<?php
defined('IN_IA') or exit('Access Denied');
define('QRCODEPATH','/addons/group_buy/public/images/');
include_once '../addons/group_buy/sms.php';
include_once '../addons/group_buy/print_sn.php';
include_once "../addons/group_buy/markrting.php";
class Group_buyModuleWxapp extends WeModuleWxapp {
public $member = 'gpb_member';//用户表
public $ah = 'gpb_application_header';//申请团长表
public $rg = 'gpb_region';//地区表
public $vg = 'gpb_village';//小区表
public $goods = 'gpb_goods';//商品表
public $goods_cate = 'gpb_goods_cate';//商品分类表
public $goods_stock = 'gpb_goods_stock';//商品库存
public $goods_stock_logs = 'gpb_goods_stock_logs';//商品库存日志
public $adv = 'gpb_banner';//banner
public $coupon = 'gpb_ticket';//优惠卷
public $user_coupon = 'gpb_user_ticket';//用户领取的优惠券
public $order = 'gpb_order';//用户订单表
public $order_log = 'gpb_order_log';//用户订单日志表
public $action = 'gpb_action';//活动表
public $address = 'gpb_receiving_address';//收获地址表
public $snapshot = 'gpb_order_snapshot';//订单商品快照表
public $ban = 'gpb_banner';//banner广告
public $sure_order = 'gpb_sure_order';//订单核销表
public $action_village ='gpb_action_village';//活动小区关系表
public $action_goods ='gpb_action_goods';//活动商品关系表
public $cart ='gpb_cart';//购物车表
public $config ='gpb_config';//配置表
public $get_cash ='gpb_get_cash';//提现表
public $back_money ='gpb_back_money';//退款表
public $distribution ='gpb_distribution_list';//配送表
public $distribution_route ='gpb_distribution_route';//配送路线表
public $supplier ='gpb_supplier';//供应商
public $spec ='gpb_goods_spec';//规格表
public $spec_item ='gpb_goods_spec_item';//规格下参数表
public $goods_option ='gpb_goods_option';//参数规格erp
