# Calendar
### Intro
Calendar component for selecting dates or date ranges.
### Install
Register component globally via `app.use`, refer to [Component Registration](#/en-US/advanced-usage#zu-jian-zhu-ce) for more registration ways.
```js
import { createApp } from 'vue';
import { Calendar } from 'vant';
const app = createApp();
app.use(Calendar);
```
## Usage
### Select Switch Mode
By default, all months will be displayed without showing the switch button. When there are too many months, it may affect the page's interactivity performance. You can display the year and month switching buttons by setting the `switch-mode` prop.
```html
<van-calendar v-model:show="show" switch-mode="year-month" />
```
### Select Single Date
The `confirm` event will be emitted after the date selection is completed.
```html
<van-cell title="Select Single Date" :value="date" @click="show = true" />
<van-calendar v-model:show="show" @confirm="onConfirm" />
```
```js
import { ref } from 'vue';
export default {
setup() {
const date = ref('');
const show = ref(false);
const formatDate = (date) => `${date.getMonth() + 1}/${date.getDate()}`;
const onConfirm = (value) => {
show.value = false;
date.value = formatDate(value);
};
return {
date,
show,
onConfirm,
};
},
};
```
### Select Multiple Date
```html
<van-cell title="Select Multiple Date" :value="text" @click="show = true" />
<van-calendar v-model:show="show" type="multiple" @confirm="onConfirm" />
```
```js
import { ref } from 'vue';
export default {
setup() {
const text = ref('');
const show = ref(false);
const onConfirm = (dates) => {
show.value = false;
text.value = `选择了 ${dates.length} 个日期`;
};
return {
text,
show,
onConfirm,
};
},
};
```
### Select Date Range
You can select a date range after setting `type` to`range`. In range mode, the date returned by the `confirm` event is an array, the first item in the array is the start time and the second item is the end time.
```html
<van-cell title="Select Date Range" :value="date" @click="show = true" />
<van-calendar v-model:show="show" type="range" @confirm="onConfirm" />
```
```js
import { ref } from 'vue';
export default {
setup() {
const date = ref('');
const show = ref(false);
const formatDate = (date) => `${date.getMonth() + 1}/${date.getDate()}`;
const onConfirm = (values) => {
const [start, end] = values;
show.value = false;
date.value = `${formatDate(start)} - ${formatDate(end)}`;
};
return {
date,
show,
onConfirm,
};
},
};
```
### Quick Select
Set `show-confirm` to `false` to hide the confirm button. In this case, the `confirm` event will be emitted immediately after the selection is completed.
```html
<van-calendar v-model:show="show" :show-confirm="false" />
```
### Custom Color
Use `color` prop to custom calendar color.
```html
<van-calendar v-model:show="show" color="#ee0a24" />
```
### Custom Date Range
Use `min-date` and `max-date` to custom date range.
```html
<van-calendar v-model:show="show" :min-date="minDate" :max-date="maxDate" />
```
```js
import { ref } from 'vue';
export default {
setup() {
const show = ref(false);
return {
show,
minDate: new Date(2010, 0, 1),
maxDate: new Date(2010, 0, 31),
};
},
};
```
### Custom Confirm Text
Use `confirm-text` and `confirm-disabled-text` to custom confirm text.
```html
<van-calendar
v-model:show="show"
type="range"
confirm-text="OK"
confirm-disabled-text="Select End Time"
/>
```
### Custom Day Text
Use `formatter` to custom day text.
```html
<van-calendar v-model:show="show" type="range" :formatter="formatter" />
```
```js
export default {
setup() {
const formatter = (day) => {
const month = day.date.getMonth() + 1;
const date = day.date.getDate();
if (month === 5) {
if (date === 1) {
day.topInfo = 'Labor Day';
} else if (date === 4) {
day.topInfo = 'Youth Day';
} else if (date === 11) {
day.text = 'Today';
}
}
if (day.type === 'start') {
day.bottomInfo = 'In';
} else if (day.type === 'end') {
day.bottomInfo = 'Out';
}
return day;
};
return {
formatter,
};
},
};
```
### Custom Position
Use `position` to custom popup position, can be set to `top`、`left`、`right`.
