package com.controller;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.*;
import javax.servlet.http.HttpServletRequest;
import com.alibaba.fastjson.JSON;
import com.utils.StringUtil;
import org.apache.commons.lang3.StringUtils;
import org.json.JSONObject;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.util.ResourceUtils;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.annotation.IgnoreAuth;
import com.baidu.aip.face.AipFace;
import com.baidu.aip.face.MatchRequest;
import com.baidu.aip.util.Base64Util;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.baomidou.mybatisplus.mapper.Wrapper;
import com.entity.ConfigEntity;
import com.service.CommonService;
import com.service.ConfigService;
import com.utils.BaiduUtil;
import com.utils.FileUtil;
import com.utils.R;
/**
* 通用接口
*/
@RestController
public class CommonController{
private static final Logger logger = LoggerFactory.getLogger(CommonController.class);
@Autowired
private CommonService commonService;
@Autowired
private ConfigService configService;
private static AipFace client = null;
private static String BAIDU_DITU_AK = null;
@RequestMapping("/location")
public R location(String lng,String lat) {
if(BAIDU_DITU_AK==null) {
BAIDU_DITU_AK = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "baidu_ditu_ak")).getValue();
if(BAIDU_DITU_AK==null) {
return R.error("请在配置管理中正确配置baidu_ditu_ak");
}
}
Map<String, String> map = BaiduUtil.getCityByLonLat(BAIDU_DITU_AK, lng, lat);
return R.ok().put("data", map);
}
/**
* 人脸比对
*
* @param face1 人脸1
* @param face2 人脸2
* @return
*/
@RequestMapping("/matchFace")
public R matchFace(String face1, String face2, HttpServletRequest request) {
if(client==null) {
/*String AppID = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "AppID")).getValue();*/
String APIKey = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "APIKey")).getValue();
String SecretKey = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "SecretKey")).getValue();
String token = BaiduUtil.getAuth(APIKey, SecretKey);
if(token==null) {
return R.error("请在配置管理中正确配置APIKey和SecretKey");
}
client = new AipFace(null, APIKey, SecretKey);
client.setConnectionTimeoutInMillis(2000);
client.setSocketTimeoutInMillis(60000);
}
JSONObject res = null;
try {
File file1 = new File(request.getSession().getServletContext().getRealPath("/upload")+"/"+face1);
File file2 = new File(request.getSession().getServletContext().getRealPath("/upload")+"/"+face2);
String img1 = Base64Util.encode(FileUtil.FileToByte(file1));
String img2 = Base64Util.encode(FileUtil.FileToByte(file2));
MatchRequest req1 = new MatchRequest(img1, "BASE64");
MatchRequest req2 = new MatchRequest(img2, "BASE64");
ArrayList<MatchRequest> requests = new ArrayList<MatchRequest>();
requests.add(req1);
requests.add(req2);
