# core-js
[](https://gitter.im/zloirock/core-js?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge) [](https://www.npmjs.com/package/core-js) [](http://npm-stat.com/charts.html?package=core-js&author=&from=2014-11-18) [](https://travis-ci.org/zloirock/core-js) [](https://david-dm.org/zloirock/core-js?type=dev)
#### As advertising: the author is looking for a good job :)
Modular standard library for JavaScript. Includes polyfills for [ECMAScript 5](#ecmascript-5), [ECMAScript 6](#ecmascript-6): [promises](#ecmascript-6-promise), [symbols](#ecmascript-6-symbol), [collections](#ecmascript-6-collections), iterators, [typed arrays](#ecmascript-6-typed-arrays), [ECMAScript 7+ proposals](#ecmascript-7-proposals), [setImmediate](#setimmediate), etc. Some additional features such as [dictionaries](#dict) or [extended partial application](#partial-application). You can require only needed features or use it without global namespace pollution.
[*Example*](http://goo.gl/a2xexl):
```js
Array.from(new Set([1, 2, 3, 2, 1])); // => [1, 2, 3]
'*'.repeat(10); // => '**********'
Promise.resolve(32).then(x => console.log(x)); // => 32
setImmediate(x => console.log(x), 42); // => 42
```
[*Without global namespace pollution*](http://goo.gl/paOHb0):
```js
var core = require('core-js/library'); // With a modular system, otherwise use global `core`
core.Array.from(new core.Set([1, 2, 3, 2, 1])); // => [1, 2, 3]
core.String.repeat('*', 10); // => '**********'
core.Promise.resolve(32).then(x => console.log(x)); // => 32
core.setImmediate(x => console.log(x), 42); // => 42
```
### Index
- [Usage](#usage)
- [Basic](#basic)
- [CommonJS](#commonjs)
- [Custom build](#custom-build-from-the-command-line)
- [Supported engines](#supported-engines)
- [Features](#features)
- [ECMAScript 5](#ecmascript-5)
- [ECMAScript 6](#ecmascript-6)
- [ECMAScript 6: Object](#ecmascript-6-object)
- [ECMAScript 6: Function](#ecmascript-6-function)
- [ECMAScript 6: Array](#ecmascript-6-array)
- [ECMAScript 6: String](#ecmascript-6-string)
- [ECMAScript 6: RegExp](#ecmascript-6-regexp)
- [ECMAScript 6: Number](#ecmascript-6-number)
- [ECMAScript 6: Math](#ecmascript-6-math)
- [ECMAScript 6: Date](#ecmascript-6-date)
- [ECMAScript 6: Promise](#ecmascript-6-promise)
- [ECMAScript 6: Symbol](#ecmascript-6-symbol)
- [ECMAScript 6: Collections](#ecmascript-6-collections)
- [ECMAScript 6: Typed Arrays](#ecmascript-6-typed-arrays)
- [ECMAScript 6: Reflect](#ecmascript-6-reflect)
- [ECMAScript 7+ proposals](#ecmascript-7-proposals)
- [stage 4 proposals](#stage-4-proposals)
- [stage 3 proposals](#stage-3-proposals)
- [stage 2 proposals](#stage-2-proposals)
- [stage 1 proposals](#stage-1-proposals)
- [stage 0 proposals](#stage-0-proposals)
- [pre-stage 0 proposals](#pre-stage-0-proposals)
- [Web standards](#web-standards)
- [setTimeout / setInterval](#settimeout--setinterval)
- [setImmediate](#setimmediate)
- [iterable DOM collections](#iterable-dom-collections)
- [Non-standard](#non-standard)
- [Object](#object)
- [Dict](#dict)
- [partial application](#partial-application)
- [Number Iterator](#number-iterator)
- [escaping strings](#escaping-strings)
- [delay](#delay)
- [helpers for iterators](#helpers-for-iterators)
- [Missing polyfills](#missing-polyfills)
- [Changelog](./CHANGELOG.md)
## Usage
### Basic
```
npm i core-js
bower install core.js
```
```js
// Default
require('core-js');
// Without global namespace pollution
var core = require('core-js/library');
// Shim only
require('core-js/shim');
```
If you need complete build for browser, use builds from `core-js/client` path:
* [default](https://raw.githack.com/zloirock/core-js/v2.5.3/client/core.min.js): Includes all features, standard and non-standard.
