package com.xz.spider.bilibili.util;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import org.apache.http.HttpEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.dom4j.DocumentException;
import org.dom4j.io.SAXReader;
import org.jsoup.Connection;
import org.jsoup.Connection.Response;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import com.alibaba.fastjson.JSONObject;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.mysql.jdbc.StandardSocketFactory;
import com.sun.tools.doclets.internal.toolkit.util.DocFinder.Output;
import com.xz.spider.bilibili.vo.Archive;
import com.xz.spider.bilibili.vo.UPer;
public class ConnectUtil {
public static Document getDocument(String url){
System.out.println(url);
Connection connection = Jsoup.connect(url);
Document document = null;
try {
connection.header("User-Agent", "Mozilla/5.0 (compatible; MSIE 9.0; Windows NT 6.1; Trident/5.0; MALC)");
document = connection.ignoreContentType(true).timeout(10000).get();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return document;
}
public static Response getResponse(String url){
Connection connection = Jsoup.connect(url);
Response response = null;
try {
connection.header("User-Agent", "Mozilla/5.0 (compatible; MSIE 9.0; Windows NT 6.1; Trident/5.0; MALC)");
response = connection.ignoreContentType(true).timeout(10000).execute();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return response;
}
public static CloseableHttpResponse doGet(String href){
CloseableHttpClient closeableHttpClient = HttpClients.createDefault();
HttpGet httpGet = new HttpGet(href);
httpGet.setHeader("User-Agent", "Mozilla/5.0 (compatible; MSIE 9.0; Windows NT 6.1; Trident/5.0; MALC)");
try {
return closeableHttpClient.execute(httpGet);
} catch (IOException e) {
return doGet(href);
}
}
public static <T>T downLoadDanmu(String href,String aid, String path){
SAXReader saxReader=new SAXReader();
InputStreamReader in= null;
HttpEntity httpEntity=null;
try {
httpEntity = ConnectUtil.doGet(href).getEntity();
in = new InputStreamReader(httpEntity.getContent(),"utf-8");
if(href.contains("rolldate")) {
ObjectMapper objectMapper=new ObjectMapper();
JsonNode jsonNode=objectMapper.readTree(in);
return (T)jsonNode;
}else {
FileUtil.writeFile(aid+".xml", in, path);
org.dom4j.Element root = saxReader.read(in).getRootElement();
if(root.element("source")!=null&&root.element("source").getText().equals("e-r")){
return null;
}else{
return (T)root;
}
}
} catch (IOException e) {
} catch (DocumentException e) {
}finally {
// try {
// EntityUtils.consume(httpEntity);
// } catch (IOException e) {
// LogUtil.getLogger().error(String.valueOf(e));
// }
}
return null;
}
/**
* 抓取1.view(观看次数)、danmaku(总弹幕数)、reply(弹幕数)、his_rank(最高全站日排行)、favorite(收藏人数)、coin(硬币数)、share(分享数)
*/
public static Archive spiderArchive(String aid){
// Connection connection = Jsoup.connect();
// connection.header("(Request-Line)", "POST /cgi-bin/login?lang=zh_CN HTTP/1.1");
// connection.header("Accept", "application/json, text/javascript, */*; q=0.01");
// connection.header("Accept-Encoding", "gzip, deflate");
// connection.header("Accept-Language", "zh-cn");
// connection.header("Cache-Control", "no-cache");
// connection.header("Connection", "Keep-Alive");
// connection.header("Content-Type", "application/x-www-form-urlencoded; charset=UTF-8");
// connection.header("Host", "mp.weixin.qq.com");
// connection.header("Referer", "https://news.qq.com/");
// connection.header("User-Agent", "Mozilla/5.0 (compatible; MSIE 9.0; Windows NT 6.1; Trident/5.0; MALC)");
Response response = getResponse("https://api.bilibili.com/x/web-interface/archive/stat?callback=jQuery17208549602856317029_1502097590081&aid="+aid+"&jsonp=jsonp&_=1502097590829");
