**NOTE:** This repository is no longer supported or updated by GitHub. If you wish to continue to develop this code yourself, we recommend you fork it.
# OctoKit
[](https://github.com/Carthage/Carthage)
OctoKit is a Cocoa and Cocoa Touch framework for interacting with the [GitHub
API](https://developer.github.com/), built using
[AFNetworking](https://github.com/AFNetworking/AFNetworking),
[Mantle](https://github.com/Mantle/Mantle), and
[ReactiveCocoa](https://github.com/ReactiveCocoa/ReactiveCocoa).
## Making Requests
In order to begin interacting with the API, you must instantiate an
[OCTClient](OctoKit/OCTClient.h). There are two ways to create a client without
[authenticating](#authentication):
1. `-initWithServer:` is the most basic way to initialize a client. It accepts
an [OCTServer](OctoKit/OCTServer.h), which determines whether to connect to
GitHub.com or a [GitHub Enterprise](https://enterprise.github.com) instance.
1. `+unauthenticatedClientWithUser:` is similar, but lets you set an _active
user_, which is required for certain requests.
We'll focus on the second method, since we can do more with it. Let's create
a client that connects to GitHub.com:
```objc
OCTUser *user = [OCTUser userWithRawLogin:username server:OCTServer.dotComServer];
OCTClient *client = [OCTClient unauthenticatedClientWithUser:user];
```
After we've got a client, we can start fetching data. Each request method on
`OCTClient` returns
a [ReactiveCocoa](https://github.com/ReactiveCocoa/ReactiveCocoa) signal, which
is kinda like a [future or
promise](https://en.wikipedia.org/wiki/Futures_and_promises):
```objc
// Prepares a request that will load all of the user's repositories, represented
// by `OCTRepository` objects.
//
// Note that the request is not actually _sent_ until you use one of the
// -subscribe… methods below.
RACSignal *request = [client fetchUserRepositories];
```
However, you don't need a deep understanding of RAC to use OctoKit. There are
just a few basic operations to be aware of.
### Receiving results one-by-one
It often makes sense to handle each result object independently, so you can
spread any processing out instead of doing it all at once:
```objc
// This method actually kicks off the request, handling any results using the
// blocks below.
[request subscribeNext:^(OCTRepository *repository) {
// This block is invoked for _each_ result received, so you can deal with
// them one-by-one as they arrive.
} error:^(NSError *error) {
// Invoked when an error occurs.
//
// Your `next` and `completed` blocks won't be invoked after this point.
} completed:^{
// Invoked when the request completes and we've received/processed all the
// results.
//
// Your `next` and `error` blocks won't be invoked after this point.
}];
```
### Receiving all results at once
If you can't do anything until you have _all_ of the results, you can "collect"
them into a single array:
```objc
[[request collect] subscribeNext:^(NSArray *repositories) {
// Thanks to -collect, this block is invoked after the request completes,
// with _all_ the results that were received.
} error:^(NSError *error) {
// Invoked when an error occurs. You won't receive any results if this
// happens.
}];
```
### Receiving results on the main thread
The blocks in the above examples will be invoked in the background, to avoid
slowing down the main thread. However, if you want to run UI code, you shouldn't
do it in the background, so you must "deliver" results to the main thread
instead:
```objc
[[request deliverOn:RACScheduler.mainThreadScheduler] subscribeNext:^(OCTRepository *repository) {
// ...
} error:^(NSError *error) {
UIAlertView *alert = [[UIAlertView alloc] initWithTitle:@"Whoops!"
message:@"Something went wrong."
delegate:nil
cancelButtonTitle:nil
otherButtonTitles:nil];
[alert show];
} completed:^{
[self.tableView reloadData];
}];
```
### Cancelling a request
All of the `-subscribe…` methods actually return
a [RACDisposable](https://github.com/ReactiveCocoa/ReactiveCocoa/blob/master/ReactiveCocoa/Objective-C/RACDisposable.h)
object. Most of the time, you don't need it, but you can hold onto it if you
want to cancel requests:
```objc
- (void)viewWillAppear:(BOOL)animated {
[super viewWillAppear:animated];
RACDisposable *disposable = [[[[self.client
fetchUserRepositories]
collect]
deliverOn:RACScheduler.mainThreadScheduler]
subscribeNext:^(NSArray *repositories) {
[self addTableViewRowsForRepositories:repositories];
} error:^(NSError *error) {
UIAlertView *alert = [[UIAlertView alloc] initWithTitle:@"Whoops!"