* [as a library](https://raw.githack.com/zloirock/core-js/v2.5.3/client/library.min.js): Like "default", but does not pollute the global namespace (see [2nd example at the top](#core-js)).
* [shim only](https://raw.githack.com/zloirock/core-js/v2.5.3/client/shim.min.js): Only includes the standard methods.
Warning: if you use `core-js` with the extension of native objects, require all needed `core-js` modules at the beginning of entry point of your application, otherwise, conflicts may occur.
### CommonJS
You can require only needed modules.
```js
require('core-js/fn/set');
require('core-js/fn/array/from');
require('core-js/fn/array/find-index');
Array.from(new Set([1, 2, 3, 2, 1])); // => [1, 2, 3]
[1, 2, NaN, 3, 4].findIndex(isNaN); // => 2
// or, w/o global namespace pollution:
var Set = require('core-js/library/fn/set');
var from = require('core-js/library/fn/array/from');
var findIndex = require('core-js/library/fn/array/find-index');
from(new Set([1, 2, 3, 2, 1])); // => [1, 2, 3]
findIndex([1, 2, NaN, 3, 4], isNaN); // => 2
```
Available entry points for methods / constructors, as above examples, and namespaces: for example, `core-js/es6/array` (`core-js/library/es6/array`) contains all [ES6 `Array` features](#ecmascript-6-array), `core-js/es6` (`core-js/library/es6`) contains all ES6 features.
##### Caveats when using CommonJS API:
* `modules` path is internal API, does not inject all required dependencies and can be changed in minor or patch releases. Use it only for a custom build and / or if you know what are you doing.
* `core-js` is extremely modular and uses a lot of very tiny modules, because of that for usage in browsers bundle up `core-js` instead of usage loader for each file, otherwise, you will have hundreds of requests.
#### CommonJS and prototype methods without global namespace pollution
In the `library` version, we can't pollute prototypes of native constructors. Because of that, prototype methods transformed to static methods like in examples above. `babel` `runtime` transformer also can't transform them. But with transpilers we can use one more trick - [bind operator and virtual methods](https://github.com/zenparsing/es-function-bind). Special for that, available `/virtual/` entry points. Example:
```js
import fill from 'core-js/library/fn/array/virtual/fill';
import findIndex from 'core-js/library/fn/array/virtual/find-index';
Array(10)::fill(0).map((a, b) => b * b)::findIndex(it => it && !(it % 8)); // => 4
// or
import {fill, findIndex} from 'core-js/library/fn/array/virtual';
Array(10)::fill(0).map((a, b) => b * b)::findIndex(it => it && !(it % 8)); // => 4
```
### Custom build (from the command-line)
```
npm i core-js && cd node_modules/core-js && npm i
npm run grunt build:core.dict,es6 -- --blacklist=es6.promise,es6.math --library=on --path=custom uglify
```
Where `core.dict` and `es6` are modules (namespaces) names, which will be added to the build, `es6.promise` and `es6.math` are modules (namespaces) names, which will be excluded from the build, `--library=on` is flag for build without global namespace pollution and `custom` is target file name.
Available namespaces: for example, `es6.array` contains [ES6 `Array` features](#ecmascript-6-array), `es6` contains all modules whose names start with `es6`.
