package com.controller;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Calendar;
import java.util.Map;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Date;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import com.utils.ValidatorUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.baomidou.mybatisplus.mapper.Wrapper;
import com.annotation.IgnoreAuth;
import com.entity.YonghuEntity;
import com.entity.view.YonghuView;
import com.service.YonghuService;
import com.service.TokenService;
import com.utils.PageUtils;
import com.utils.R;
import com.utils.MD5Util;
import com.utils.MPUtil;
import com.utils.CommonUtil;
/**
* 用户
* 后端接口
* @author
* @email
* @date 2021-02-26 20:12:43
*/
@RestController
@RequestMapping("/yonghu")
public class YonghuController {
@Autowired
private YonghuService yonghuService;
@Autowired
private TokenService tokenService;
/**
* 登录
*/
@IgnoreAuth
@RequestMapping(value = "/login")
public R login(String username, String password, String captcha, HttpServletRequest request) {
YonghuEntity user = yonghuService.selectOne(new EntityWrapper<YonghuEntity>().eq("zhanghao", username));
if(user==null || !user.getMima().equals(password)) {
return R.error("账号或密码不正确");
}
String token = tokenService.generateToken(user.getId(), username,"yonghu", "用户" );
return R.ok().put("token", token);
}
/**
* 注册
*/
@IgnoreAuth
@RequestMapping("/register")
public R register(@RequestBody YonghuEntity yonghu){
//ValidatorUtils.validateEntity(yonghu);
YonghuEntity user = yonghuService.selectOne(new EntityWrapper<YonghuEntity>().eq("zhanghao", yonghu.getZhanghao()));
if(user!=null) {
return R.error("注册用户已存在");
}
Long uId = new Date().getTime();
yonghu.setId(uId);
yonghuService.insert(yonghu);
