package com.yeeee.crowdfunding.service.impl;
import cn.hutool.core.collection.CollectionUtil;
import com.github.pagehelper.Page;
import com.github.pagehelper.PageHelper;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Lists;
import com.yeeee.crowdfunding.convert.*;
import com.yeeee.crowdfunding.exception.BizException;
import com.yeeee.crowdfunding.mapper.*;
import com.yeeee.crowdfunding.model.entity.*;
import com.yeeee.crowdfunding.model.vo.*;
import com.yeeee.crowdfunding.service.ProjectService;
import com.yeeee.crowdfunding.utils.BusinessUtils;
import com.yeeee.crowdfunding.utils.DateConvertUtil;
import lombok.RequiredArgsConstructor;
import lombok.extern.slf4j.Slf4j;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import vip.yeee.memo.integrate.common.websecurity.context.SecurityContext;
import java.util.Arrays;
import java.util.Date;
import java.util.List;
import java.util.Optional;
import java.util.stream.Collectors;
/**
* description......
*
* @author yeeee
* @since 2022/4/29 21:38
*/
@Slf4j
@RequiredArgsConstructor
@Service
public class ProjectServiceImpl implements ProjectService {
private final ProjectMapper projectMapper;
private final ProjectConvert projectConvert;
private final InitiatorInfoVOConvert initiatorInfoVOConvert;
private final ProjectDetailConvert projectDetailConvert;
private final ProjectRepayConvert projectRepayConvert;
private final CommentConvert commentConvert;
private final ProjectProgressConvert projectProgressConvert;
private final UserConvert userConvert;
private final OrderMapper orderMapper;
private final ProjectDetailMapper projectDetailMapper;
