/* =========================================================================
Unity - A Test Framework for C
ThrowTheSwitch.org
Copyright (c) 2007-24 Mike Karlesky, Mark VanderVoord, & Greg Williams
SPDX-License-Identifier: MIT
========================================================================= */
#include "unity.h"
#ifndef UNITY_PROGMEM
#define UNITY_PROGMEM
#endif
/* If omitted from header, declare overrideable prototypes here so they're ready for use */
#ifdef UNITY_OMIT_OUTPUT_CHAR_HEADER_DECLARATION
void UNITY_OUTPUT_CHAR(int);
#endif
/* Helpful macros for us to use here in Assert functions */
#define UNITY_FAIL_AND_BAIL do { Unity.CurrentTestFailed = 1; UNITY_OUTPUT_FLUSH(); TEST_ABORT(); } while (0)
#define UNITY_IGNORE_AND_BAIL do { Unity.CurrentTestIgnored = 1; UNITY_OUTPUT_FLUSH(); TEST_ABORT(); } while (0)
#define RETURN_IF_FAIL_OR_IGNORE do { if (Unity.CurrentTestFailed || Unity.CurrentTestIgnored) { TEST_ABORT(); } } while (0)
struct UNITY_STORAGE_T Unity;
#ifdef UNITY_OUTPUT_COLOR
const char UNITY_PROGMEM UnityStrOk[] = "\033[42mOK\033[0m";
const char UNITY_PROGMEM UnityStrPass[] = "\033[42mPASS\033[0m";
const char UNITY_PROGMEM UnityStrFail[] = "\033[41mFAIL\033[0m";
const char UNITY_PROGMEM UnityStrIgnore[] = "\033[43mIGNORE\033[0m";
#else
const char UNITY_PROGMEM UnityStrOk[] = "OK";
const char UNITY_PROGMEM UnityStrPass[] = "PASS";
const char UNITY_PROGMEM UnityStrFail[] = "FAIL";
const char UNITY_PROGMEM UnityStrIgnore[] = "IGNORE";
#endif
static const char UNITY_PROGMEM UnityStrNull[] = "NULL";
static const char UNITY_PROGMEM UnityStrSpacer[] = ". ";
static const char UNITY_PROGMEM UnityStrExpected[] = " Expected ";
static const char UNITY_PROGMEM UnityStrWas[] = " Was ";
static const char UNITY_PROGMEM UnityStrGt[] = " to be greater than ";
static const char UNITY_PROGMEM UnityStrLt[] = " to be less than ";
static const char UNITY_PROGMEM UnityStrOrEqual[] = "or equal to ";
static const char UNITY_PROGMEM UnityStrNotEqual[] = " to be not equal to ";
static const char UNITY_PROGMEM UnityStrElement[] = " Element ";
static const char UNITY_PROGMEM UnityStrByte[] = " Byte ";
static const char UNITY_PROGMEM UnityStrMemory[] = " Memory Mismatch.";
static const char UNITY_PROGMEM UnityStrDelta[] = " Values Not Within Delta ";