### Custom build (from external scripts)
[`core-js-builder`](https://www.npmjs.com/package/core-js-builder) package exports a function that takes the same parameters as the `build` target from the previous section. This will conditionally include or exclude certain parts of `core-js`:
```js
require('core-js-builder')({
modules: ['es6', 'core.dict'], // modules / namespaces
blacklist: ['es6.reflect'], /
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
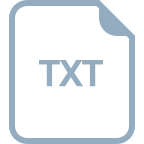
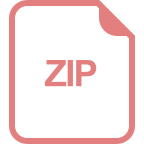
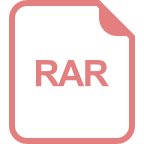
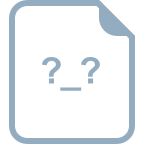
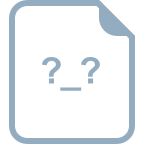
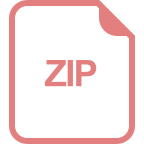
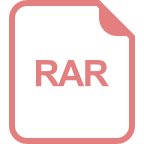
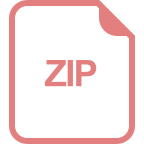
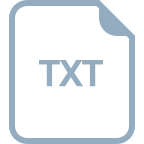
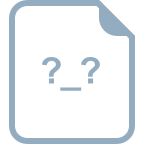
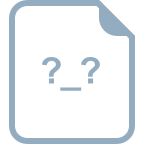
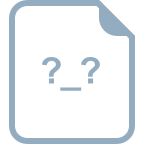
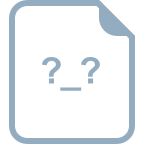
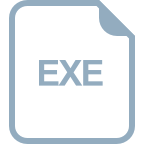
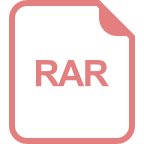
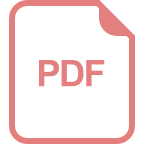
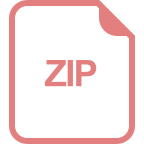
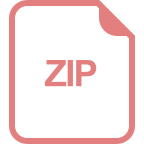
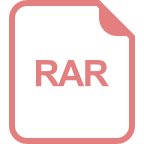
