package com.example.controller;
import cn.hutool.core.collection.CollUtil;
import cn.hutool.core.collection.CollectionUtil;
import cn.hutool.core.io.IoUtil;
import cn.hutool.core.util.ObjectUtil;
import cn.hutool.core.util.StrUtil;
import cn.hutool.crypto.SecureUtil;
import cn.hutool.json.JSONObject;
import cn.hutool.poi.excel.ExcelUtil;
import cn.hutool.poi.excel.ExcelWriter;
import com.example.common.Result;
import com.example.common.ResultCode;
import com.example.entity.ShangpinxinxiInfo;
import com.example.dao.ShangpinxinxiInfoDao;
import com.example.service.ShangpinxinxiInfoService;
import com.example.exception.CustomException;
import com.example.common.ResultCode;
import com.example.vo.EchartsData;
import com.example.vo.ShangpinxinxiInfoVo;
import com.github.pagehelper.PageHelper;
import com.github.pagehelper.PageInfo;
import com.example.service.*;
import org.springframework.web.bind.annotation.*;
import org.springframework.beans.factory.annotation.Value;
import cn.hutool.core.util.StrUtil;
import org.springframework.web.multipart.MultipartFile;
import javax.annotation.Resource;
import javax.servlet.ServletOutputStream;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.util.*;
import java.util.stream.Collectors;
@RestController
@RequestMapping(value = "/shangpinxinxiInfo")
public class ShangpinxinxiInfoController {
@Resource
private ShangpinxinxiInfoService shangpinxinxiInfoService;
@Resource
private ShangpinxinxiInfoDao shangpinxinxiInfoDao;
@PostMapping
public Result<ShangpinxinxiInfo> add(@RequestBody ShangpinxinxiInfoVo shangpinxinxiInfo) {
//mixmajixami
shangpinxinxiInfoService.add(shangpinxinxiInfo);
return Result.success(shangpinxinxiInfo);
}
@GetMapping("/tixing")