message:@"Something went wrong."
delegate:nil
cancelButtonTitle:nil
otherButtonTitles:nil];
[alert show];
}];
// Save the disposable into a `strong` property, so we can access it later.
self.repositoriesDisposable = disposable;
}
- (void)viewWillDisappear:(BOOL)animated {
[super viewWillDisappear:animated];
// Cancels the request for repositories if it's still in progress. If the
// request already terminated, nothing happens.
[self.repositoriesDisposable dispose];
}
```
## Authentication
OctoKit supports two variants of [OAuth2](https://developer.github.com/v3/oauth/)
for signing in. We recommend the [browser-based
approach](#signing-in-through-a-browser), but you can also implement a [native
sign-in flow](#signing-in-through-the-app) if desired.
In both cases, you will need to [register your OAuth
application](https://github.com/settings/applications/new), and provide OctoKit
with your client ID and client secret before trying to authenticate:
```objc
[OCTClient setClientID:@"abc123" clientSecret:@"654321abcdef"];
```
### Signing in through a browser
With this API, the user will be redirected to their default browser (on OS X) or
Safari (on iOS) to sign in, and then redirected back to your app. This is the
easiest approach to implement, and means the user never has to enter their
password directly into your app — plus, they may even be signed in through the
browser already!
To get started, you must [implement a custom URL
scheme](https://developer.apple.com/library/ios/documentation/iPhone/Conceptual/iPhoneOSProgrammingGuide/AdvancedAppTricks/AdvancedAppTricks.html#//apple_ref/doc/uid/TP40007072-CH7-SW50)
for your app, then use something matching that scheme for your [OAuth
application's](https://github.com/settings/applications) callback URL. The
actual URL doesn't matter to OctoKit, so you can use whatever you'd like, just
as long as the URL scheme is correct.
Whenever your app is opened from your URL, or asked to open it, you must pass it
directly into `OCTClient`:
```objc
- (BOOL)application:(UIApplication *)application openURL:(NSURL *)URL sourceApplication:(NSString *)sourceApplication annotation:(id)annotation {
// For handling a callback URL like my-app://oauth
if ([URL.host isEqual:@"oauth"]) {
[OCTClient completeSignInWithCallbackURL:URL];
return YES;
} else {
return NO;
}
}
```
After that's set up properly, you can present the sign in page at any point. The
pattern is very similar to [making a request](#making-requests), except that you
receive an `OCTClient` instance as a reply:
```objc
[[OCTClient
signInToServerUsingWebBrowser:OCTServer.dotComServer scopes:OCTClientAuthorizationScopesUser]
subscribeNext
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
