CryptoAuthLib - Microchip CryptoAuthentication Library
====================================================
Introduction
------------------------
This code base implements an object-oriented C library which supports
Microchip CryptoAuth devices. The family of devices supported currently are:
- [ATSHA204A](http://www.microchip.com/ATSHA204A)
- [ATECC108A](http://www.microchip.com/ATECC108A)
- [ATECC508A](http://www.microchip.com/ATECC508A)
- [ATECC608A](http://www.microchip.com/ATECC608A)
Online documentation is at https://microchiptech.github.io/cryptoauthlib/
Latest software and examples can be found at:
- http://www.microchip.com/SWLibraryWeb/product.aspx?product=CryptoAuthLib
- https://github.com/MicrochipTech/cryptoauthtools
Prerequisite skills:
- strong C programming and code reading
- Atmel Studio familiarity
- Knowledge of flashing microcontrollers with new code
- Familiarity with Microchip CryptoAuth device functionality
Prerequisite hardware to run CryptoAuthLib examples:
- [ATSAMR21 Xplained Pro]( http://www.microchip.com/atsamr21-xpro )
or [ATSAMD21 Xplained Pro]( http://www.microchip.com/ATSAMD21-XPRO )
- [CryptoAuth Xplained Pro Extension](http://www.microchip.com/developmenttools/productdetails.aspx?partno=atcryptoauth-xpro-b )
or [CryptoAuthentication SOIC Socket Board](http://www.microchip.com/developmenttools/productdetails.aspx?partno=at88ckscktsoic-xpro )
to accept SOIC parts
For most development, using socketed top-boards is preferable until your
configuration is well tested, then you can commit it to a CryptoAuth Xplained
Pro Extension, for example. Keep in mind that once you lock a device, it will
not be changeable.
There are two major compiler defines that affect the operation of the library.
- ATCA_NO_POLL can be used to revert to a non-polling mechanism for device
responses. Normally responses are polled for after sending a command,
giving quicker response times. However, if ATCA_NO_POLL is defined, then
the library will simply delay the max execution time of a command before
reading the response.
- ATCA_NO_HEAP can be used to remove the use of malloc/free from the main
library. This can be helpful for smaller MCUs that don't have a heap
implemented. If just using the basic API, then there shouldn't be any code
changes required. The lower-level API will no longer use the new/delete
functions and the init/release functions should be used directly.
Examples
-----------
- Watch [CryptoAuthLib Documents](http://www.microchip.com/design-centers/security-ics/cryptoauthentication/overview )
for new examples coming online.
- Node Authentication Example Using Asymmetric PKI is a complete, all-in-one
example demonstrating all the stages of crypto authentication starting from
provisioning the Crypto Authentication device ATECC608A/ATECC508A with keys
and certificates to demonstrating an authentication sequence using
asymmetric techniques.
http://www.microchip.com/SWLibraryWeb/product.aspx?product=CryptoAuthLib
Release notes
-----------
11/22/2019
- Patches for CVE-2019-16128 & CVE-2019-16129: Ensure reported packet length
is valid for the packet being processed.
- Improvement to encrypted read operations to allow supply of a host nonce
(prevent replay of a read sequence to the host). Default API is changed
but can be reverted by setting the option ATCA_USE_CONSTANT_HOST_NONCE
- Added Azure compatible TNGTLS and TNGLORA certificates. Use the TNG client
API to retrieve the proper certificate based on the device.
- Misc Python updates (updated APIs for encrypted reads to match the C-API change)
atcacert_cert_element_t now initializes properly
08/30/2019
- Added big-endian architecture support
- Fixes to atcah_gen_dig() and atcah_nonce()
05/17/2019
- Added support for TNG devices (cert transforms, new API)
- atcab_write_pub_key() now works when the data zone is unlocked
03/04/2019
- mbed TLS wrapper added
- Minor bug fixes
01/25/2019
- Python JWT support
- Python configuration structures added
- Restructure of secure boot app
01/04/2019
- Added GCM functions
- Split AES modes into separate files
- Bug fix in SWI START driver
10/25/2018
- Added basic certificate functions to the python wrapper.
- Added Espressif ESP32 I2C driver.
- Made generic Atmel START drivers to support most MCUs in START.
- Added AES-CTR mode functions.
- Python wrapper functions now return single values with AtcaReference.
- Added mutex support to HAL and better support for freeRTOS.