return R.ok();
}
/**
* 退出
*/
@RequestMapping("/logout")
public R logout(HttpServletRequest request) {
request.getSession().invalidate();
return R.ok("退出成功");
}
/**
* 获取用户的session用户信息
*/
@RequestMapping("/session")
public R getCurrUser(HttpServletRequest request){
Long id = (Long)request.getSession().getAttribute("userId");
YonghuEntity user = yonghuService.selectById(id);
return R.ok().put("data", user);
}
/**
* 密码重置
*/
@IgnoreAuth
@RequestMapping(value = "/resetPass")
public R resetPass(String username, HttpServletRequest request){
YonghuEntity user = yonghuService.selectOne(new EntityWrapper<YonghuEntity>().eq("zhanghao", username));
if(user==null) {
return R.error("账号不存在");
}
user.setMima("123456");
yonghuService.updateById(user);
return R.ok("密码已重置为:123456");
}
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,YonghuEntity yonghu, HttpServletRequest request){
EntityWrapper<YonghuEntity> ew = new EntityWrapper<YonghuEntity>();
PageUtils page = yonghuService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, yonghu), params), params));
request.setAttribute("data", page);
return R.ok().put("data", page);
}
/**
* 前端列表
*/
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,YonghuEntity yonghu, HttpServletRequest request){
EntityWrapper<YonghuEntity> ew = new EntityWrapper<YonghuEntity>();
PageUtils page = yonghuService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, yonghu), params), params));
request.setAttribute("data", page);
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/lists")
public R list( YonghuEntity yonghu){
EntityWrapper<YonghuEntity> ew = new EntityWrapper<YonghuEntity>();
ew.allEq(MPUtil.allEQMapPre( yonghu, "yonghu"));
return R.ok().put("data", yonghuService.selectListView(ew));