private final ProjectProgressMapper projectProgressMapper;
private final ProjectRepayMapper projectRepayMapper;
private final CommentMapper commentMapper;
private final UserMapper userMapper;
private final InitiatorPersonInfoMapper initiatorPersonInfoMapper;
private final InitiatorCompanyInfoMapper initiatorCompanyInfoMapper;
private final ProjectCategoryMapper projectCategoryMapper;
private final ReceiveInformationMapper receiveInformationMapper;
private final ReceiveInfoConvert receiveInfoConvert;
private final ProjectCategoryConvert projectCategoryConvert;
@Override
public IndexProjectListVO getIndexShowProject() {
IndexProjectListVO indexProjectListVO = new IndexProjectListVO();
// 热门
List<Project> hotList = projectMapper.getOrderLimitList(new Project().setHasIndex(1)
, ImmutableMap.of("orderField", "has_fund_raising", "orderSort", "desc", "limit", 3));
List<ProjectVO> hotVOList = Optional.ofNullable(hotList).orElseGet(Lists::newArrayList)
.stream()
.map(projectConvert::project2VO)
.collect(Collectors.toList());
indexProjectListVO.setHotList(hotVOList);
// 公益
List<Project> welfareList = projectMapper.getOrderLimitList(new Project().setCategoryId(1)
, ImmutableMap.of("orderField", "has_fund_raising", "orderSort", "desc", "limit", 3));
List<ProjectVO> welfareVOList = Optional.ofNullable(welfareList).orElseGet(Lists::newArrayList)
.stream()
.map(projectConvert::project2VO)
.collect(Collectors.toList());
indexProjectListVO.setWelfareList(welfareVOList);
// 农业
List<Project> agList = projectMapper.getOrderLimitList(new Project().setCategoryId(2)
