数据结构实验代码链表的基本操作.rar
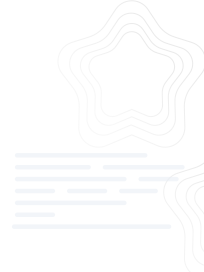

在计算机科学中,数据结构是组织、存储和处理数据的方式,它是编程的基础,尤其是在算法设计中扮演着核心角色。链表是一种重要的数据结构,它在内存中不是连续存储的,而是通过指针链接各个元素。这个"数据结构实验代码链表的基本操作.rar"文件包含了关于链表操作的实践代码,这将有助于我们深入理解链表的工作原理。 链表分为单链表、双链表和循环链表等类型,其中单链表是最基础的形式。单链表的每个节点包含两部分:数据域和指针域。数据域用于存储数据,而指针域则存储下一个节点的地址。链表的操作主要包括以下几点: 1. **创建链表**:初始化一个空链表,通常从头节点开始,头节点的指针域指向第一个元素节点,如果没有元素,则头节点的指针域为null。 2. **插入节点**:在链表中插入节点分为在头部插入、尾部插入以及在某个特定位置插入。头部插入只需改变头节点;尾部插入需要遍历链表找到最后一个节点,然后在其后插入;在特定位置插入则需要找到插入位置的前一个节点,然后插入新节点。 3. **删除节点**:删除操作也需要找到待删除节点的前一个节点,更新其指针域以跳过待删除节点,然后释放被删除节点的内存。 4. **查找节点**:在链表中查找特定值的节点,需要从头节点开始逐个比较,直到找到匹配的节点或遍历完链表。 5. **打印链表**:遍历链表并打印每个节点的数据,通常从头节点开始,沿着指针链直至末尾。 6. **反转链表**:这是一个常见的链表操作,需要重新设置节点间的指针关系,使链表反向。可以采用迭代或递归方式实现。 7. **判断链表是否有环**:通过快慢指针(也称龟兔赛跑法)可以检测链表中是否存在环,快指针每次移动两个节点,慢指针每次移动一个节点,如果两者相遇则链表有环。 8. **计算链表长度**:从头节点开始,每经过一个节点计数加一,直到达到尾节点。 9. **合并两个已排序的链表**:保持排序顺序,将两个链表合并成一个。可以采用迭代方法,比较当前节点的大小,选择较小的节点作为新的当前节点,并继续比较下一节点。 这些基本操作构成了链表操作的核心,理解和熟练掌握这些操作对于理解和编写涉及链表的复杂算法至关重要。在数据结构实验中,通过编写和测试这些代码,可以提升对链表的理解,进一步提高编程能力。在实际的软件开发中,链表广泛应用于各种场景,如缓存管理、队列、堆栈等。因此,对链表的深入理解对于任何IT专业人员来说都是非常重要的。
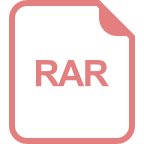
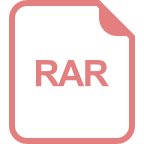
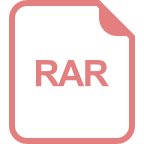
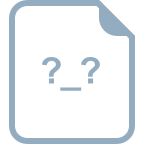
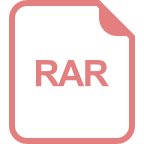
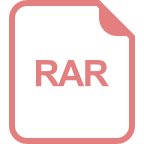
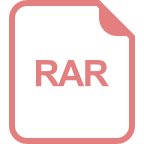
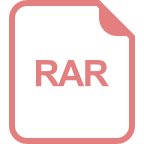
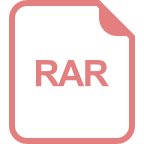
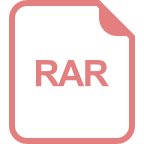
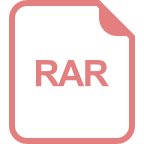
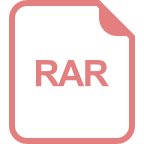
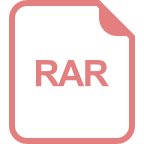
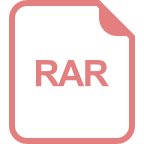
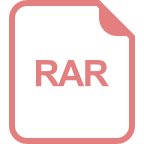
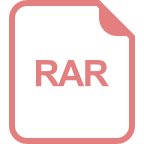
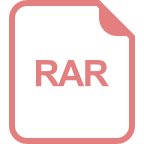
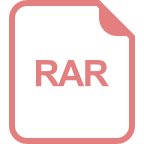



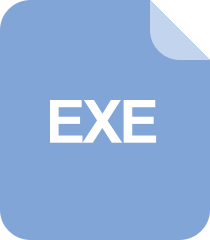
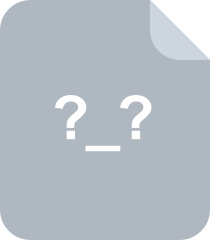