String json = regxJson(response.body());
//System.out.println(json);
JSONObject jsonObject = JSONObject.parseObject(json);
Archive archive = JSONObject.parseObject(jsonObject.get("data").toString(),Archive.class);
System.out.println(archive);
return archive;
}
/**
* 获取详情页
*/
public static Document getDetail(String url){
Document document = getDocument(url);
return document;
}
/**
* 提取cid
*/
public static String getCid(Document document){
Element e = document.body();
String cid = null;
Elements es = e.select("script");
for (int i = 0; i < es.size(); i++) {
String a = es.html();
if (!a.contains("EmbedPlayer(")) {
continue;
}
cid = a.substring(a.indexOf("cid=")+4, a.indexOf("&aid"));
}
//System.out.println(cid);
return cid;
}
/**
* 根据cid获取弹幕页面
*/
public static void getBarrage(String cid, String aid, String path) throws IOException{
ConnectUtil.downLoadDanmu("https://comment.bilibili.com/"+cid+".xml", aid, path);
}
//将一个字符串中的json剥离
public static String regxJson(String exJson){
String json = null;
if(exJson.indexOf("({")>-1){
json = exJson.substring(exJson.indexOf("(")+1,exJson.lastIndexOf(")"));
}else{
json = exJson;
}
return json;
}
/**
* 获取mid
*/
public static String getMid(Document document){
String mid = null;
mid = document.select(".upinfo>.u-face>a").attr("mid");
return mid;
}
/**
* 获取up主信息
* @param mid
* @return
*/
public static UPer getUPer(String mid){
Response response = getResponse(ApiUrl.UPer.getUrl(mid));
String json = response.body();
System.out.println(json);
JSONObject obj = JSONObject.parseObject(json);
UPer up = null;
if(obj.containsKey("data")){
System.out.println(obj.get("data").toString());
JSONObject obj1 = JSONObject.parseObject(obj.get("data").toString());
up = JSONObject.parseObject(obj1.get("card").toString(),UPer.class);
}
return up;
}
/**
* 获取回复数
* @param mid
* @return
*/
public static String getReply(String oid){
Response response = getResponse(ApiUrl.Reply.getUrl(oid));
String json = response.body();
System.out.println(json);
JSONObject obj = JSONObject.parseObject(json);
String reply = null;
UPer up = null;
if(obj.containsKey("data")){
JSONObject obj1 = JSONObject.parseObject(obj.get("data").toString());
JSONObject obj2 = JSONObject.parseObject(obj1.get("page").toString());
reply = obj2.get("acount").toString();
}
return reply;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
基于springboot+mybatis的java网络爬虫,一期爬取bilibili站点的一些视频基本信息+源代码+文档说明
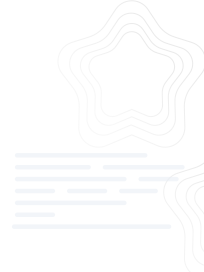
共26个文件
java:14个
xml:4个
prefs:3个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 30 浏览量
2023-11-28
16:05:27
上传
评论
收藏 23KB ZIP 举报
温馨提示
- ## 项目备注 1、该资源内项目代码都经过测试运行成功,功能ok的情况下才上传的,请放心下载使用! 2、本项目适合计算机相关专业(如计科、人工智能、通信工程、自动化、电子信息等)的在校学生、老师或者企业员工下载学习,也适合小白学习进阶,当然也可作为毕设项目、课程设计、作业、项目初期立项演示等。 3、如果基础还行,也可在此代码基础上进行修改,以实现其他功能,也可用于毕设、课设、作业等。 下载后请首先打开README.md文件(如有),仅供学习参考, 切勿用于商业用途。
资源推荐
资源详情
资源评论
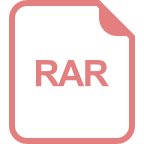
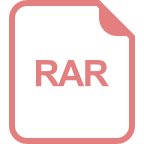
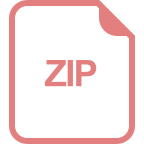
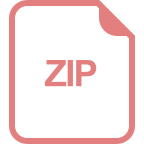
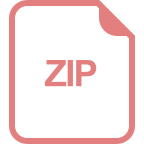
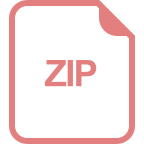
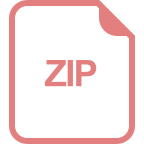
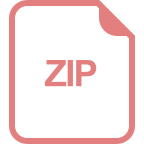
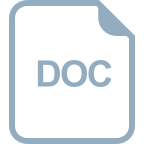
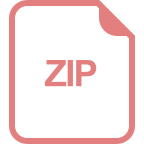
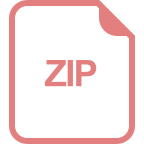
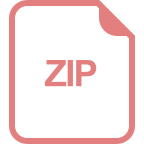
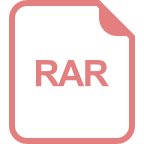
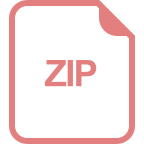
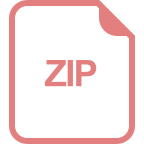
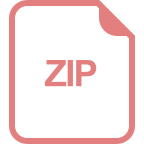
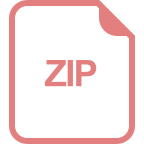
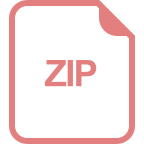
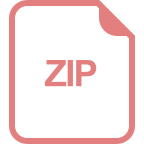
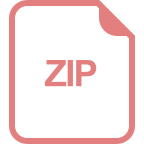
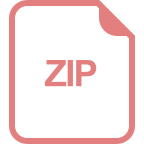
收起资源包目录