}
/**
* 查询
*/
@RequestMapping("/query")
public R query(YonghuEntity yonghu){
EntityWrapper< YonghuEntity> ew = new EntityWrapper< YonghuEntity>();
ew.allEq(MPUtil.allEQMapPre( yonghu, "yonghu"));
YonghuView yonghuView = yonghuService.selectView(ew);
return R.ok("查询用户成功").put("data", yonghuView);
}
/**
* 后端详情
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") Long id){
YonghuEntity yonghu = yonghuService.selectById(id);
return R.ok().put("data", yonghu);
}
/**
* 前端详情
*/
@RequestMapping("/detail/{id}")
public R detail(@PathVariable("id") Long id){
YonghuEntity yonghu = yonghuService.selectById(id);
return R.ok().put("data", yonghu);
}
/**
* 后端保存
*/
@RequestMapping("/save")
public R save(@RequestBody YonghuEntity yonghu, HttpServletRequest request){
yonghu.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(yonghu);
YonghuEntity user = yonghuService.selectOne(new EntityWrapper<YonghuEntity>().eq("zhanghao", yonghu.getZhanghao()));
if(user!=null) {
return R.error("用户已存在");
}
yonghu.setId(new Date().getTime());
yonghuService.insert(yonghu);
return R.ok();
}
/**
* 前端保存
*/
@RequestMapping("/add")
public R add(@RequestBody YonghuEntity yonghu, HttpServletRequest request){
yonghu.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(yonghu);
YonghuEntity user = yonghuService.selectOne(new EntityWrapper<YonghuEntity>().eq("zhanghao", yonghu.getZhanghao()));
if(user!=null) {
return R.error("用户已存在");
}
yonghu.setId(new Date().getTime());
yonghuService.insert(yonghu);
return R.ok();
}
/**
* 修改
*/
@RequestMapping("/update")
public R update(@RequestBody YonghuEntity yonghu, HttpServletRequest request){
//ValidatorUtils.validateEntity(yonghu);
yonghuService.updateById(yonghu);//全部更新
return R.ok();
