package com.dao;
import com.db.DBHelper;
import com.bean.StudentBean;
import java.util.*;
import java.sql.*;
/**
* 学生DAO类
* @author
*
*/
public class StudentDao {
/**
* 验证登录
* @param username 用户名
* @param password 密码
* @return
*/
public String CheckLogin(String username, String password){
String id = null;
String sql="select * from Student where Student_Username='"+username+"' and Student_Password='"+password+"' and Student_State='入住'";
Statement stat = null;
ResultSet rs = null;
Connection conn = new DBHelper().getConn();
try{
stat = conn.createStatement();
rs = stat.executeQuery(sql);
while (rs.next()) {
id = rs.getString("Student_ID");
}
}
catch(SQLException ex){}
return id;
}
/**
* 验证密码
* @param id 学生编号
* @param password 密码
* @return
*/
public boolean CheckPassword(String id, String password){
boolean ps = false;
String sql="select * from Student where Student_ID='"+id+"' and Student_Password='"+password+"'";
Statement stat = null;
ResultSet rs = null;
Connection conn = new DBHelper().getConn();
try{
stat = conn.createStatement();
rs = stat.executeQuery(sql);
while (rs.next()) {
ps=true;
}
}
catch(SQLException ex){}
return ps;
}
/**
* 获取所有列表
* @param strwhere 条件
* @param strorder 排序字段
* @return
*/
public List<StudentBean> GetAllList(String strwhere,String strorder){
String sql="select * from Student";
if(!(isInvalid(strwhere)))
{
sql+=" where "+strwhere;
}
if(!(isInvalid(strorder)))
{
sql+=" order by "+strorder;
}
// System.out.println(sql);
Statement stat = null;
ResultSet rs = null;
Connection conn = new DBHelper().getConn();
List<StudentBean> list=new ArrayList<StudentBean>();
try{
stat = conn.createStatement();
rs = stat.executeQuery(sql);
while(rs.next()){
StudentBean cnbean=new StudentBean();