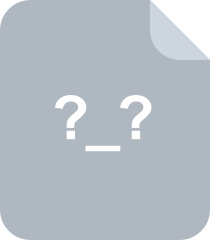

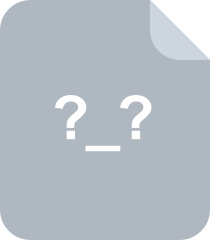
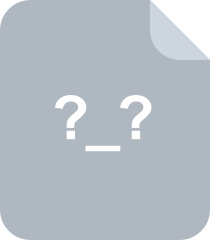
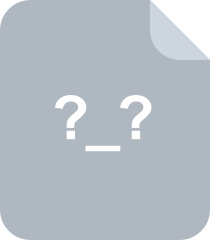
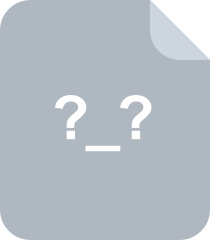



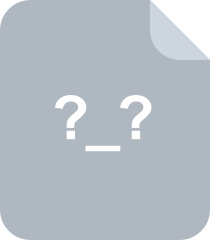

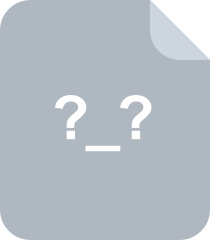
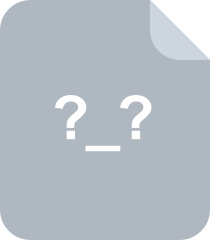
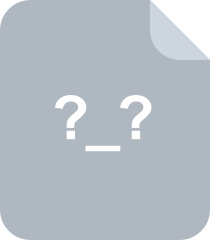
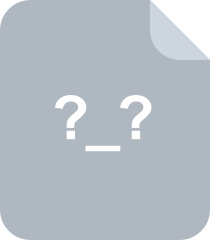
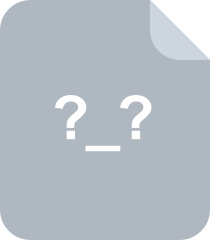





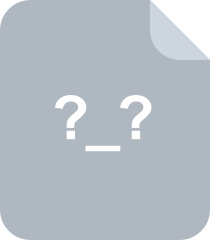

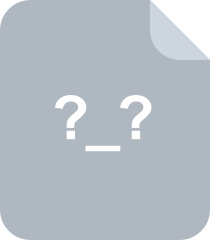
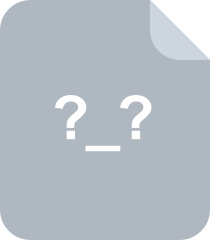

