# postinstall script for pywin32
#
# copies PyWinTypesxx.dll and PythonCOMxx.dll into the system directory,
# and creates a pth file
import glob
import os
import shutil
import sys
import sysconfig
try:
import winreg as winreg
except:
import winreg
# Send output somewhere so it can be found if necessary...
import tempfile
tee_f = open(os.path.join(tempfile.gettempdir(), "pywin32_postinstall.log"), "w")
class Tee:
def __init__(self, file):
self.f = file
def write(self, what):
if self.f is not None:
try:
self.f.write(what.replace("\n", "\r\n"))
except IOError:
pass
tee_f.write(what)
def flush(self):
if self.f is not None:
try:
self.f.flush()
except IOError:
pass
tee_f.flush()
# For some unknown reason, when running under bdist_wininst we will start up
# with sys.stdout as None but stderr is hooked up. This work-around allows
# bdist_wininst to see the output we write and display it at the end of
# the install.
if sys.stdout is None:
sys.stdout = sys.stderr
sys.stderr = Tee(sys.stderr)
sys.stdout = Tee(sys.stdout)
com_modules = [
# module_name, class_names
("win32com.servers.interp", "Interpreter"),
("win32com.servers.dictionary", "DictionaryPolicy"),
("win32com.axscript.client.pyscript", "PyScript"),
]
# Is this a 'silent' install - ie, avoid all dialogs.
# Different than 'verbose'
silent = 0
# Verbosity of output messages.
verbose = 1
root_key_name = "Software\\Python\\PythonCore\\" + sys.winver
try:
# When this script is run from inside the bdist_wininst installer,
# file_created() and directory_created() are additional builtin
# functions which write lines to Python23\pywin32-install.log. This is
# a list of actions for the uninstaller, the format is inspired by what
# the Wise installer also creates.
file_created
is_bdist_wininst = True
except NameError:
is_bdist_wininst = False # we know what it is not - but not what it is :)
def file_created(file):
pass
def directory_created(directory):
pass
def get_root_hkey():
try:
winreg.OpenKey(
winreg.HKEY_LOCAL_MACHINE, root_key_name, 0, winreg.KEY_CREATE_SUB_KEY
)
return winreg.HKEY_LOCAL_MACHINE
except OSError:
# Either not exist, or no permissions to create subkey means
# must be HKCU
return winreg.HKEY_CURRENT_USER
try:
create_shortcut
except NameError:
# Create a function with the same signature as create_shortcut provided
# by bdist_wininst
def create_shortcut(
path, description, filename, arguments="", workdir="", iconpath="", iconindex=0
):
import pythoncom
from win32com.shell import shell
ilink = pythoncom.CoCreateInstance(
shell.CLSID_ShellLink,
None,
pythoncom.CLSCTX_INPROC_SERVER,
shell.IID_IShellLink,
)
ilink.SetPath(path)
ilink.SetDescription(description)
if arguments:
ilink.SetArguments(arguments)
if workdir:
ilink.SetWorkingDirectory(workdir)
if iconpath or iconindex:
ilink.SetIconLocation(iconpath, iconindex)
# now save it.
ipf = ilink.QueryInterface(pythoncom.IID_IPersistFile)
ipf.Save(filename, 0)
# Support the same list of "path names" as bdist_wininst.
def get_special_folder_path(path_name):
from win32com.shell import shell, shellcon
for maybe in """
CSIDL_COMMON_STARTMENU CSIDL_STARTMENU CSIDL_COMMON_APPDATA
CSIDL_LOCAL_APPDATA CSIDL_APPDATA CSIDL_COMMON_DESKTOPDIRECTORY
CSIDL_DESKTOPDIRECTORY CSIDL_COMMON_STARTUP CSIDL_STARTUP
CSIDL_COMMON_PROGRAMS CSIDL_PROGRAMS CSIDL_PROGRAM_FILES_COMMON
CSIDL_PROGRAM_FILES CSIDL_FONTS""".split():
if maybe == path_name:
csidl = getattr(shellcon, maybe)
return shell.SHGetSpecialFolderPath(0, csidl, False)
raise ValueError("%s is an unknown path ID" % (path_name,))
def CopyTo(desc, src, dest):
import win32api
import win32con
while 1:
try:
win32api.CopyFile(src, dest, 0)
return
except win32api.error as details:
if details.winerror == 5: # access denied - user not admin.