static const char UNITY_PROGMEM UnityStrPointless[] = " You Asked Me To Compare Nothing, Which Was Pointless.";
static const char UNITY_PROGMEM UnityStrNullPointerForExpected[] = " Expected pointer to be NULL";
static const char UNITY_PROGMEM UnityStrNullPointerForActual[] = " Actual pointer was NULL";
#ifndef UNITY_EXCLUDE_FLOAT
static const char UNITY_PROGMEM UnityStrNot[] = "Not ";
static const char UNITY_PROGMEM UnityStrInf[] = "Infinity";
static const char UNITY_PROGMEM UnityStrNegInf[] = "Negative Infinity";
static const char UNITY_PROGMEM UnityStrNaN[] = "NaN";
static const char UNITY_PROGMEM UnityStrDet[] = "Determinate";
static const char UNITY_PROGMEM UnityStrInvalidFloatTrait[] = "Invalid Float Trait";
#endif
const char UNITY_PROGMEM UnityStrErrShorthand[] = "Unity Shorthand Support Disabled";
const char UNITY_PROGMEM UnityStrErrFloat[] = "Unity Floating Point Disabled";
const char UNITY_PROGMEM UnityStrErrDouble[] = "Unity Double Precision Disabled";
const char UNITY_PROGMEM UnityStrErr64[] = "Unity 64-bit Support Disabled";
static const char UNITY_PROGMEM UnityStrBreaker[] = "-----------------------";
static const char UNITY_PROGMEM UnityStrResultsTests[] = " Tests ";
static const char UNITY_PROGMEM UnityStrResultsFailures[] = " Failures ";
static const char UNITY_PROGMEM UnityStrResultsIgnored[] = " Ignored ";
#ifndef UNITY_EXCLUDE_DETAILS
static const char UNITY_PROGMEM UnityStrDetail1Name[] = UNITY_DETAIL1_NAME " ";
static const char UNITY_PROGMEM UnityStrDetail2Name[] = " " UNITY_DETAIL2_NAME " ";
#endif
/*-----------------------------------------------
* Pretty Printers & Test Result Output Handlers
*-----------------------------------------------*/
/*-----------------------------------------------*/
/* Local helper function to print characters. */
static void UnityPrintChar(const char* pch)
{
/* printable characters plus CR & LF are printed */
if ((*pch <= 126) && (*pch >= 32))
{
UNITY_OUTPUT_CHAR(*pch);
}
/* write escaped carriage returns */
else if (*pch == 13)
{
UNITY_OUTPUT_CHAR('\\');
UNITY_OUTPUT_CHAR('r');
}
/* write escaped line feeds */
else if (*pch == 10)
{
UNITY_OUTPUT_CHAR('\\');
UNITY_OUTPUT_CHAR('n');
}
/* unprintable characters are shown as codes */
else
{
UNITY_OUTPUT_CHAR('\\');
UNITY_OUTPUT_CHAR('x');
UnityPrintNumberHex((UNITY_UINT)*pch, 2);
}
}