, ImmutableMap.of("orderField", "has_fund_raising", "orderSort", "desc", "limit", 3));
List<ProjectVO> agVOList = Optional.ofNullable(agList).orElseGet(Lists::newArrayList)
.stream()
.map(projectConvert::project2VO)
.collect(Collectors.toList());
indexProjectListVO.setAgList(agVOList);
// 出版
List<Project> publishList = projectMapper.getOrderLimitList(new Project().setCategoryId(3)
, ImmutableMap.of("orderField", "has_fund_raising", "orderSort", "desc", "limit", 3));
List<ProjectVO> publishVOList = Optional.ofNullable(publishList).orElseGet(Lists::newArrayList)
.stream()
.map(projectConvert::project2VO)
.collect(Collectors.toList());
indexProjectListVO.setPublishList(publishVOList);
// 艺术
List<Project> artList = projectMapper.getOrderLimitList(new Project().setCategoryId(4)
, ImmutableMap.of("orderField", "has_fund_raising", "orderSort", "desc", "limit", 3));
List<ProjectVO> artVOList = Optional.ofNullable(artList).orElseGet(Lists::newArrayList)
.stream()
.map(projectConvert::project2VO)
.collect(Collectors.toList());
indexProjectListVO.setArtList(artVOList);
return indexProjectListVO;
}
@Override
public PageVO<ProjectVO> getProjectPageList(ProjectPageReqVO reqVO) {
Page<ProjectVO> page = PageHelper.startPage(reqVO.getPageNum(), reqVO.getPageSize());
Project query = new Project();
if (reqVO.getProjectVO() != null) {
query.setKeyword(reqVO.getProjectVO().getKeyword())
.setCategoryId(reqVO.getProjectVO().getProjectType());
}
query.setHasAudits(1);
List<Project> projectList = projectMapper.getList(query);
List<ProjectVO> result = Optional.ofNullable(projectList).orElseGet(Lists::newArrayList)
.stream()