cnbean.setStudent_ID(rs.getInt("Student_ID"));
cnbean.setStudent_DomitoryID(rs.getInt("Student_DomitoryID"));
cnbean.setStudent_Username(rs.getString("Student_Username"));
cnbean.setStudent_Password(rs.getString("Student_Password"));
cnbean.setStudent_Name(rs.getString("Student_Name"));
cnbean.setStudent_Sex(rs.getString("Student_Sex"));
cnbean.setStudent_Class(rs.getString("Student_Class"));
cnbean.setStudent_State(rs.getString("Student_State"));
list.add(cnbean);
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (conn != null)
conn.close();
if (stat != null)
stat.close();
if (rs != null)
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return list;
}
/**
* 获取列表
* @param strwhere 条件
* @param strorder 排序字段
* @return
*/
public List<StudentBean> GetList(String strwhere,String strorder){
String sql="select * from Student,Domitory,Building where Student_DomitoryID=Domitory_ID and Domitory_BuildingID=Building_ID";
if(!(isInvalid(strwhere)))
{
sql+=" and "+strwhere;
}
if(!(isInvalid(strorder)))
{
sql+=" order by "+strorder;
}
// System.out.println(sql);
Statement stat = null;
ResultSet rs = null;
Connection conn = new DBHelper().getConn();
List<StudentBean> list=new ArrayList<StudentBean>();
try{
stat = conn.createStatement();
rs = stat.executeQuery(sql);
while(rs.next()){
StudentBean cnbean=new StudentBean();
cnbean.setStudent_ID(rs.getInt("Student_ID"));
cnbean.setStudent_DomitoryID(rs.getInt("Student_DomitoryID"));
cnbean.setStudent_Username(rs.getString("Student_Username"));
cnbean.setStudent_Password(rs.getString("Student_Password"));
cnbean.setStudent_Name(rs.getString("Student_Name"));
cnbean.setStudent_Sex(rs.getString("Student_Sex"));
cnbean.setStudent_Class(rs.getString("Student_Class"));