```html
<van-calendar v-model:show="show" :round="false" position="right" />
```
### Max Range
When selecting a date range, you can use the `max-range` prop to specify the maximum number of selectable days.
```html
<van-calendar type="range" :max-range="3" :style="{ height: '500px' }" />
```
### Custom First Day Of Week
Use `first-day-of-week` to custom the start day of week
```html
<van-calendar first-day-of-week="1" />
```
### Tiled display
Set `poppable` to `false`, the calendar will be displayed directly on the page instead of appearing as a popup
```html
<van-calendar
title="Calendar"
:poppable="false"
:show-confirm="false"
:style="{ height: '500px' }"
/>
```
## API
### Props
| Attribute | Description | Type | Default |
| --- | --- | --- | --- |
| type | Type, can be set to `range` `multiple` | _string_ | `single` |
| switch-mode `v4.9.0` | Switch mode:<br>`none` Display all months in a tiled format without switch buttons <br>`month` Support switching by month, displaying buttons for previous month/next month <br>`year-month` Support switching by year, as well as by month, displaying buttons for previous year/next year and previous month/next month | _string_ | `none` |
| title | Title of calendar | _string_ | `Calendar` |
| color | Color for the bottom button and selected date | _string_ | `#1989fa` |
| min-date | Min date | _Date_ | When `switch-mode` is set to `none`, the default value is the today |
| max-date | Max date | _Date_ | When `switch-mode` is set to `none`, the default value is six months after the today |
| default-date | Default selected date | _Date \| Date[] \| null_ | Today |
| row-height | Row height | _number \| string_ | `64` |
| formatter | Day formatter | _(day: Day) => Day_ | - |
| poppable | Whether to show the calendar inside a popup | _boolean_ | `true` |
| lazy-render | Whether to enable lazy render | _boolean_ | `true` |
| show-mark | Whether to show background month mark | _boolean_ | `true` |
| show-title | Whether to show title | _boolean_ | `true` |
| show-subtitle | Whether to show subtitle | _boolean_ | `true` |
| show-confirm | Whether to show confirm button | _boolean_ | `true` |
| readonly | Whether to be readonly | _boolean_ | `false` |
| confirm-text | Confirm button text | _string_ | `Confirm` |
| confirm-disabled-text | Confirm button text when disabled | _string_ | `Confirm` |
| first-day-of-week | Set the start day of week | _0-6_ | `0` |
### Calendar Poppable Props
Following props are supported when the poppable is true
| Attribute | Description | Type | Default |
| --- | --- | --- | --- |
| v-model:show | Whether to show calendar | _boolean_ | `false` |
| position | Popup position, can be set to `top` `right` `left` | _string_ | `bottom` |