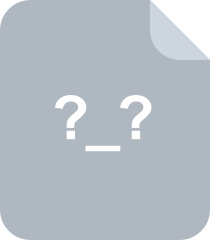
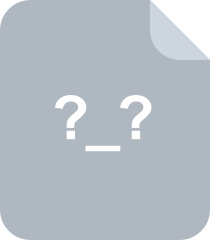

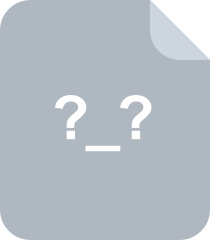
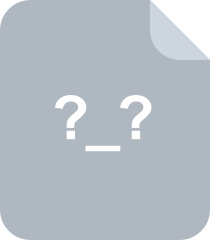

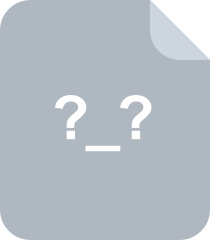
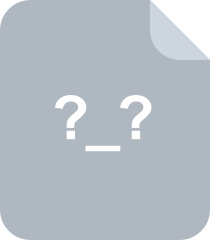
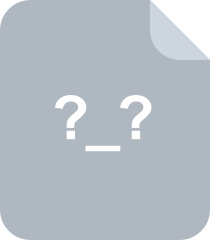
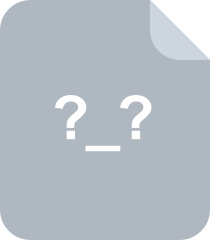
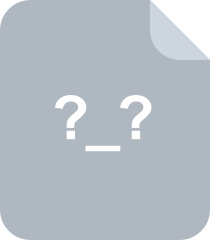

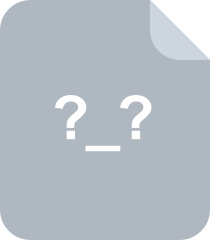
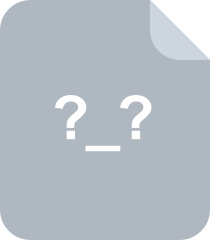
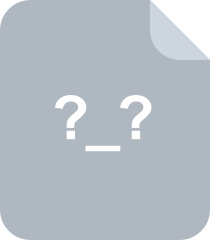
共 26 条
- 1
资源评论
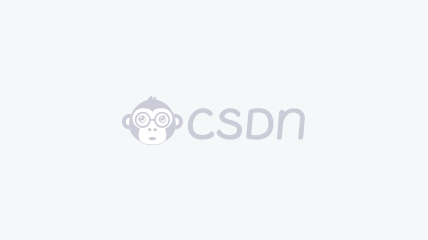

奋斗奋斗再奋斗的ajie
- 粉丝: 1216
- 资源: 2590

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

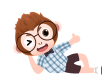
最新资源
- 白色简洁风格的房产交易中心企业网站源码下载.zip
- 白色简洁风格的发型形象设计整站网站源码下载.zip
- 白色简洁风格的风光摄影相册源码下载.zip
- 白色简洁风格的分类摄影图库源码下载.zip
- 白色简洁风格的风力发电网站模板下载.zip
- 白色简洁风格的服装商品网店整站网站源码下载.zip
- 白色简洁风格的服装电商整站网站源码下载.zip
- 白色简洁风格的高端婚礼定制网页模板下载.zip
- 白色简洁风格的服装鞋包品牌商城网站源码下载.zip
- 白色简洁风格的服装设计师企业网站模板下载.zip
- 白色简洁风格的高端家私家具企业网站源码下载.zip
- 白色简洁风格的高端西服定制商城网站模板.zip
- 白色简洁风格的高端汽车预订企业网站源码下载.zip
- 白色简洁风格的高尔夫运动网站模板下载.zip
- 白色简洁风格的高级西餐牛排模板下载.zip
- 白色简洁风格的高科技产品HTML5网站模板.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


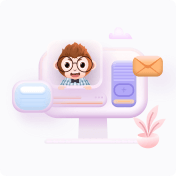
安全验证
文档复制为VIP权益,开通VIP直接复制