cnbean.setStudent_State(rs.getString("Student_State"));
cnbean.setDomitory_Name(rs.getString("Domitory_Name"));
cnbean.setBuilding_Name(rs.getString("Building_Name"));
cnbean.setDomitory_Type(rs.getString("Domitory_Type"));
cnbean.setDomitory_Number(rs.getString("Domitory_Number"));
cnbean.setDomitory_Tel(rs.getString("Domitory_Tel"));
list.add(cnbean);
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (conn != null)
conn.close();
if (stat != null)
stat.close();
if (rs != null)
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return list;
}
/**
* 获取指定ID的实体Bean
* @param strwhere 条件
* @return
*/
public StudentBean GetAllFirstBean(String strwhere){
String sql="select * from Student where "+strwhere;
Statement stat = null;
ResultSet rs = null;
Connection conn = new DBHelper().getConn();
StudentBean cnbean=new StudentBean();
try{
stat = conn.createStatement();
rs = stat.executeQuery(sql);
if(rs.next()){
cnbean.setStudent_ID(rs.getInt("Student_ID"));
cnbean.setStudent_DomitoryID(rs.getInt("Student_DomitoryID"));
cnbean.setStudent_Username(rs.getString("Student_Username"));
cnbean.setStudent_Password(rs.getString("Student_Password"));
cnbean.setStudent_Name(rs.getString("Student_Name"));
cnbean.setStudent_Sex(rs.getString("Student_Sex"));
cnbean.setStudent_Class(rs.getString("Student_Class"));
cnbean.setStudent_State(rs.getString("Student_State"));
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (conn != null)
conn.close();
if (stat != null)
stat.close();
if (rs != null)
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return cnbean;
}
/**
* 获取指定ID的实体Bean
* @param strwhere 条件
* @return
*/
public StudentBean GetFirstBean(String strwhere){
