package servlet;
import java.io.IOException;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import dao.*;
import mysql.MysqlUtil;
import pojo.DorInfo;
/**
* Servlet implementation class Search
*/
@WebServlet("/SearchServlet")
public class SearchServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public SearchServlet() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse
* response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
/*
* response.getWriter().append("Served at: ").append(request.getContextPath());
*/
request.setCharacterEncoding("UTF-8");
String name = request.getParameter("name");
String value = request.getParameter("value");
String selected = request.getParameter("selected");
String table = request.getParameter("table");
HttpSession session = request.getSession();
if (table.equals("dor_info")) {
if (selected.equals("完整查询")) {
if (value == null || value.equals("")) {
response.setContentType("text/html;charset=utf-8");
response.getWriter().write(
"<script>alert('值不能为空!');window.location.href='../adminLeft/adminLeftFare.jsp';</script>");
} else {
List dorInfoList = DorInfoDao.readOneList2(name, value);
if (null != dorInfoList && dorInfoList.size() != 0) {
session.setAttribute("dorInfo", dorInfoList);
request.getRequestDispatcher("/adminLeft/adminLeftDorInfo.jsp").forward(request, response);
} else {
List dorInfoList2 = new ArrayList();
dorInfoList2 = DorInfoDao.readList();
session.setAttribute("dorInfo", dorInfoList2);
response.setContentType("text/html;charset=utf-8");
response.getWriter().write(
"<script>alert('查无此结果!');window.location.href='../adminLeft/adminLeftDorInfo.jsp';</script>");
}
}
} else if (selected.equals("模糊查询")) {
if (value == null || value.equals("")) {
response.setContentType("text/html;charset=utf-8");
response.getWriter().write(