res = client.match(requests);
System.out.println(res.get("result"));
} catch (FileNotFoundException e) {
e.printStackTrace();
return R.error("文件不存在");
} catch (IOException e) {
e.printStackTrace();
}
return R.ok().put("data", com.alibaba.fastjson.JSONObject.parse(res.get("result").toString()));
}
/**
* 获取table表中的column列表(联动接口)
* @return
*/
@RequestMapping("/option/{tableName}/{columnName}")
@IgnoreAuth
public R getOption(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName,String level,String parent) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableName);
params.put("column", columnName);
if(StringUtils.isNotBlank(level)) {
params.put("level", level);
}
if(StringUtils.isNotBlank(parent)) {
params.put("parent", parent);
}
List<String> data = commonService.getOption(params);
return R.ok().put("data", data);
}
/**
* 根据table中的column获取单条记录
* @return
*/
@RequestMapping("/follow/{tableName}/{columnName}")
@IgnoreAuth
public R getFollowByOption(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName, @RequestParam String columnValue) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableName);
params.put("column", columnName);
params.put("columnValue", columnValue);
Map<String, Object> result = commonService.getFollowByOption(params);
return R.ok().put("data", result);
}
/**
* 修改table表的sfsh状态
* @param map
* @return
*/
@RequestMapping("/sh/{tableName}")
public R sh(@PathVariable("tableName") String tableName, @RequestBody Map<String, Object> map) {
map.put("table", tableName);
commonService.sh(map);
return R.ok();
}
/**
* 获取需要提醒的记录数
* @param tableName
* @param columnName
* @param type 1:数字 2:日期
* @param map
* @return
*/
@RequestMapping("/remind/{tableName}/{columnName}/{type}")
@IgnoreAuth
public R remindCount(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName,
@PathVariable("type") String type,@RequestParam Map<String, Object> map) {
map.put("table", tableName);
map.put("column", columnName);
map.put("type", type);
if(type.equals("2")) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Calendar c = Calendar.getInstance();
Date remindStartDate = null;
Date remindEndDate = null;
if(map.get("remindstart")!=null) {
Integer remindStart = Integer.parseInt(map.get("remindstart").toString());
c.setTime(new Date());
c.add(Calendar.DAY_OF_MONTH,remindStart);
remindStartDate = c.getTime();
map.put("remindstart", sdf.format(remindStartDate));
}
if(map.get("remindend")!=null) {
Integer remindEnd = Integer.parseInt(map.get("remindend").toString());
c.setTime(new Date());
c.add(Calendar.DAY_OF_MONTH,remindEnd);
remindEndDate = c.getTime();
map.put("remindend", sdf.format(remindEndDate));
}
}
int count = commonService.remindCount(map);
return R.ok().put("count", count);
}
/**
* 圖表统计
*/
@IgnoreAuth
@RequestMapping("/group/{tableName}")