.map(projectConvert::project2VO)
.collect(Collectors.toList());
return new PageVO<>(page.getPageNum(), page.getPageSize(), page.getPages(), page.getTotal(), result);
}
@Override
public PageVO<ProjectVO> getMyselfProjectList(ProjectPageReqVO reqVO) {
Page<ProjectVO> page = PageHelper.startPage(reqVO.getPageNum(), 5);
Project query = new Project();
query.setUserId(BusinessUtils.getCurUserId());
List<Project> projectList = projectMapper.getList(query);
List<ProjectVO> result = Optional.ofNullable(projectList).orElseGet(Lists::newArrayList)
.stream()
.map(projectConvert::project2VO)
.collect(Collectors.toList());
return new PageVO<>(page.getPageNum(), page.getPageSize(), page.getPages(), page.getTotal(), result);
}
@Override
public ProjectDetailVO getIndexProjectDetail(Integer id) {
if (id == null) {
throw new BizException("项目ID不能为空");
}
Project project = projectMapper.getOne(new Project().setId(id));
if (project == null) {
throw new BizException("项目不存在");
}
ProjectDetailVO projectDetailVO = projectConvert.project2DetailVO(project);
List<ProjectItemVO> projectItemVOS = Optional.ofNullable(projectDetailMapper.getList(new ProjectDetail().setProjectId(project.getId()))).orElseGet(Lists::newArrayList)
.stream()
.map(projectDetailConvert::detail2VO)
.collect(Collectors.toList());
projectDetailVO.setItemVOList(projectItemVOS);
List<ProjectRepayVO> repayVOList = Optional.ofNullable(projectRepayMapper.getList(new ProjectRepay().setProjectId(project.getId()))).orElseGet(Lists::newArrayList)
.stream()
.map(projectRepayConvert::projectRepay2VO)
.collect(Collectors.toList());
projectDetailVO.setRepayVOList(repayVOList);