"<script>alert('值不能为空!');window.location.href='../adminLeft/adminLeftFare.jsp';</script>");
} else {
List<DorInfo> dorInfoList = DorInfoDao.readOneList2(name, value);
if (null != dorInfoList && dorInfoList.size() != 0) {
session.removeAttribute("dorInfo");
session.setAttribute("dorInfo", dorInfoList);
request.getRequestDispatcher("/adminLeft/adminLeftDorInfo.jsp").forward(request, response);
} else {
List dorInfoList2 = new ArrayList();
dorInfoList2 = DorInfoDao.readList();
session.setAttribute("dorInfo", dorInfoList2);
response.setContentType("text/html;charset=utf-8");
response.getWriter().write(
"<script>alert('查无此结果!');window.location.href='../adminLeft/adminLeftDorInfo.jsp';</script>");
}
}
}
}
if (table.equals("dor_admin")) {
if (selected.equals("完整查询")) {
if (value == null || value.equals("")) {
response.setContentType("text/html;charset=utf-8");
response.getWriter().write(
"<script>alert('值不能为空!');window.location.href='../adminLeft/adminLeftFare.jsp';</script>");
} else {
/*
* System.out.println(name); System.out.println(value);
*/
List dorAdminInfoList = DorAdminInfoDao.readOneList(name, value);
if (null != dorAdminInfoList && dorAdminInfoList.size() != 0) {
session.setAttribute("dorAdminInfo", dorAdminInfoList);
request.getRequestDispatcher("/adminLeft/adminLeftDorAdmin.jsp").forward(request, response);
} else {
List dorAdminInfoList2 = new ArrayList();
dorAdminInfoList2 = DorAdminInfoDao.readList();
session.removeAttribute("dorAdminInfo");
session.setAttribute("dorAdminInfo", dorAdminInfoList2);
response.setContentType("text/html;charset=utf-8");
response.getWriter().write(
"<script>alert('查无此结果!');window.location.href='../adminLeft/adminLeftDorAdmin.jsp';</script>");
}
}
} else if (selected.equals("模糊查询")) {
if (value == null || value.equals("")) {