| round | Whether to show round corner | _boolean_ | `true` |
| close-on-popstate | Whether to close when popstate | _boolean_ | `true` |
| close-on-click-overlay | Whether to close when overlay is clicked | _boolean_ | `true` |
| safe-area-inset-top | Whether to enable top safe area adaptation | _boolean_ | `false` |
| safe-area-inset-bottom | Whether to enable bottom safe area adaptation | _boolean_ | `true` |
| teleport | Specifies a target element where Calendar will be mounted | _string \| Element_ | - |
### Calendar Range Props
Following props are supported when the type is range
| Attribute | Description | Type | Default |
| --- | --- | --- | --- |
| max-range | Number of selectable days | _numb
没有合适的资源?快使用搜索试试~ 我知道了~
教你如何用Github找开源项目(保姆级教程)
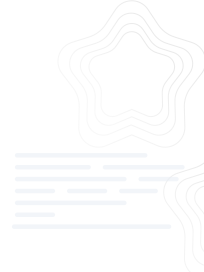
共1567个文件
ts:564个
md:245个
snap:235个

需积分: 1 3 下载量 140 浏览量
2024-09-13
09:22:12
上传
评论
收藏 10.09MB ZIP 举报
温馨提示
教你如何用Github找开源项目(保姆级教程)教你如何用Github找开源项目(保姆级教程)教你如何用Github找开源项目(保姆级教程)教你如何用Github找开源项目(保姆级教程)教你如何用Github找开源项目(保姆级教程)教你如何用Github找开源项目(保姆级教程)教你如何用Github找开源项目(保姆级教程)教你如何用Github找开源项目(保姆级教程)教你如何用Github找开源项目(保姆级教程)教你如何用Github找开源项目(保姆级教程)教你如何用Github找开源项目(保姆级教程)教你如何用Github找开源项目(保姆级教程)教你如何用Github找开源项目(保姆级教程)教你如何用Github找开源项目(保姆级教程)教你如何用Github找开源项目(保姆级教程)教你如何用Github找开源项目(保姆级教程)教你如何用Github找开源项目(保姆级教程)教你如何用Github找开源项目(保姆级教程)教你如何用Github找开源项目(保姆级教程)教你如何用Github找开源项目(保姆级教程)教你如何用Github找开源项目(保姆级教程)教你如何用Github找开源项目(
