package com.util;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Random;
import java.util.Set;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/*import dao.CommDAO;*/
import jxl.Workbook;
import jxl.write.Label;
import jxl.write.WritableSheet;
import jxl.write.WritableWorkbook;
import jxl.write.WriteException;
import jxl.write.biff.RowsExceededException;
public class Info {
//public static String popheight = "alliframe.height=document.body.clientHeight+";
public static String popheight = "alliframe.style.height=document.body.scrollHeight+";
public static HashMap getUser(HttpServletRequest request)
{
HashMap map = (HashMap)(request.getSession().getAttribute("admin")==null?request.getSession().getAttribute("user"):request.getSession().getAttribute("admin"));
return map;
}
public static int getBetweenDayNumber(String dateA, String dateB) {
long dayNumber = 0;
//1小时=60分钟=3600秒=3600000
long mins = 60L * 1000L;
//long day= 24L * 60L * 60L * 1000L;计算天数之差
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd HH:mm");
try {
java.util.Date d1 = df.parse(dateA);
java.util.Date d2 = df.parse(dateB);
dayNumber = (d2.getTime() - d1.getTime()) / mins;
} catch (Exception e) {
e.printStackTrace();
}
return (int) dayNumber;
}
public static void main(String[] g )
{
System.out.print(Info.getBetweenDayNumber("2012-11-11 11:19", "2012-11-11 11:11"));
}
public static String getselect(String name,String tablename,String zdname)
{
String select = "<select name=\""+name+"\" id=\""+name+"\" >";
/*for(HashMap permap:new CommDAO().select("select * from "+tablename+" order by id desc")){
select+="<option value=\""+permap.get(zdname)+"\">"+permap.get(zdname)+"</option>";
}
select+="</select>";*/
return select;
}