public $diy_page ='gpb_diy_page';//diy页面信息
public $diy_temp ='gpb_diy_temp';//diy模版信息
public $stream = 'gpb_order_stream';//流水
public $article = 'gpb_article';//文章表
public $article_class = 'gpb_article_class';//文章分类
public $team_cancel_goods = 'gpb_team_cancel_goods';//团长未开启的商品记录
public $head_history = 'gpb_head_history';//团长未开启的商品记录
public $pay_setting;
public $weid,$http,$https,$http_type,$https_type;
public $pageindex = 10;
//开始时间,固定一个小于当前时间的毫秒数即可
const twepoch = 1474990000000;//2016/9/28 0:0:0
//机器标识占的位数
const workerIdBits = 0;
//数据中心标识占的位数
const datacenterIdBits = 0;
//毫秒内自增数点的位数
const sequenceBits = 1;
protected $workId = 1;
protected $datacenterId = 1;
static $lastTimestamp = -1;
static $sequence = 0;
public function __construct( $workId=0, $datacenterId=0 )
{
global $_W, $_GPC;
if(!isset($_W['account']['key']) || empty($_W['account']['key'])){
$this->result("1","小程序appid有误");
}
if(!isset($_W['account']['secret']) || empty($_W['account']['secret'])){
$this->result("1","小程序secret有误");
}
$this->appid = $_W['account']['key'];//小程序appid
$this->secret = $_W['account']['secret'];//小程序secret
if(is_array($_W['account'])){
$is_true = true;
if(!isset($_W['account']['setting']['payment']['wechat']['mchid']) || empty($_W['account']['setting']['payment']['wechat']['mchid'])){
//$this->result("1","商户号有误");
$is_true = false;
}
if(!isset($_W['account']['setting']['payment']['wechat']['signkey']) || empty($_W['account']['setting']['payment']['wechat']['signkey'])){
//$this->result("1","支付密钥有误");
$is_true = false;
}
if($is_true){
$pay = [
'wechat'=>[