List<CommentVO> commentVOList = Optional.ofNullable(commentMapper.getList(new Comment().setProject(project.getId()))).orElseGet(Lists::newArrayList)
.stream()
.map(commentConvert::comment2VO)
.collect(Collectors.toList());
projectDetailVO.setCommentVOList(co
没有合适的资源?快使用搜索试试~ 我知道了~
基于springboot+mybatis+mysql实现的众筹管理系统(分前后台) 【源码+数据库】
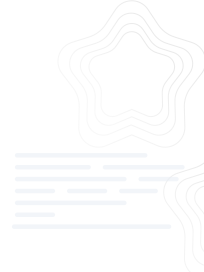
共822个文件
java:167个
js:167个
jpg:132个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 30 浏览量
2024-11-06
22:52:06
上传
评论
收藏 27.21MB ZIP 举报
温馨提示
一、、技术实现 开发语言:Java 框架:springboot,mybatis,spring JDK版本:JDK1.8 数据库:mysql 5.5及以上 数据库工具:Navicat11 开发软件:eclipse/idea 二、系统划分与功能 系统分为后台管理员和前台普通用户两种角色,主要功能如下: 用户登录 用户注册 首页,热门推荐 奖励众筹 公益众筹 立即支持,分享, 评论:可发布图片或文字 个人中心: 我的发起 我的订单 订单处理 我的关注 消息 我的评论 我的私信 设置 个人资料 修改密码 收货地址 系统注销 项目管理模块 用户管理模块 订单管理模块 项目类别管理模块等功能
资源推荐
资源详情
资源评论
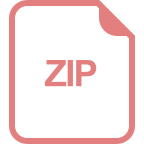
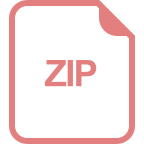
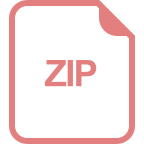
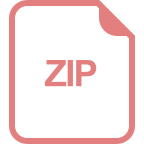
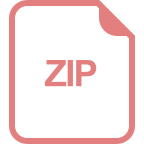
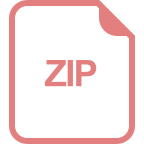
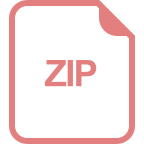
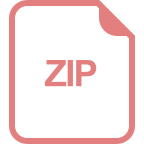
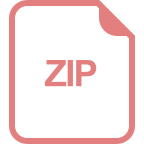
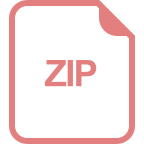
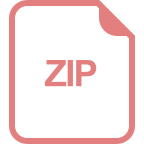
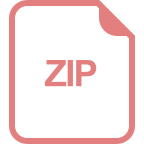
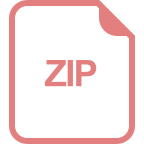
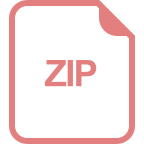
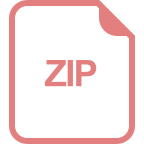
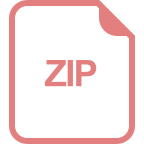
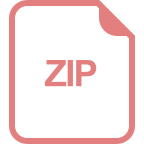
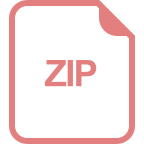
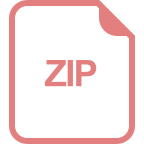