response.setContentType("text/html;charset=utf-8");
response.getWriter().write(
"<script>alert('值不能为空!');window.location.href='../adminLeft/adminLeftFare.jsp';</script>");
} else {
/*
* System.out.println(name); System.out.println(value);
*/
List dorAdminInfoList = DorAdminInfoDao.readOneList2(name, value);
if (null != dorAdminInfoList && dorAdminInfoList.size() != 0) {
session.setAttribute("dorAdminInfo", dorAdminInfoList);
request.getRequestDispatcher("/adminLeft/adminLeftDorAdmin.jsp").forward(request, response);
} else {
List dorAdminInfoList2 = new ArrayList();
dorAdminInfoList2 = DorAdminInfoDao.readList();
session.removeAttribute("dorAdminInfo");
session.setAttribute("dorAdminInfo", dorAdminInfoList2);
response.setContentType("text/html;charset=utf-8");
response.getWriter().write(
"<script>alert('查无此结果!');window.location.href='../adminLeft/adminLeftDorAdmin.jsp';</script>");
}
}
}
}
if (table.equals("stu_info")) {
if (selected.equals("完整查询")) {
if (value == null || value.equals("")) {
response.setContentType("text/html;charset=utf-8");
response.getWriter().write(
"<script>alert('值不能为空!');window.location.href='../adminLeft/adminLeftFare.jsp';</script>");
} else {
List studentInfoList = StudentInfoDao.readOneList(name, value);
if (null != studentInfoList && studentInfoList.size() != 0) {
session.setAttribute("stuInfo", studentInfoList);
request.getRequestDispatcher("/adminLeft/adminLeftStu.jsp").forward(request, response);
} else {
/*
* System.out.println(name); System.out.println(value);
*/
List studentInfoList2 = new ArrayList();
studentInfoList2 = StudentInfoDao.readList();
session.setAttribute("stuInfo", studentInfoList2);
response.setContentType("text/html;charset=utf-8");
response.getWriter().write(
"<script>alert('查无此结果!');window.location.href='../adminLeft/adminLeftStu.jsp';</script>");