public static String getselect(String name,String tablename,String zdname,String where)
{
String select = "<select name=\""+name+"\" id=\""+name+"\" >";
select+="<option value=\"\">不限</option>";
/*for(HashMap permap:new CommDAO().select("select * from "+tablename+" where "+where+" order by id desc")){
String optionstr = "";
if(zdname.split(";").length==1){
optionstr=permap.get(zdname.split("~")[0]).toString();
}else{
for(String str:zdname.split(";"))
{
String zdstr = str.split("~")[0];
String zdnamestr = str.split("~")[1].equals("无名")?"":(str.split("~")[1]+":");
optionstr+=zdnamestr+permap.get(zdstr)+" - ";
}
}
if(optionstr.indexOf(" - ")>-1)optionstr=optionstr.substring(0,optionstr.length()-3);
select+="<option value=\""+optionstr+"\">"+optionstr+"</option>";
}
select+="</select>";*/
return select;
}
public static String getradio(String name,String tablename,String zdname,String where)
{
String radio="";
int dxii = 0;
/*for(HashMap permap:new CommDAO().select("select * from "+tablename+" where "+where+" order by id desc")){
String check="";
if(dxii==0)check="checked=checked";
String optionstr = "";
for(String str:zdname.split(";"))
{
String zdstr = str.split("~")[0];
String zdnamestr = str.split("~")[1].equals("无名")?"":(str.split("~")[1]+":");
optionstr+=zdnamestr+permap.get(zdstr)+" - ";
}
if(optionstr.length()>0)optionstr=optionstr.substring(0,optionstr.length()-3);
radio+="<label><input type='radio' name='"+name+"' "+check+" value=\""+optionstr+"\">"+optionstr+"</label>\n";
dxii++;
}*/
return radio;
}
public static void writeExcel(String fileName,String prosstr,java.util.List<List> plist,HttpServletRequest request, HttpServletResponse response){
WritableWorkbook wwb = null;
String cols = "";