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@RequestBody Long[] ids){
yonghuService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
}
/**
* 提醒接口
*/
@RequestMapping("/remind/{columnName}/{type}")
public R remindCount(@PathVariable("columnName") String columnName, HttpServletRequest request,
@PathVariable("type") String type,@RequestParam Map<String, Object> map) {
map.put("column", columnName);
map.put("type", type);
if(type.equals("2")) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Calendar c = Calendar.getInstance();
Date remindStartDate = null;
Date remindEndDate = null;
if(map.get("remindstart")!=null) {
Integer remindStart = Integer.parseInt(map.get("remindstart").toString());
c.setTime(new Dat
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
源码是经过本地编译可运行的,下载完成之后配置相应环境即可使用。源码功能都是经过老师肯定的,都能满足要求,有需要放心下载即可。源码是经过本地编译可运行的,下载完成之后配置相应环境即可使用。源码功能都是经过老师肯定的,都能满足要求,有需要放心下载即可。源码是经过本地编译可运行的,下载完成之后配置相应环境即可使用。源码功能都是经过老师肯定的,都能满足要求,有需要放心下载即可。源码是经过本地编译可运行的,下载完成之后配置相应环境即可使用。源码功能都是经过老师肯定的,都能满足要求,有需要放心下载即可。源码是经过本地编译可运行的,下载完成之后配置相应环境即可使用。源码功能都是经过老师肯定的,都能满足要求,有需要放心下载即可。源码是经过本地编译可运行的,下载完成之后配置相应环境即可使用。源码功能都是经过老师肯定的,都能满足要求,有需要放心下载即可。源码是经过本地编译可运行的,下载完成之后配置相应环境即可使用。源码功能都是经过老师肯定的,都能满足要求,有需要放心下载即可。源码是经过本地编译可运行的,下载完成之后配置相应环境即可使用。源码功能都是经过老师肯定的,都能满足要求,有需要放心下载即可。源码是经
资源推荐
资源详情
资源评论
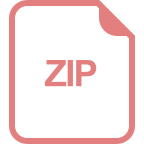
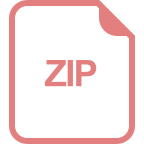
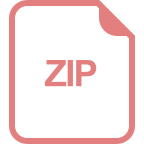
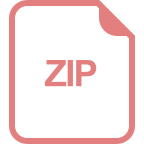
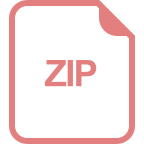
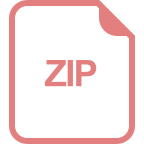
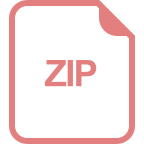
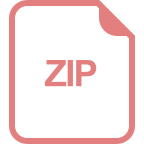
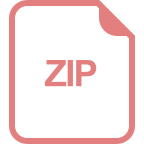
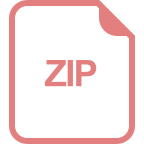