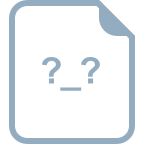
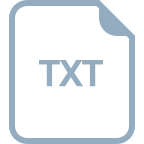
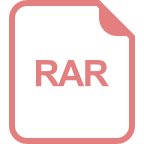
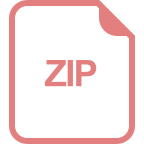
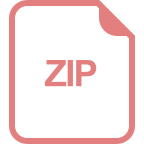
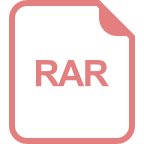
收起资源包目录

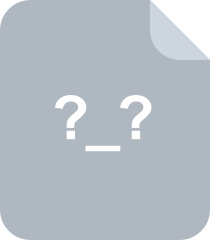
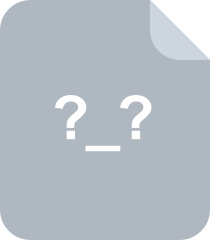
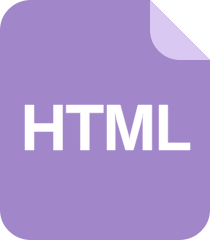
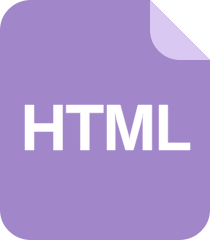
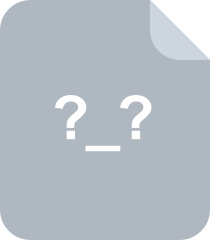
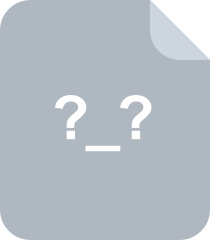
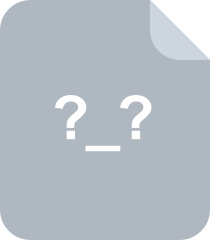
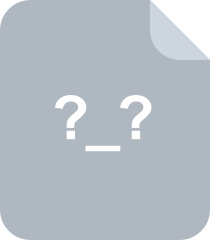
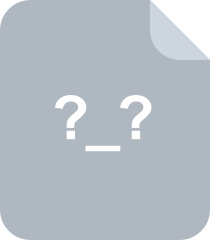
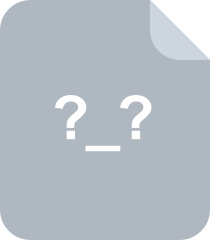
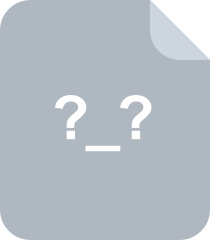
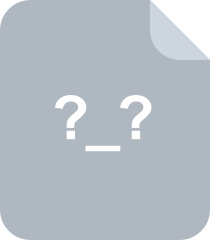
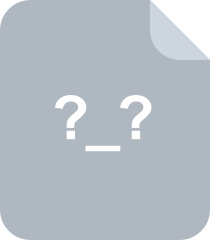
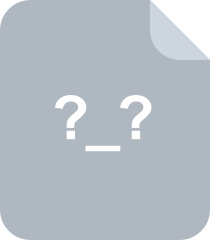
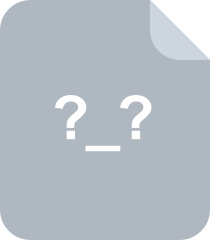
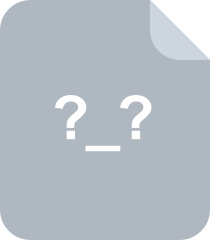
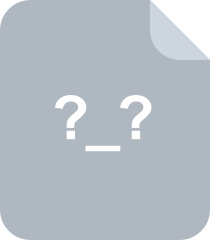
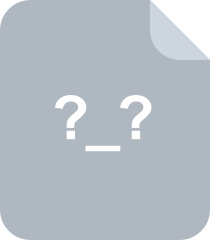
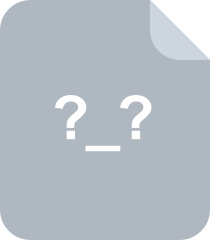
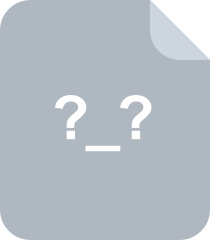
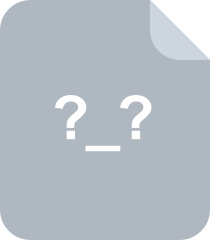
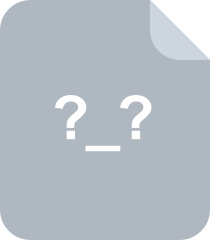
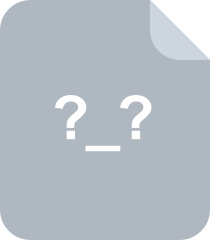
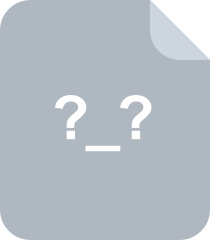
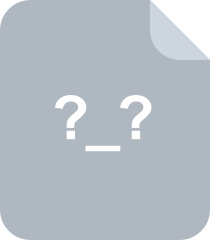
