package com.bjpowernode.finance.entity;
import java.util.ArrayList;
import java.util.List;
public class UserExample {
protected String orderByClause;
protected boolean distinct;
protected List<Criteria> oredCriteria;
public UserExample() {
oredCriteria = new ArrayList<Criteria>();
}
public void setOrderByClause(String orderByClause) {
this.orderByClause = orderByClause;
}
public String getOrderByClause() {
return orderByClause;
}
public void setDistinct(boolean distinct) {
this.distinct = distinct;
}
public boolean isDistinct() {
return distinct;
}
public List<Criteria> getOredCriteria() {
return oredCriteria;
}
public void or(Criteria criteria) {
oredCriteria.add(criteria);
}
public Criteria or() {
Criteria criteria = createCriteriaInternal();
oredCriteria.add(criteria);
return criteria;
}
public Criteria createCriteria() {
Criteria criteria = createCriteriaInternal();
if (oredCriteria.size() == 0) {
oredCriteria.add(criteria);
}
return criteria;
}
protected Criteria createCriteriaInternal() {
Criteria criteria = new Criteria();
return criteria;
}
public void clear() {
oredCriteria.clear();
orderByClause = null;
distinct = false;
}
protected abstract static class GeneratedCriteria {
protected List<Criterion> criteria;
protected GeneratedCriteria() {
super();
criteria = new ArrayList<Criterion>();
}
public boolean isValid() {
return criteria.size() > 0;
}
public List<Criterion> getAllCriteria() {
return criteria;
}
public List<Criterion> getCriteria() {
return criteria;
}
protected void addCriterion(String condition) {
if (condition == null) {
throw new RuntimeException("Value for condition cannot be null");
}
criteria.add(new Criterion(condition));
}
protected void addCriterion(String condition, Object value, String property) {
if (value == null) {
throw new RuntimeException("Value for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value));
}
protected void addCriterion(String condition, Object value1, Object value2, String property) {
if (value1 == null || value2 == null) {
throw new RuntimeException("Between values for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value1, value2));
}
public Criteria andIdIsNull() {
addCriterion("id is null");
return (Criteria) this;
}
public Criteria andIdIsNotNull() {
addCriterion("id is not null");
return (Criteria) this;
}
public Criteria andIdEqualTo(Integer value) {
addCriterion("id =", value, "id");
return (Criteria) this;
}
public Criteria andIdNotEqualTo(Integer value) {
addCriterion("id <>", value, "id");
return (Criteria) this;
}
public Criteria andIdGreaterThan(Integer value) {
addCriterion("id >", value, "id");
return (Criteria) this;
}
public Criteria andIdGreaterThanOrEqualTo(Integer value) {
addCriterion("id >=", value, "id");
return (Criteria) this;
}
public Criteria andIdLessThan(Integer value) {
addCriterion("id <", value, "id");
return (Criteria) this;
}
public Criteria andIdLessThanOrEqualTo(Integer value) {
addCriterion("id <=", value, "id");
return (Criteria) this;
}
public Criteria andIdIn(List<Integer> values) {
addCriterion("id in", values, "id");
return (Criteria) this;
}
public Criteria andIdNotIn(List<Integer> values) {
addCriterion("id not in", values, "id");
return (Criteria) this;
}
public Criteria andIdBetween(Integer value1, Integer value2) {
addCriterion("id between", value1, value2, "id");
return (Criteria) this;
}
public Criteria andIdNotBetween(Integer value1, Integer value2) {
addCriterion("id not between", value1, value2, "id");