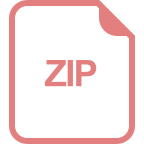
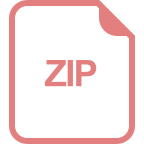
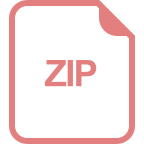
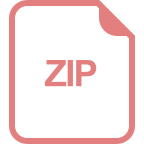
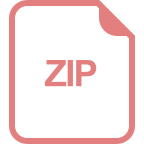
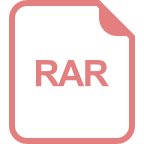
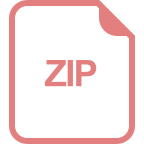
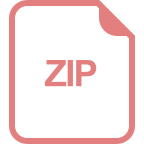
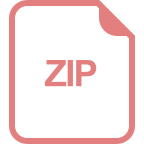
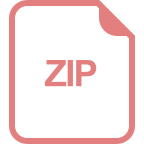
收起资源包目录

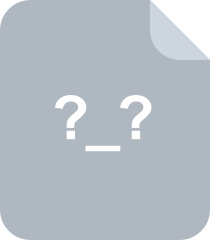
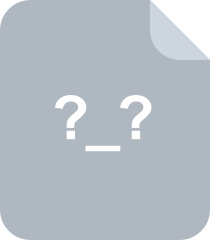
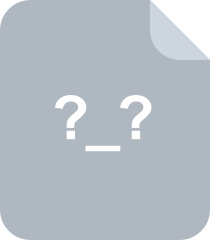
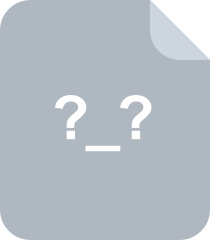
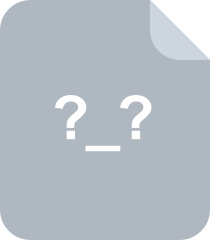
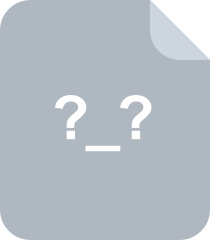
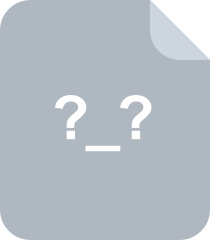
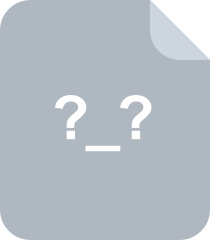
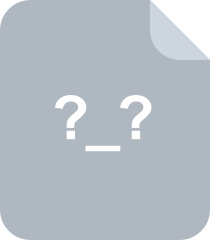
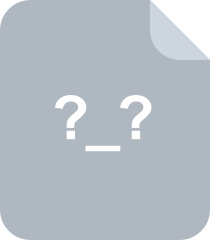
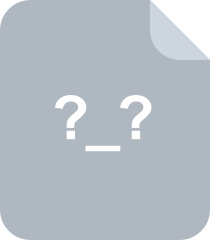
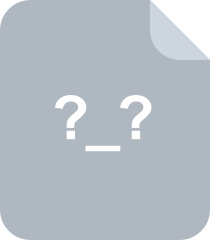
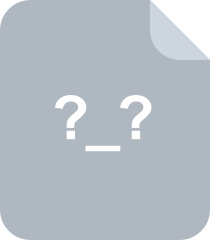
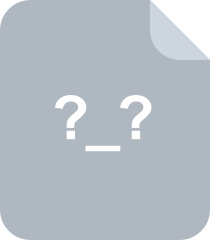
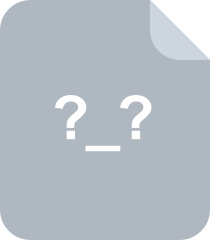
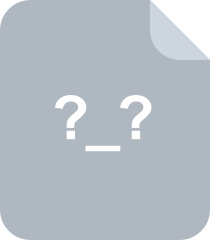
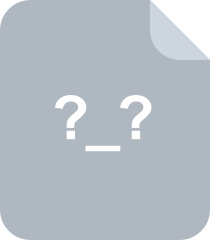
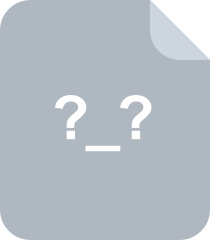
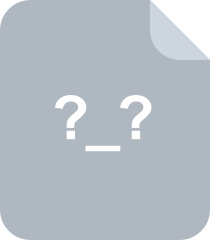
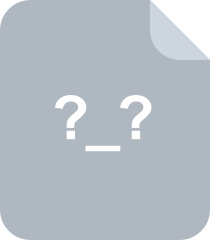
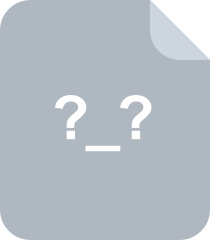
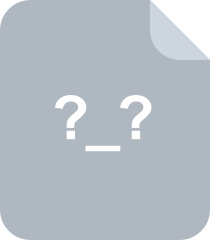
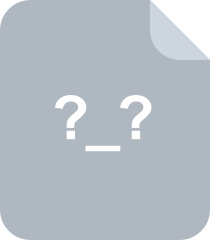
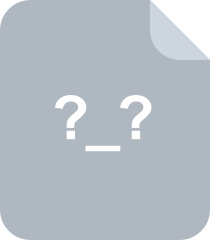
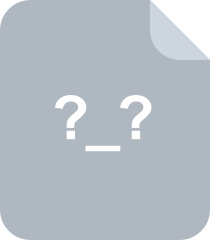
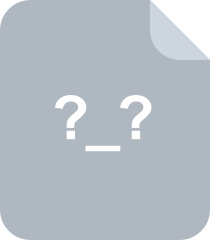
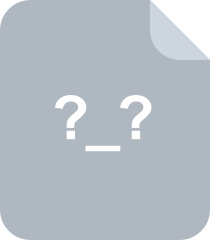
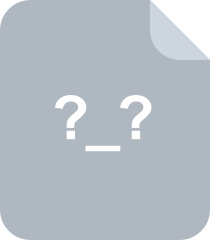
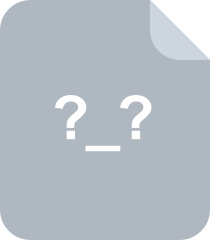
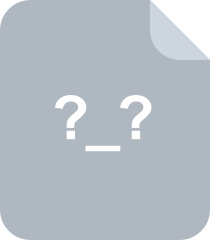
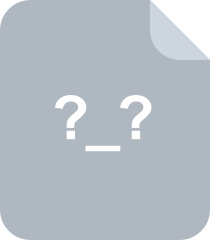
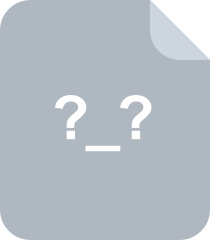