'mchid'=>$_W['account']['setting']['payment']['wechat']['mchid'],
'signkey'=>$_W['account']['setting']['payment']['wechat']['signkey'],
]
];
}
}else{
$is_true = true;
if(!isset($_W['account']->setting['payment']['wechat']['mchid']) || empty($_W['account']->setting['payment']['wechat']['mchid'])){
// $this->result("1","商户号有误");
$is_true = false;
}
if(!isset($_W['account']->setting['payment']['wechat']['signkey']) || empty($_W['account']->setting['payment']['wechat']['signkey'])){
// $this->result("1","支付密钥有误");
$is_true = false;
}
if($is_true){
$pay = [
'wechat'=>[
'mchid'=>$_W['account']->setting['payment']['wechat']['mchid'],
'signkey'=>$_W['account']->setting['payment']['wechat']['signkey'],
]
];
}
}
$this->pay_setting = $pay;
$this->weid = $_GPC['__uniacid'];
$this->pre = $_W['config']['db']['tablepre'];
//获取是否是对象储存
//判断当前是HTTP还是https
$this->http_type = ((isset($_SERVER['HTTPS']) && $_SERVER['HTTPS'] == 'on') || (isset($_SERVER['HTTP_X_FORWARDED_PROTO']) && $_SERVER['HTTP_X_FORWARDED_PROTO'] == 'https')) ? 'https://' : 'http://';
//获取是否是对象储存 tomedia解决
//机器ID范围判断
$maxWorkerId = -1 ^ (-1 << self::workerIdBits);
if($workId > $maxWorkerId || $workId< 0){
throw new \Exception("workerId can't be greater than ".$this->maxWorkerId." or
less than 0");
}
//数据中心ID范围判断
$maxDatacenterId = -1 ^ (-1 << self::datacenterIdBits);
if ($datacenterId > $maxDatacenterId || $datacenterId < 0) {
throw new \Exception("datacenter Id can't be greater than ".$maxDatacenterId."
or less than 0");
}
//赋值
$this->workId = $workId;
$this->datacenterId = $datacenterId;
}
//生成一个ID
public function nextId(){
$timestamp = $this->timeGen();