return (Criteria) this;
}
public Criteria andUsernameIsNull() {
addCriterion("username is null");
return (Criteria) this;
}
public Criteria andUsernameIsNotNull() {
addCriterion("username is not null");
return (Criteria) this;
}
public Criteria andUsernameEqualTo(String value) {
addCriterion("username =", value, "username");
return (Criteria) this;
}
public Criteria andUsernameNotEqualTo(String value) {
addCriterion("username <>", value, "username");
return (Criteria) this;
}
public Criteria andUsernameGreaterThan(String value) {
addCriterion("username >", value, "username");
return (Criteria) this;
}
public Criteria andUsernameGreaterThanOrEqualTo(String value) {
addCriterion("username >=", value, "username");
return (Criteria) this;
}
public Criteria andUsernameLessThan(String value) {
addCriterion("username <", value, "username");
return (Criteria) this;
}
public Criteria andUsernameLessThanOrEqualTo(String value) {
addCriterion("username <=", value, "username");
return (Criteria) this;
}
public Criteria andUsernameLike(String value) {
addCriterion("username like", value, "username");
return (Criteria) this;
}
public Criteria andUsernameNotLike(String value) {
addCriterion("username not like", value, "username");
return (Criteria) this;
}
public Criteria andUsernameIn(List<String> values) {
addCriterion("username in", values, "username");
return (Criteria) this;
}
public Criteria andUsernameNotIn(List<String> values) {
addCriterion("username not in", values, "username");
return (Criteria) this;
}
public Criteria andUsernameBetween(String value1, String value2) {
addCriterion("username between", value1, value2, "username");
return (Criteria) this;
}
public Criteria andUsernameNotBetween(String value1, String value2) {
addCriterion("username not between", value1, value2, "username");
return (Criteria) this;
}
public Criteria andRealnameIsNull() {
addCriterion("realname is null");
return (Criteria) this;
}
public Criteria andRealnameIsNotNull() {
addCriterion("realname is not null");
return (Criteria) this;
}
public Criteria andRealnameEqualTo(String value) {
addCriterion("realname =", value, "realname");
return (Criteria) this;
}
public Criteria andRealnameNotEqualTo(String value) {
addCriterion("realname <>", value, "realname");
return (Criteria) this;
}
public Criteria andRealnameGreaterThan(String value) {

老了敲不动了
- 粉丝: 87
- 资源: 4618
最新资源
- 串口转网络模块源码,uart tcp ip 以太网关模块 stm32f107主控,曾经量产过 pcb ad10工程 mcu keil工程 上位机 vc6工程 提供所有设计文件源码,可学习,可生
- MW54 微型涡喷发动机 涡轮喷气发动机 平面图纸+三维建模,文件格式是STP,通用格
- 码垛机图纸,伺料码垛机图纸,腻子粉码垛机图纸,可借鉴学习,参考设计
- 共90套左右各类污水处理设备三维模型,管道设备三维模型,石油化工设备三维模型 sw打开,大部分是可以编辑修改尺寸的 有装配体模型,有零部件模型
- springboot 集成 lemon-imui vue tio 完成即时通讯
- 30天开发操作系统 第 13 天 - 定时器 v2.0
- 基于React框架的Airbnb风格民宿租赁门户网站设计源码
- 燃料电池汽车Cruise整车仿真模型(燃料电池电电混动整车仿真模型) 1.基于Cruise与MATLAB Simulink联合仿真完成整个模型搭建,策略为多点恒功率(多点功率跟随)式控制策略,策略模
- 基于Vue3、uniapp的树洞公众号语聊搭子陪玩社交社区论坛礼物特效IM聊天系统设计源码
- comsol 离散裂隙 两相流模型
- FLAC3D后处理,将云图转为三维,可视化更强 图一为flac原图,图二图三为处理后的图 内容包括:案例文件,fish代码和matlab代码
- 汽车BCM程序源代码 国产车BCM程序源代码 外部灯光:前照灯、小灯、转向灯、前后雾灯、日间行车灯、倒车灯、制动灯、角灯、泊车灯等 内部灯光:顶灯、钥匙光圈、门灯 前后雨
- 纯电动汽车仿真、纯电动公交、纯电动客车、纯电动汽车动力性仿真、经济性仿真、续航里程仿真 模型包括电机、电池、车辆模型 有两种模型2选1: 1 完全用matlab simulink搭建的模型 2用
- 电机控制器,两种基于滑模观测器的PMSM无感矢量控制仿真(开关设置区分): 1. PLL+滑模(降低高频开关噪声); 2. arctan+滑模; 有配套算法原理资料
- 包含光热电站的综合能源系统优化运行规划(MATLAB+cplex) 采用Matlab程序Yalmip+Cplex求解 系统中包含电、热、冷、气 系统中机组有:风力,光伏,燃气轮机,P2G, 电制冷,O
- 双馈风力发电系统模型 Matlab simulink仿真运行 可直接跑
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


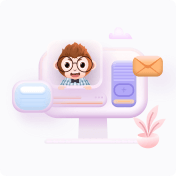