String sql="select * from Student,Domitory,Building where Student_DomitoryID=Domitory_ID and Domitory_BuildingID=Building_ID and "+strwhere;
Statement stat = null;
ResultSet rs = null;
Connection conn = new DBHelper().getConn();
StudentBean cnbean=new StudentBean();
try{
stat = conn.createStatement();
rs = stat.executeQuery(sql);
if(rs.next()){
cnbean.setStudent_ID(rs.getInt("Student_ID"));
cnbean.setStudent_DomitoryID(rs.getInt("Student_DomitoryID"));
cnbean.setStudent_Username(rs.getString("Student_Username"));
cnbean.setStudent_Password(rs.getString("Student_Password"));
cnbean.setStudent_Name(rs.getString("Student_Name"));
cnbean.setStudent_Sex(rs.getString("Student_Sex"));
cnbean.setStudent_Class(rs.getString("Student_Class"));
cnbean.setStudent_State(rs.getString("Student_State"));
cnbean.setDomitory_Name(rs.getString("Domitory_Name"));
cnbean.setBuilding_Name(rs.getString("Building_Name"));
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (conn != null)
conn.close();
if (stat != null)
stat.close();
if (rs != null)
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return cnbean;
}
/**
* 获取指定ID的实体Bean
* @param id 学生编号
* @return
*/
public StudentBean GetAllBean(int id){
String sql="select * from Student where Student_ID="+id;
Statement stat = null;
ResultSet rs = null;
Connection conn = new DBHelper().getConn();
StudentBean cnbean=new StudentBean();
try{
stat = conn.createStatement();
rs = stat.executeQuery(sql);
while(rs.next()){
cnbean.setStudent_ID(rs.getInt("Student_ID"));
cnbean.setStudent_DomitoryID(rs.getInt("Student_DomitoryID"));
cnbean.setStudent_Username(rs.getString("Student_Username"));
cnbean.setStudent_Password(rs.getString("Student_Passw
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