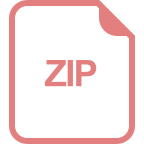
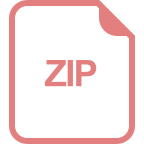
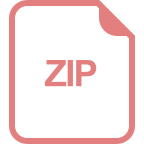
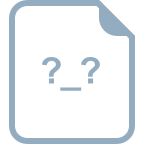
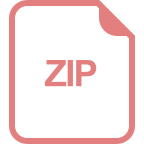
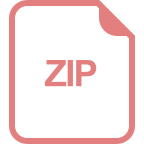
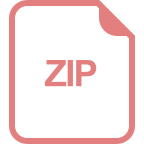
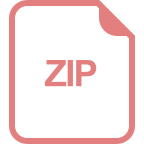
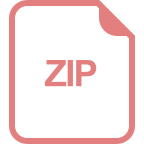
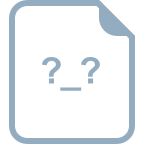
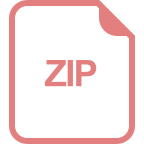
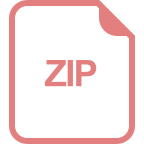
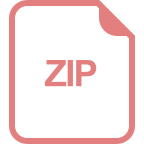
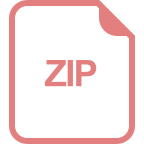
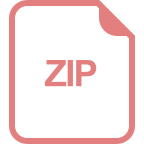
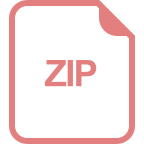
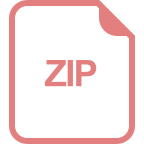
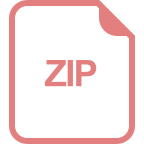
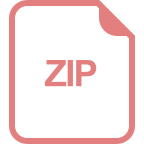
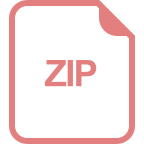
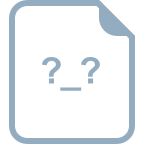
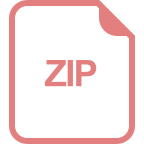
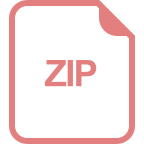
收起资源包目录

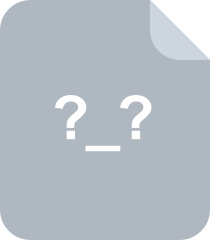
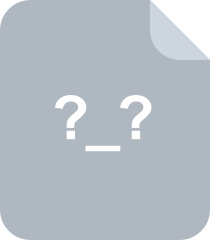
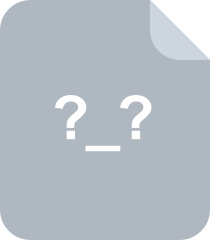
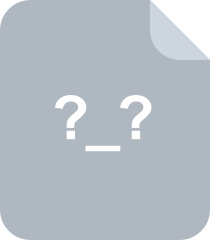
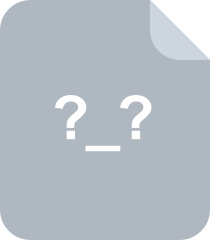
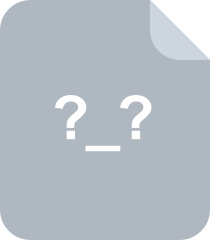
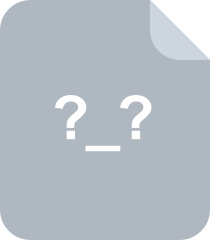
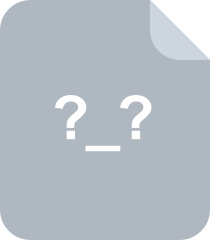
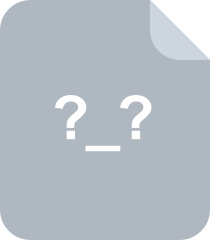