08/17/2018
- Better support for multiple kit protocol devices
07/25/2018
- Clean up python wrapper
07/18/2018
- Added ATCA_NO_HEAP define to remove use of malloc/free.
- Moved PEM functions to their own file in atcacert.
- Added wake retry to accomodate power on self test delay.
- Added ca_cert_def member to atcacert_def_s so cert chains can be traversed
as a linked list.
03/29/2018
- Added support for response polling by default, which will make commands
return faster (define ATCA_NO_POLL to use old delay method).
- Removed atcatls related files as they were of limited value.
- Test framework generates a prompt before locking test configuration.
- Test framework puts device to sleep between tests.
- Fixed mode parameter issue in atcah_gen_key_msg().
- ATECC608A health test error code added.
01/15/2018
- Added AES-128 CBC implementation using AES command
- Added AES-128 CMAC implementation using AES command
11/22/2017
- Added support for FLEXCOM6 on SAMG55 driver
11/17/2017
- Added library support for the ATECC608A device
- Added support for Counter command
- atca_basic functions and tests now split into multiple files based on
command
- Added support for multiple base64 encoding rules
- Added support for JSON Web Tokens (jwt)
- Fixed atcab_write_enc() function to encrypt the data even when the device
is unlocked
- Fixed atcab_base64encode_() for the extra newline
- Updated atcab_ecdh_enc() to work more consistently
07/01/2017
- Removed assumption of SN[0:1]=0123, SN[8]=EE. SN now needs to be passed in