/*-----------------------------------------------*/
/* Local helper function to print ANSI escape strings e.g. "\033[42m". */
#ifdef UNITY_OUTPUT_COLOR
static UNITY_UINT UnityPrintAnsiEscapeString(const char* string)
{
const char* pch = string;
UNITY_UINT count = 0;
while (*pch && (*pch != 'm'))
{
UNITY_OUTPUT_CHAR(*pch);
pch++;
count++;
}
UNITY_OUTPUT_CHAR('m');
count++;
return count;
}
#endif
/*-----------------------------------------------*/
void UnityPrint(const char* string)
{
const char* pch = string;
if (pch != NULL)
{
while (*pch)
{
#ifdef UNITY_OUTPUT_COLOR
/* print ANSI escape code */
if ((*pch == 27) && (*(pch + 1) == '['))
{
pch += UnityPrintAnsiEscapeString(pch);
continue;
}
#endif
UnityPrintChar(pch);
pch++;
}
}
}
/*-----------------------------------------------*/
void UnityPrintLen(const char* string, const UNITY_UINT32 length)
{
const char* pch = string;
if (pch != NULL)
{
while (*pch && ((UNITY_UINT32)(pch - string) < length))
{
/* printable characters plus CR & LF are printed */
if ((*pch <= 126) && (*pch >= 32))
{
UNITY_OUTPUT_CHAR(*pch);
}
/* write escaped carriage returns */
else if (*pch == 13)
{
UNITY_OUTPUT_CHAR('\\');
UNITY_OUTPUT_CHAR('r');
}
/* write escaped line feeds */
else if (*pch == 10)
{
UNITY_OUTPUT_CHAR('\\');
UNITY_OUTPUT_CHAR('n');
}
/* unprintable characters are shown as codes */
else
{
UNITY_OUTPUT_CHAR('\\');
UNITY_OUTPUT_CHAR('x');
UnityPrintNumberHex((UNITY_UINT)*pch, 2);
}
pch++;
}
}
}
/*-----------------------------------------------*/
void UnityPrintNumberByStyle(const UNITY_INT number, const UNITY_DISPLAY_STYLE_T style)
{
if ((style & UNITY_DISPLAY_RANGE_INT) == UNITY_DISPLAY_RANGE_INT)
{
if (style == UNITY_DISPLAY_STYLE_CHAR)
{
/* printable characters plus CR & LF are printed
没有合适的资源?快使用搜索试试~ 我知道了~
使用CodeBlocks软件搭建的UnityTest测试框架
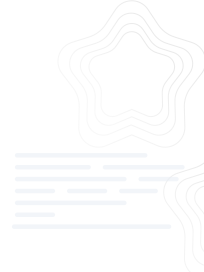
共33个文件
o:11个
c:10个
h:8个

需积分: 2 0 下载量 199 浏览量
2024-08-04
18:51:12
上传
评论
收藏 104KB RAR 举报
温馨提示
CodeBlocks版本: Code::Blocks 17.12 UnityTest测试框架: V2.6.0 核心文件: unity.c unity_fixture.c 测试代码: C代码 运行要求: 下载即可运行。使用 CodeBlocks 软件 打开 CodeBlocksUnityFixtureTest.cbp 软件即可运行。详细介绍可查看专栏 《【时时三省】主流测试框架分析和搭建》
资源推荐
资源详情
资源评论
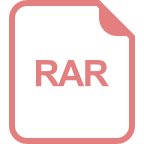
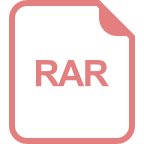
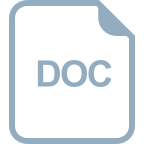
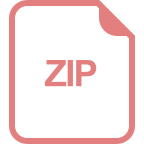