资源推荐
资源详情
资源评论
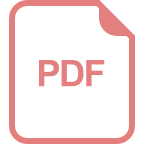
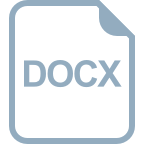
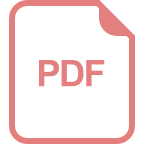
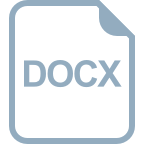
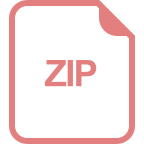
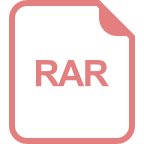
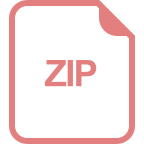
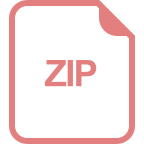
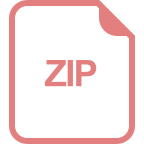
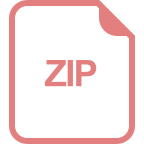
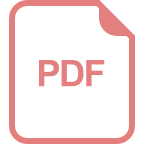
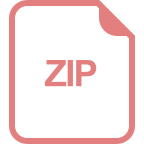
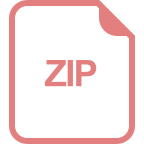
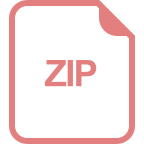
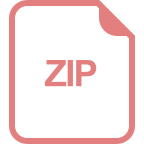
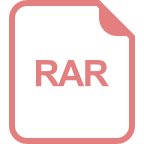
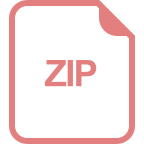
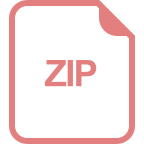
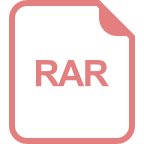
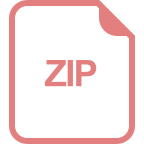
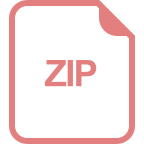
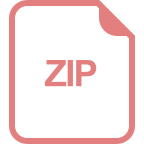
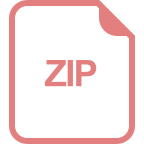
收起资源包目录

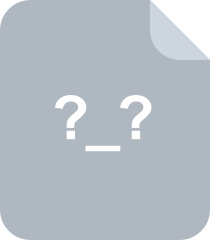
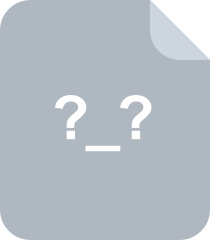
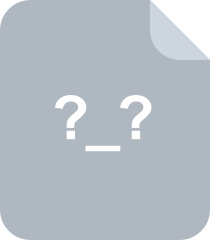
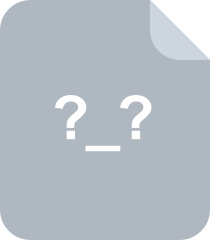
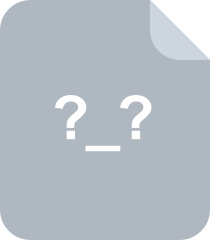
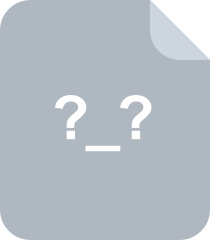
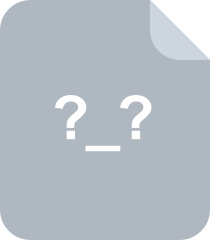
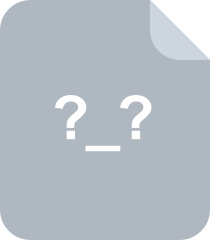