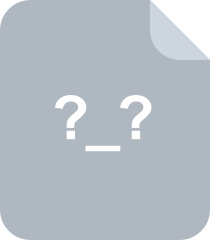
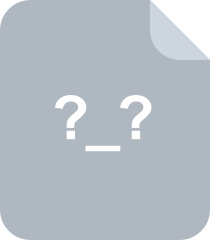
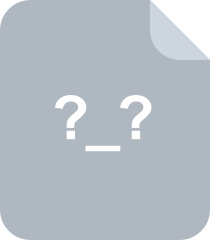
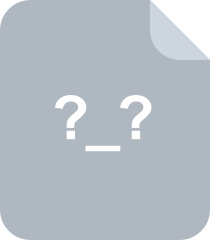
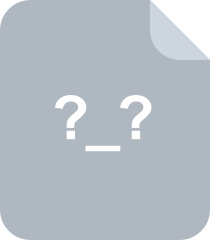
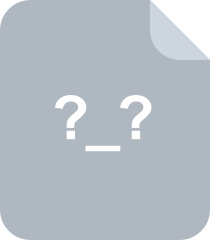
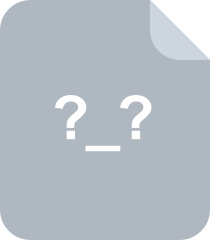
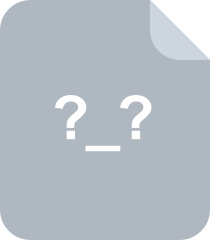
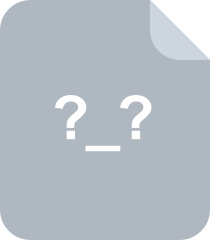
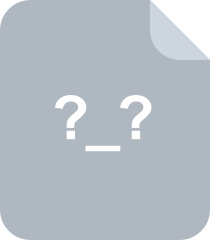
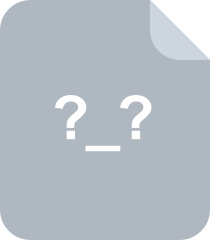
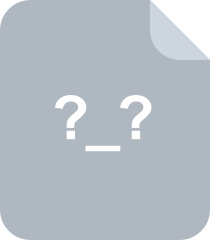
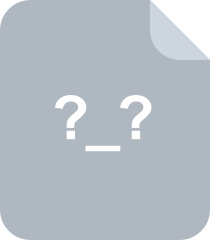
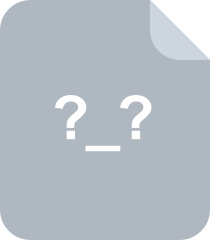
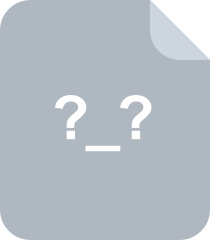
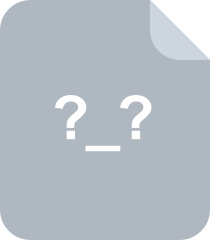
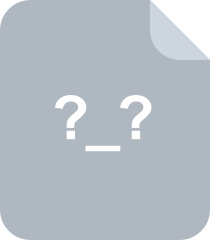
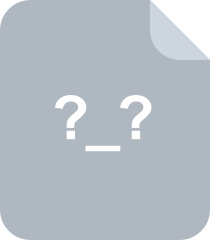
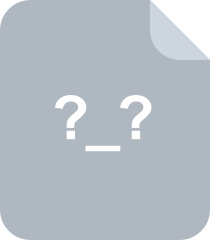
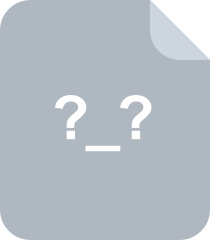
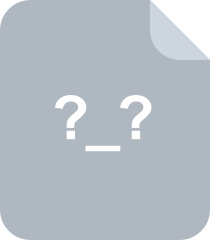
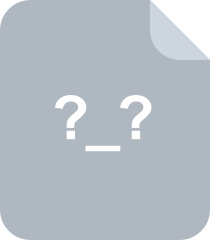
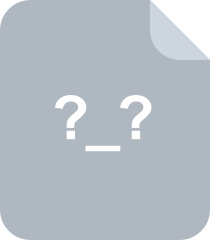
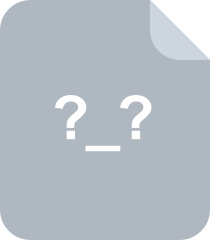
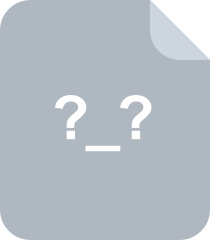
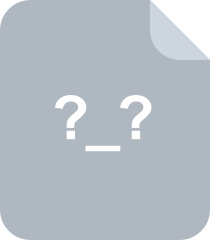
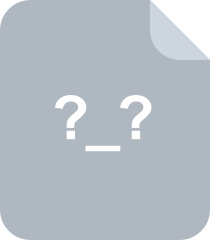
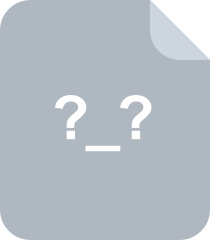
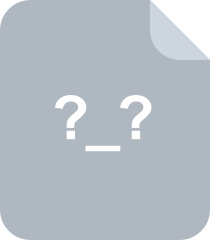
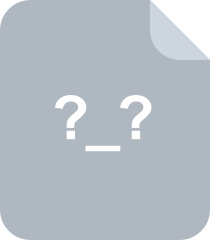
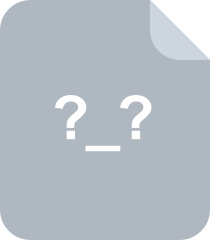
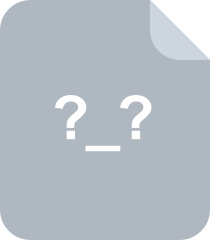
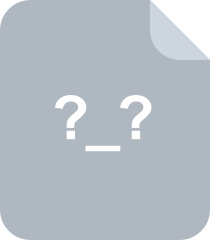