}
}
} else if (selected.equals("模糊查询")) {
if (value == null || value.equals("")) {
response.setContentType("text/html;charset=utf-8");
response.getWriter().write(
"<script>alert('值不能为空!');window.location.href='../adminLeft/adminLeftFare.jsp';</script>");
} else {
List studentInfoList = StudentInfoDao.readOneList2(name, value);
if (null != studentInfoList && studentInfoList.size() != 0) {
session.removeAttribute("stuInfo");
session.setAttribute("stuInfo", studentInfoList);
request.getRequestDispatcher("/adminLeft/adminLeftStu.jsp").forward(request, response);
} else {
/*
* System.out.println(name); System.out.println(value);
*/
List studentInfoList2 = new ArrayList();
studentInfoList2 = StudentInfoDao.readList();
session.setAttribute("stuInfo", studentInfoList2);
response.setContentType("text/html;charset=utf-8");
response.getWriter().write(
"<script>alert('查无此结果!');window.location.href='../adminLeft/adminLeftStu.jsp';</script>");
}
}
}
}
if (table.equals("water_and_electricity")) {
System.out.printl
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
一、项目简介 本项目是一套基于servlet学生宿舍管理系统,主要针对计算机相关专业的正在做bishe的学生和需要项目实战练习的Java学习者。 包含:项目源码、数据库脚本等,该项目可以直接作为bishe使用。 项目都经过严格调试,确保可以运行! 二、技术实现 使用框架:javaWeb,servlet 前端技术:css,js,jquery,jsp 开发工具:IDEA/MyEclipse/Eclipse 数据库:MySQL 5.7/8.0 数据库工具:Navicat/sqlyong/phpstudy JDK版本:jdk1.8 二、功能介绍 系统分为三种角色:管理员,宿舍管理员,学生 功能包括: 宿管信息:宿管账号、所管理宿舍栋号、联系电话 学生管理信息:学号、宿舍号、姓名、性别、所在系、所在班级等 宿舍信息:宿舍号、宿舍号码、可住人数、已住人数 水电费缴纳信息:包括宿舍号、日期、用水量、水费、用电量、电费等 宿舍水电费缴纳情况:饼图展示 详见 https://blog.csdn.net/weixin_43860634/article/details/129201057
资源推荐
资源详情
资源评论
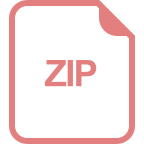
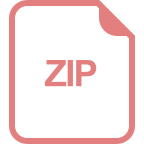
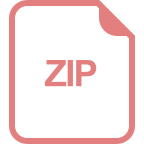
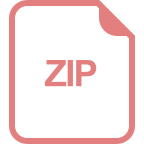
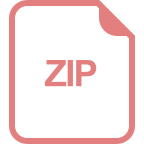