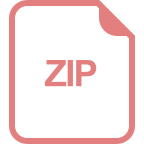
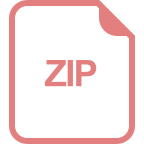
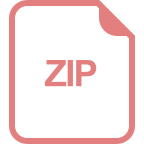
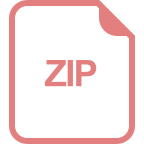
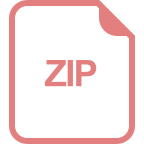
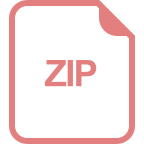
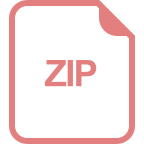
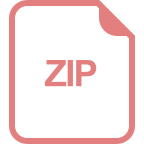
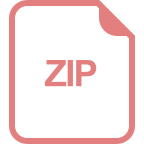
收起资源包目录

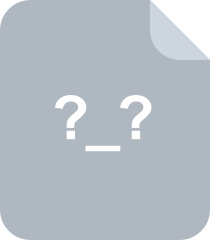
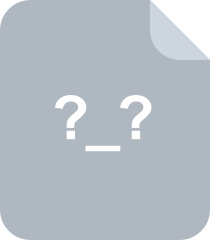
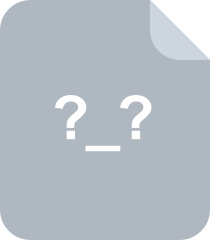
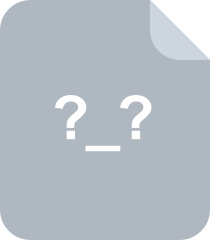
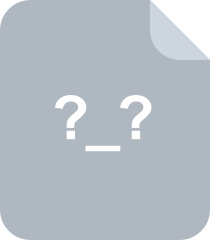
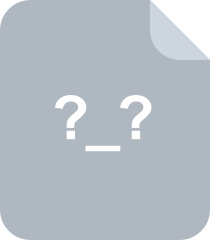
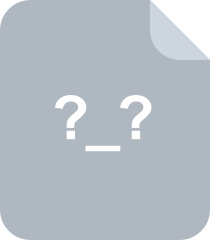
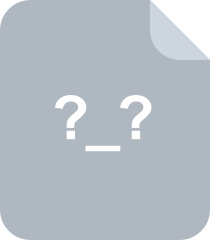
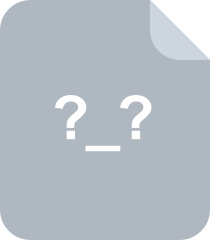
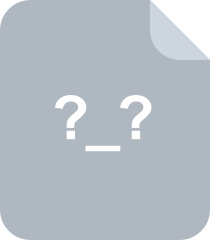
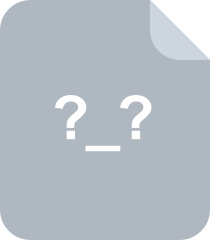
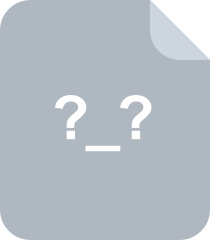
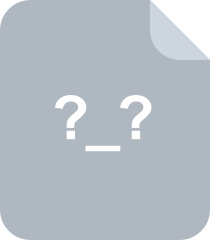
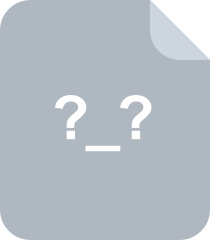
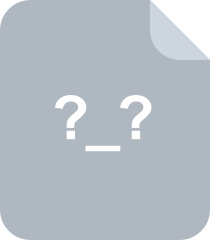
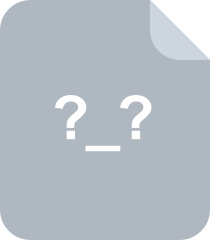
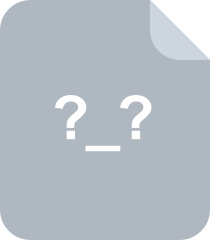
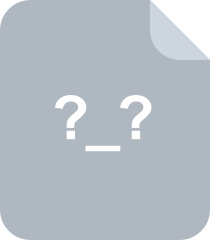
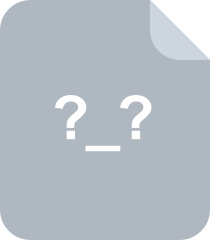
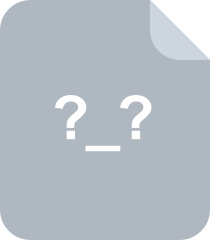
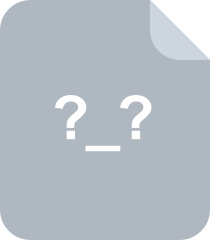
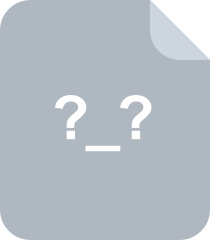
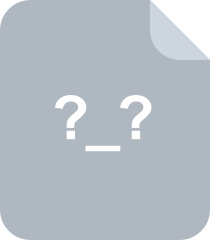
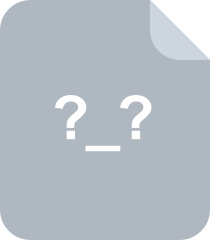
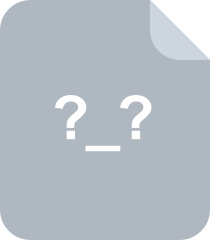
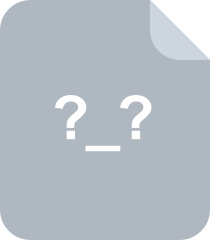
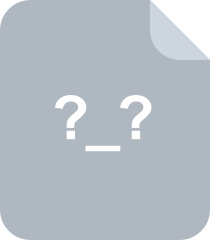
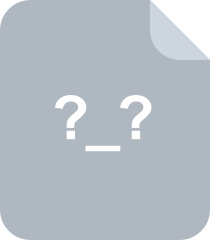
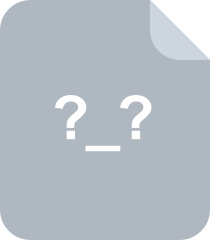
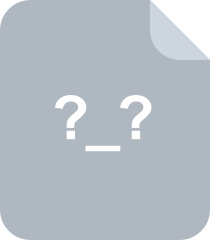