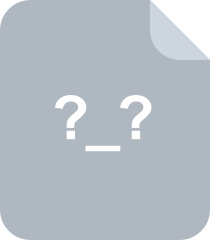
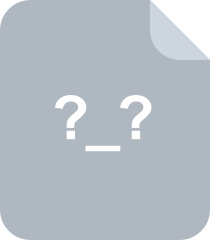
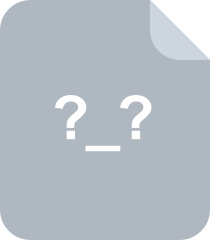
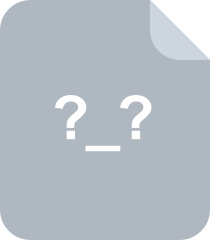
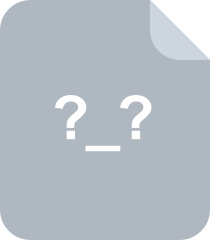
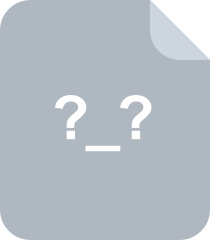
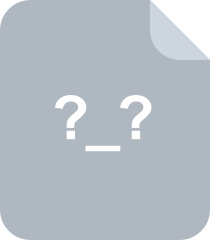
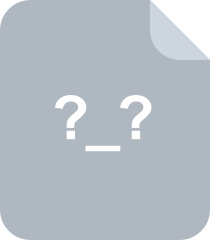
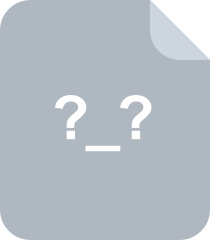
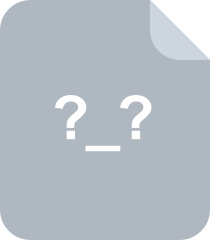
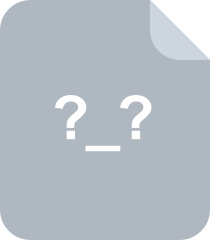
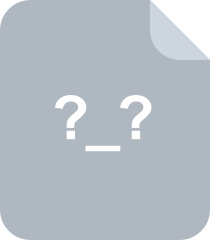
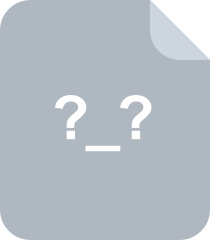
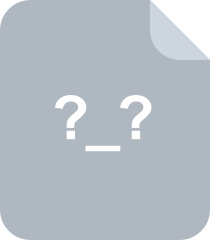
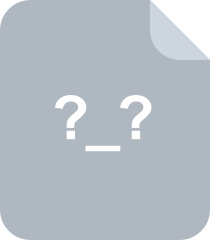
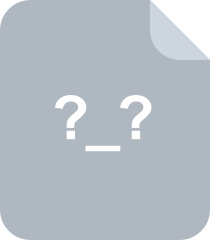
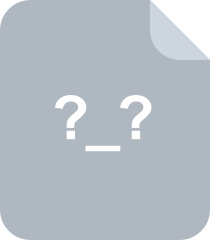
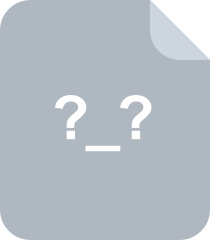
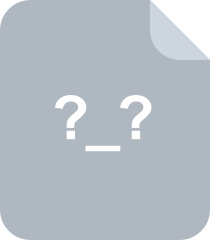
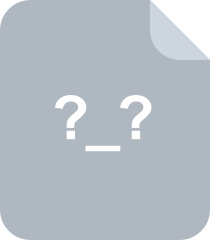
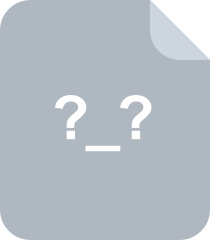
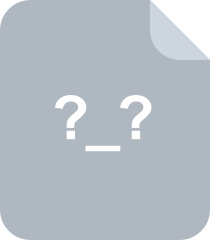
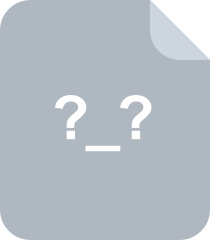
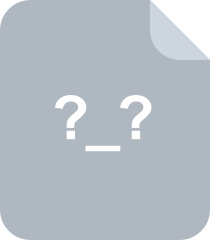
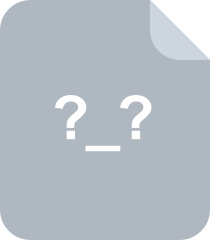
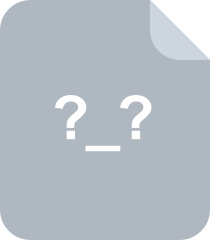
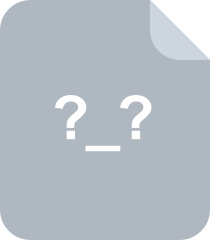
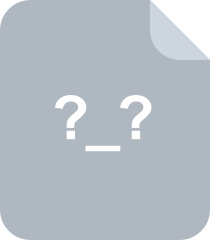
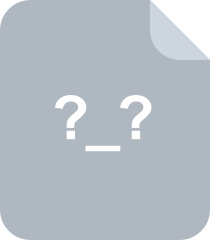
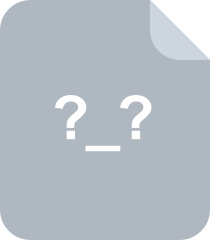