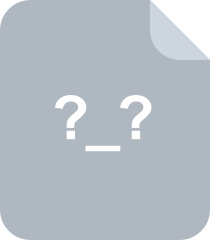
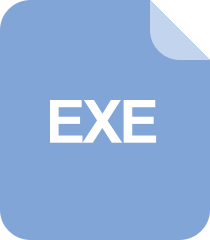

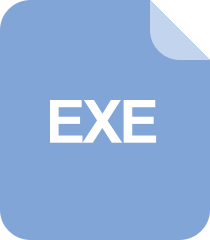
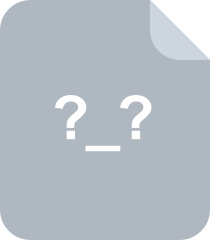

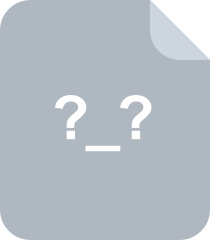
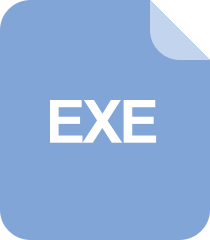

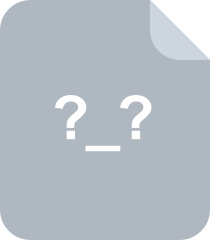
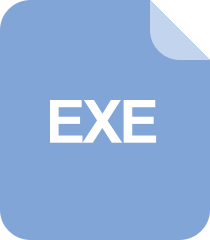

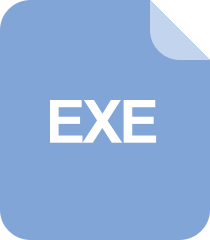
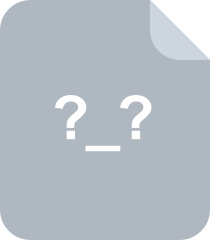

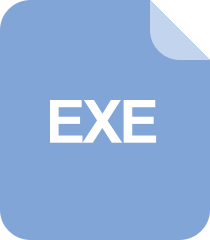
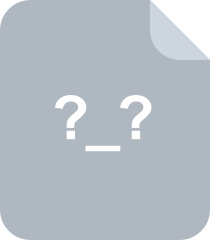
- 1
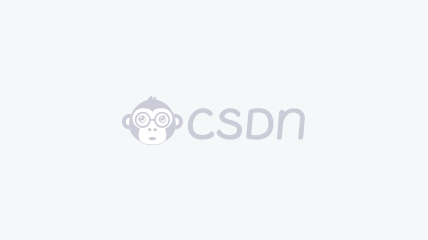


- 粉丝: 1099
- 资源: 4115
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

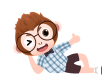
最新资源
- 基于matlab的传统滤波、Butterworth滤波、FIR、移动平均滤波、中值滤波、现代滤波、维纳滤波、自适应滤波、小波变,七种滤波方法,可替自己的数据进行滤波,程序已调通,可直接运行
- 基于Java语言开发的ASR+TTS+声纹识别功能的智能聊天小程序设计源码
- 含风电-光伏-光热电站电力系统N-k安全优化调度模型 关键词:N-K安全约束 光热电站 优化调度 参考文档:参考《光热电站促进风电消纳的电力系统优化调度》光热电站模型; 仿真软件: matlab+y
- 基于TypeScript和JavaScript的每日饮食与运动记录工具设计源码
- 基于JavaScript的仪器预约系统设计源码
- 基于Vue的依沫一站式内容资源变现博客设计源码
- 基于SSM框架与微信小程序的宠物管理系统源码设计
- 基于宝塔Linux面板7.9.0免费版的7.9.2兼容CSS美化设计源码
- 基于ActiveReports的C#报表控件设计源码
- 基于C#与Shell语言的SangServerTool服务器DDNS与SSL证书申请工具设计源码
- 基于SpringBoot+Vue的智能停车场管理系统设计源码
- 基于Shell、Python、PHP、HTML的zzxia-op-super-invincible-lollipop代码构建部署运维工具箱设计源码
- 华为FusionCompute 8.0.1 集成设计指导书
- 基于C语言实现的新型疫苗接种管理系统设计源码
- 基于JavaScript和微信小程序的抖音本地生活团购系统源码搭建与部署方案
- 电力电子boost升压电路MATLAB仿真,pi控制闭环(15r)滑模控制改进版(29r)24升48V,电压可修改 基于反激变器的升压电路,降压电路boost buck的MATLAB仿真,PLECS也

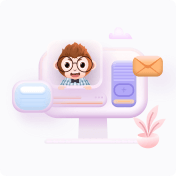