for(String str:prosstr.split("@"))
{
cols+=str.split("-")[0]+",";
}
cols = cols.substring(0,cols.length()-1);
String where = request.getAttribute("where")==null?"":request.getAttribute("where").toString();
/* List<List> mlist = new CommDAO().selectforlist("select "+cols+" from "+fileName+" "+where+" order by id desc");*/
fileName = request.getRealPath("/")+"/upfile/"+Info.generalFileName("a.xls");
String[] pros = prosstr.split("@");
try {
//首先要使用Workbook类的工厂方法创建一个可写入的工作薄(Workbook)对象
wwb = Workbook.createWorkbook(new File(fileName));
} catch (IOException e) {
e.printStackTrace();
}
if(wwb!=null){
//创建一个可写入的工作表
//Workbook的createSheet方法有两个参数,第一个是工作表的名称,第二个是工作表在工作薄中的位置
WritableSheet ws = wwb.createSheet("sheet1", 0);
ws.setColumnView(0,20);
ws.setColumnView(1,20);
ws.setColumnView(2,20);
ws.setColumnView(3,20);
ws.setColumnView(4,20);
ws.setColumnView(5,20);
try {
for(int i=0;i<pros.length;i++)
{
Label label1 = new Label(i, 0,"");
label1.setString(pros[i]);
ws.addCell(label1);
}
} catch (RowsExceededException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
} catch (WriteException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
//下面开始添加单元格
/*int i=1;*/
/* for(List t:mlist){
try {
Iterator it = t.iterator();
int jj=0;
while(it.hasNext())
{
Label label1 = new Label(jj, i,"");
String a = it.next().toString();
label1.setString(a);
ws.addCell(label1);
jj++;
}
i++;
} catch (RowsExceededException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
} catch (WriteException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
}
try {
//从内存中写入文件中
wwb.write();
//关闭资源,释放内存
wwb.close();
} catch (IOException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
try {