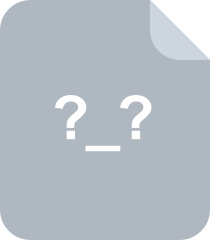
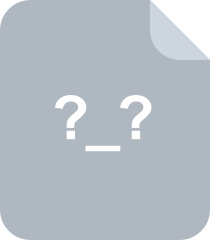
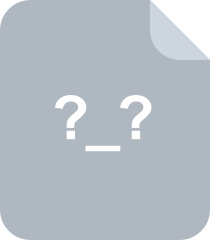
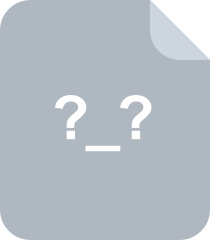
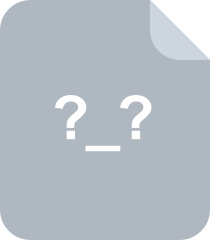
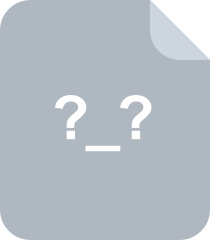
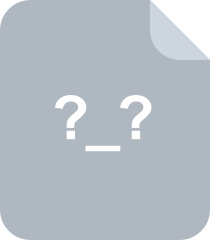
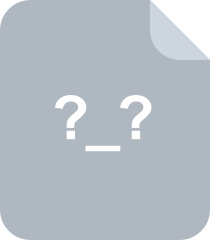
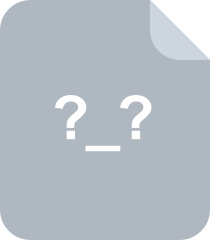
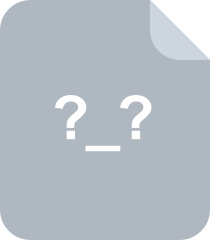
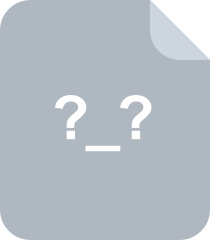
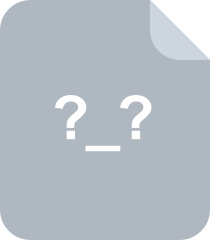
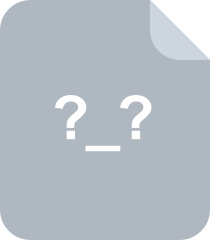
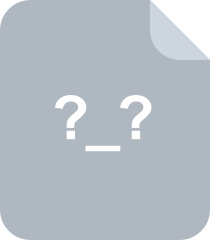
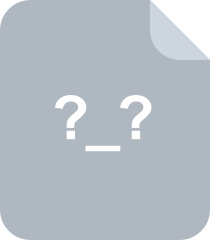
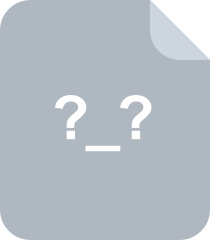
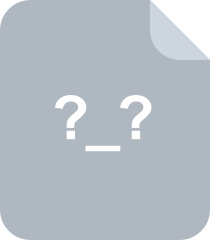
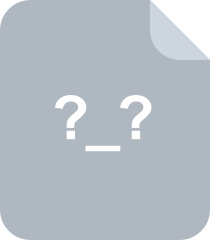
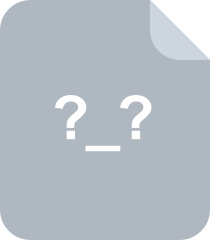
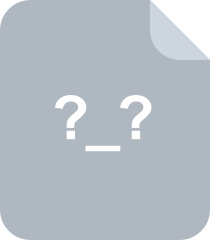
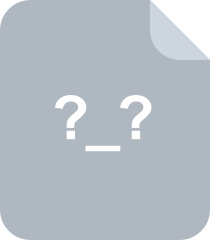
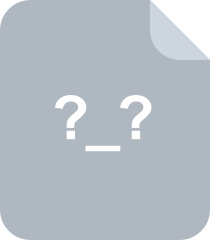
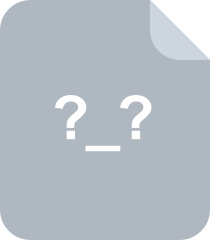
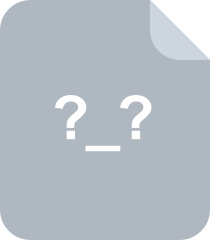
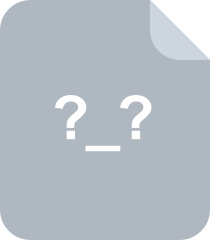
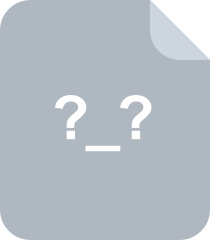
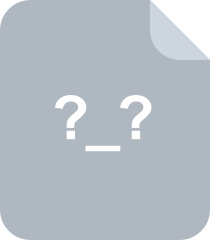
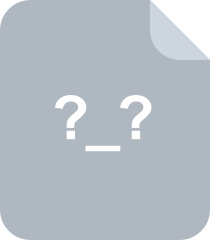
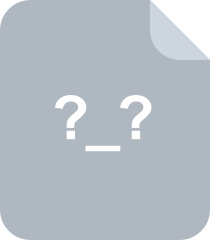
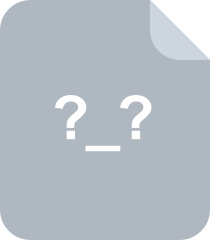
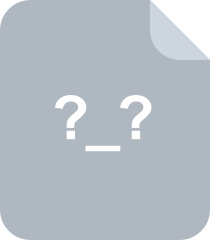
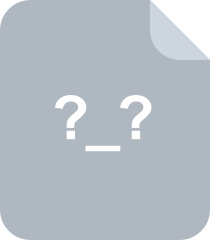
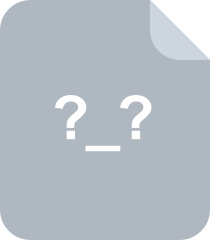
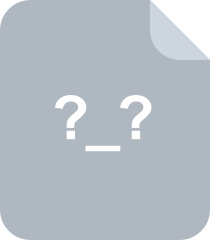
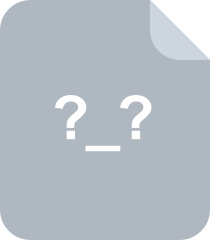
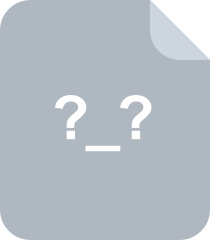
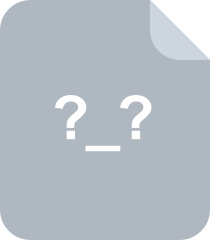
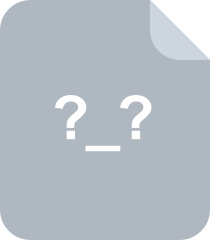
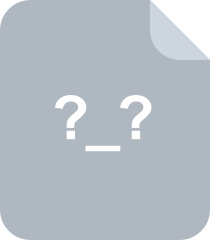
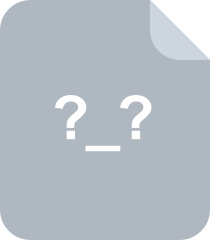
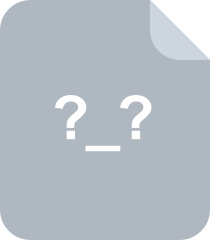
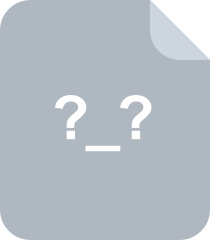