for functions in atca_host and atca_basic functions will now read the
config zone for the SN if needed.
- Renamed atcab_gendig_host() to atcab_gendig() since it's not a host
function. Removed original atcab_gendig(), which had limited scope.
- Fixed atcah_hmac() for host side HMAC calculations. Added atcab_hmac().
- Removed unnecessary ATCADeviceType parameters from some atca_basic
functions.
- Added atcacert_create_csr() to create a signed CSR.
- New HAL implementation for Kit protocol over HID on Linux. Please see the
Incorporating CryptoAuthLib in a Linux project using USB HID devices
section in this file for more information.
- Added atcacert_write_cert() for writing certificates to the device.
- Added support for dynamic length certificate serial numbers in atcacert.
- Added atcab_write() for lower level write commands.
- Fixed atcah_write_auth_mac(), which had wrong OpCode.
- Added atcab_verify() command for lower level verify commands.
- Added atcab_verify_stored() for verifying data with a stored public key.
- Removed atcab_write_bytes_slot(). Use atcab_write_bytes_zone() instead.
- Modified atcab_write_bytes_zone() and atcab_read_bytes_zone() to specify a
slot
- Added atcab_verify_validate() and atcab_verify_invalidate()
- Improvements to host functions to handle more cases.
- Added atcab_updateextra(), atcab_derive_key()
- Added support for more certificate formats.
- Added general purpose hardware SHA256 functions. See atcab_hw_sha2_256().
- Removed device specific config read/write. Generic now handles both.