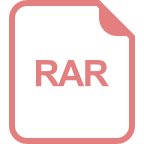
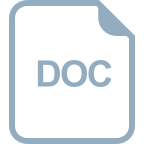
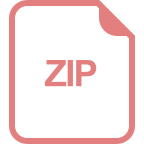
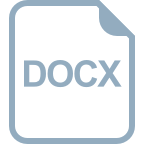
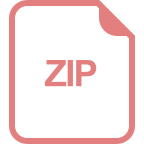
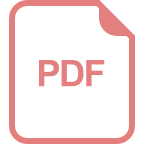
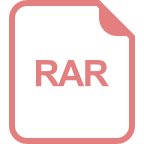
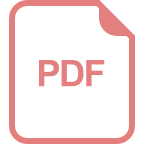
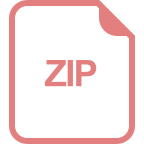
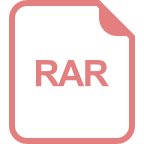
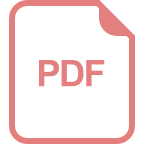
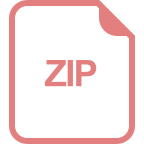
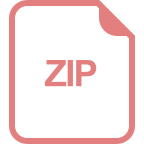
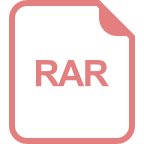
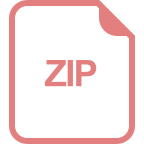
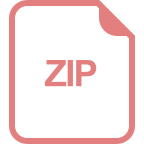
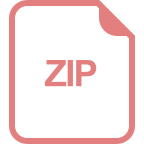
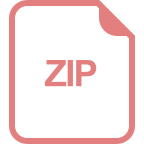
收起资源包目录


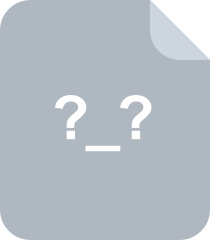
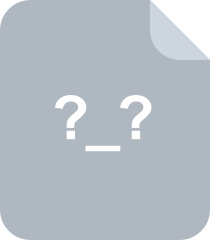
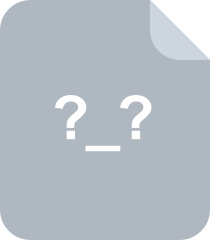
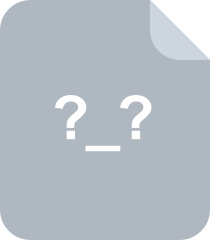
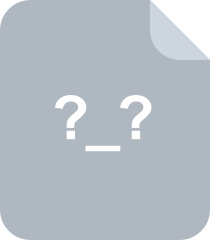


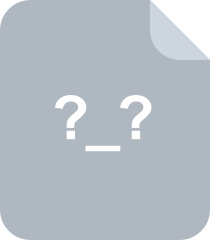
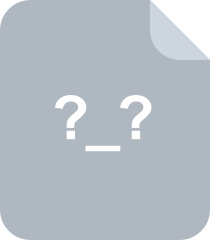
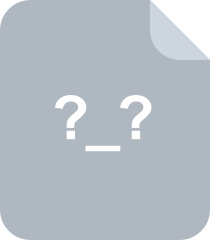
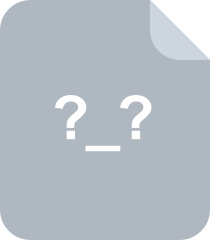
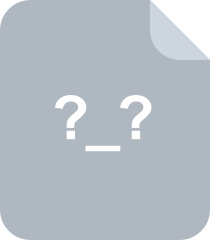
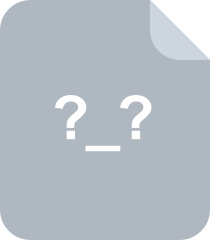
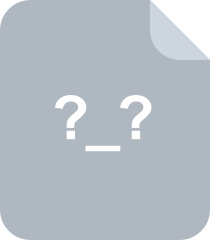
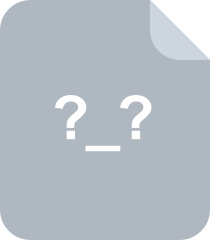
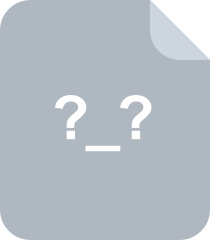
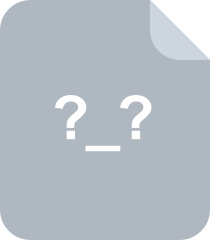
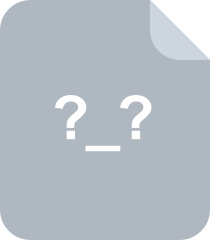
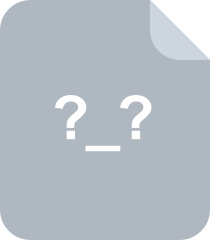
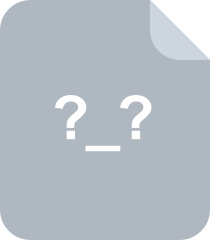
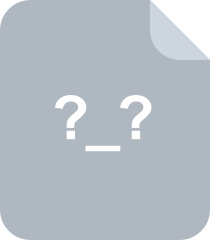
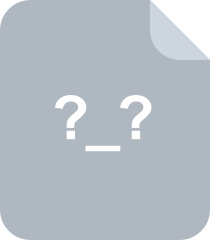
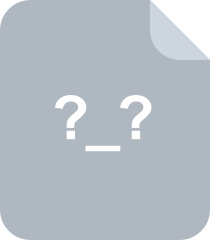
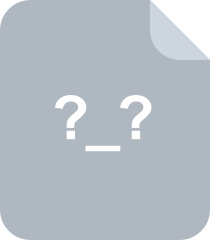
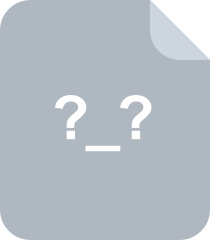
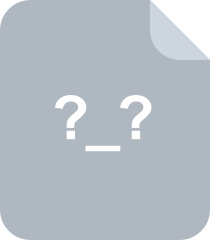


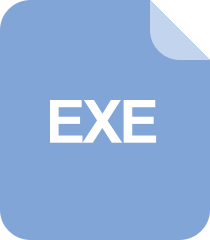
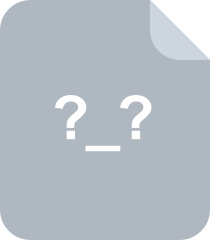
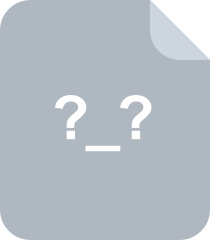
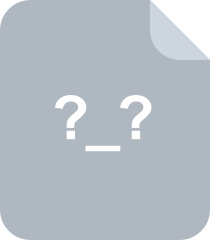
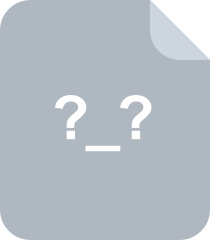
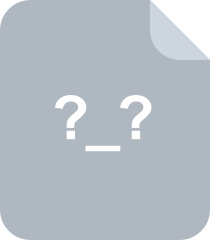
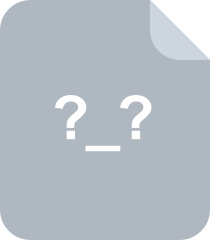
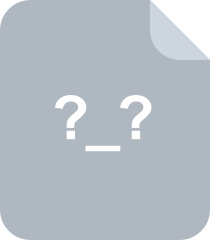
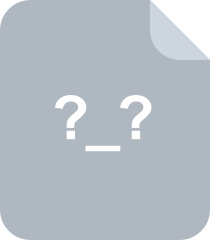
共 33 条
- 1
资源评论
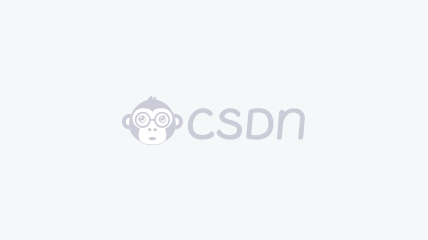

时时三省
- 粉丝: 1140
- 资源: 4
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

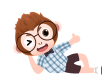
安全验证
文档复制为VIP权益,开通VIP直接复制