response.sendRedirect("/pianotrainwebstie/upload?filename="+fileName.substring(fileName.lastIndexOf("/")+1));
} catch (IOException e) {
e.printStackTrace();*/
}
}
public static String getcheckbox(String name,String tablename,String zdname,String where)
{
String checkbox="";
/* f
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于Java+SSM的商品信息分类管理系统毕业设计(源码+说明+演示视频).zip 【项目技术】 java+mysql+ssm+b/s 【实现功能】 一、系统管理员 1)系统管理员可以定期修改自己的登录密码,以确保系统的安全性得到保障; 2)系统管理员可以对本系统中的员工用户进行添加和信息管理; 3)系统管理员可以管理本系统中的商品类别信息及商品详细信息; 4)系统管理员可以根据商品的销售情况进行缺货申请或者停止上货的安排; 5)系统管理员可以对系统中的商品数据及员工数据进行报表的统计和导出。 二、员工用户 1)员工用户可以在符合企业各种规定的前提下进行账户的注册和登录; 2)员工用户可以通过个人后台界面对自己的个人信息进行修改; 3)员工用户可以在个人后台界面中查看商品信息并可以对其进行转移、入库、缺货及停止进货等操作; 4)员工用户在使用本系统时添加商品的入库、缺货及停止进货等信息; 5)员工用户可以进行商品信息及商品入库等信息的打印及文件导出。
资源推荐
资源详情
资源评论
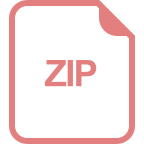
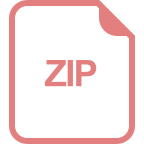
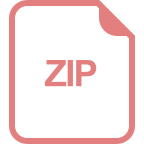
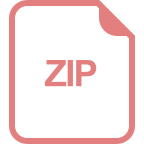
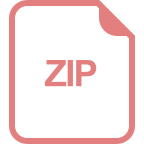
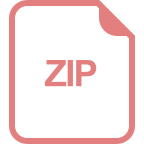
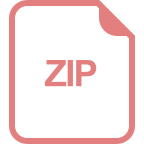
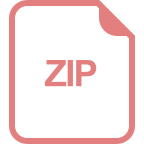
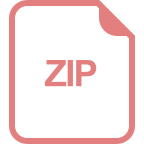
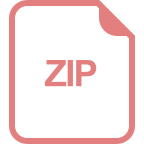
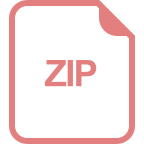
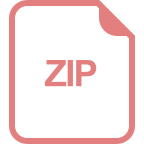
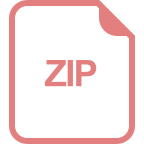
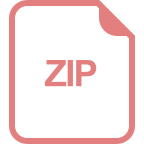
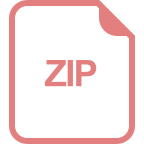
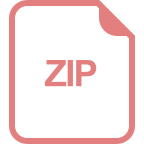
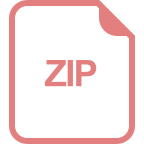
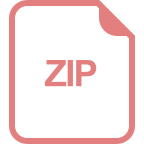
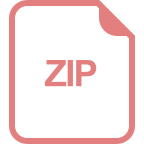
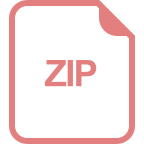
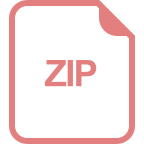
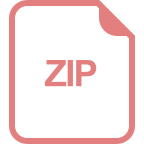
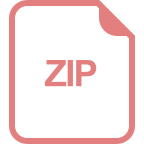
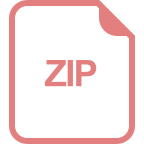