public Result<Integer> tixing() { Integer count = shangpinxinxiInfoService.tixing(); return Result.success(count); }
// @GetMapping("/getByDiqu")
// public Result<List<Map<String,String>>> qidu() {
// return Result.success(shangpinxinxiInfoService.findByDiqu());
// }
@DeleteMapping("/{id}")
public Result delete(@PathVariable Long id) {
shangpinxinxiInfoService.delete(id);
return Result.success();
}
@PutMapping
public Result update(@RequestBody ShangpinxinxiInfoVo shangpinxinxiInfo) {
shangpinxinxiInfoService.update(shangpinxinxiInfo);
return Result.success();
}
//@PutMapping("/update2")
// public Result update2(@RequestBody ShangpinxinxiInfoVo shangpinxinxiInfo) {
// shangpinxinxiInfoService.update2(shangpinxinxiInfo);
// return Result.success();
// }
@GetMapping("/{id}")
public Result<ShangpinxinxiInfo> detail(@PathVariable Long id) {
ShangpinxinxiInfo shangpinxinxiInfo = shangpinxinxiInfoService.findById(id);
return Result.success(shangpinxinxiInfo);
}
@GetMapping("/changeStatus/{id}")
public Result<ShangpinxinxiInfo> changeStatus(@PathVariable Long id) {
shangpinxinxiInfoService.changeStatus(id);
return Result.success();
}
@GetMapping
public Result<List<ShangpinxinxiInfoVo>> all() {
return Result.success(shangpinxinxiInfoService.findAll());
}
@GetMapping("/page/{name}")
public Result<PageInfo<ShangpinxinxiInfoVo>> page(@PathVariable String name,
@RequestParam(defaultValue = "1") Integer pageNum,
@RequestParam(defaultValue = "5") Integer pageSize,
HttpServletRequest request) {
return Result.success(shangpinxinxiInfoService.findPage(name, pageNum, pageSize, request));
}
@GetMapping("/pageqt/{name}")
public Result<PageInfo<ShangpinxinxiInfoVo>> pageqt(@PathVariable String name,