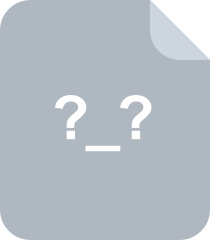
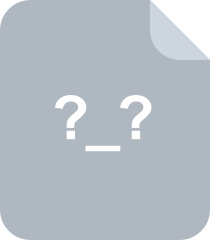
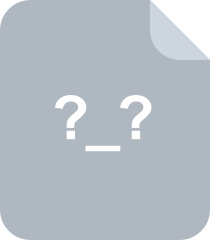
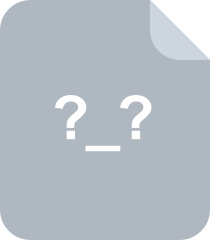
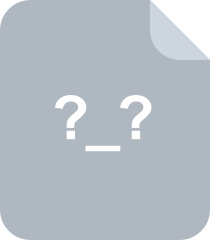
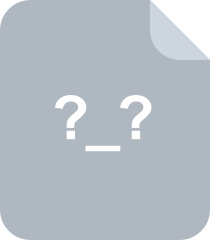
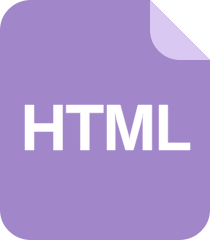
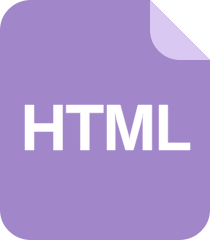
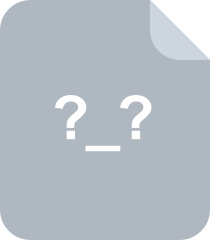
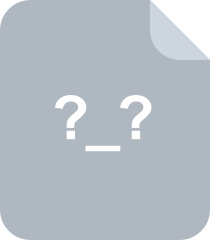
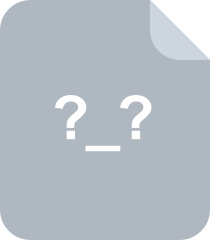
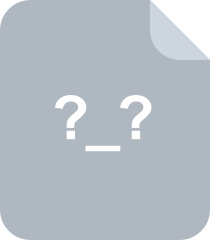
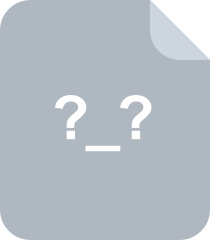
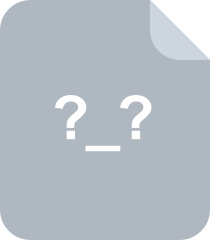
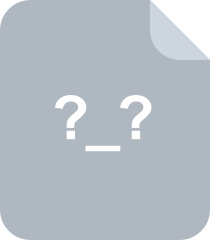
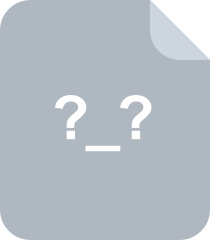
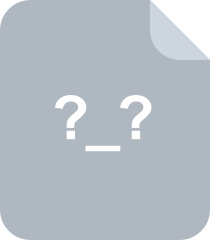
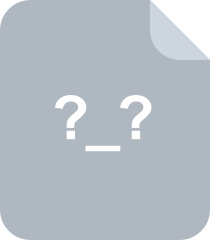
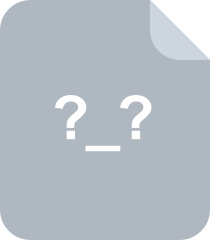
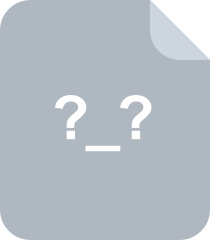
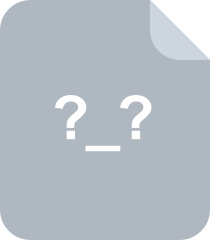
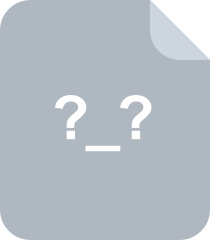
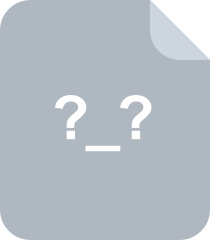
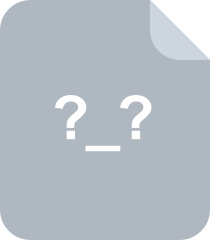
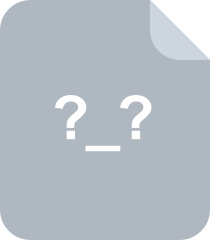
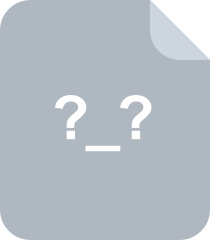
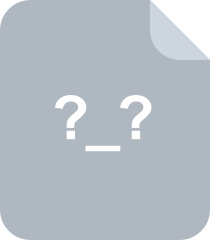
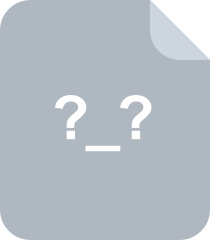
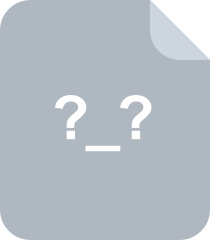
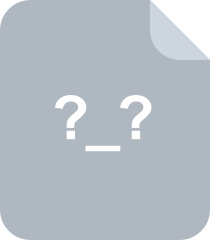
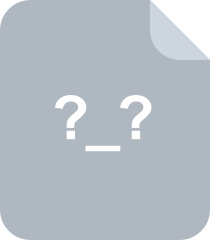
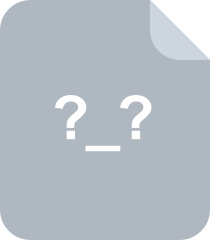
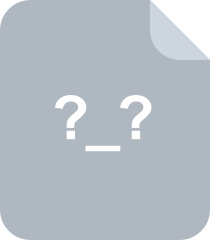
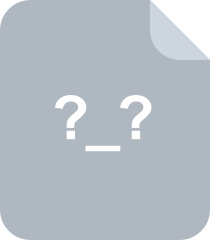