- Removed unnecessary response parameter from lock commands.
- Enhanced and added unit tests.
- Encrypted read and write functions now handle keys with S
没有合适的资源?快使用搜索试试~ 我知道了~
基于springboot的物联网智能家居软硬件系统源码.zip
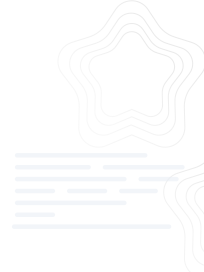
共2000个文件
txt:493个
c:473个
java:396个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 49 浏览量
2023-06-17
14:54:11
上传
评论 1
收藏 15.15MB ZIP 举报
温馨提示
源码是经过本地编译可运行的,下载完成之后配置相应环境即可使用。源码功能都是经过老师肯定的,都能满足要求,有需要放心下载即可。源码是经过本地编译可运行的,下载完成之后配置相应环境即可使用。源码功能都是经过老师肯定的,都能满足要求,有需要放心下载即可。源码是经过本地编译可运行的,下载完成之后配置相应环境即可使用。源码功能都是经过老师肯定的,都能满足要求,有需要放心下载即可。源码是经过本地编译可运行的,下载完成之后配置相应环境即可使用。源码功能都是经过老师肯定的,都能满足要求,有需要放心下载即可。源码是经过本地编译可运行的,下载完成之后配置相应环境即可使用。源码功能都是经过老师肯定的,都能满足要求,有需要放心下载即可。源码是经过本地编译可运行的,下载完成之后配置相应环境即可使用。源码功能都是经过老师肯定的,都能满足要求,有需要放心下载即可。源码是经过本地编译可运行的,下载完成之后配置相应环境即可使用。源码功能都是经过老师肯定的,都能满足要求,有需要放心下载即可。源码是经过本地编译可运行的,下载完成之后配置相应环境即可使用。源码功能都是经过老师肯定的,都能满足要求,有需要放心下载即可。源码是经
资源推荐
资源详情
资源评论
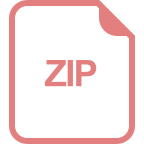
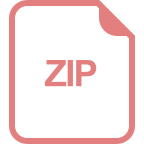
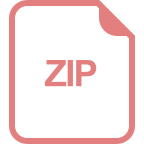
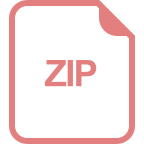
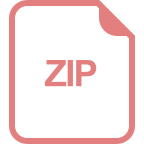
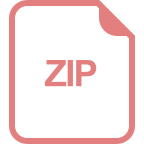
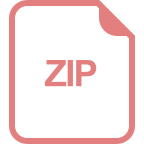
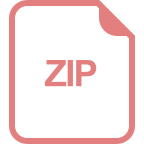
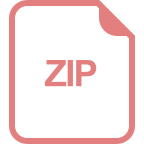
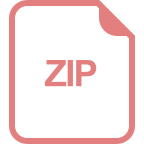
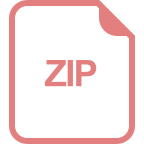
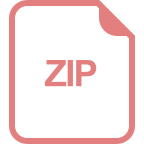
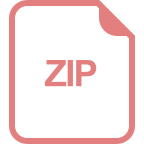
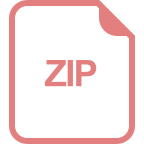
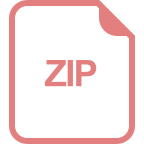