$lastTimestamp = self::$lastTimestamp;
//判断时钟是否正常
if ($timestamp < $lastTimestamp) {
throw new \Exception("Clock moved backwards. Refusing to generate id for %d
milliseconds", ($lastTimestamp - $timestamp));
}
//生成唯一序列
if ($lastTimestamp == $timestamp) {
$sequenceMask = -1 ^ (-1 << self::sequenceBits);
self::$sequence = (self::$sequence + 1) & $sequenceMask;
if (self::$sequence == 0) {
$timestamp = $this->tilNextMillis($lastTimestamp);
}
} else {
self::$sequence = 0;
}
self::$lastTimestamp = $timestamp;
//时间毫秒/数据中心ID/机器ID,要左移的位数
$timestampLeftShift = self::sequenceBits + self::workerIdBits +
self::datacenterIdBits;
$datacenterIdShift = self::sequenceBits + self::workerIdBits;
$workerIdShift = self::sequenceBits;
//组合4段数据返回: 时间戳.数据标识.工作机器.序列
$nextId = (($timestamp - self::twepoch) << $timestampLeftShift) |
($this->datacenterId << $datacenterIdShift) |
($this->workId << $workerIdShift) | self::$sequence;
$id = str_pad(abs($nextId)
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
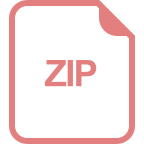
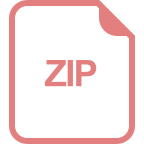
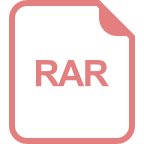
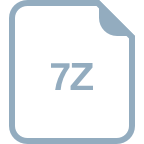
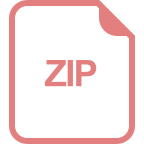
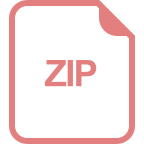
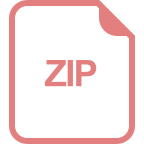
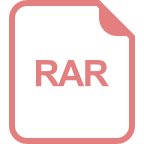
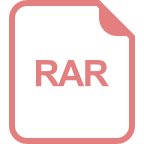
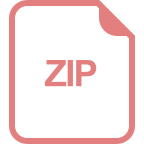
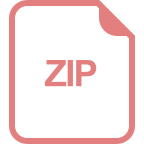
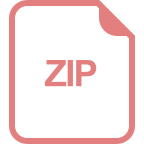
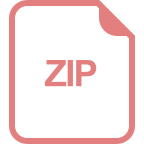
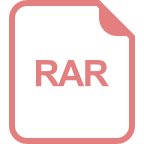