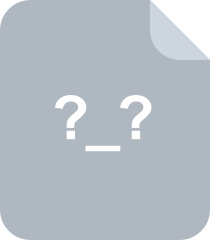
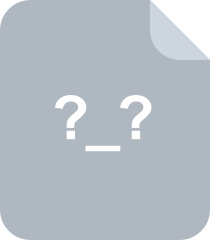
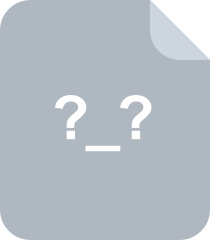
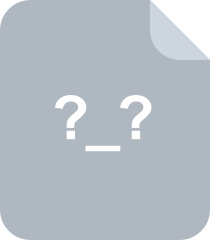
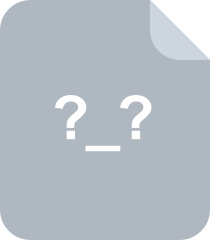
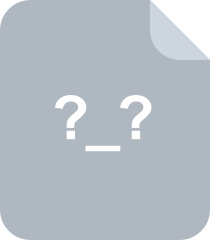
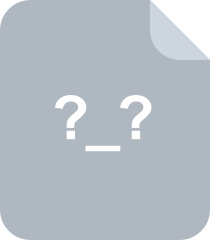
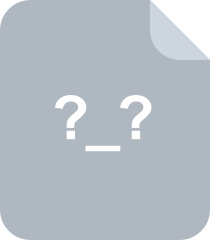
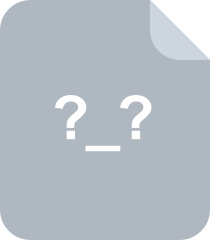
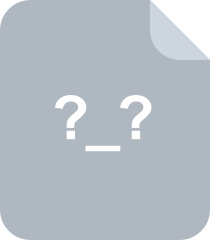
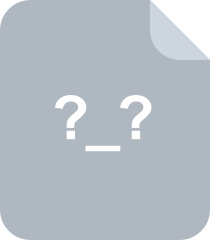
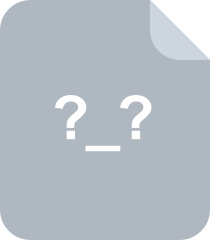
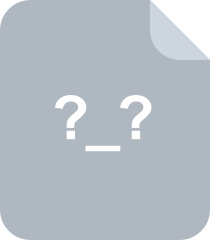
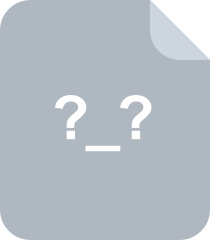
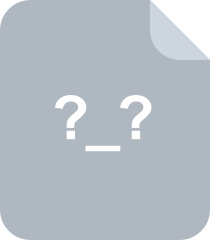
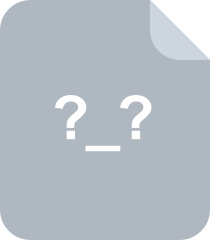
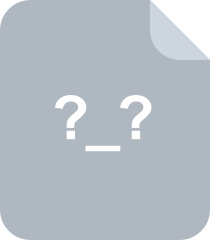
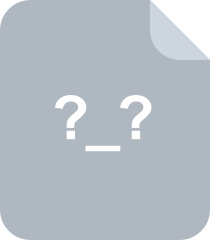
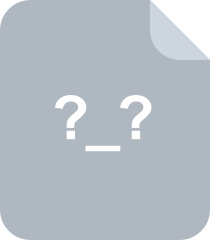
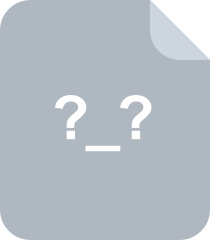
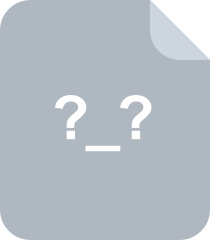
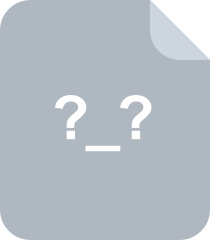
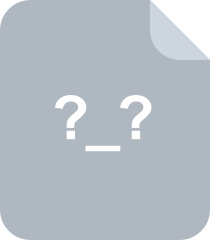
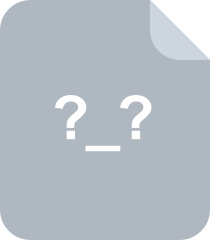
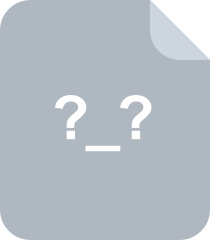
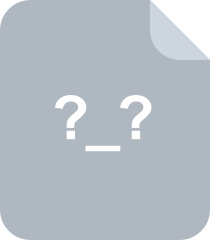
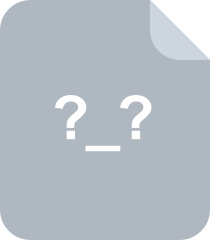
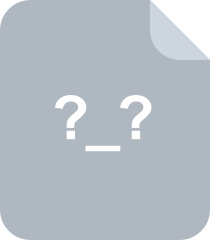
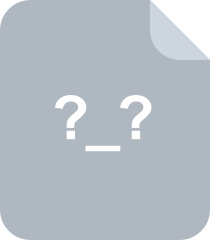
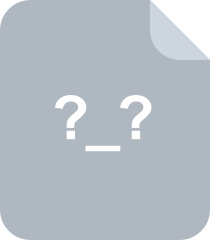
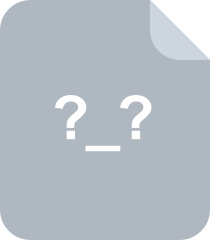
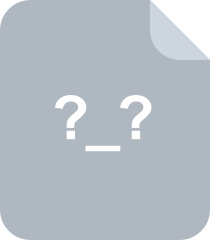
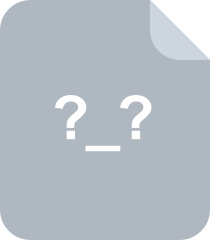
