libpng-manual.txt - A description on how to use and modify libpng
libpng version 1.6.21 - January 15, 2016
Updated and distributed by Glenn Randers-Pehrson
<glennrp at users.sourceforge.net>
Copyright (c) 1998-2016 Glenn Randers-Pehrson
This document is released under the libpng license.
For conditions of distribution and use, see the disclaimer
and license in png.h
Based on:
libpng versions 0.97, January 1998, through 1.6.21 - January 15, 2016
Updated and distributed by Glenn Randers-Pehrson
Copyright (c) 1998-2016 Glenn Randers-Pehrson
libpng 1.0 beta 6 - version 0.96 - May 28, 1997
Updated and distributed by Andreas Dilger
Copyright (c) 1996, 1997 Andreas Dilger
libpng 1.0 beta 2 - version 0.88 - January 26, 1996
For conditions of distribution and use, see copyright
notice in png.h. Copyright (c) 1995, 1996 Guy Eric
Schalnat, Group 42, Inc.
Updated/rewritten per request in the libpng FAQ
Copyright (c) 1995, 1996 Frank J. T. Wojcik
December 18, 1995 & January 20, 1996
TABLE OF CONTENTS
I. Introduction
II. Structures
III. Reading
IV. Writing
V. Simplified API
VI. Modifying/Customizing libpng
VII. MNG support
VIII. Changes to Libpng from version 0.88
IX. Changes to Libpng from version 1.0.x to 1.2.x
X. Changes to Libpng from version 1.0.x/1.2.x to 1.4.x
XI. Changes to Libpng from version 1.4.x to 1.5.x
XII. Changes to Libpng from version 1.5.x to 1.6.x
XIII. Detecting libpng
XIV. Source code repository
XV. Coding style
XVI. Y2K Compliance in libpng
I. Introduction
This file describes how to use and modify the PNG reference library
(known as libpng) for your own use. In addition to this
file, example.c is a good starting point for using the library, as
it is heavily commented and should include everything most people
will need. We assume that libpng is already installed; see the
INSTALL file for instructions on how to configure and install libpng.
For examples of libpng usage, see the files "example.c", "pngtest.c",
and the files in the "contrib" directory, all of which are included in
the libpng distribution.
Libpng was written as a companion to the PNG specification, as a way
of reducing the amount of time and effort it takes to support the PNG
file format in application programs.
The PNG specification (second edition), November 2003, is available as
a W3C Recommendation and as an ISO Standard (ISO/IEC 15948:2004 (E)) at
<http://www.w3.org/TR/2003/REC-PNG-20031110/
The W3C and ISO documents have identical technical content.
The PNG-1.2 specification is available at
<http://png-mng.sourceforge.net/pub/png/spec/1.2/>.
It is technically equivalent
to the PNG specification (second edition) but has some additional material.
The PNG-1.0 specification is available as RFC 2083
<http://png-mng.sourceforge.net/pub/png/spec/1.0/> and as a
W3C Recommendation <http://www.w3.org/TR/REC-png-961001>.
Some additional chunks are described in the special-purpose public chunks
documents at <http://www.libpng.org/pub/png/spec/register/>
Other information
about PNG, and the latest version of libpng, can be found at the PNG home
page, <http://www.libpng.org/pub/png/>.
Most users will not have to modify the library significantly; advanced
users may want to modify it more. All attempts were made to make it as
complete as possible, while keeping the code easy to understand.
Currently, this library only supports C. Support for other languages
is being considered.
Libpng has been designed to handle multiple sessions at one time,
to be easily modifiable, to be portable to the vast majority of
machines (ANSI, K&R, 16-, 32-, and 64-bit) available, and to be easy
to use. The ultimate goal of libpng is to promote the acceptance of
the PNG file format in whatever way possible. While there is still
work to be done (see the TODO file), libpng should cover the
majority of the needs of its users.
Libpng uses zlib for its compression and decompression of PNG files.
Further information about zlib, and the latest version of zlib, can
be found at the zlib home page, <http://zlib.net/>.
The zlib compression utility is a general purpose utility that is
useful for more than PNG files, and can be used without libpng.
See the documentation delivered with zlib for more details.
You can usually find the source files for the zlib utility wherever you
find the libpng source files.
Libpng is thread safe, provided the threads are using different
instances of the structures. Each thread should have its own
png_struct and png_info instances, and thus its own image.
Libpng does not protect itself against two threads using the
same instance of a structure.
II. Structures
There are two main structures that are important to libpng, png_struct
and png_info. Both are internal structures that are no longer exposed
in the libpng interface (as of libpng 1.5.0).
The png_info structure is designed to provide information about the
PNG file. At one time, the fields of png_info were intended to be
directly accessible to the user. However, this tended to cause problems
with applications using dynamically loaded libraries, and as a result
a set of interface functions for png_info (the png_get_*() and png_set_*()
functions) was developed, and direct access to the png_info fields was
deprecated..