@RequestParam(defaultValue = "1") Integer pageNum,
@RequestParam(defaultValue = "8") Integer pageSize,
HttpServletRequest request) {
return Result.success(shangpinxinxiInfoService.findPageqt(name, pageNum, pageSize, request));
}
@GetMapping("/findByType/{type}")
public Result<List<ShangpinxinxiInfoVo>> findByType(@PathVariable String type) {
return Result.success(shangpinxinxiInfoService.findByType(type));
}
// @PostMapping("/register")
// public Result<ShangpinxinxiInfo> register(@RequestBody ShangpinxinxiInfo shangpinxinxiInfo) {
// if (StrUtil.isBlank(shangpinxinxiInfo.getName()) || StrUtil.isBlank(shangpinxinxiInfo.getPassword())) {
// throw new CustomException(ResultCode.PARAM_ERROR);
// }
// return Result.success(shangpinxinxiInfoService.add(shangpinxinxiInfo));
// }
/**
* 批量通过excel添加信息
* @param file excel文件
* @throws IOException
*/
@PostMapping("/upload")
public Result upload(MultipartFile file) throws IOException {
List<ShangpinxinxiInfo> infoList = ExcelUtil.getReader(file.getInputStream()).readAll(ShangpinxinxiInfo.class);
if (!CollectionUtil.isEmpty(infoList)) {
// 处理一下空数据
List<ShangpinxinxiInfo> resultList = infoList.stream().filter(x -> ObjectUtil.isNotEmpty(x.getShangpinbianhao())).collect(Collectors.toList());
for (ShangpinxinxiInfo info : resultList) {
shangpinxinxiInfoService.add(info);
}
}
return Result.success();
}
@GetMapping("/get/shangpinxinxi_tj_shangpinleibie")
Result<List<EchartsData>> shangpinxinxi_tj_shangpinleibie() {
List<EchartsData> list = new ArrayList<>();
List<Map<String, Object>> shangpinxinxi_tj_shangpinleibieList = shangpinxinxiInfoDao.shangpinxinxi_tj_shangpinleibie();
Map<String, Double> typeMap = new HashMap<>();