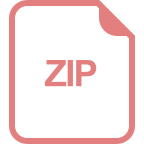
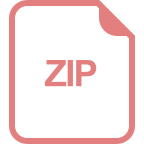
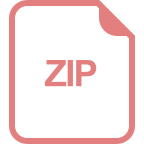
收起资源包目录

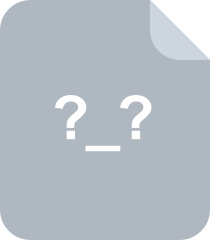
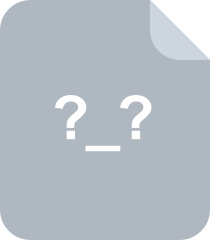
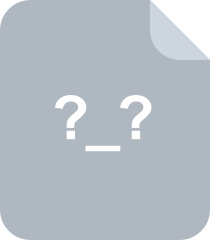
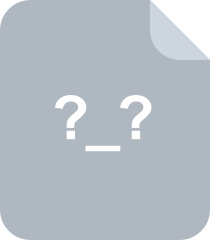
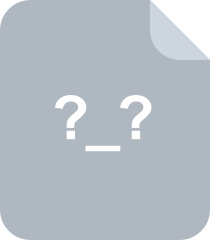
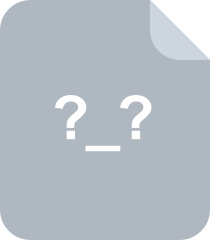
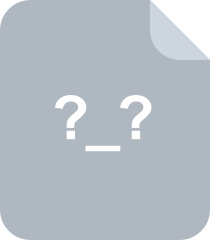
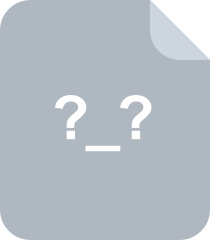
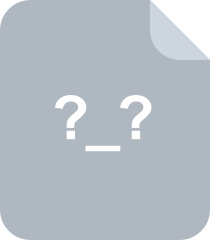
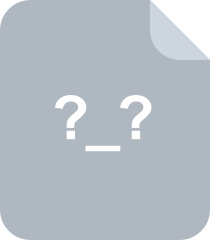
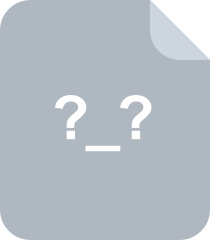
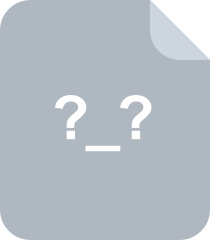
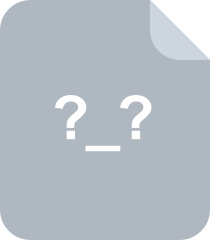
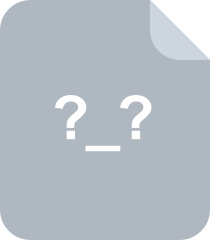
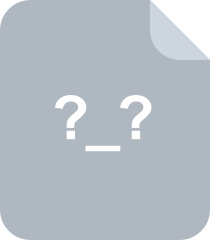
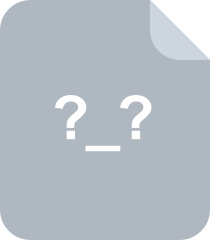
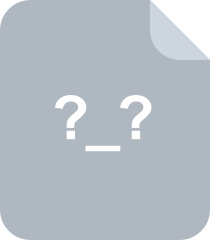
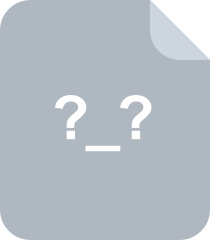
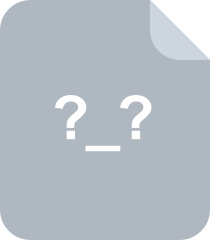
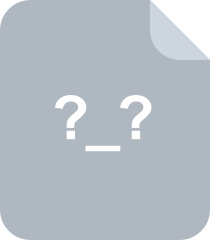
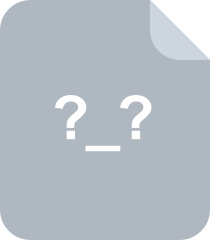
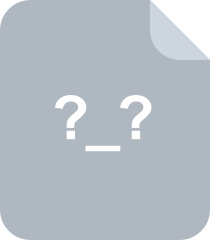
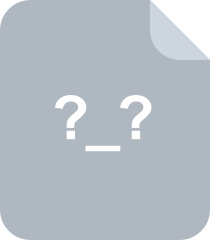
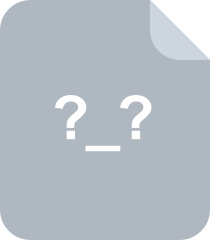
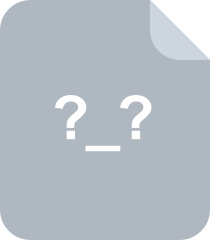
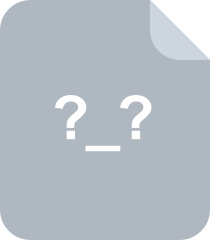
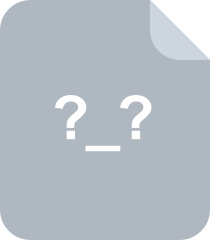
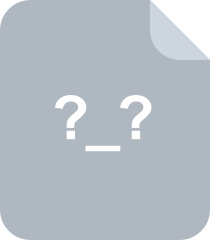
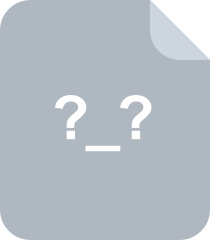
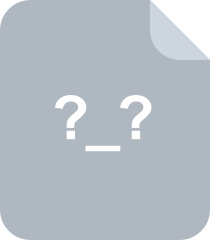
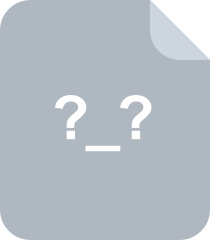
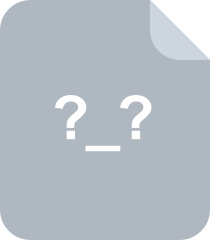
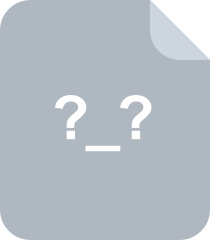
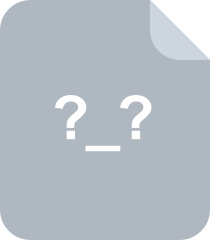