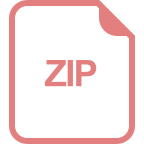
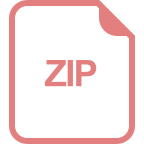
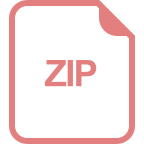
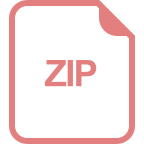
收起资源包目录

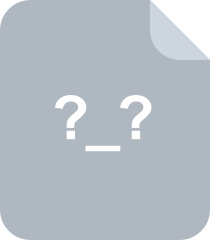
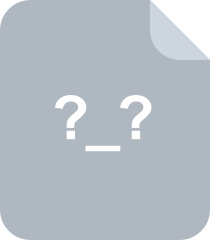
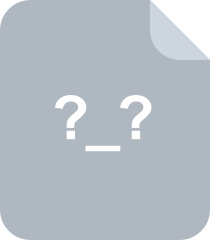
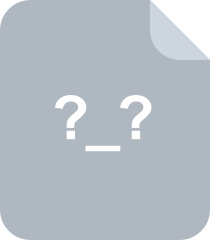
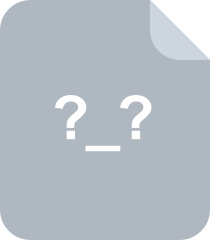
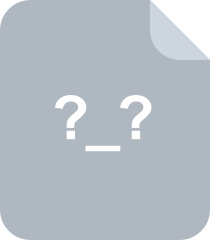
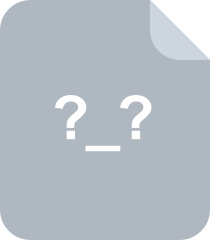
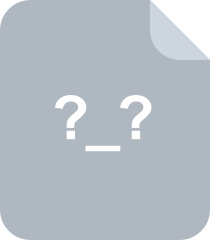
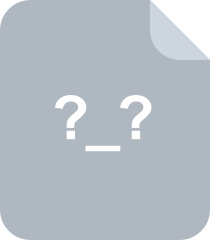
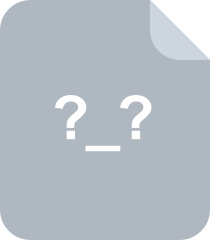
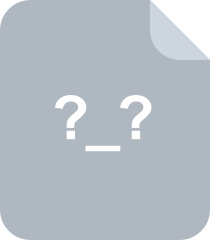
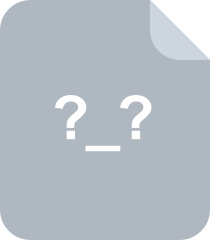
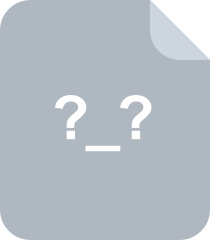
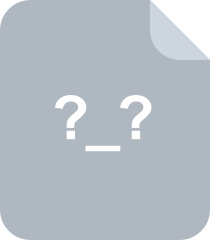
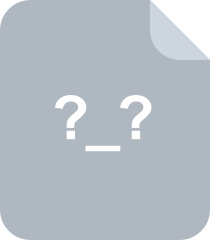
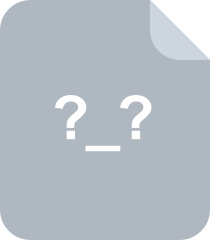
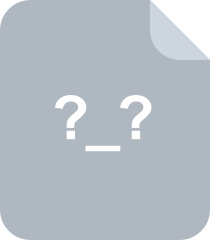
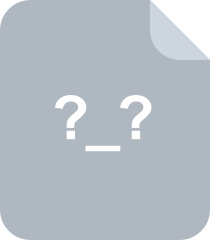
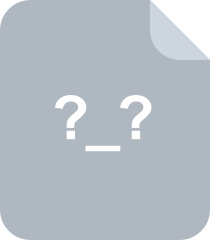
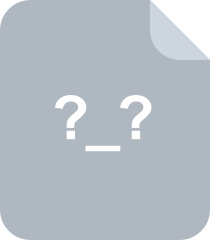
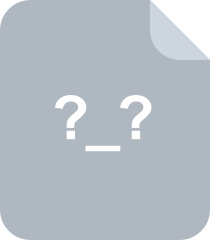
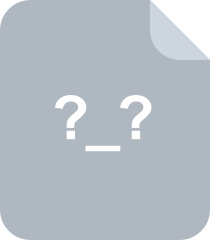
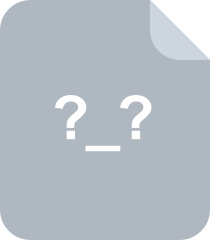
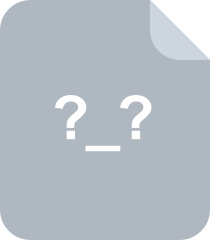
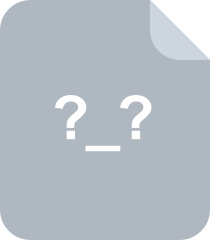
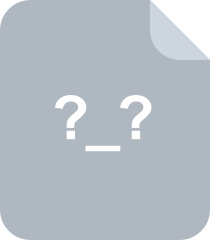
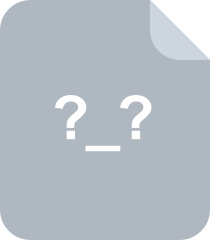
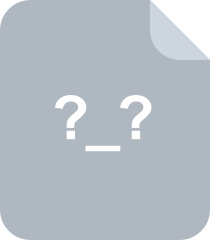
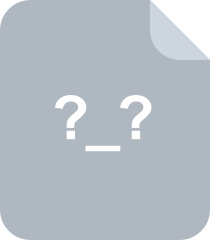
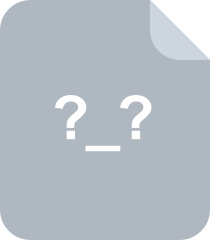
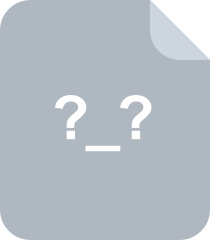
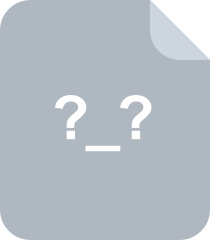
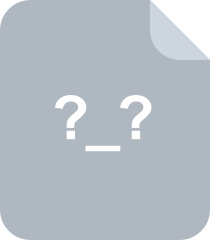
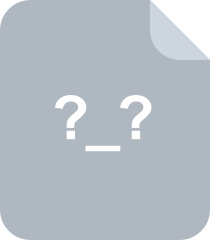
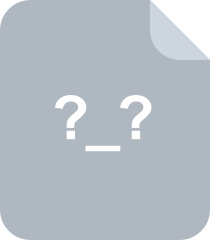
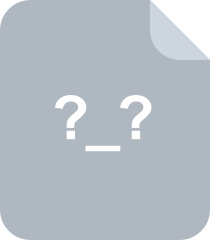
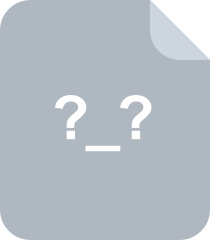
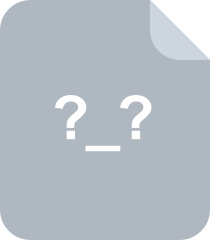