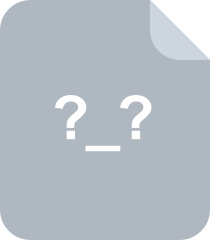
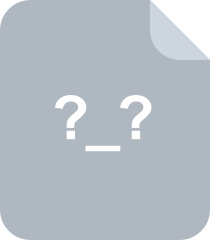
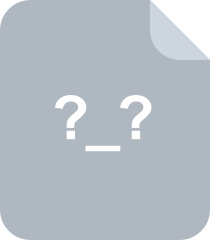
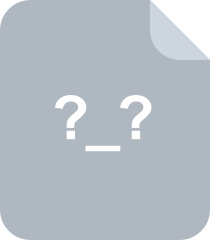
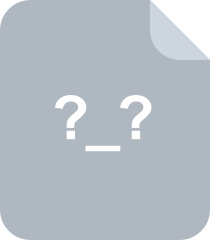
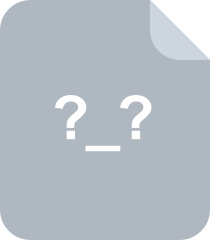
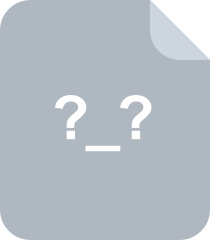
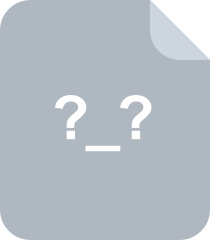
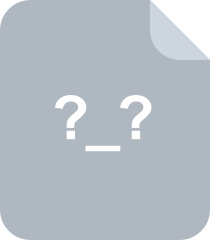
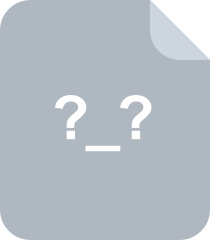
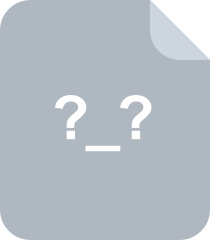
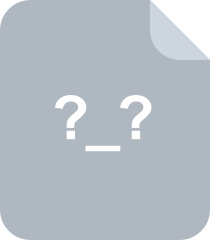
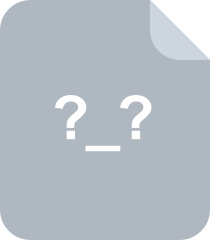
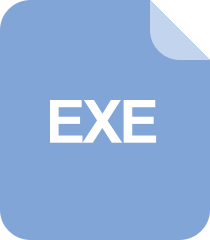
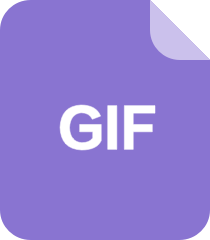
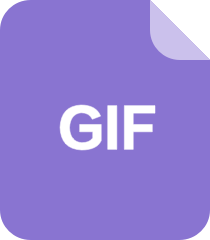
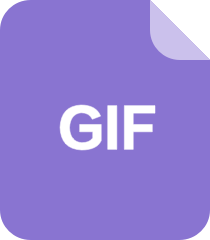
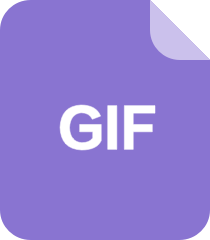
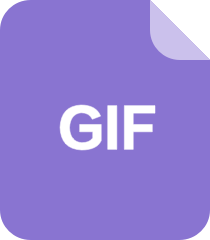
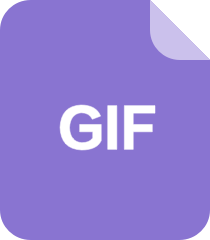
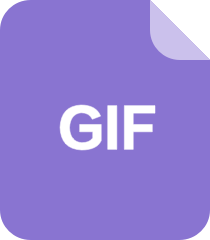
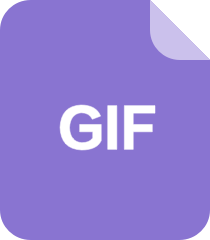
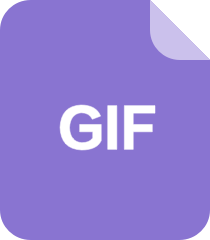
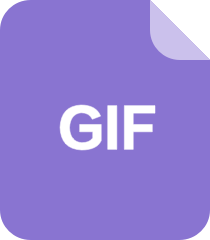
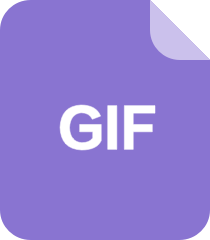
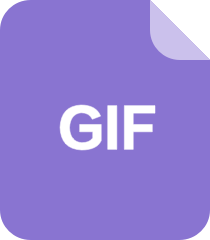
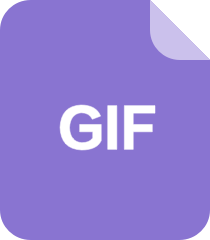
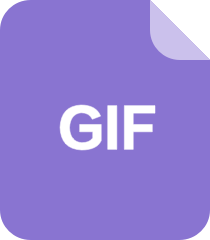
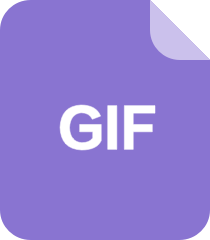
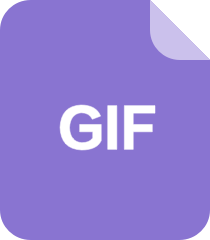
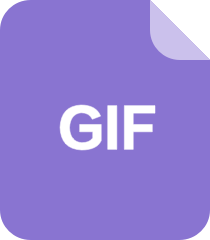
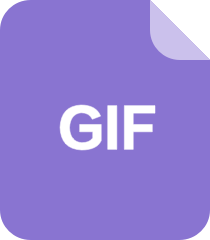
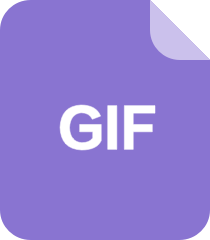
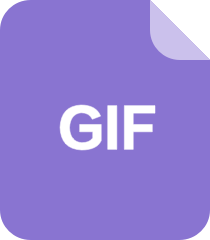
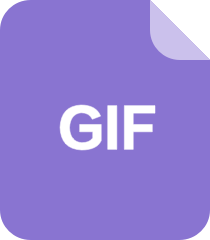
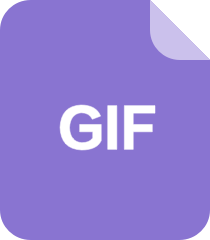
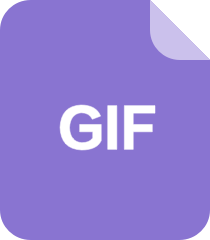
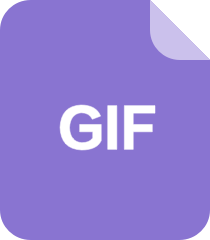
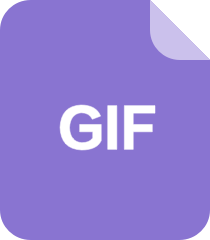