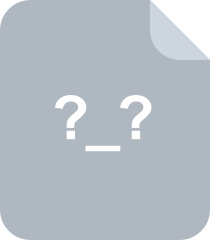
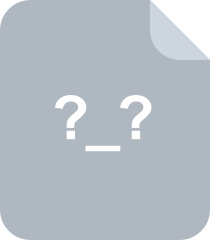
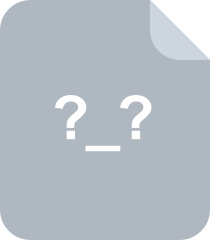
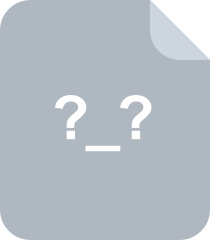
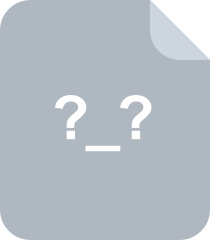
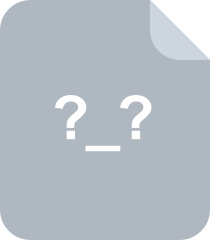
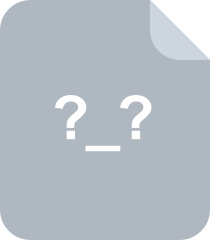
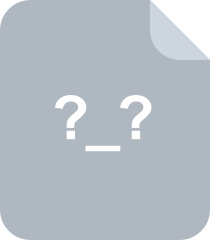
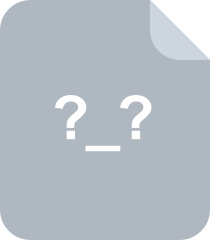
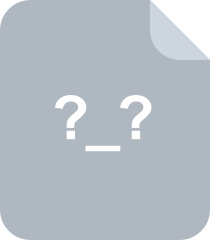
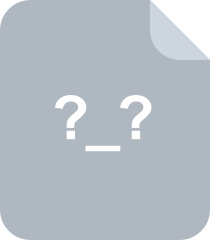
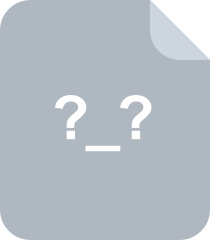
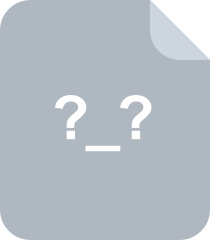
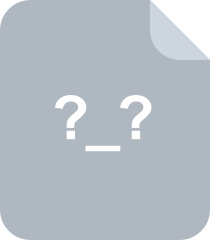
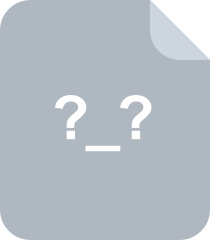
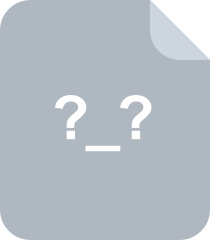
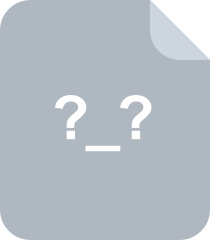
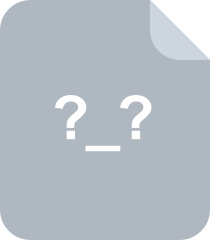
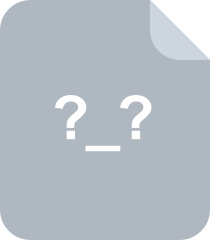
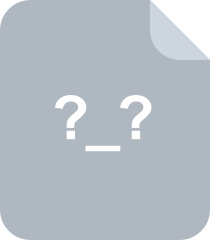
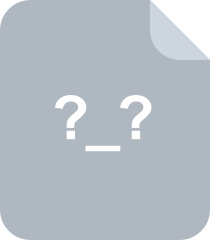
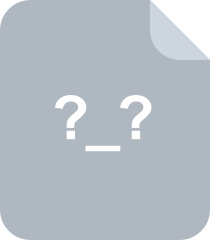
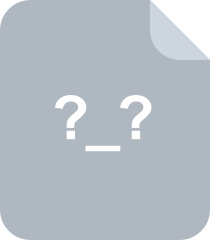
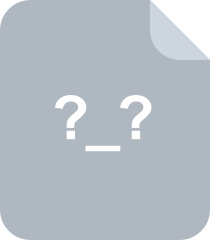
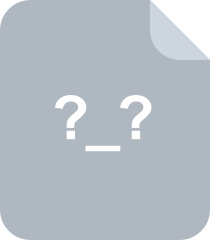
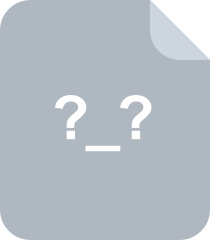
共 868 条
- 1
- 2
- 3
- 4
- 5
- 6
- 9
资源评论
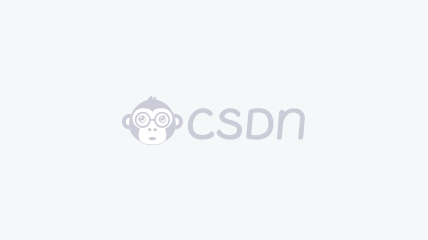

知一NN
- 粉丝: 42
- 资源: 4157
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

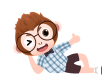
最新资源
- 高效会议管理技巧.doc
- 管理经济学(MBA全景教程之二).doc
- 公司理论与实务(MBA全景教程之五).doc
- 共赢领导力--提升领导力5种技术.doc
- 顾问式销售技术.doc
- 管理流程设计与管理流程再造.doc
- 管理信息系统(MBA全景教程之十).doc
- 管理学(MBA全景教程之四).doc
- 海尔模式.doc
- 培育核心竞争力的成功模式.doc
- 六西格玛在中国企业的实施—质量与流程能力的双重提升.doc
- 企业供应链物流管理—海尔、沃尔玛成功模式.doc
- 企业采购与供应商管理七大实战技能.doc
- 企业发展战略设计与实施要务.doc
- 企业核心竞争力的培育方法与误区分析.doc
- 企业国际化经营(MBA全景教程之七).doc
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


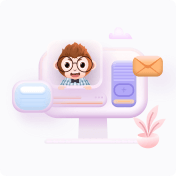
安全验证
文档复制为VIP权益,开通VIP直接复制