for (Map<String, Object> map : shangpinxinxi_tj_shangpinleibieList) {
typeMap.put((String)map.get("aa"), (Double.valueOf((String)map.get("bb").toString())));
}
getPieData("商品信息按商品类别统计", list, typeMap);
getBarData("商品信息按商品类别统计", list, typeMap);
return Result.success(list);
}
@GetMapping("/getExcelModel")
public void getExcelModel(HttpServletResponse response) throws IOException {
// 1. 生成excel
Map<String, Object> row = new LinkedHashMap<>();
row.put("shangpinbianhao", "A商品编号");
row.put("shangpinmingcheng", "A商品名称");
row.put("shangpinleibie", "A商品类别");
row.put("jiage", "A价格");
row.put("kucun", "A库存");
row.put("xiaoliang", "A销量");
row.put("tupian", "A图片");
row.put("beizhu", "A备注");
row.put("status", "是");
row.put("level", "shangpinxinxi");
List<Map<String, Object>> list = CollUtil.newArrayList(row);
// 2. 写excel
ExcelWriter writer = ExcelUtil.getWriter(true);
writer.write(list, true);
response.setContentType("application/vnd.openxmlformats-officedocument.spreadsheetml.sheet;charset=utf-8");
response.setHeader("Content-Disposition","attachment;filename=shangpinxinxiInfoModel.xlsx");
ServletOutputStream out = response.getOutputStream();
writer.flush(out, true);
writer.close();
IoUtil.close(System.out);
}
@GetMapping("/getExcel")
public void getExcel(HttpServletResponse response) throws IOException {
// 1. 生成excel
Map<String, Object> row = new LinkedHashMap<>();
row.put("shangpinbianhao", "A商品编号");
row.put("shangpinmingcheng", "A商品名称");
row.put("shangpinleibie", "A商品类�
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
【微信小程序项目实例】惠农小店系统设计与开发(源码+说明+数据库+演示视频).zip 【项目技术】 微信小程序开发工具+mysql+java+b/s 【实现功能】 小程序的主要功能有系统首页、商品、购物车、我的。后台的主要功能包括系统用户管理、农产品新闻管理、会员等级管理、注册用户管理、农产品类别管理和商品管理和购买管理。
资源推荐
资源详情
资源评论