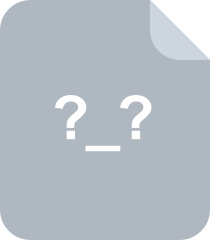
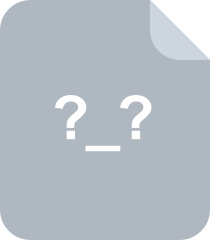
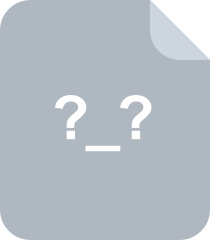
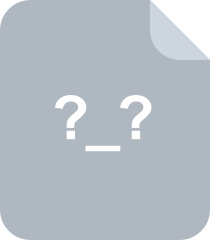
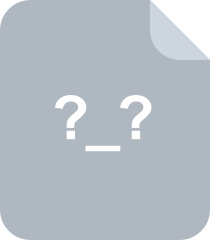
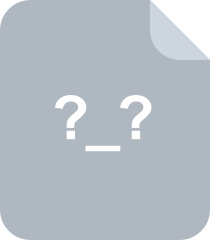
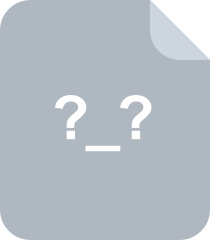
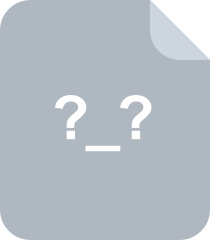
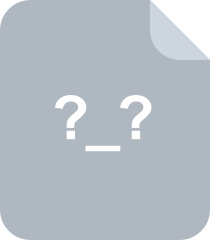
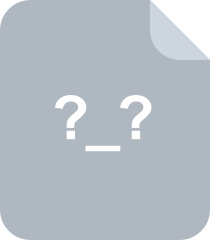
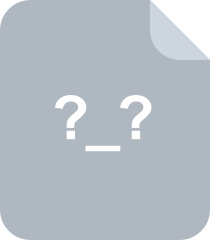
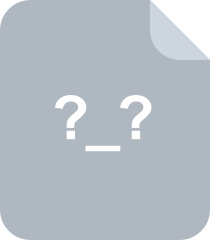
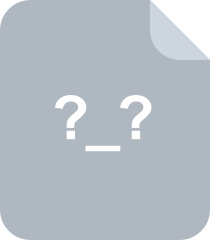
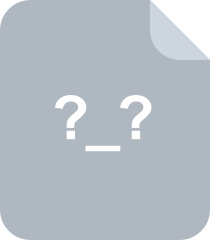
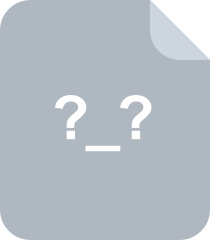
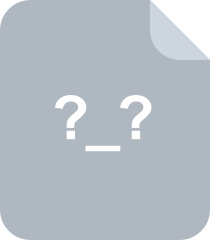
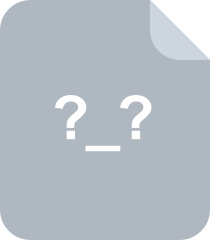
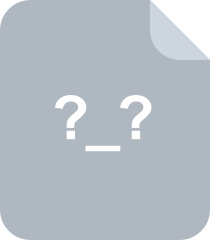
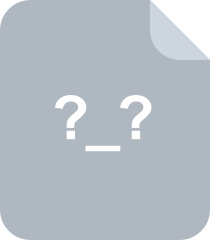
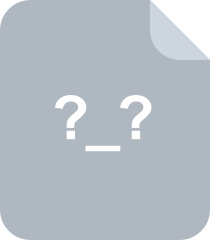
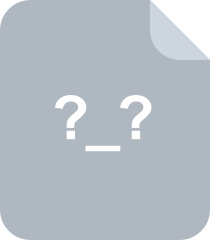
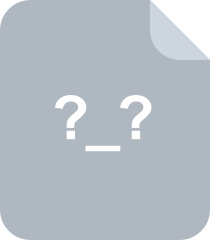
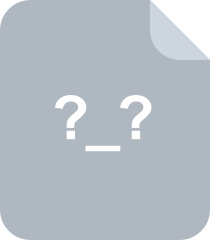
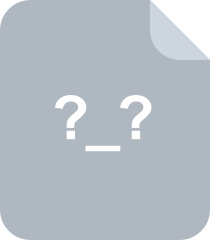
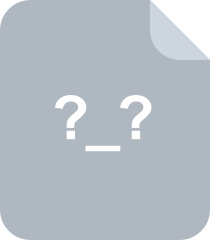
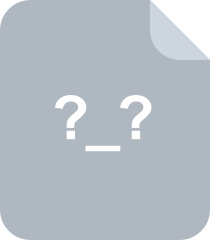
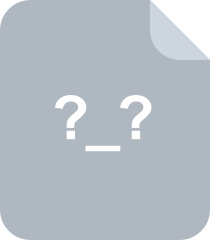
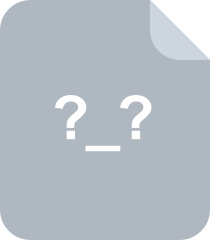
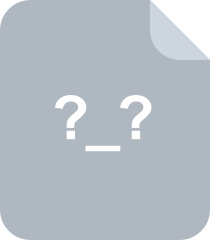
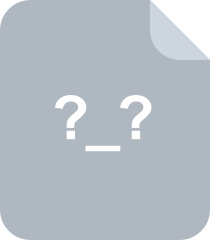