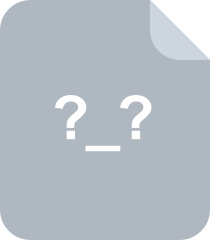
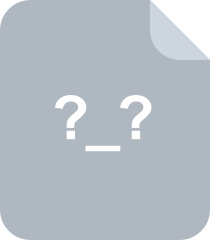
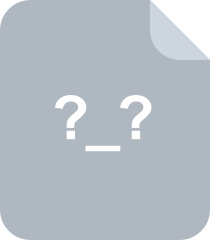
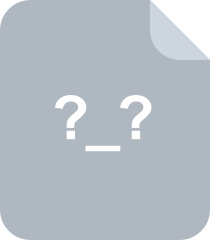
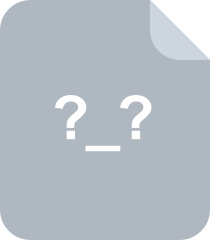
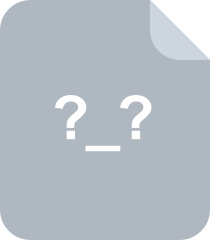
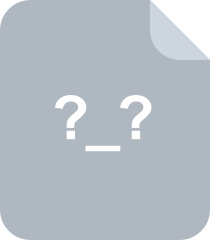
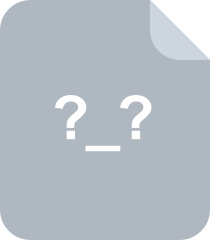
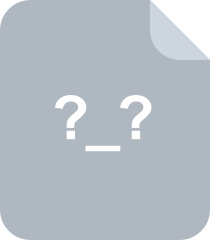
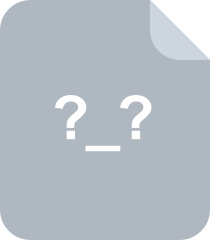
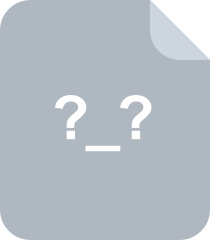
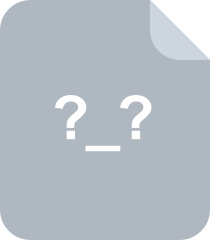
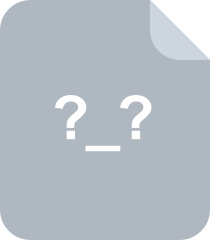
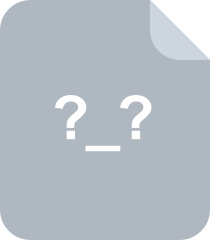
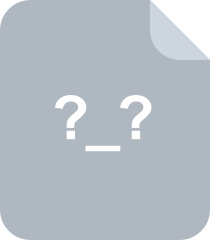
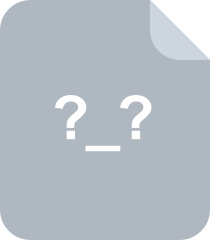
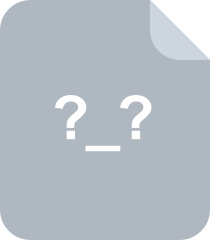
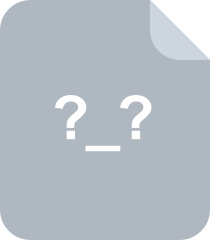
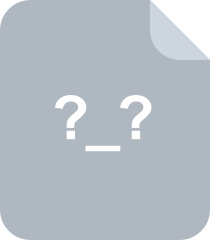
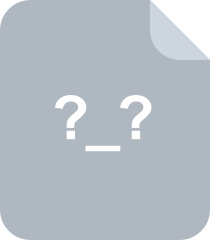
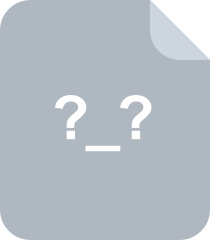
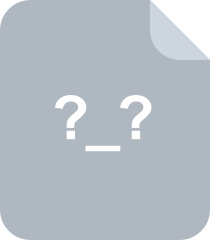
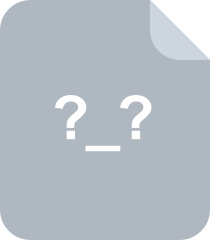
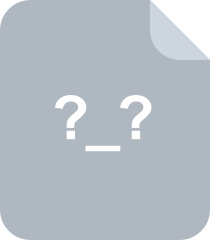
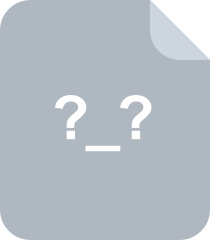
共 1567 条
- 1
- 2
- 3
- 4
- 5
- 6
- 16
资源评论
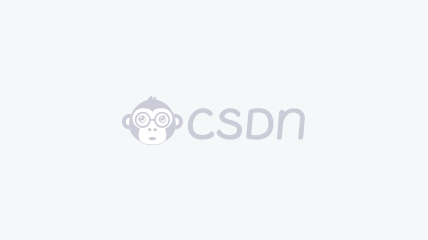

脚步的影子
- 粉丝: 2130
- 资源: 186
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

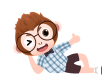
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


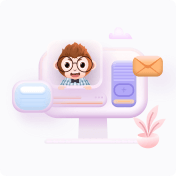
安全验证
文档复制为VIP权益,开通VIP直接复制