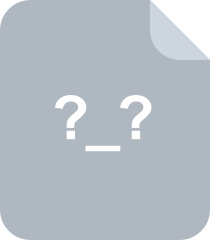
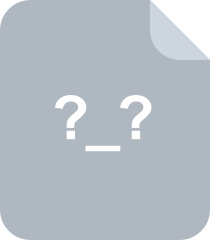
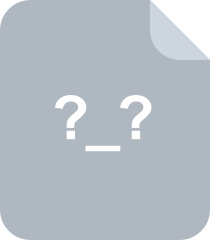
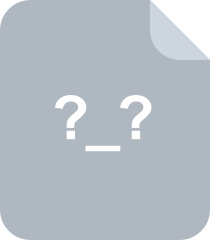
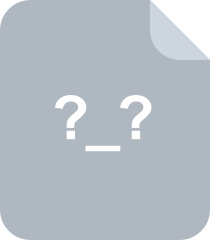
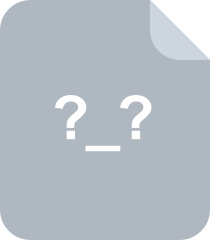
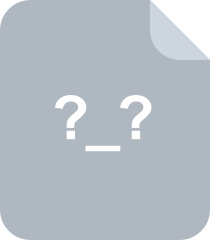
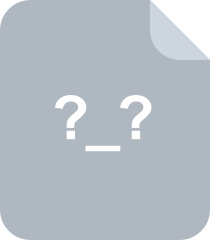
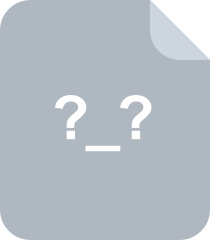
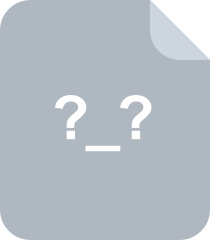
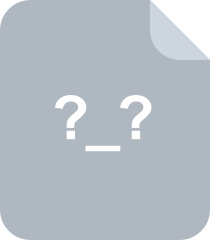
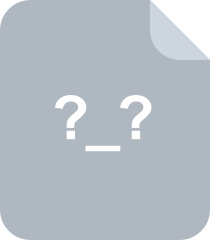
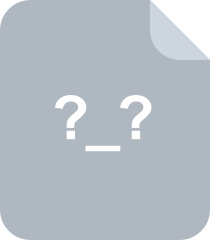
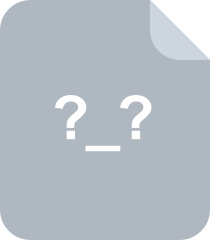
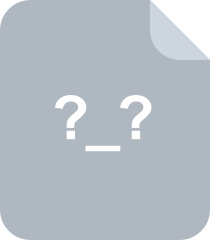
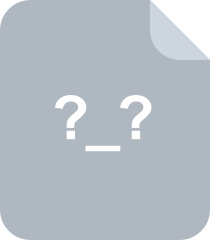
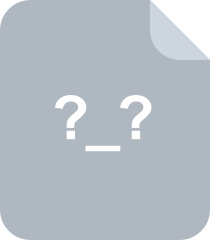
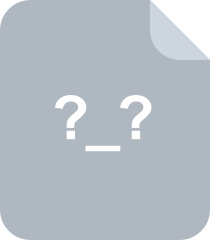
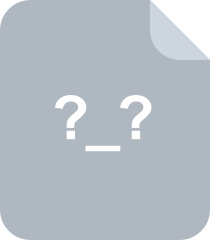
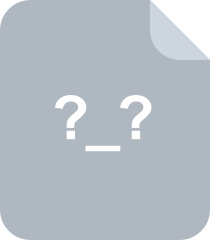
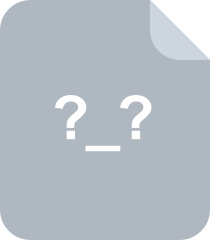
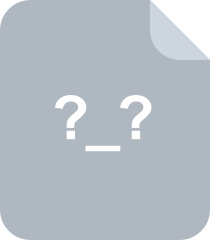
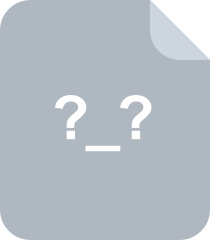
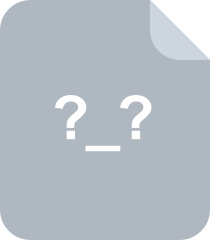
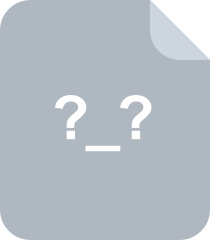
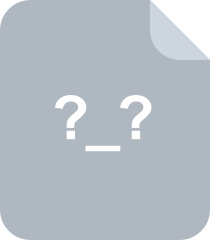
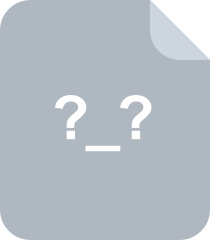
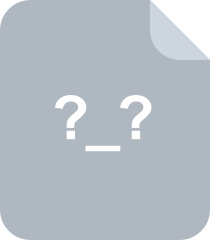
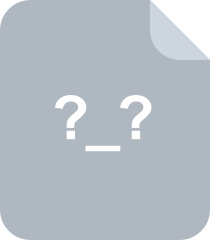
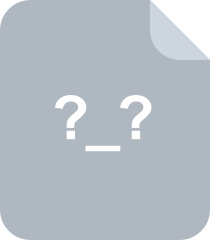
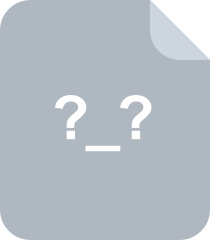
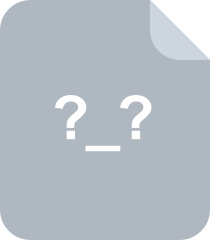
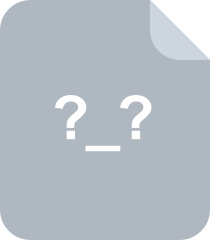
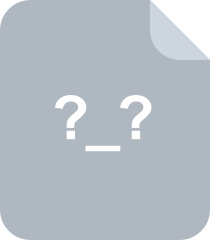
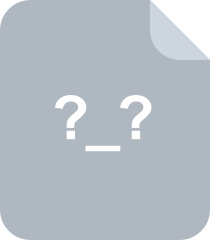
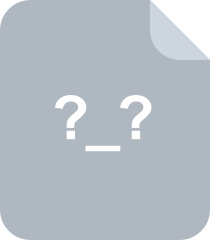
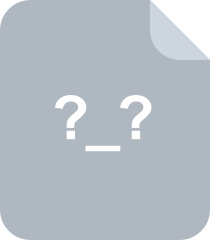
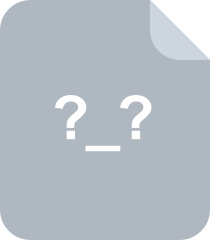
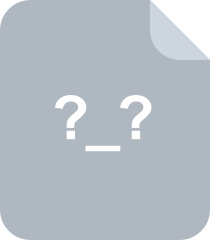
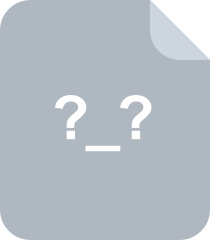
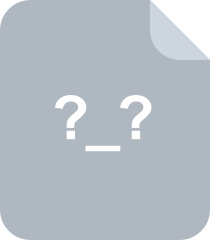
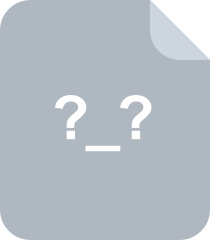
