕业设计 源码+数据库+论文(有项目截图)+启动教程
- Linux操作系统课程设计实验报告-涵盖多线程同步、内核模块编程、进程树打印、系统调用添加及块设备驱动实现
- 素材网站解析源码支持21个网站解析可搭建运营
- 285个地级市灯光数据和雾霾数据.zip
- 全桥谐振和变压器计算 看截图,知识文档,含有LLC谐振半桥计算,全桥LLC谐振和变压器的计算,描述详细使用,表格都有引用公式依据,便于理解
- vlan dnsp 111
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


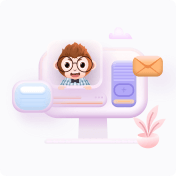
安全验证
文档复制为VIP权益,开通VIP直接复制