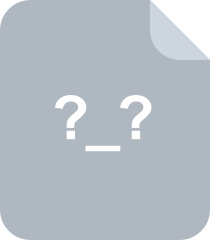
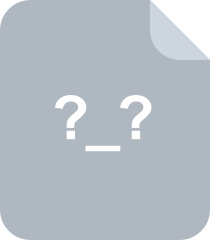
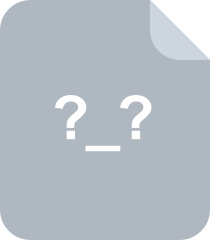
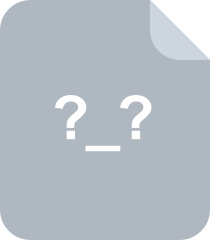
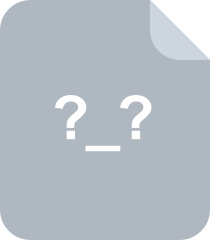
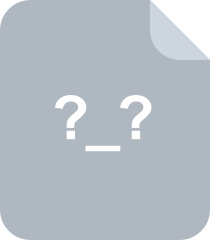
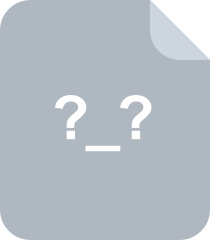
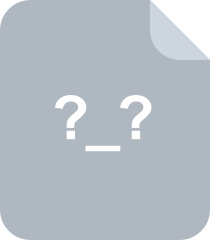
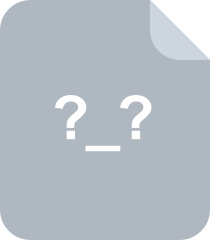
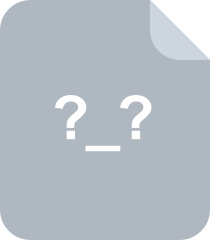
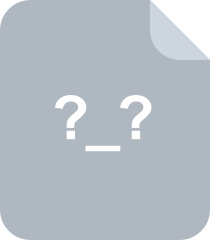
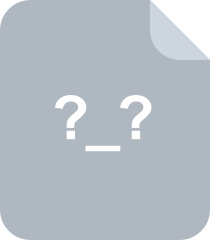
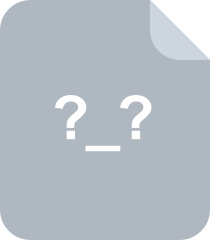
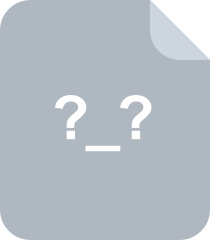
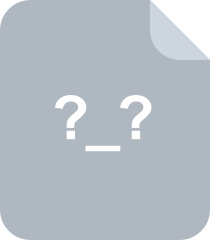
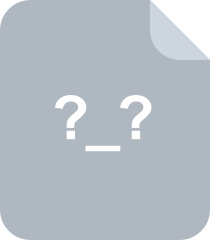
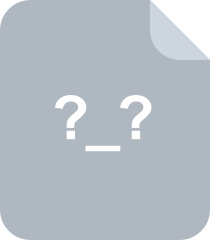
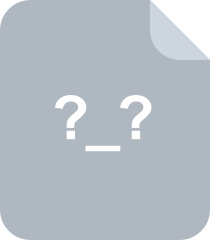
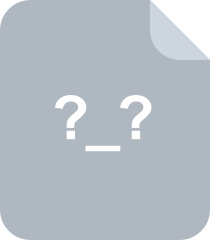
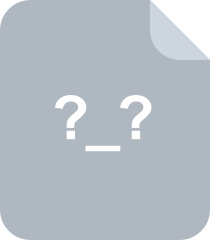
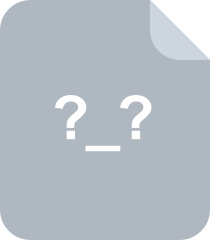
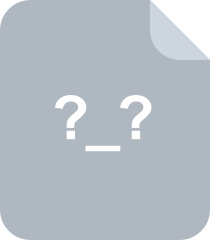
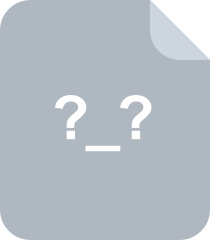
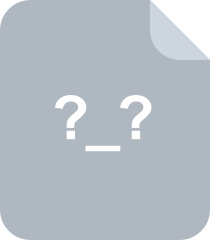
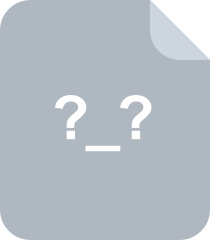
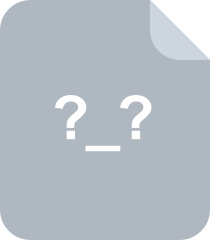
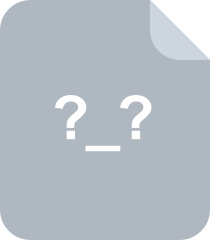
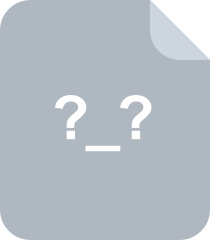
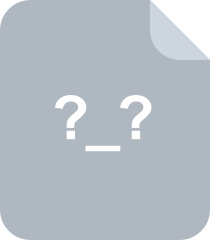
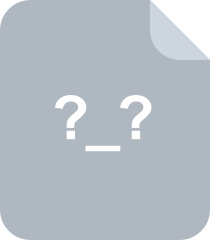
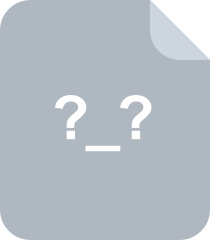
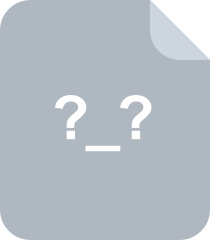
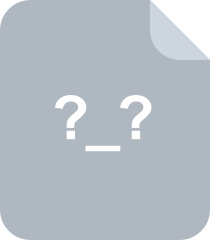