一、项目简介 本项目是一套基于JavaWeb+Jsp实现的校园宿舍管理系统,主要针对计算机相关专业的正在做bishe的学生和需要项目实战练习的Java学习者。 包含:项目源码、数据库脚本等,该项目可以直接作为bishe使用。 项目都经过严格调试,确保可以运行! 二、技术选择 JavaWeb,jsp,css,jquery,js Web服务器:Tomcat7及其以上版本 数据库服务器:Mysql5.0及以上 eclipse或者idea navicat 三、功能描述 后台首页 楼宇管理员管理 学生管理 楼宇管理 宿舍管理 学生入住登记 学生寝室调换 学生迁出登记 学生缺寝记录 迁出记录 修改密码 退出系统
资源推荐
资源详情
资源评论


























收起资源包目录

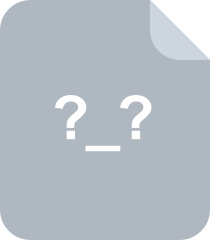
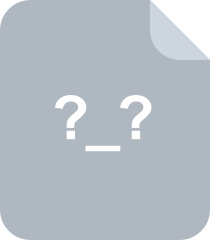
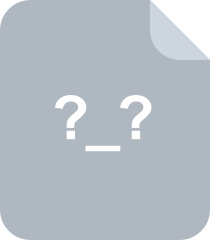
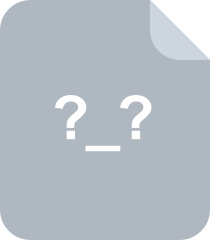
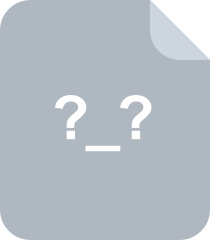
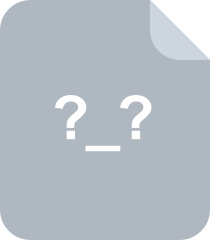
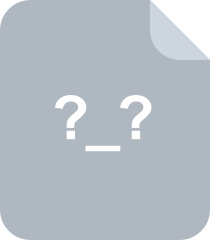
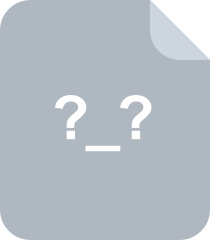
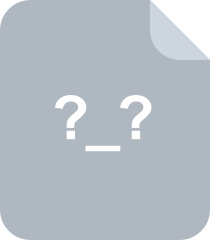
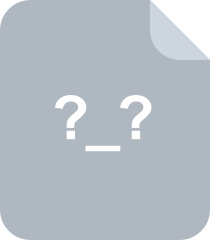
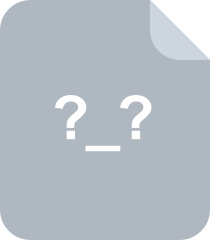
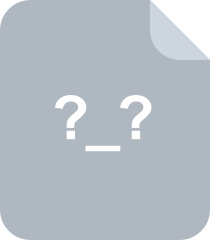
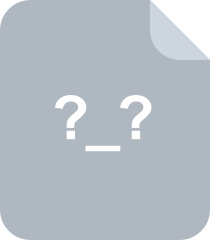
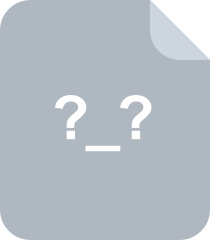
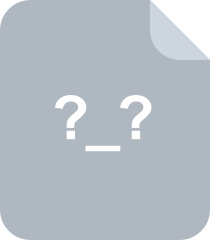
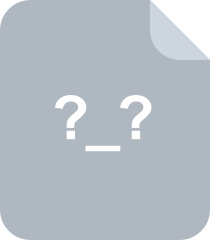
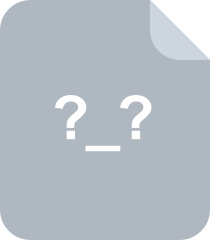
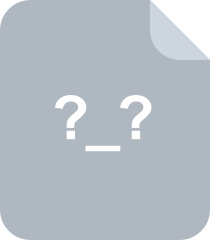
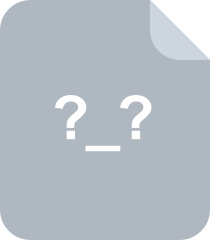
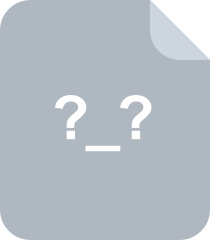
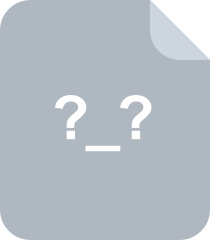
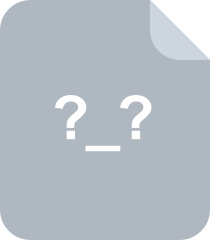
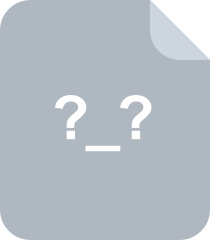
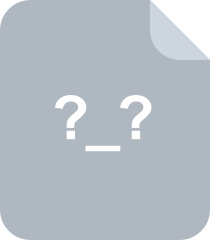
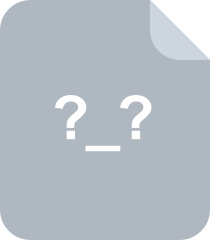
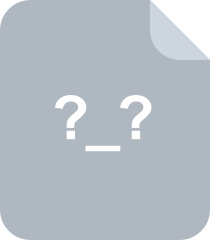
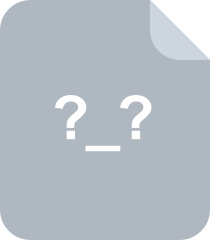
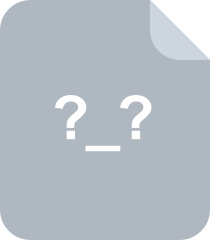
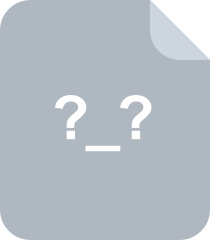
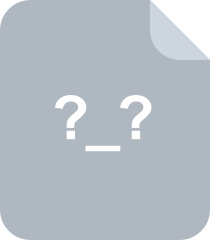
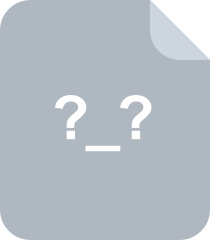
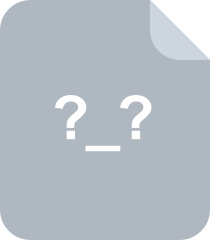
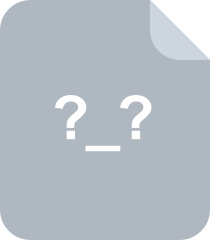