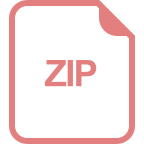
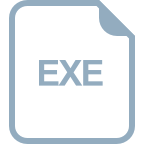
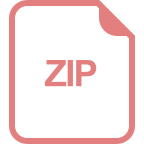
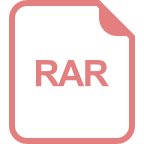
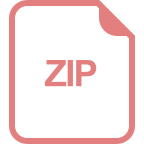
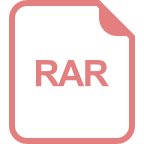
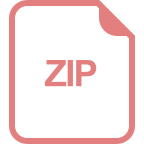
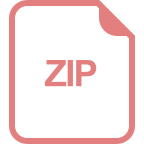
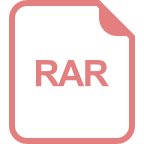
收起资源包目录

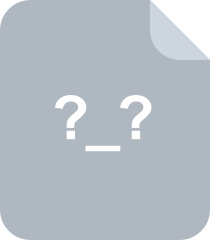
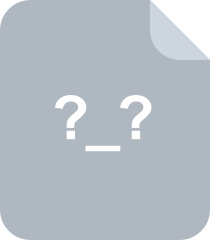
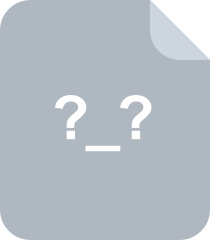
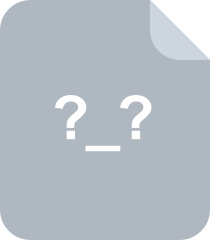
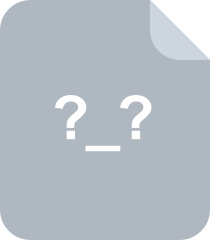
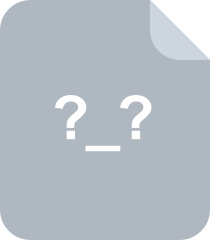
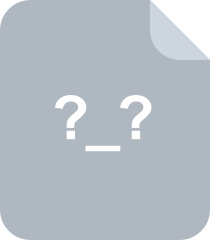
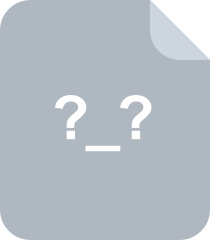
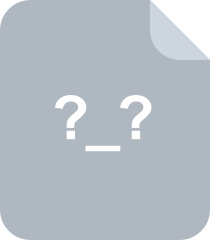
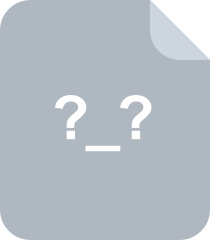
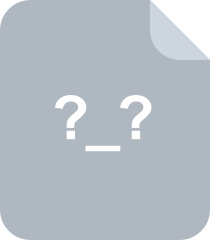
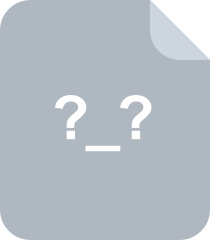
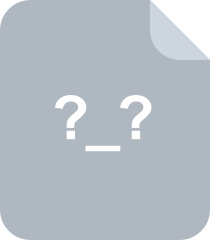
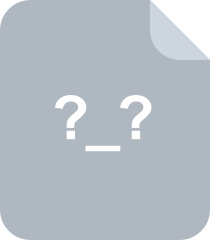
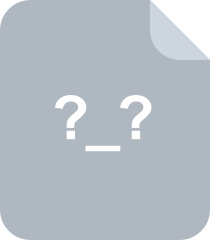
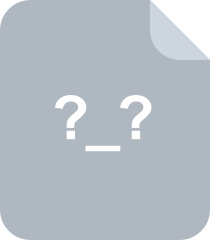
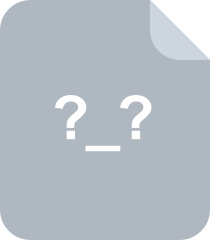
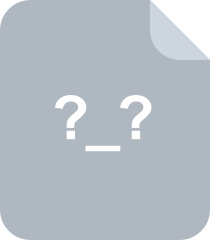
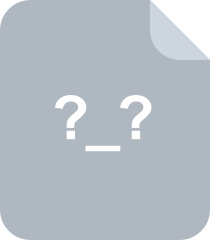
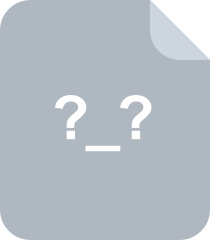
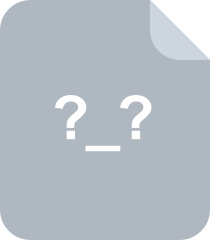
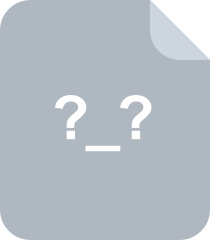
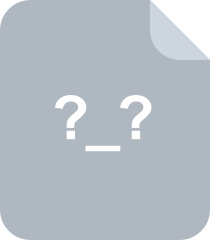
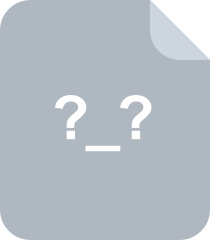
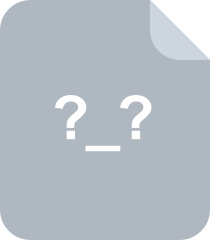
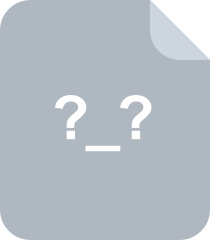
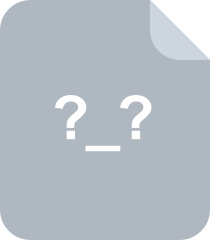
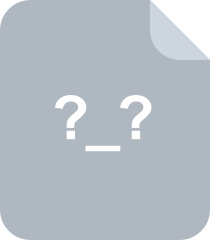
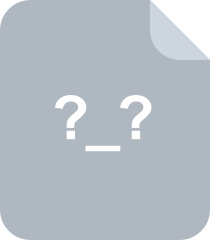
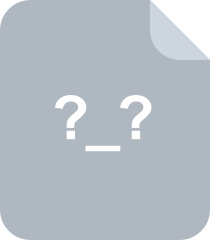