raise
if silent:
# Running silent mode - just re-raise the error.
raise
full_desc = (
"Error %s\n\n"
"If you have any Python applications running, "
"please close them now\nand select 'Retry'\n\n%s"
% (desc, details.strerror)
)
rc = win32api.MessageBox(
0, full_desc, "Installation Error", win32con.MB_ABORTRETRYIGNORE
)
if rc == win32con.IDABORT:
raise
elif rc == win32con.IDIGNORE:
return
# else retry - around we go again.
# We need to import win32api to determine the Windows system directory,
# so we can copy our system files there - but importing win32api will
# load the pywintypes.dll already in the system directory preventing us
# from updating them!
# So, we pull the same trick pywintypes.py does, but it loads from
# our pywintypes_system32 directory.
def LoadSystemModule(lib_dir, modname):
# See if this is a debug build.
import importlib.machinery
import importlib.util
suffix = "_d" if "_d.pyd" in importlib.machinery.EXTENSION_SUFFIXES else ""
filename = "%s%d%d%s.dll" % (
modname,
sys.version_info[0],
sys.version_info[1],
suffix,
)
filename = os.path.join(lib_dir, "pywin32_system32", filename)
loader = importlib.machinery.ExtensionFileLoader(modname, filename)
spec = importlib.machinery.ModuleSpec(name=modname, loader=loader, origin=filename)
mod = importlib.util.module_from_spec(spec)
spec.loader.exec_module(mod)
def SetPyKeyVal(key_name, value_name, value):
root_hkey = get_root_hkey()
root_key = winreg.OpenKey(root_hkey, root_key_name)
try:
my_key = winreg.CreateKey(root_key, key_name)
try:
winreg.SetValueEx(my_key, value_name, 0, winreg.REG_SZ, value)
if verbose:
print("-> %s\\%s[%s]=%r" % (root_key_name, key_name, value_name, value))
finally:
my_key.Close()
finally:
root_key.Close()
def UnsetPyKeyVal(key_name, value_name, delete_key=False):
root_hkey = get_root_hkey()
root_key = winreg.OpenKey(root_hkey, root_key_name)
try:
my_key = winreg.OpenKey(root_key, key_name, 0, winreg.KEY_SET_VALUE)
try:
winreg.DeleteValue(my_key, value_name)
if verbose:
print("-> DELETE %s\\%s[%s]" % (root_key_name, key_name, value_name))
finally:
my_key.Close()
if delete_key:
winreg.DeleteKey(root_key, key_name)
if verbose:
print("-> DELETE %s\\%s" % (root_key_name, key_name))
except OSError as why:
winerror = getattr(why, "winerror", why.errno)
if winerror != 2: # file not found
raise
finally:
root_key.Close()
def RegisterCOMObjects(register=True):
import win32com.server.register
if register:
func = win32com.server.register.RegisterClasses
else:
func = win32com.server.register.UnregisterClasses
flags = {}
if not verbose:
flags["quiet"] = 1
for module, klass_name in com_modules:
__import__(module)
mod = sys.modules[module]
flags["finalize_register"] = getattr(mod, "DllRegisterServer", None)
flags["finalize_unregi

程序员柳
- 粉丝: 8324
- 资源: 1469
最新资源
- 机械设计在线打标机锂电类sw17可编辑项目全套技术资料.zip
- 机械设计在线式方壳打标机sw17可编辑项目全套技术资料.zip
- C语言入门到高级实例源码
- Spirent-TestCenter-Automation-Overview-Manual
- 机械设计圆剪纸机sw18项目全套技术资料.zip
- 基于springboot的景区民宿预约系统的设计与实现源码(java毕业设计完整源码+LW).zip
- 基于springboot的社区智慧养老监护管理平台设计与实现源码(java毕业设计完整源码+LW).zip
- 基于springboot的公交线路查询系统源码(java毕业设计完整源码+LW).zip
- 图像加密解密技术及应用场景详细介绍.zip
- 基于springboot的体育馆管理系统的设计与实现源码(java毕业设计完整源码+LW).zip
- 4ad004-基于Android的实时健康感知系统_springboot+vue.zip
- 4ad003-健康饮食APP_springboot+vue+android.zip
- google浏览器插件下载postwoman1.2.3插件
- 基于springboot的美食推荐商城的设计与实现源码(java毕业设计完整源码+LW).zip
- 光伏发电三相并网 光伏加+Boost+三相并网逆变器 PLL锁相环 MPPT最大功率点跟踪控制(扰动观察法) dq解耦控制, 电流内环电压外环的并网控制策略 电压外环控制直流母线电压稳住750V TH
- 基于springboot的党员教育和管理系统源码(java毕业设计完整源码+LW).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


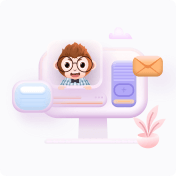