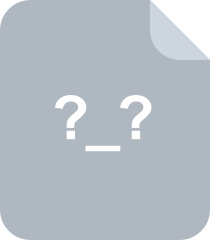
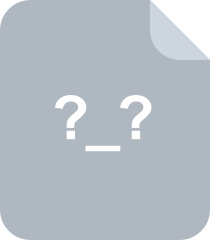
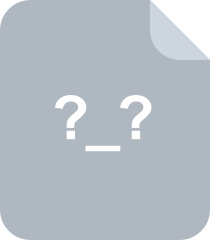
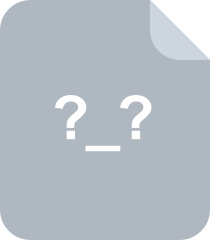
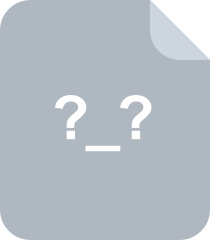
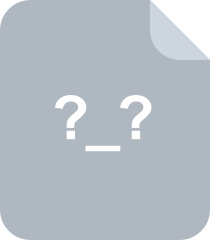
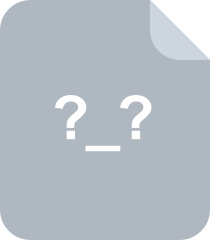
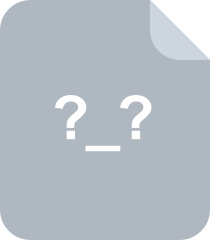
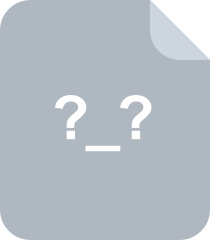
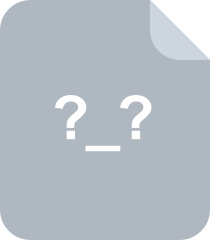
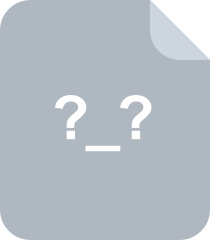
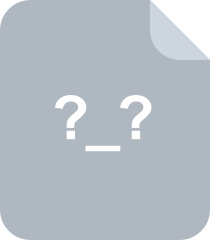
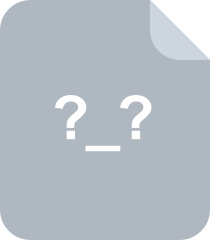
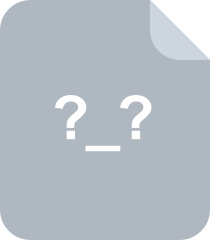
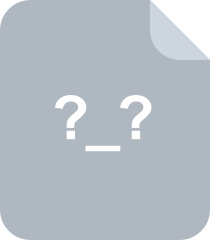
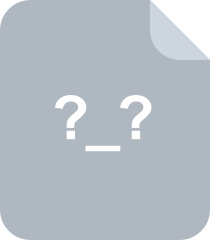
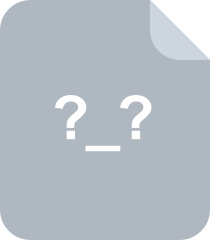
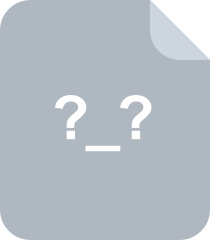
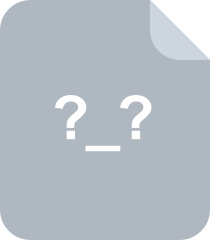
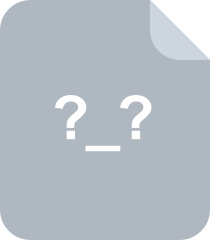
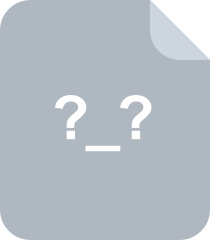
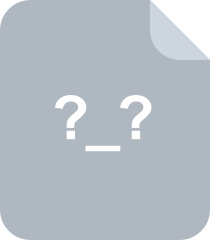
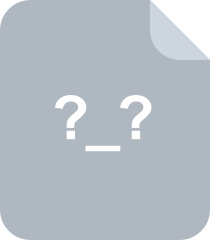
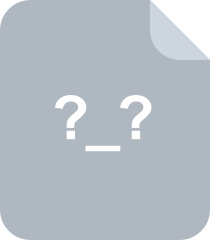
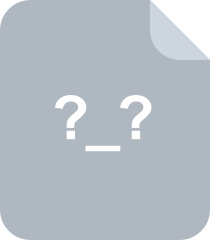
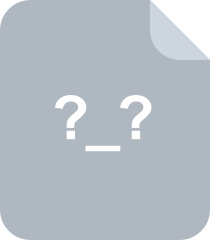
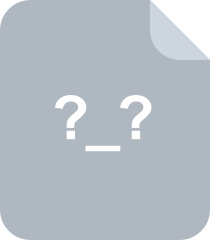
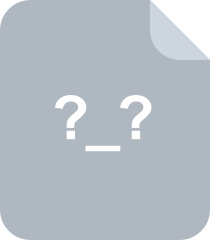
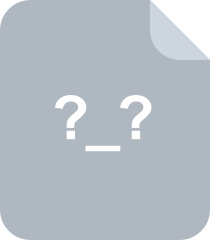
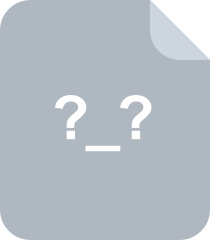
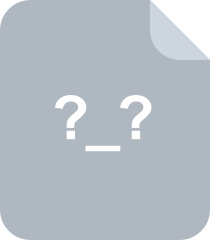
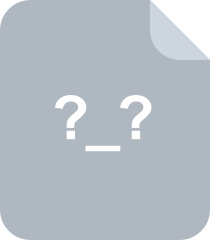
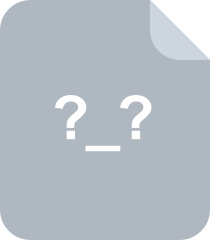
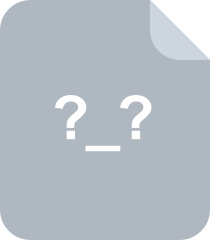
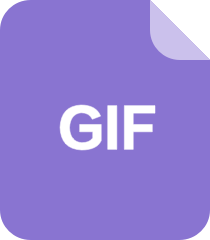
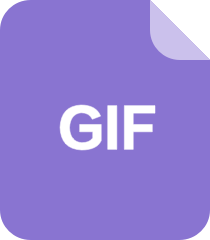
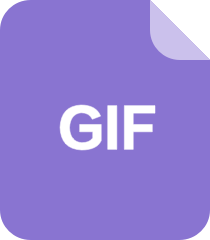
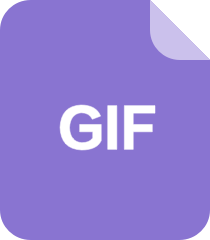
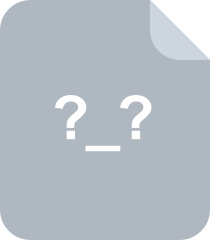
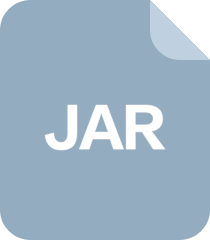
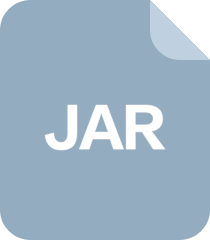
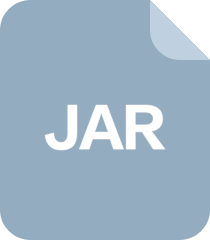