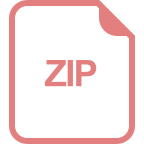
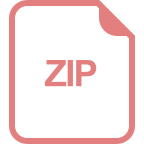
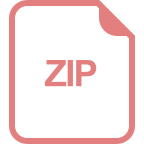
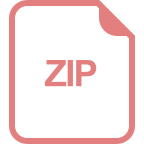
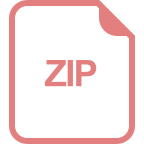
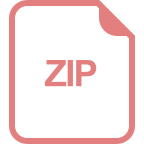
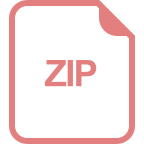
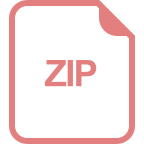
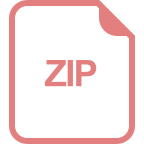
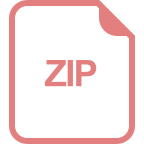
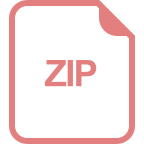
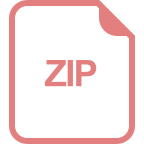
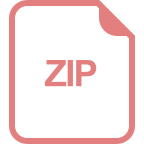
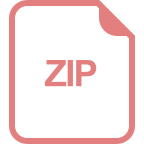
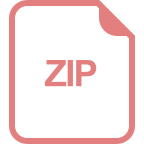
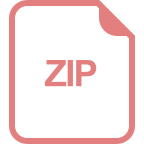
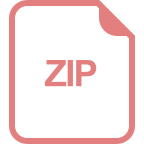
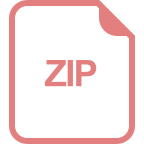
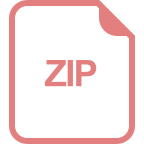
收起资源包目录

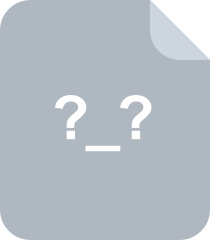
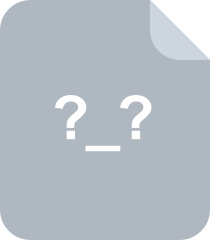
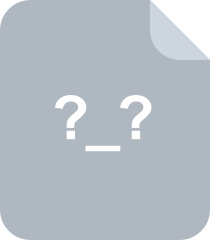
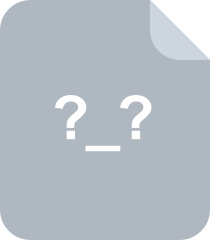
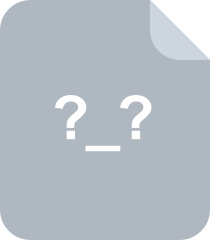
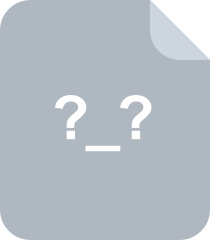
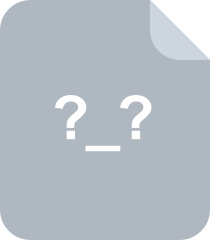
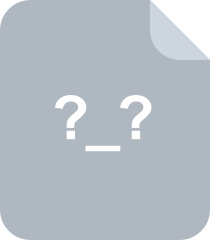
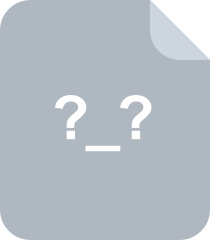
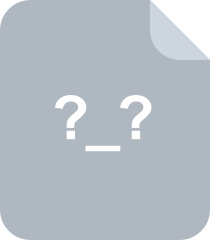
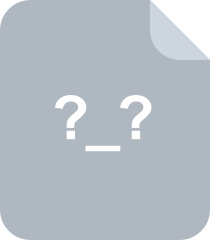
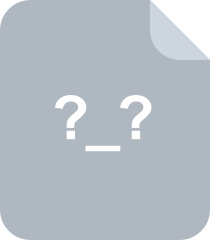
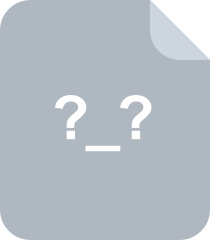
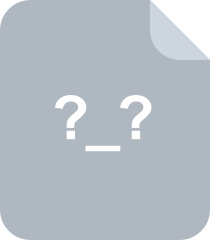
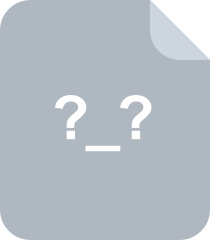
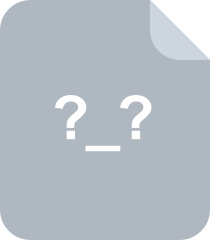
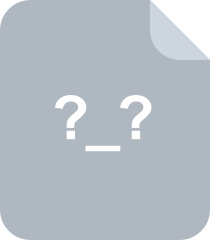
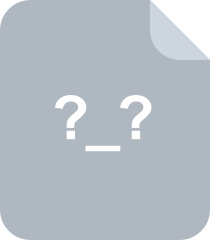
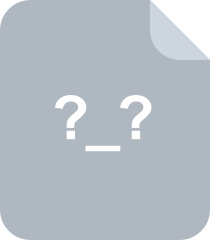
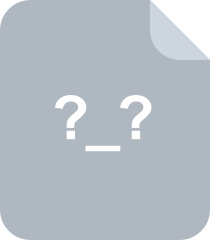
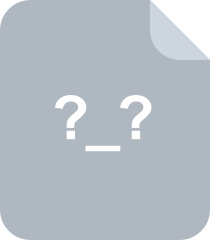
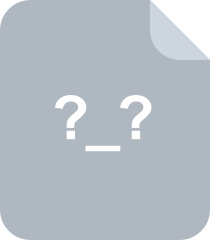