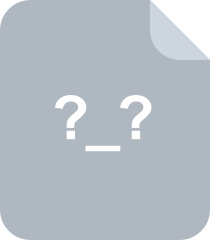
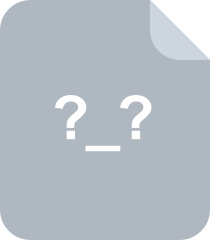
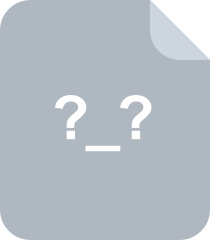
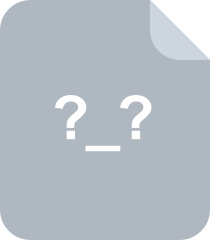
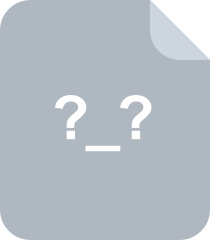
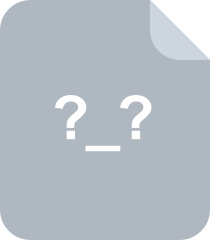
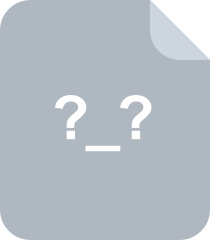
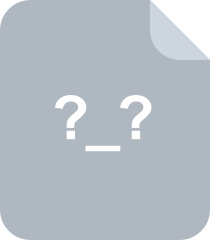
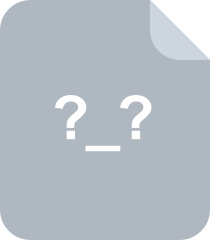
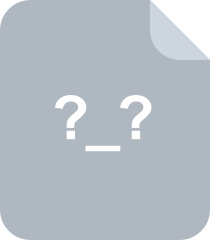
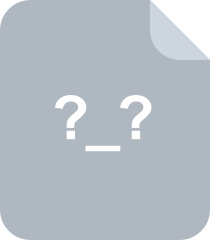
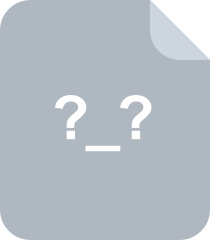
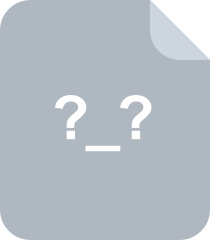
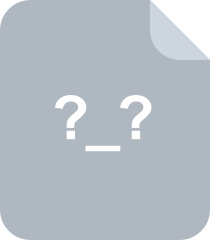
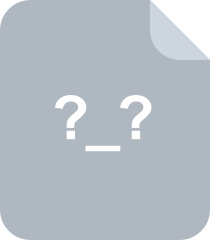
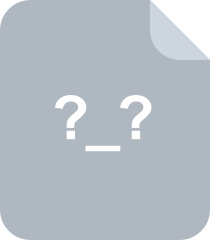
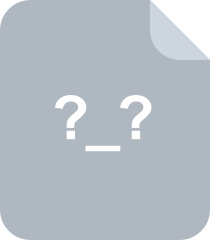
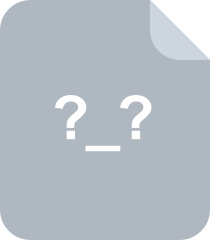
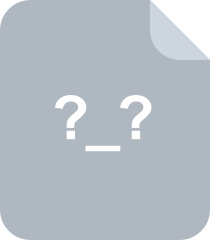
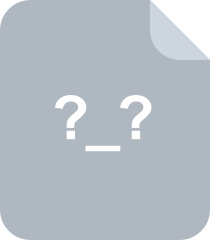
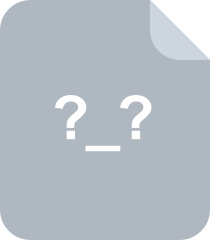
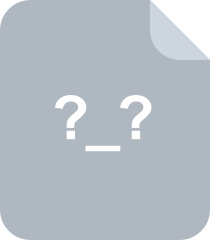
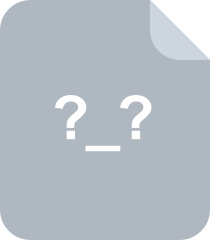
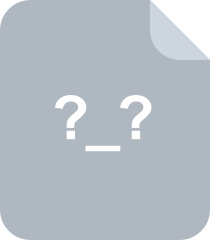
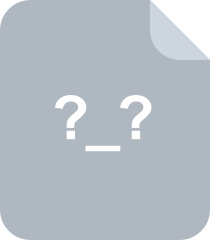
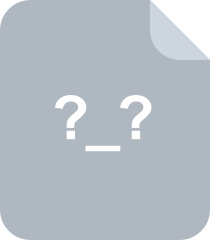
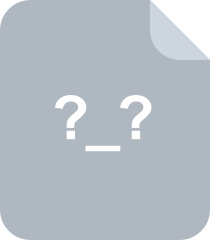
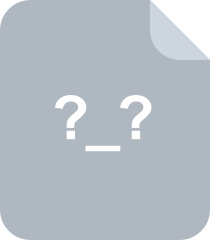
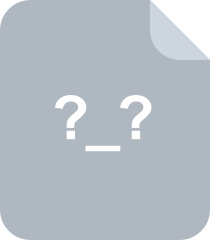
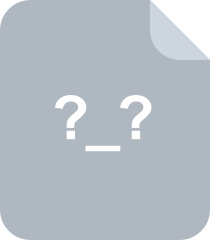
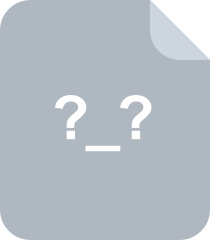
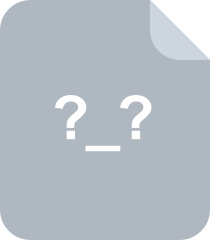
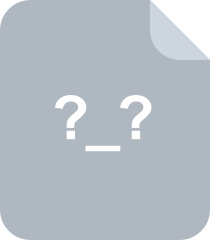
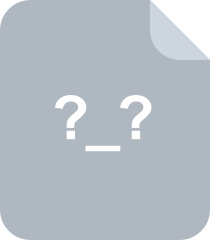
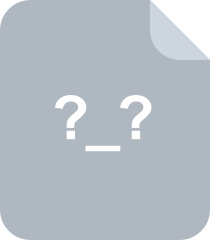
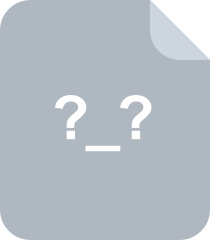
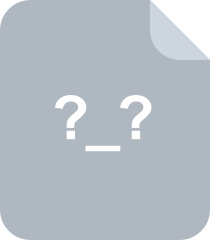
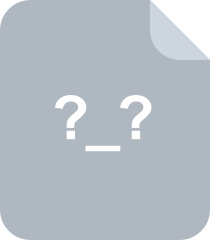
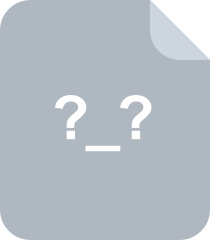