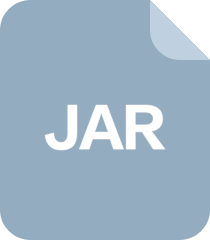
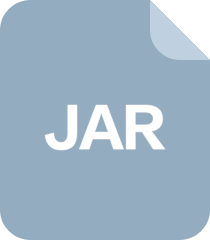
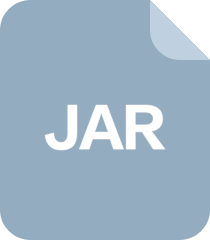
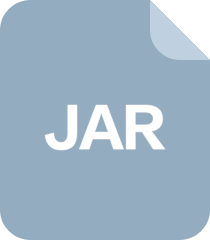
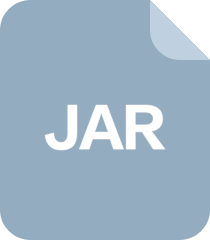
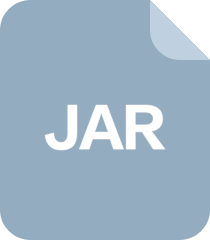
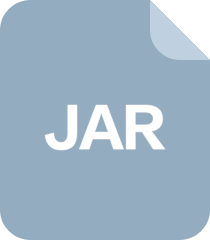
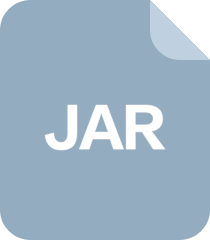
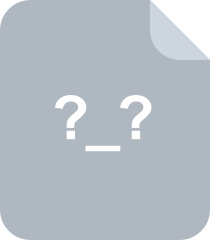
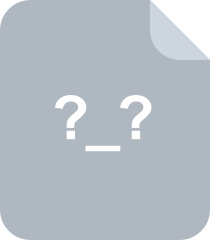
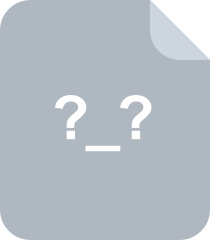
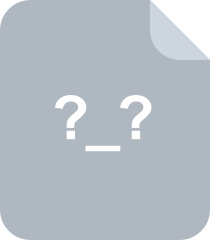
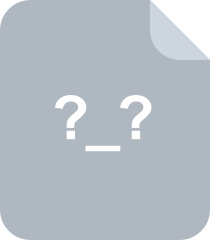
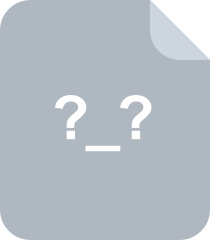
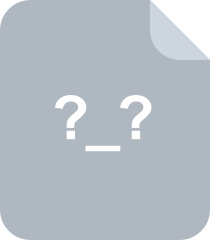
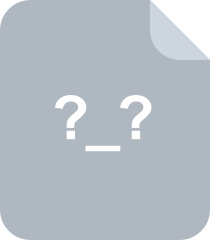
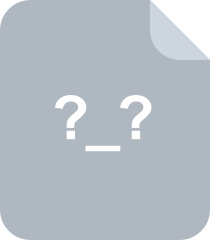
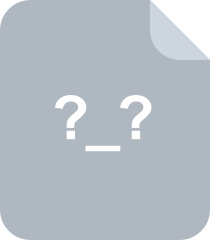
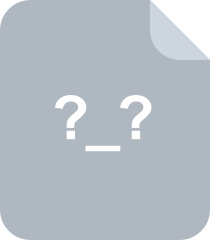
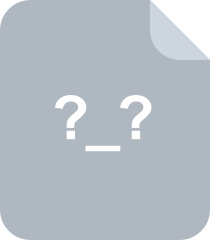
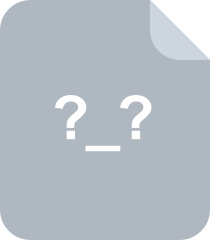
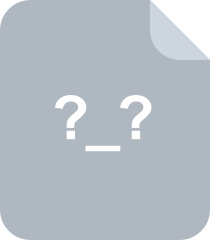
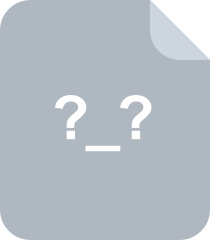
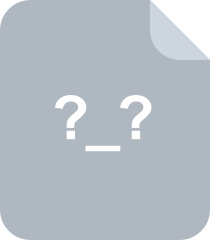
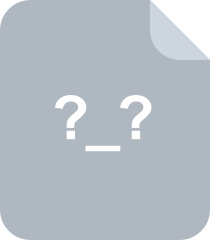
共 198 条
- 1
- 2
资源评论
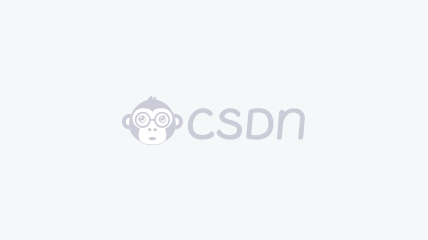

程序猿小D
- 粉丝: 4427
- 资源: 1584
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

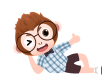
最新资源
- Auins-vmware虚拟机安装教程
- lilishop 商城 java商城-c语言
- fastpip-anaconda安装
- shopTNT电商系统-前端(PC端 商家PC端 管理端)-C语言资源
- minotes-android studio下载
- workflow-C++资源
- LinkWeChat-Java资源
- Goldfish Scheme-Python资源
- lilishop 商城 电商前端-c语言
- swift-Swift资源
- Java_Android_天气预报系统-android studio下载
- StockAnalysisSystem-anaconda安装
- gebi1-redpill-vmware虚拟机安装教程
- jvs-机器人开发资源
- qbbang-pycharm安装教程
- Kotlin-Kotlin资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


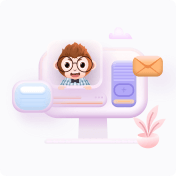
安全验证
文档复制为VIP权益,开通VIP直接复制