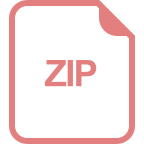
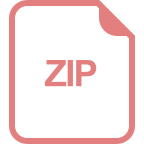
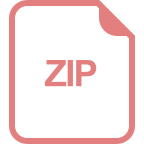
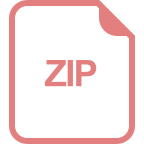
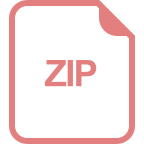
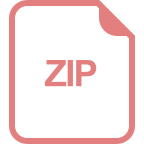
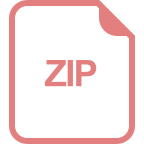
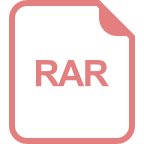
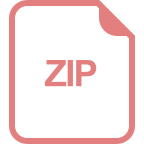
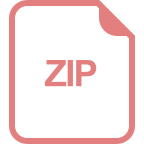
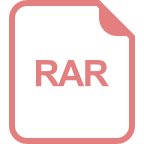
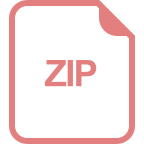
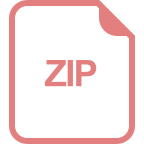
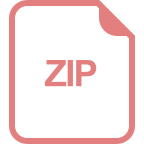
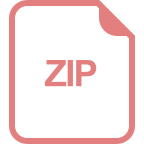
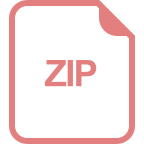
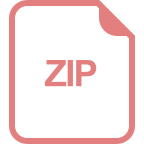
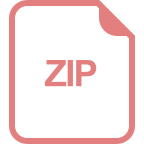
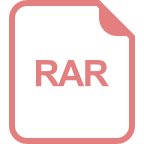
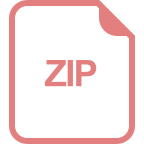
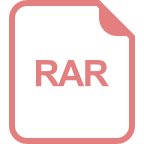
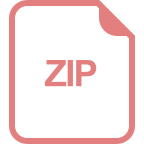
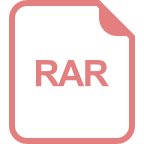
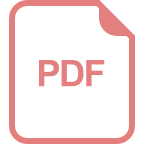
收起资源包目录

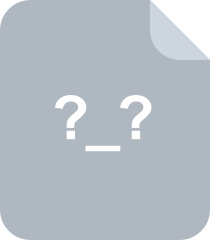
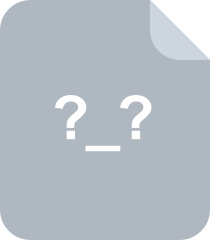
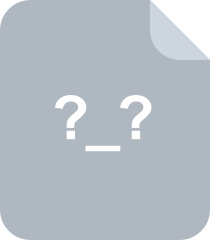
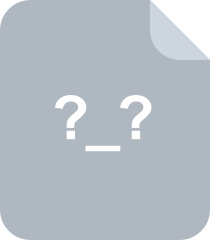
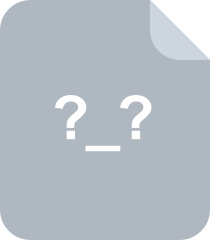
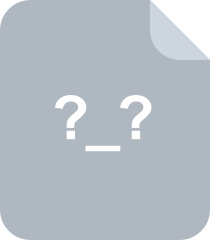
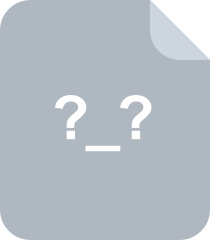
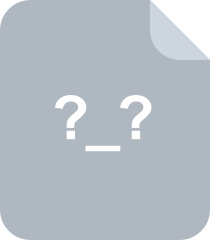
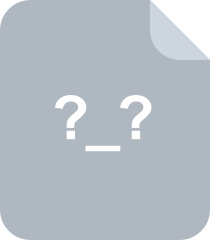
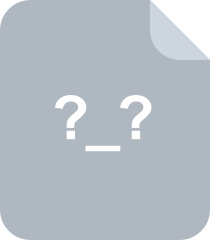
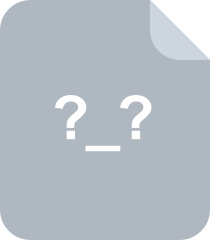
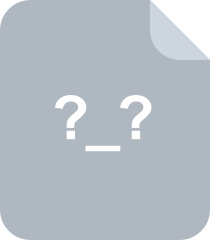
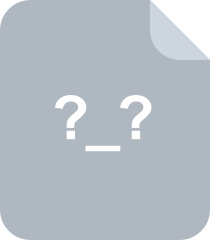
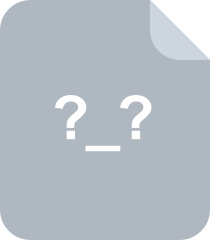
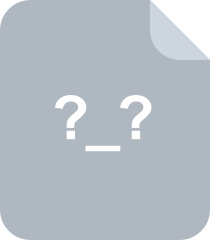
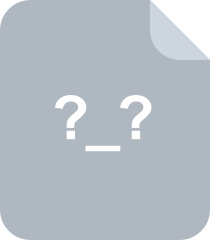
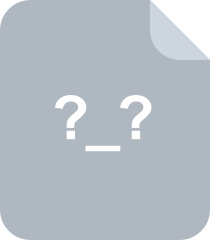
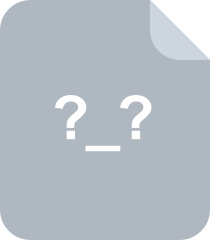
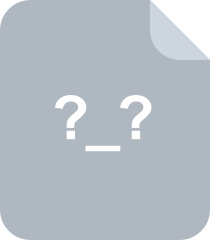
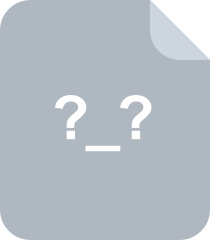
