package com.flower.web.admin;
import org.apache.commons.lang.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.PageRequest;
import org.springframework.data.domain.Pageable;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import com.flower.entity.Classification;
import com.flower.entity.Product;
import com.flower.entity.pojo.ResultBean;
import com.flower.service.ClassificationService;
import com.flower.service.ProductService;
import com.flower.utils.FileUtil;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.File;
import java.io.IOException;
import java.net.URLEncoder;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.Date;
import java.util.List;
import java.util.Map;
@Controller
@RequestMapping("/admin/product")
public class AdminProductController {
@Autowired
private ProductService productService;
@Autowired
private ClassificationService classificationService;
@RequestMapping("/toList.html")
public String toList() {
return "admin/product/list";
}
@RequestMapping("/toAdd.html")
public String toAdd() {
return "admin/product/add";
}
@RequestMapping("/toEdit.html")
public String toEdit(int id, Map<String, Object> map) {
Product product = productService.findById(id);
Classification classification = classificationService.findById(product.getCsid());
product.setCategorySec(classification);
map.put("product", product);
return "admin/product/edit";
}
@ResponseBody
@RequestMapping("/list.do")
public ResultBean<List<Product>> listProduct(int pageindex,
@RequestParam(value = "pageSize", defaultValue = "15") int pageSize) {
Pageable pageable = new PageRequest(pageindex, pageSize, null);
List<Product> list = productService.findAll(pageable).getContent();
return new ResultBean<>(list);
}
@ResponseBody
@RequestMapping("/getTotal")
public ResultBean<Integer> getTotal() {
Pageable pageable = new PageRequest(1, 15, null);
int total = (int) productService.findAll(pageable).getTotalElements();
return new ResultBean<>(total);
}
@RequestMapping("/del.do")
@ResponseBody
public ResultBean<Boolean> del(int id) {
productService.delById(id);
return new ResultBean<>(true);
}
@RequestMapping(method = RequestMethod.POST, value = "/add.do")
public void add(MultipartFile image,
String title,
Double marketPrice,
Double shopPrice,
int isHot,
String desc,
int csid,
HttpServletRequest request,
HttpServletResponse response) throws Exception {
Product product = new Product();
product.setTitle(title);
product.setMarketPrice(marketPrice);
product.setShopPrice(shopPrice);
product.setDesc(desc);
product.setIsHot(isHot);
product.setCsid(csid);
product.setPdate(new Date());
String imgUrl = FileUtil.saveFile(image);
product.setImage(imgUrl);
int id = productService.create(product);
if (id <= 0) {
request.setAttribute("message", "添加失败!");
request.getRequestDispatcher("toAdd.html").forward(request, response);
} else {
request.getRequestDispatcher("toEdit.html?id=" + id).forward(request, response);
}
}
@RequestMapping(method = RequestMethod.POST, value = "/update.do")
public void update(int id,
String title,
Double marketPrice,
Double shopPrice,
String desc,
int csid,
int isHot,
MultipartFile image,
HttpServletRequest request,
HttpServletResponse response) throws Exception {
Product product = productService.findById(id);
product.setTitle(title);
product.setMarketPrice(marketPrice);
product.setShopPrice(shopPrice);
product.setDesc(desc);
product.setIsHot(isHot);
product.setCsid(csid);
product.setPdate(new Date());
String imgUrl = FileUtil.saveFile(image);
if (StringUtils.isNotBlank(imgUrl)) {
product.setImage(imgUrl);
}
boolean flag = false;
try {
productService.update(product);
flag = true;
} catch (Exception e) {
throw new Exception(e);
}
if (!flag) {
request.setAttribute("message", "更新失败!");
}
response.sendRedirect("toList.html");
}
@RequestMapping(method = RequestMethod.GET, value = "/img/{filename:.+}")
public void getImage(@PathVariable(name = "filename", required = true) String filename,
HttpServletResponse res) throws IOException {
File file = new File("file/" + filename);
if (file != null && file.exists()) {
res.setHeader("content-type", "application/octet-stream");
res.setHeader("Content-Disposition", "attachment;filename=" + URLEncoder.encode(filename, "UTF-8"));
res.setContentLengthLong(file.length());
Files.copy(Paths.get(file.toURI()), res.getOutputStream());
}
}
}

程序猿小D
- 粉丝: 4241
- 资源: 918
最新资源
- 【创新未发表】基于鲸鱼优化算法WOA-ELM实现北半球光伏数据预测附Matlab代码.rar
- 【创新未发表】基于鲸鱼优化算法WOA-ESN实现北半球光伏数据预测附Matlab代码.rar
- 【创新未发表】基于开普勒优化算法KOA-ELM实现北半球光伏数据预测附Matlab代码.rar
- 【创新未发表】基于开普勒优化算法KOA-CNN实现交通量预测附Matlab代码.rar
- 【创新未发表】基于开普勒优化算法KOA-ESN实现北半球光伏数据预测附Matlab代码.rar
- 【python毕业设计】基于时间序列分析的大气污染预测软件(django)源码(完整前后端+mysql+说明文档+LW).zip
- Delphi 12 控件fmxlinux1.78.exe
- 【python毕业设计】网上购物商城(vue)源码(完整前后端+mysql+说明文档+LW).zip
- 【创新未发表】基于粒子群优化算法PSO-ESN实现北半球光伏数据预测附Matlab代码.rar
- 【创新未发表】基于粒子群优化算法PSO-ELM实现北半球光伏数据预测附Matlab代码.rar
- 【创新未发表】基于粒子群优化算法PSO-CNN实现交通量预测附Matlab代码.rar
- 【创新未发表】基于凌日优化算法TSOA-ELM实现北半球光伏数据预测附Matlab代码.rar
- 【创新未发表】基于凌日优化算法TSOA-ESN实现北半球光伏数据预测附Matlab代码.rar
- 【创新未发表】基于凌日优化算法TSOA-CNN实现交通量预测附Matlab代码.rar
- 【创新未发表】基于龙格库塔优化算法RUN-ELM实现北半球光伏数据预测附Matlab代码.rar
- 【创新未发表】基于龙格库塔优化算法RUN-CNN实现交通量预测附Matlab代码.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


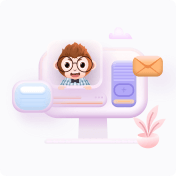