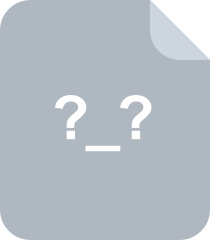
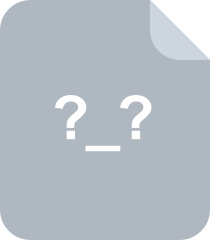
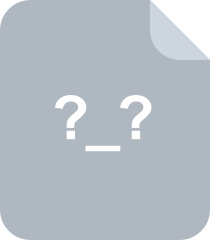
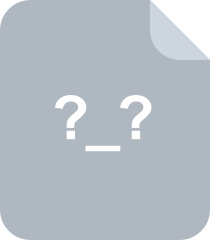
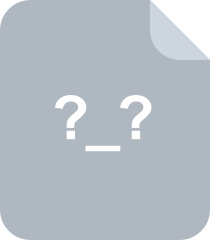
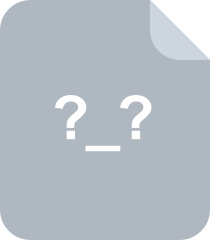
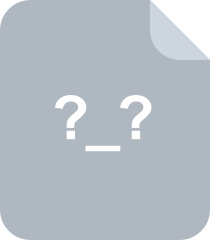
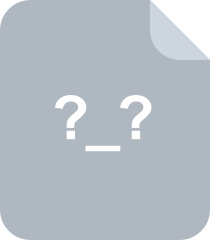
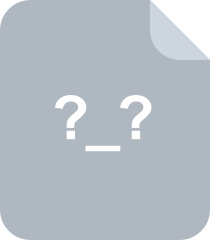
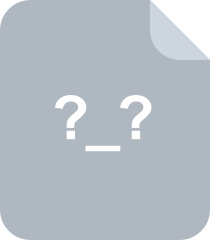
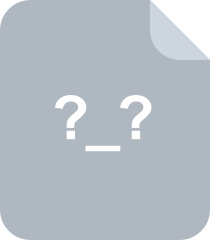
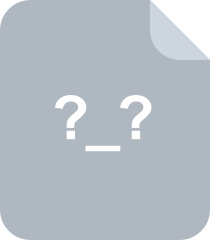
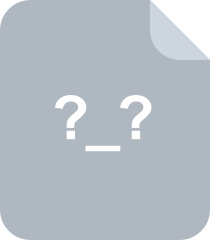
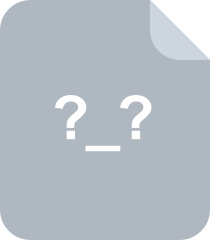
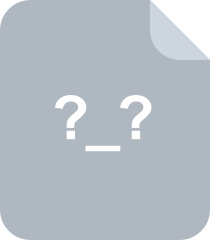
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
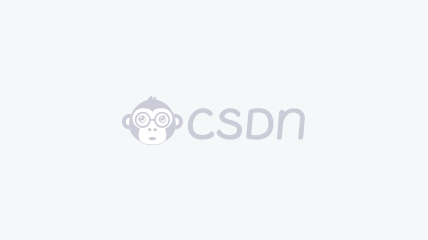

小谷2513
- 粉丝: 0
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

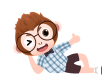
最新资源
- 基于FPGA的占空比测量模块-verilog语言
- c语言一个简单的线程池,实现不超过100行.zip
- C语言- 简易三子棋.zip
- 计算机网络课设-协议及流程分析
- COStream 工具在动态类型语言 js 上部署,目的是代码定制 & 易读 & 易测试.zip
- COIMS是应西安某救助站需求,义务为其开发的简易流浪者病历管理软件,基于GTK+2.24版本、SQLite3并采用C语言开发 不以成败论英雄 .zip
- CN编程语言,一个快速、便捷、易学的中文编程语言.zip
- 迈微88NV1120量产工具
- 伺服电机选型的技术指导及其应用场景
- CMM(C语言的一个简单版本)语言的解释器(用C++编写).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


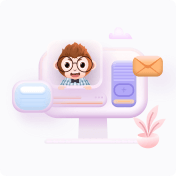
安全验证
文档复制为VIP权益,开通VIP直接复制