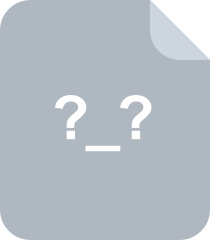
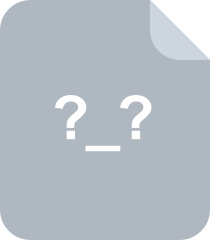
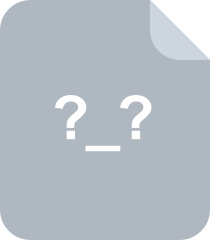
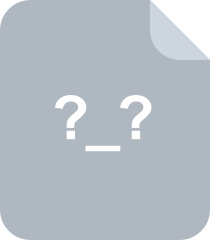
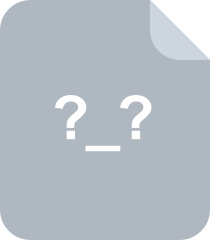
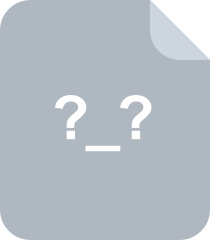
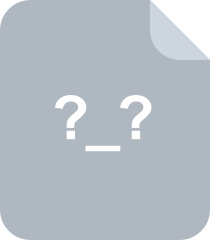
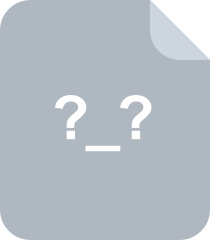
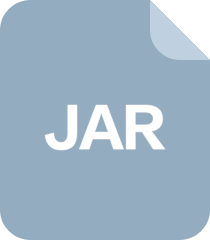
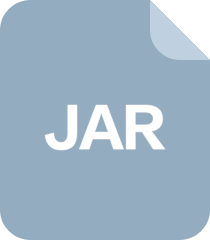
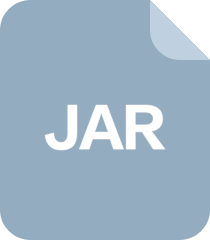
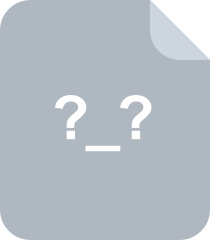
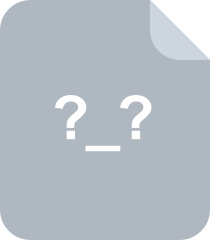
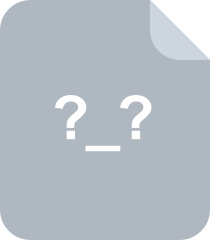
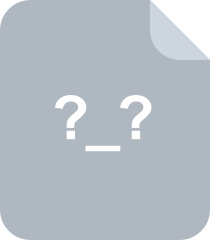
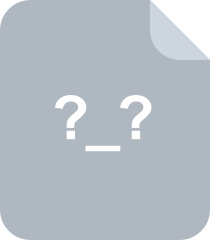
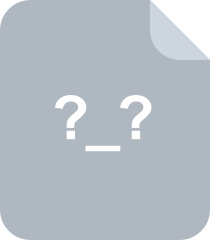
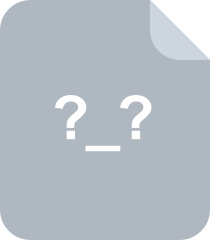
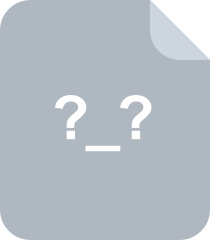
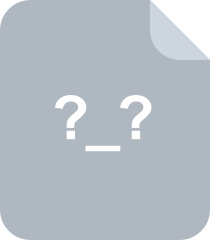
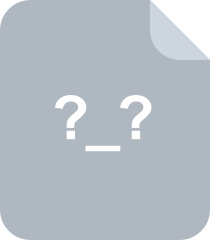
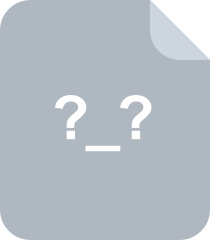
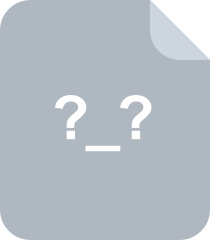
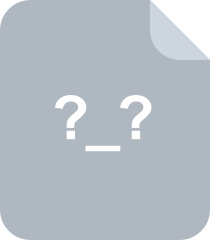
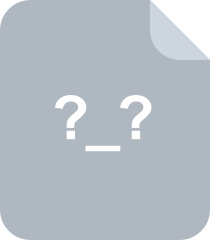
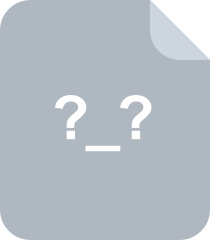
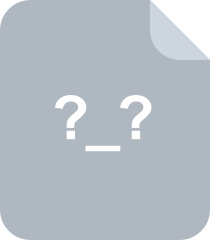
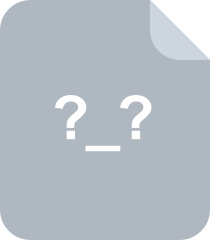
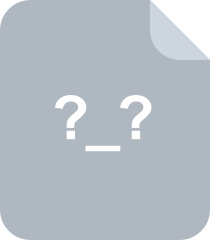
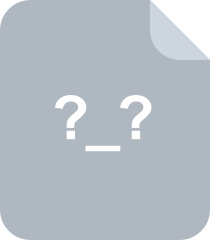
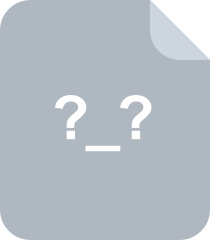
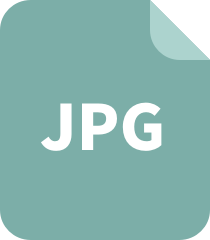
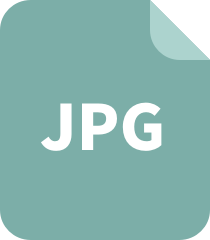
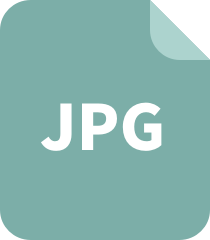
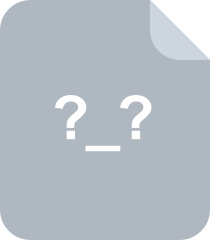
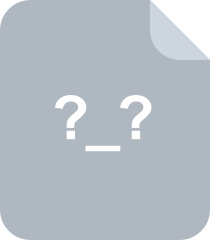
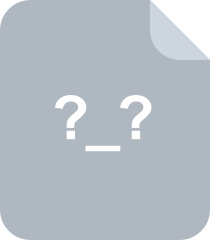
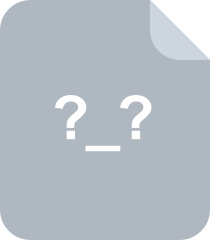
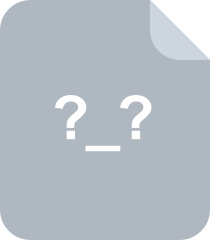
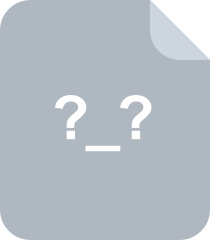
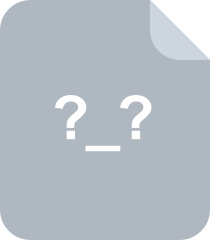
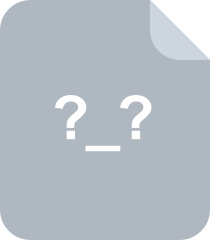
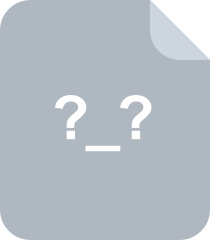
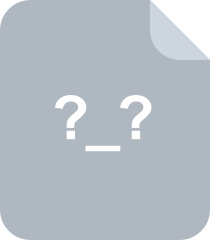