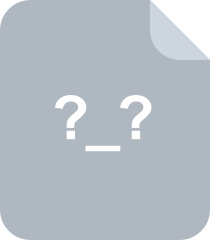
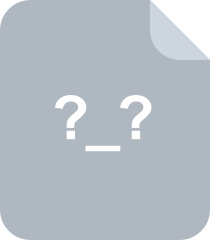
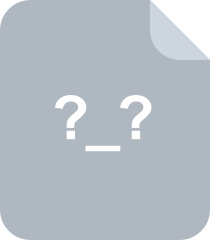
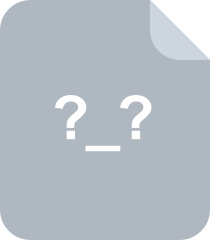
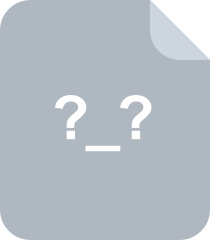
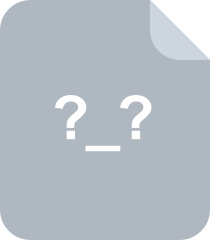
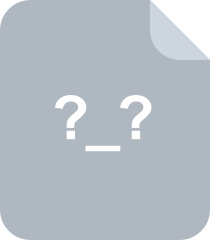
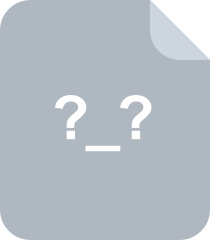
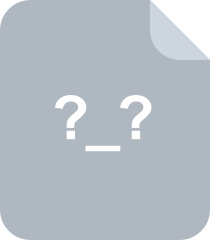
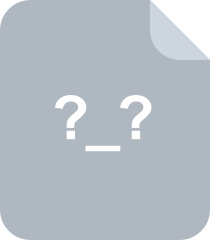
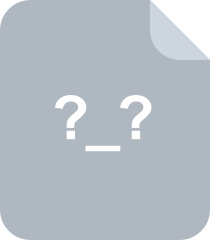
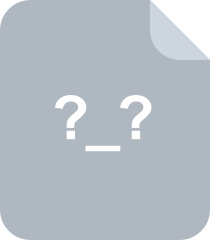
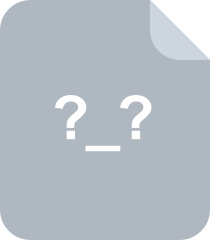
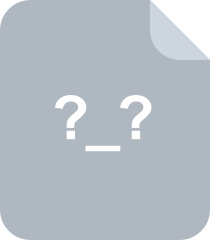
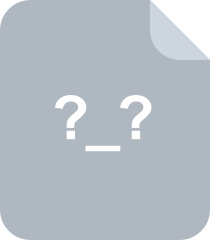
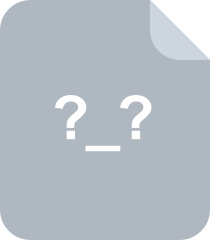
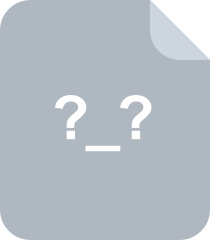
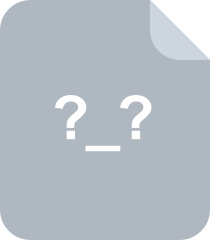
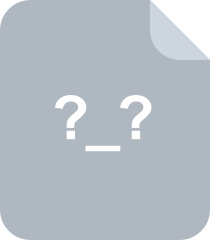
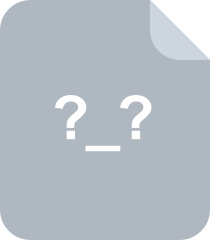
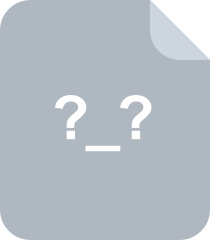
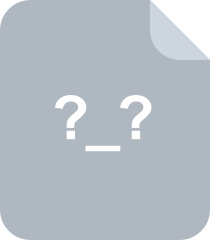
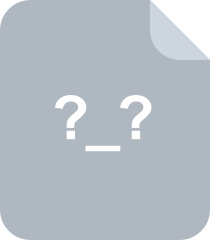
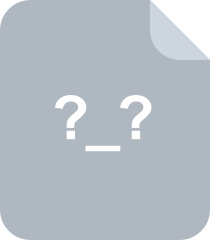
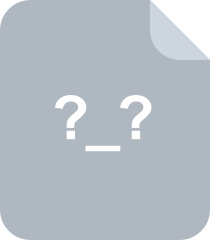
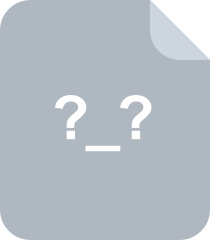
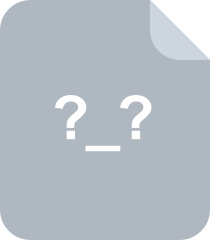
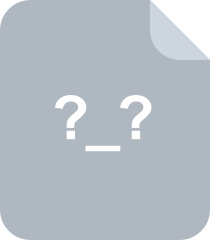
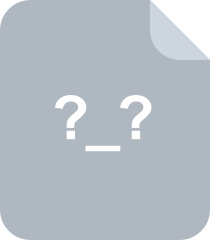
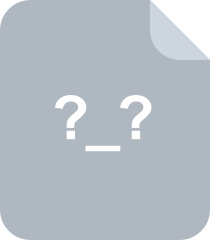
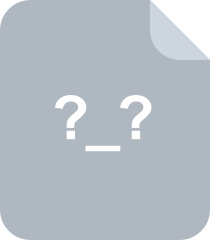
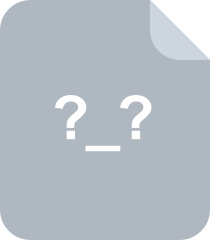
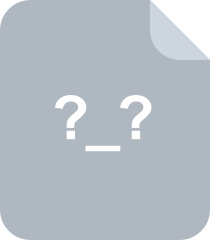
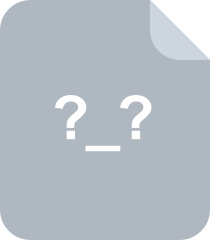
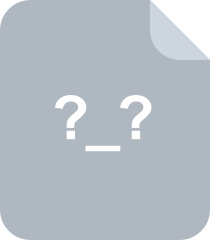
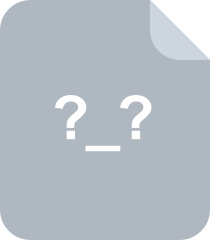
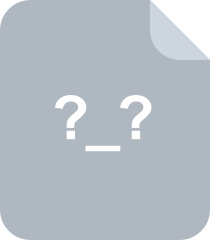
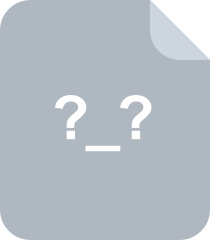
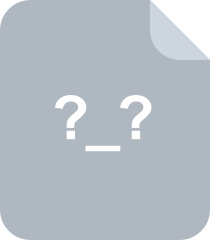
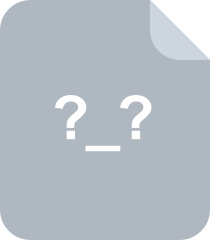
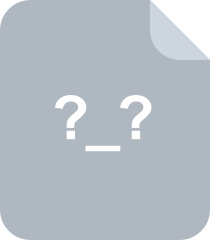
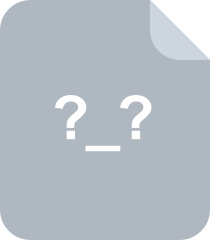