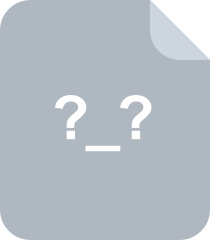
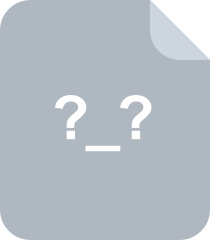
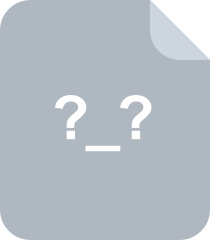
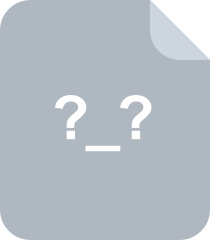
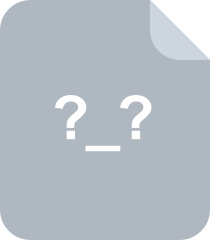
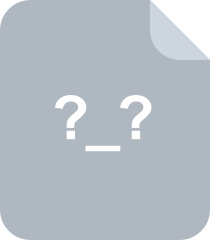
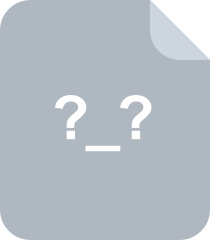
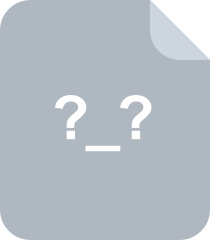
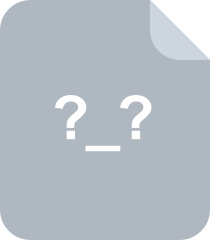
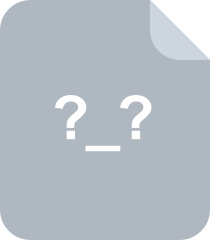
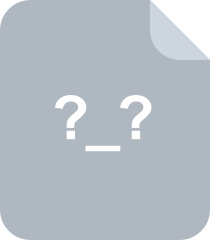
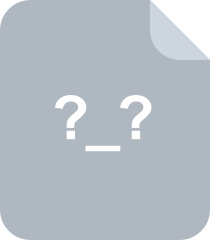
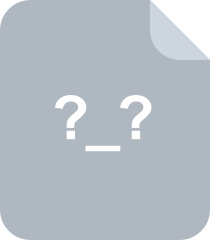
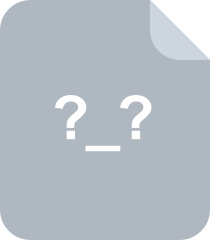
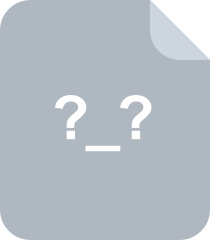
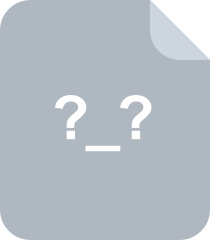
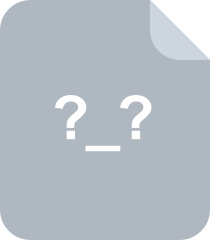
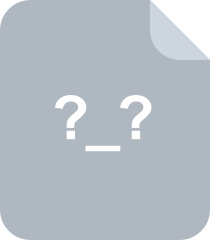
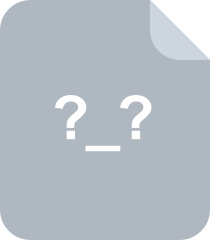
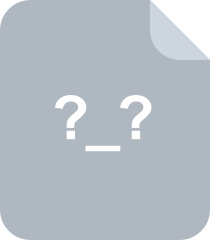
共 518 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
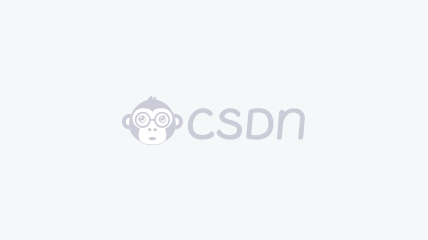

职场程序猿
- 粉丝: 6451
- 资源: 3706
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

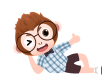
最新资源
- 打卡没事,等你打完看到吗水浇地
- 电力变压器中的故障分析数据集
- 车载软件平台:面向未来的新型ADAS架构推动零事故愿景
- pytorch实现基于LSTM的高速公路车辆轨迹预测源码+数据集+说明(高分项目)
- pytorch实现基于LSTM的高速公路车辆轨迹预测源码+数据集+说明
- Java 飞机订票系统实训报告
- 教育领域的新年主题环创:从幼儿园到中学的创意布置与新年活动设计
- 智能小车路径规划 算法:RRT与Dubins相结合的方法,混合A*与Dubins相结合的方法 实现智能小车最短路径规划
- 枸杞虫害图像分类数据集【已标注,约9,000张数据】
- 基于Python(tkinter)+sqlite3的图书信息管理系统源码+数据库(高分课程设计)
- FOC+SMO+PLL的Simlink仿真模型
- 基于Java 和MySQL的飞机订票系统
- 信号处理辅助软件课程设计报告 信号处理辅助软件中GUIDE工具的计算器和绘图程序的设计与实现
- OpenCV计算机视觉基础 ppt(非教材自带ppt)
- 三相异步电机矢量控制仿真模型
- 多模态应用-基于BERT+ResNet的多种融合方式实现多模态的情感分析源码(高分大作业&课设)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


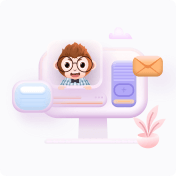
安全验证
文档复制为VIP权益,开通VIP直接复制