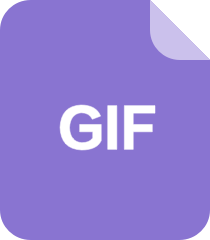
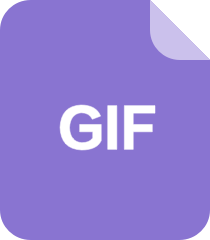
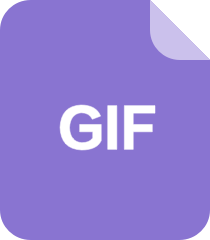
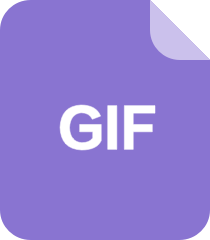
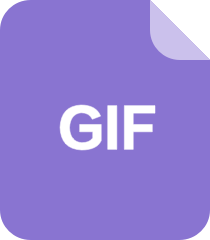
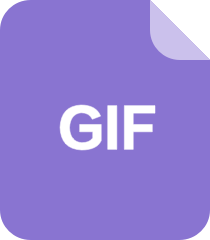
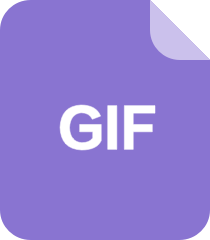
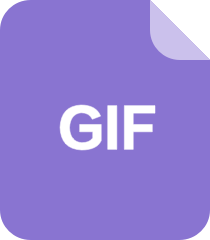
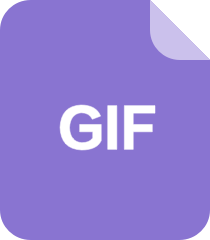
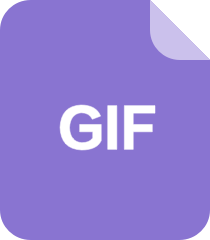
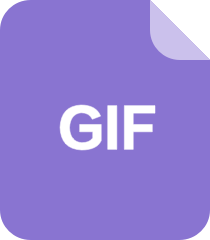
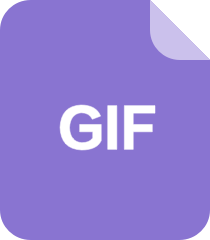
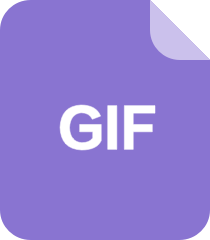
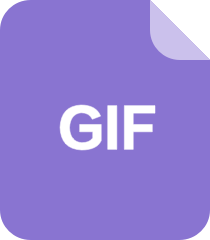
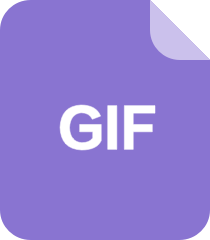
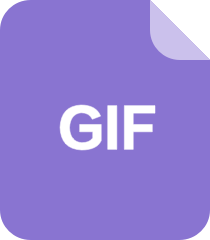
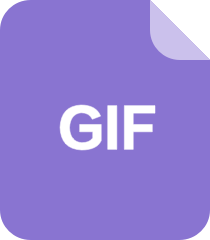
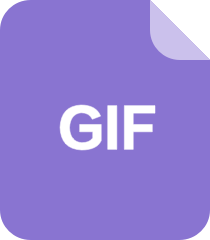
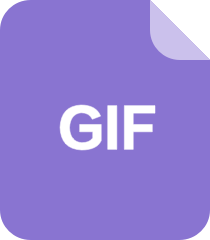
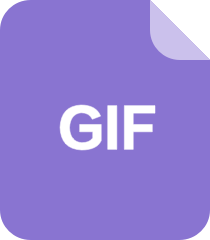
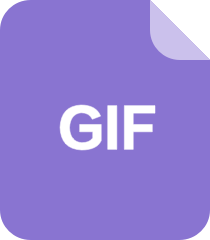
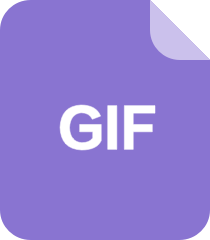
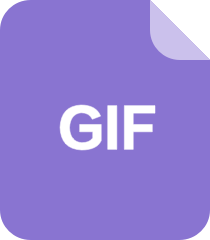
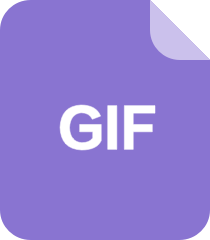
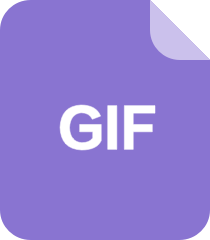
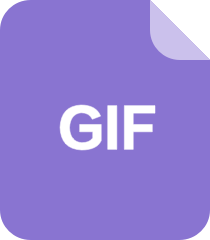
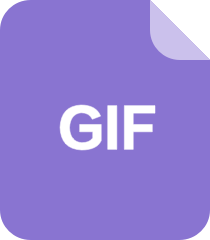
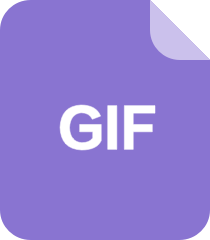
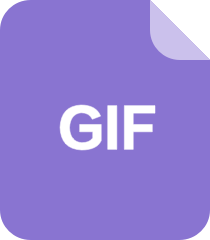
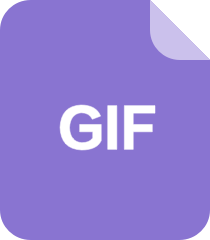
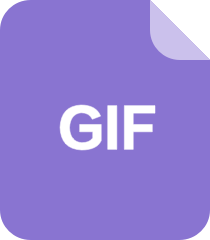
共 822 条
- 1
- 2
- 3
- 4
- 5
- 6
- 9
资源评论
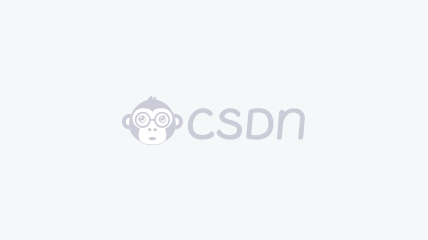

程序猿小D
- 粉丝: 4231
- 资源: 877
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

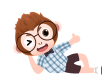
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


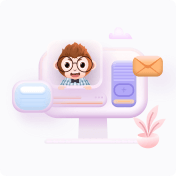
安全验证
文档复制为VIP权益,开通VIP直接复制