The png_struct structure is the object used by the library to decode a
single image. As of 1.5.0 this structure is also not exposed.
Almost all libpng APIs require a pointer to a png_struct as the first argument.
Many (in particular the png_set and png_get APIs) also require a pointer
to png_info as the second argument. Some application visible macros
defined in png.h designed for basic data access (reading and writing
integers in the PNG format) don't take a png_info pointer, but it's almost
always safe to assume that a (png_struct*) has to be passed to call an API
function.
You can have more than one png_info structure associated with an image,
as illustrated in pngtest.c, one for information valid prior to the
IDAT chunks and another (called "end_info" below) for things after them.
The png.h header file is an invaluable reference for programming with libpng.
And while I'm on the topic, make sure you include the libpng header file:
#include <png.h>
and also (as of libpng-1.5.0) the zlib header file, if you need it:
#include <zlib.h>
Types
The png.h header file defines a number of integral types used by the
APIs. Most of these are fairly obvious; for example types corresponding
to integers of particular sizes and types for passing color values.
One exception is how non-integral numbers are handled. For application
convenience most APIs that take such numbers have C (double) arguments;
however, internally PNG, and libpng, use 32 bit signed integers and encode
the value by multiplying by 100,000. As of libpng 1.5.0 a convenience
macro PNG_FP_1 is defined in png.h along with a type (png_fixed_point)
which is simply (png_int_32).
All APIs that take (double) arguments also have a matching API that
takes the corresponding fixed point integer arguments. The fixed point
API has the same name as the floating point one with "_fixed" appended.
The actual range of values permitted in the APIs is frequently less than
the full range of (png_fixed_point) (-21474 to +21474). When APIs require
a non-negative argument the type is recorded as png_uint_32 above. Consult
the header file and the text below for more information.
Special care must be take with sCAL chunk handling because the chunk itself
uses non-integral values encoded as strings containing decimal floating point
numbers. See the comments in the header file.
Configuration
The main header file function declarations are frequently protected by C
preprocessing directives of the form:
#ifdef PNG_feature_SUPPORTED
declare-function
#endif
...
#ifdef PNG_feature_SUPPORTED
use-function
#endif
The library can be built without support for thes

QWQ雨落轻尘
- 粉丝: 48
- 资源: 4
最新资源
- 10 kV和35 kV配电网系统的间歇性电弧接地过电压
- 直流无刷电机,外径41mm,径向长23.39mm,转速6000rpm,功率200W,气息长度预留1mm,槽满率67.5%,效率80.7%,最大输出功率320W
- 基于BP神经网络的多个输出数据的回归预测 matlab代码
- 基于高斯过程回归(GPR)的时间序列区间预测
- APA水平泊车算法,matlab和C++联合仿真,内含道路地图生成仿真算法,路径跟踪算法,车辆横向纵向控制算法,倒车路径规划算法,数据处理分析 车辆定位:通过车载传感器获取车辆当前位置和方向 目标
- 适用方向:基于LQR控制算法的直接横摆力矩控制(DYC)的四轮独立电驱动汽车的横向稳定性控制研究 主要内容:利用carsim建模,在simulink中搭建控制器,然后进行联合 实现汽车在高速低附着路
- 大厂FPGA APB verilog源代码,企业级应用源码,适合需要学习ic设计验证及soc开发的工程师 提供databook资料和verilog完整源代码 代码架构清晰、规范,便于阅读理解,可直接
- 特征值、左右特征向量计算,参与因子分析MATLAB代码
- maxwell电机电磁仿真 绕线式感应电机设计,串电阻启动等 电机仿真
- 自动紧急避撞系统(AEB),Carsim与simulink联合仿真; 车辆逆动力学模型; 制动安全距离计算; 定加速度; 可实现前车减速,前车静止,前车匀速纵向避撞;
- 改进A星算法 剔除冗余节点,光滑转折点 对比优化前后路径
- 无位置传感器无刷直流电机,一篇Sci的复现,采用反相电动势观测器的方法进行无位置传感器控制,反相电动势观测值和电机实际输出值很好吻合
- 电动叉车系统设计,重量检测,电机控制 电动随车叉车控制系统设计 程序,仿真,有演示视频 1、电机1-4模拟叉车车轮,四个按键,控制叉车前进、后 、左转、右转 2、电机5、6模拟叉车前叉、后叉;上叉、
- 基于fpga的native接口的DDR3的多功能读写测试 支持单字节读写测试 支持多字节读写测试 支持自动读写测试 带仿真文件,同时上板验证过 可用于学习
- 改进蚁群算法+动态窗口算法全局结合局部路径规划仿真 静态路径规划算法 采用改进蚁群算法,有单独对比代码 动态实时规划 采用动态窗口算法避开未知障碍物 可自行设置地图 未知静态障碍物 移动障碍物
- 并联混合动力电动汽车模型
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


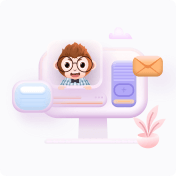