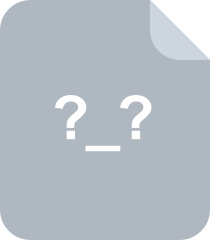
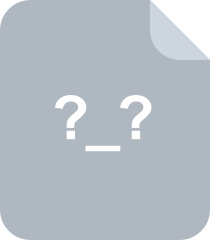
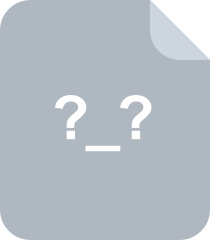
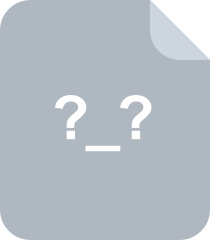
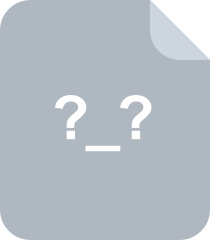
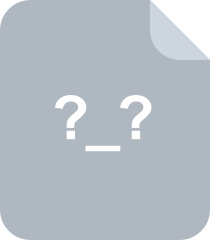
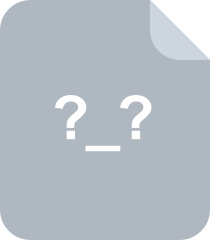
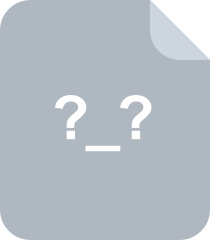
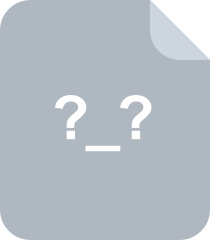
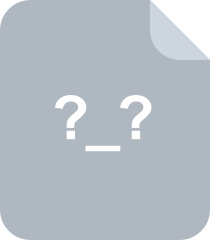
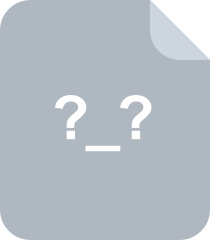
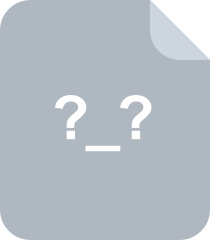
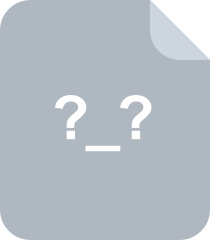
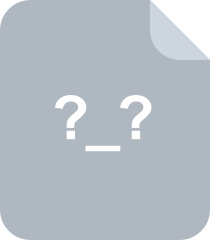
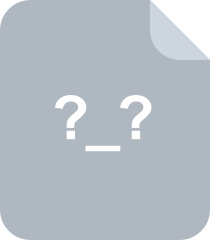
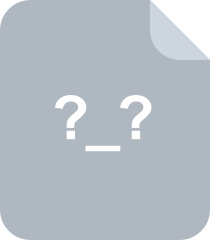
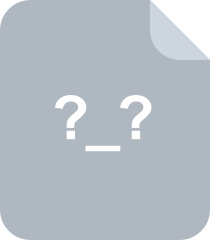
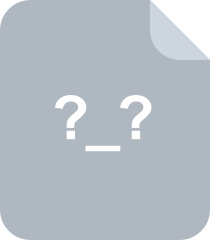
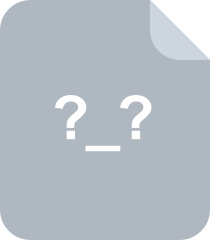
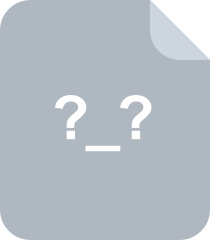
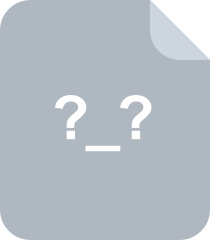
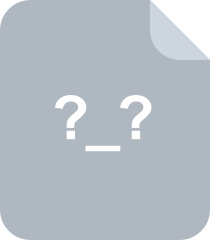
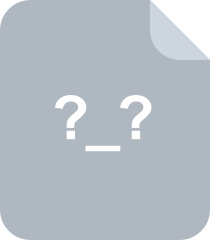
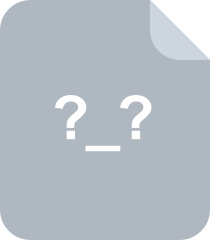
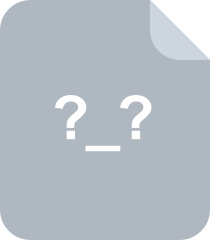
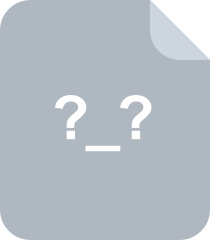
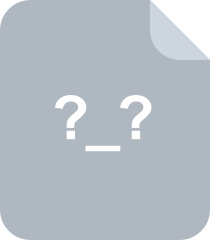
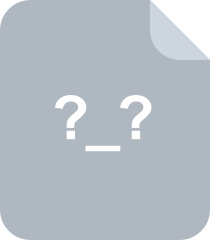
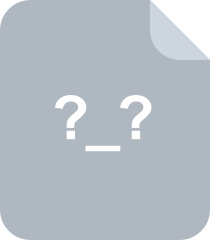
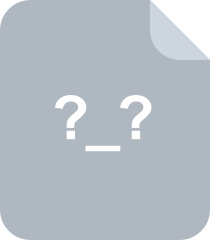
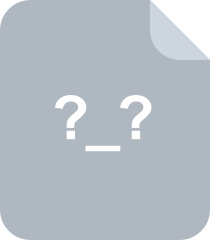
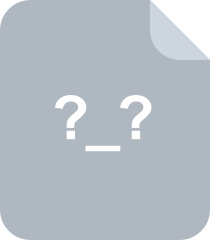
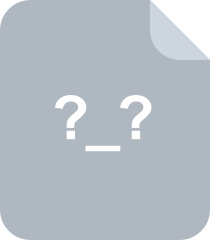
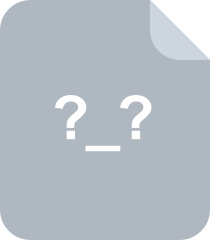
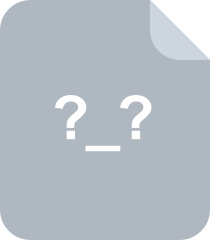
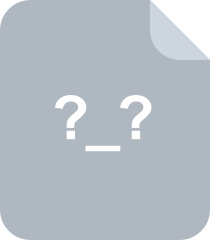
共 777 条
- 1
- 2
- 3
- 4
- 5
- 6
- 8
资源评论
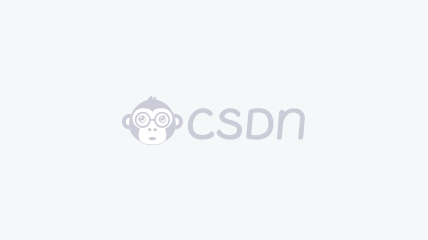

职场程序猿
- 粉丝: 6156
- 资源: 3706
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

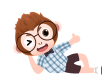
安全验证
文档复制为VIP权益,开通VIP直接复制