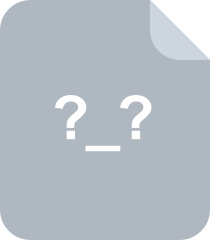
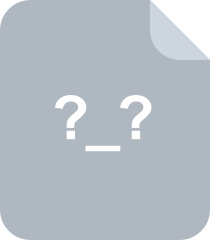
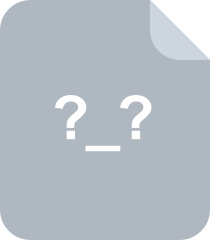
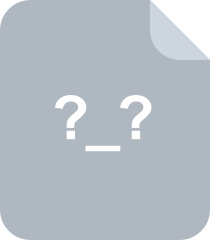
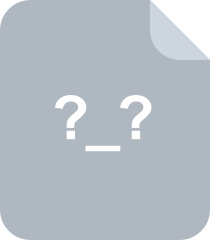
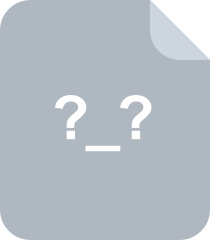
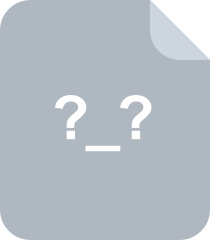
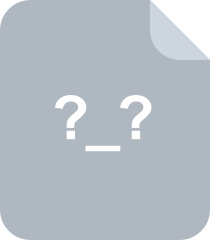
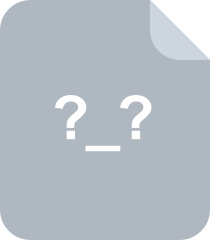
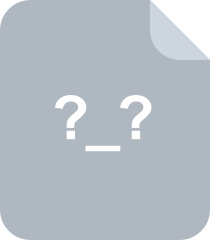
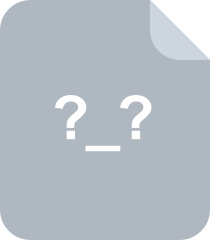
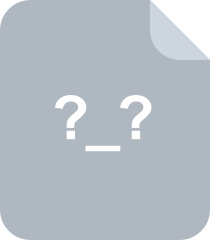
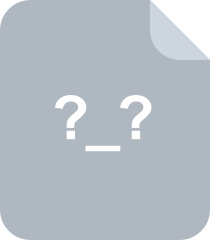
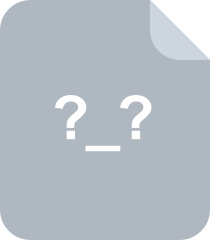
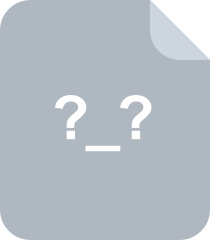
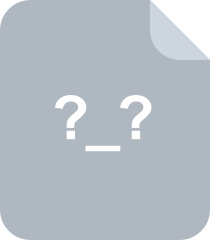
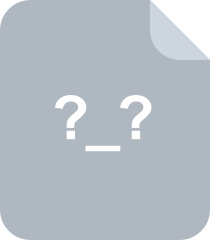
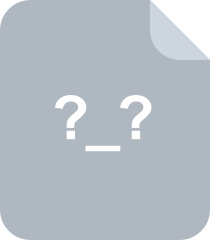
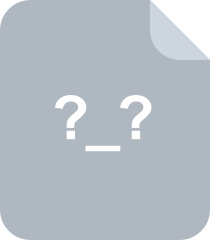
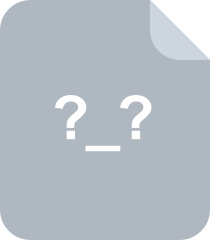
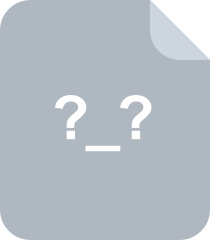
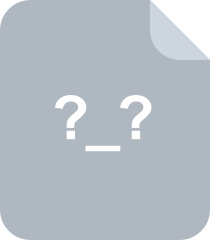
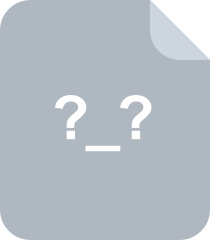
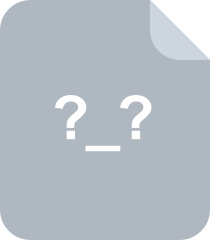
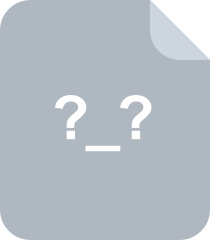
共 223 条
- 1
- 2
- 3
资源评论
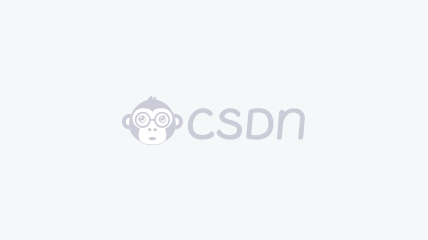

暮苍梧~
- 粉丝: 41
- 资源: 258
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

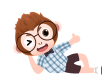
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


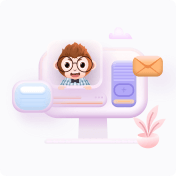
安全验证
文档复制为VIP权益,开通VIP直接复制