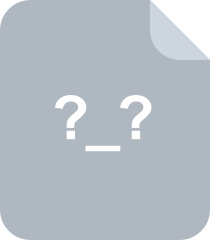
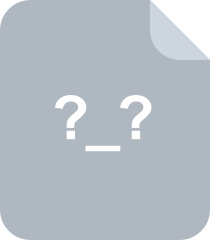
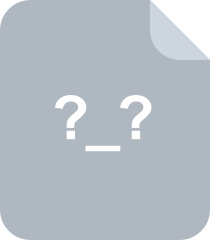
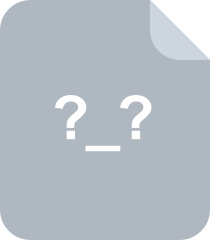
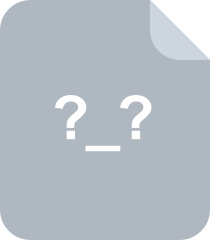
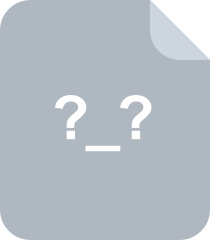
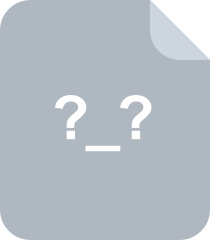
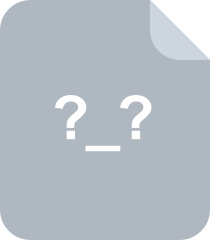
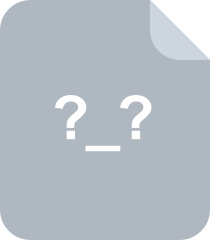
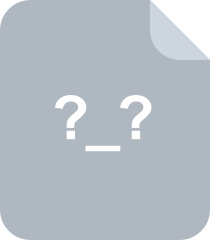
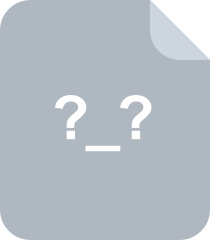
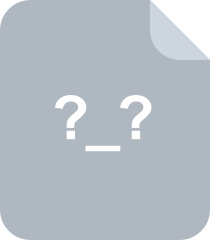
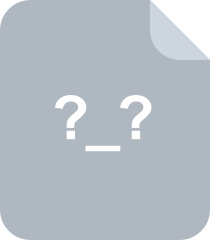
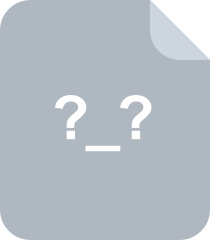
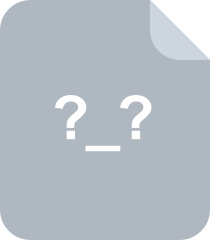
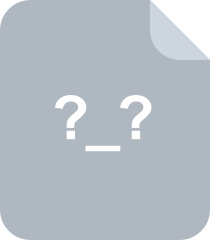
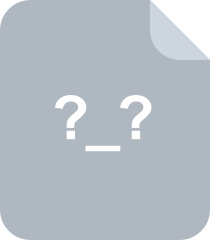
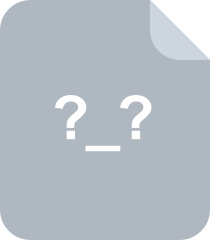
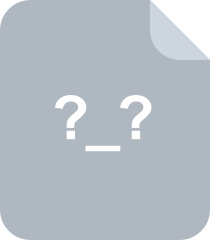
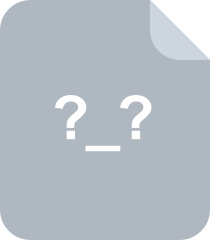
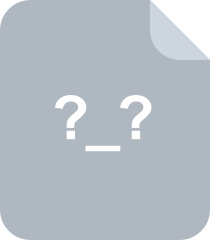
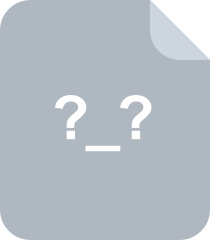
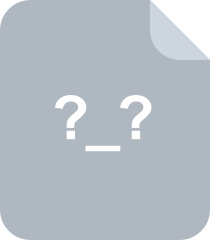
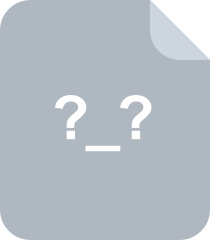
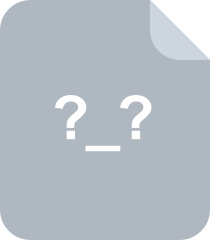
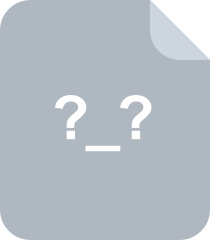
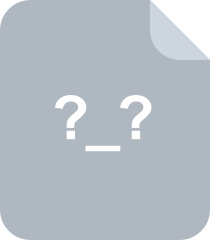
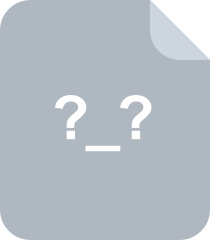
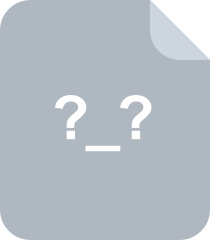
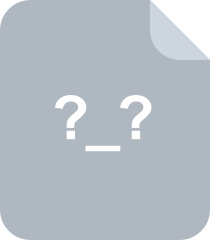
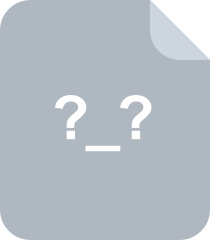
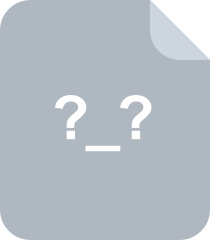
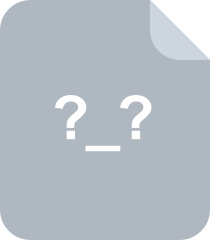
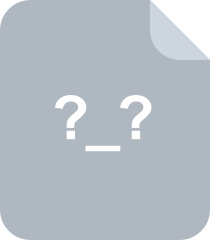
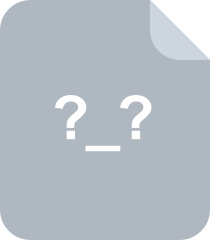
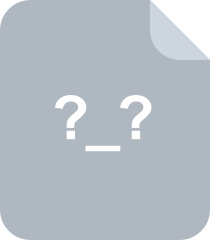
共 134 条
- 1
- 2
资源评论
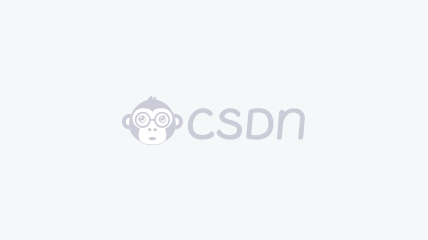

程序猿小D
- 粉丝: 3918
- 资源: 386
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

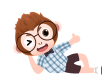
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


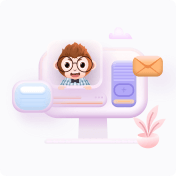
安全验证
文档复制为VIP权益,开通VIP直接复制