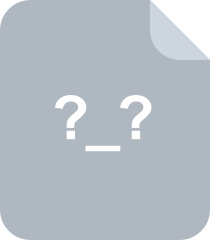
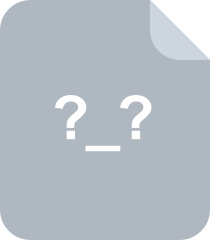
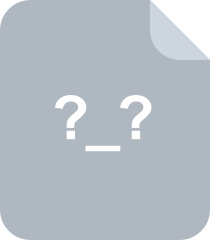
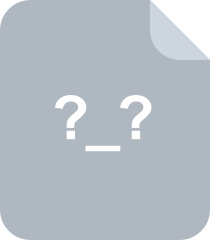
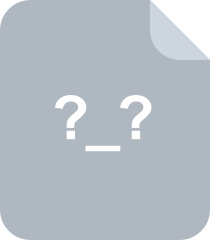
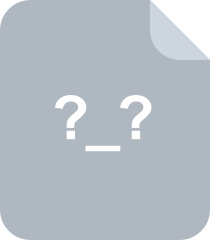
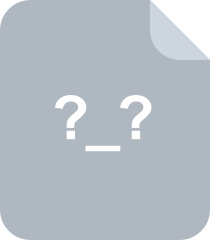
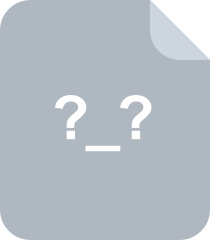
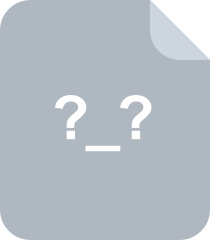
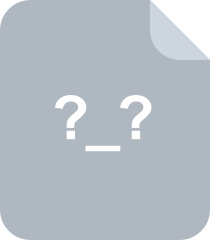
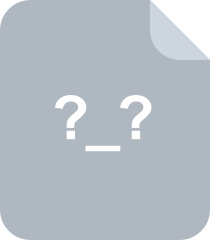
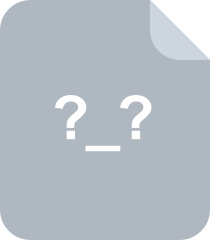
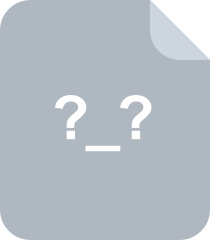
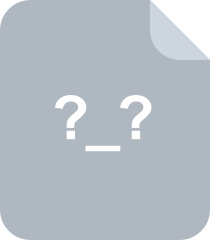
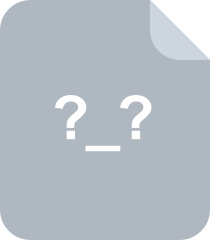
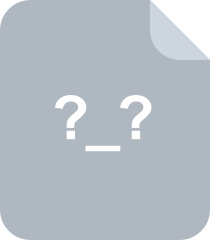
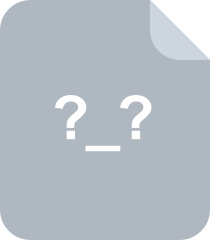
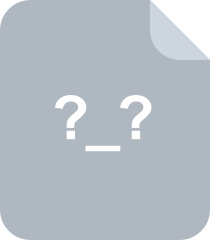
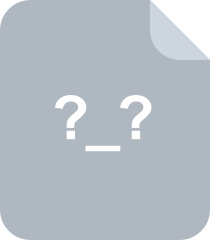
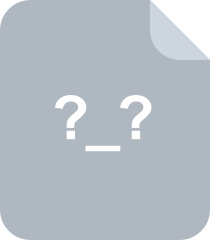
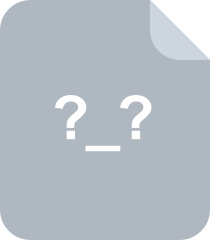
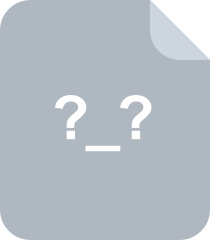
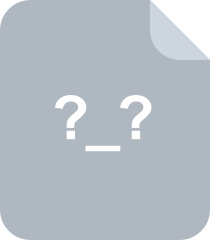
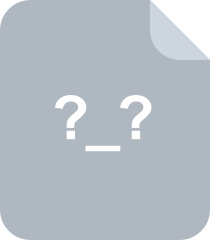
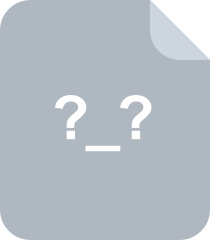
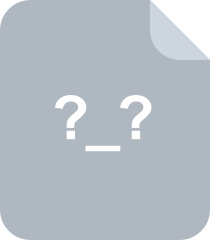
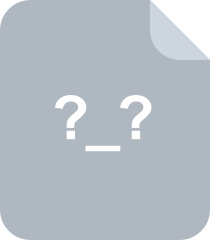
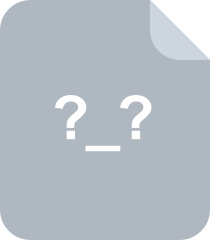
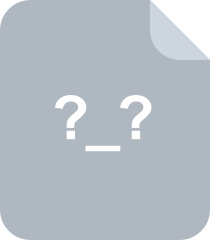
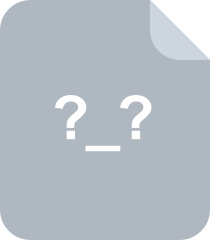
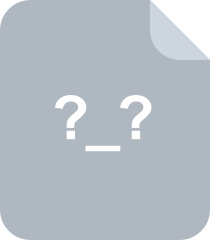
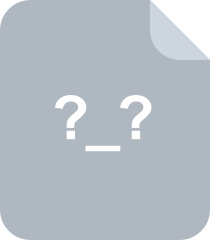
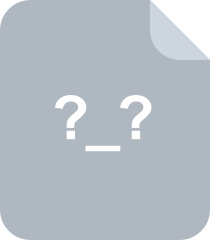
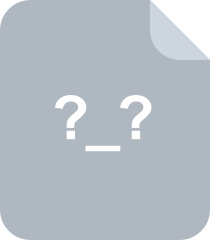
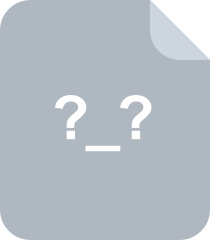
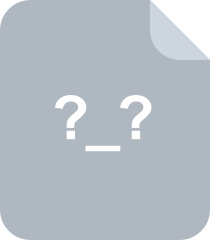
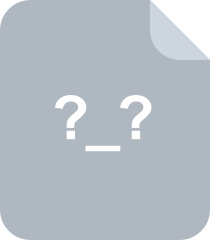