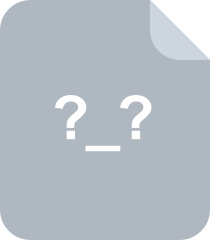
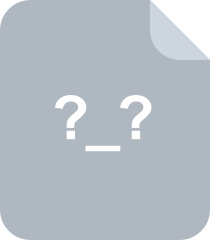
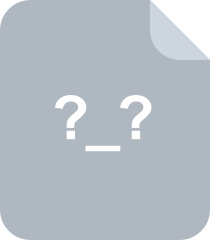
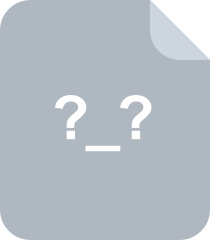
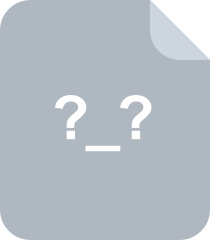
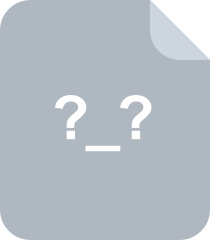
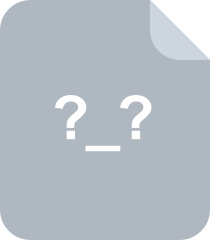
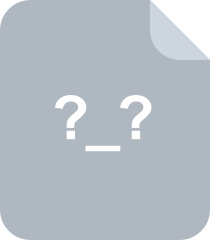
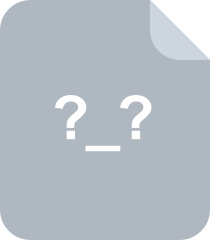
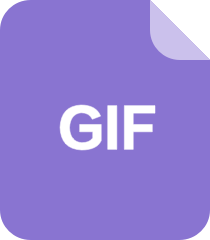
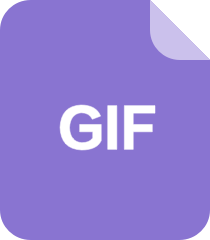
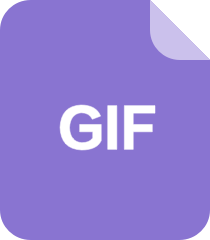
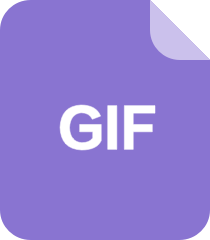
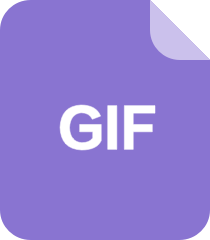
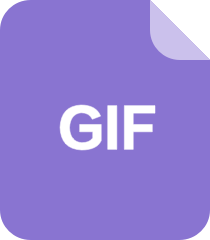
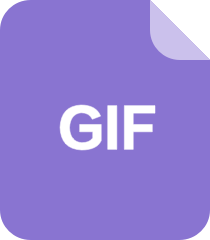
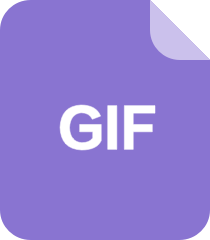
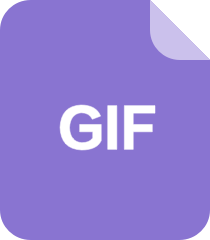
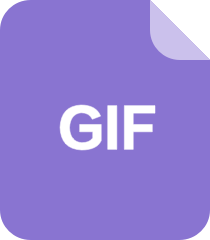
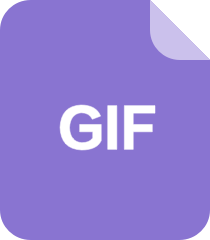
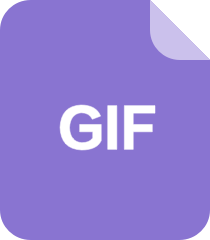
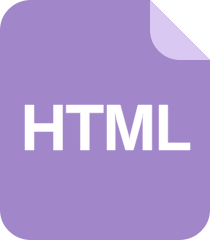
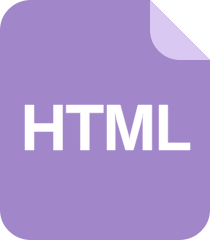
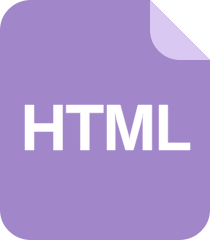
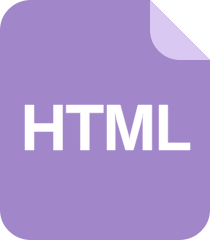
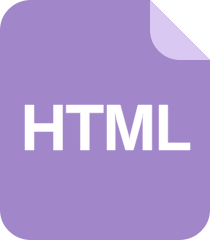
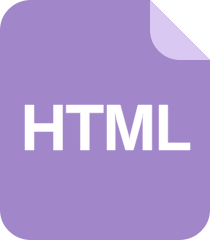
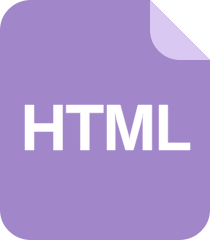
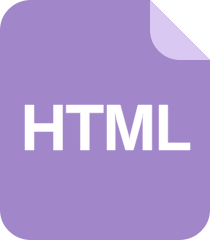
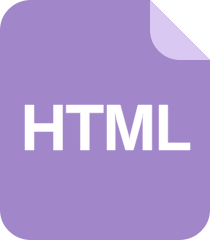
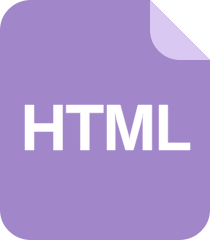
共 1546 条
- 1
- 2
- 3
- 4
- 5
- 6
- 16
资源评论
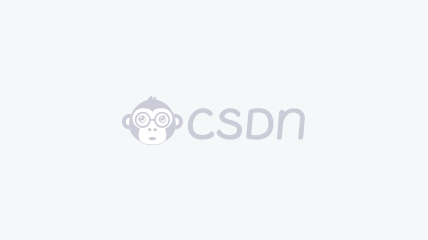

coding已疯狂
- 粉丝: 974
- 资源: 1362
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

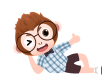
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


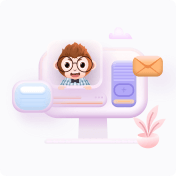
安全验证
文档复制为VIP权益,开通VIP直接复制