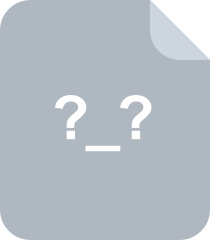
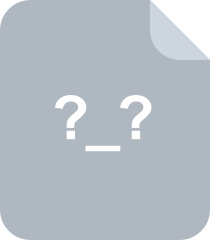
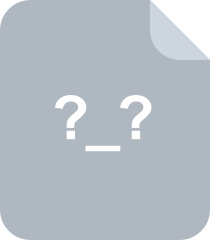
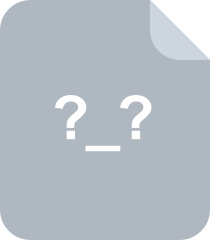
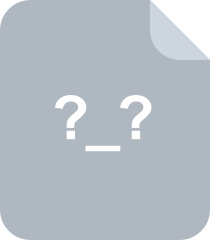
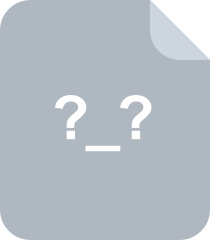
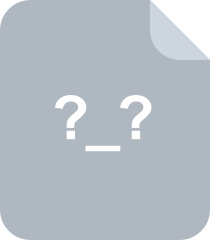
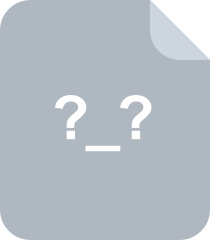
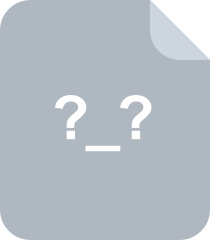
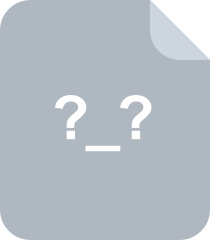
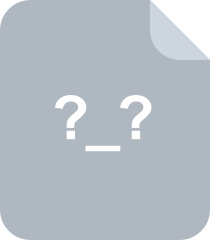
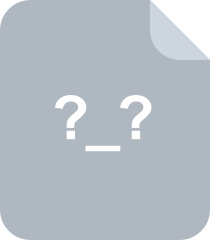
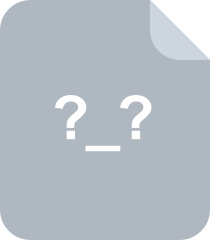
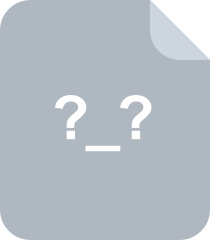
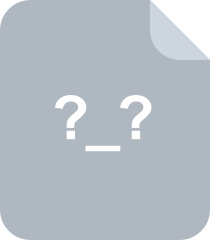
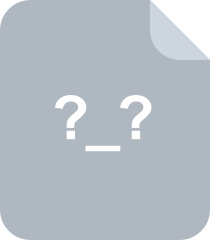
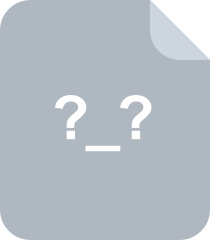
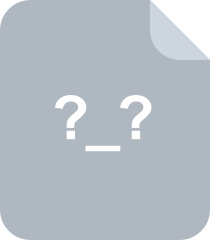
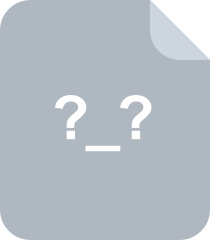
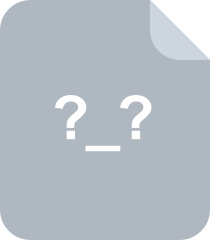
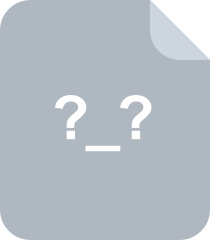
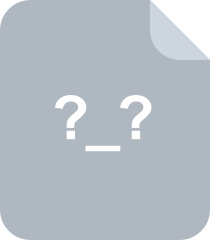
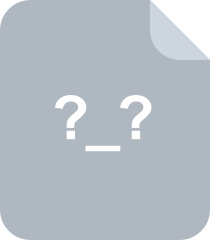
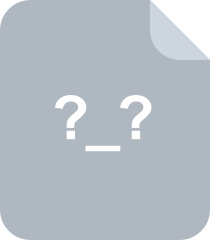
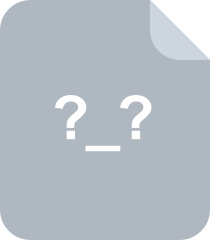
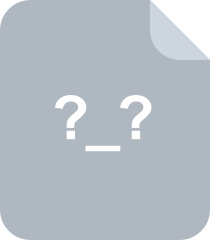
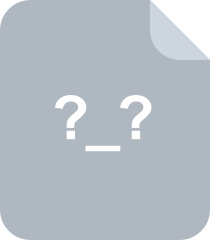
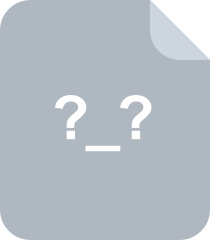
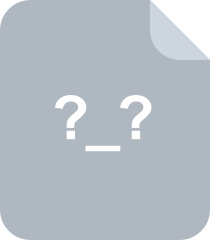
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
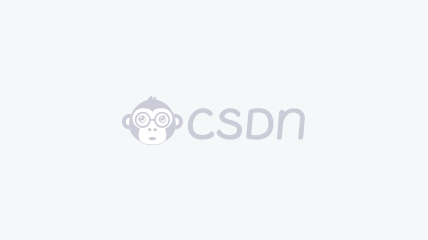

知一NN
- 粉丝: 42
- 资源: 4157
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

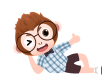
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


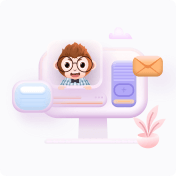
安全验证
文档复制为VIP权益,开通VIP直接复制