public R group1(@PathVariable("tableName") String tableName, @RequestParam Map<String,Object> params) {
params.put("table1", tableName);
List<Map<String, Object>> result = commonService.chartBoth(params);
return R.ok().put("data", result);
}
/**
* 单列求和
*/
@RequestMapping("/cal/{tableName}/{columnName}")
@IgnoreAuth
public R cal(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableName);
params.put("column", columnName);
Map<String, Object> result = commonService.selectCal(params);
return R.ok().put("data", result);
}
/**
* 分组统计
*/
@RequestMapping("/group/{tableName}/{columnName}")
@IgnoreAuth
public R group(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableN
没有合适的资源?快使用搜索试试~ 我知道了~
毕业论文管理-JAVA-基于springboot-vue的毕业论文管理系统设计与实现(毕业论文)
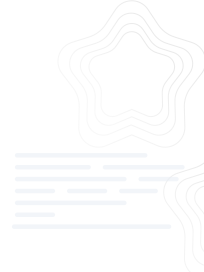
共445个文件
svg:161个
java:117个
vue:68个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 81 浏览量
2025-01-09
19:51:50
上传
评论
收藏 18.71MB RAR 举报
温馨提示
毕业论文管理系统旨在为高校学生、教师和管理人员提供一个高效、便捷的论文管理平台。以下是该系统的主要功能描述: 1. 用户管理 注册与登录:支持学生、教师和管理员的注册与登录,确保身份验证和数据安全。 角色分配:根据用户角色(如学生、指导教师、管理员)授予不同权限。 2. 论文选题与指导 选题发布:教师可以发布可供选择的论文选题,学生可浏览并申请。 选题申请与审核:学生申请特定选题后,教师可进行审核和确认。 指导记录:教师可记录指导过程中的交流和建议,帮助学生完善论文。 3. 论文提交与管理 论文上传:学生可在线提交论文草稿及最终版本,支持多种文件格式。 版本控制:系统自动记录论文的不同版本,方便学生随时查看和修改。 论文状态跟踪:学生可实时查询论文审核状态,如待审、已审、退回等。 4. 查重与审核 论文查重:集成查重工具,对提交的论文进行相似度检测,确保学术诚信。 审核反馈:教师可对论文进行评审和反馈,提出修改意见。 5. 成绩管理 评分系统:教师可在线为每篇论文打分,并输入评语,系统自动统计总分。 成绩公示:完成的论文成绩可在系统中公示,方便学生查询。 6. 文献管理 参考文献管理
资源推荐
资源详情
资源评论
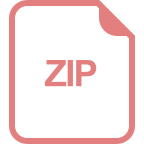
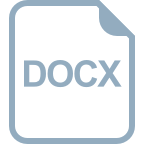
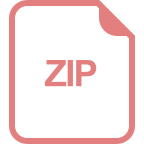
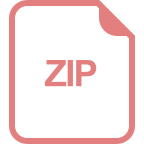
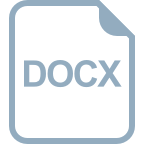
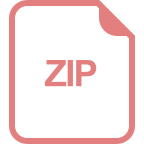
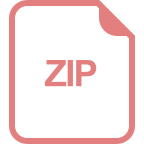
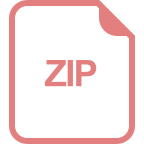
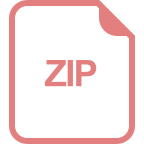
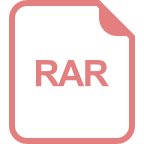
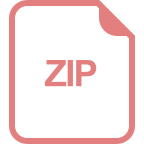
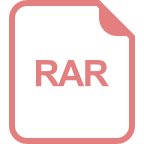
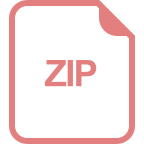
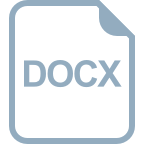
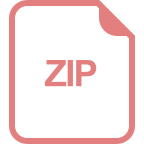
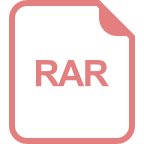
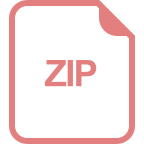
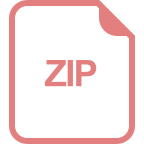
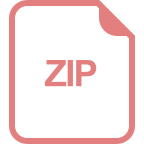
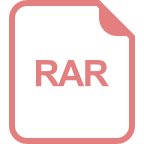
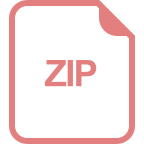
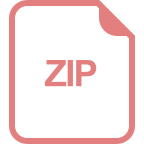
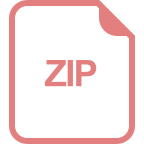
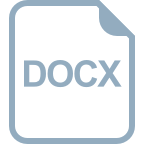
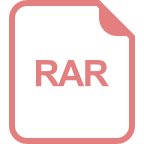
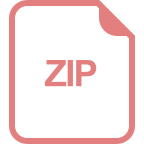
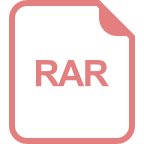
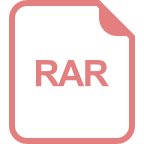
收起资源包目录

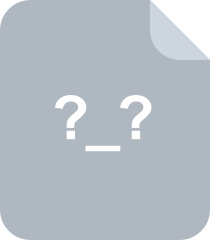
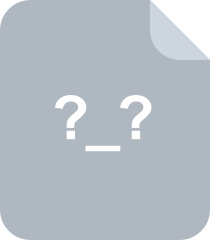
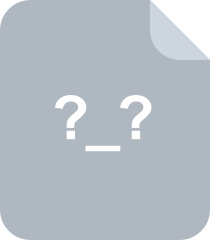
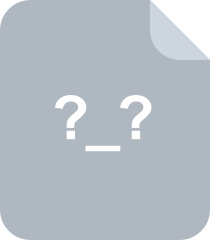
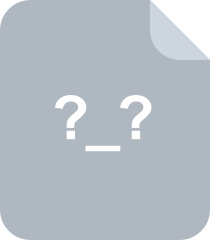
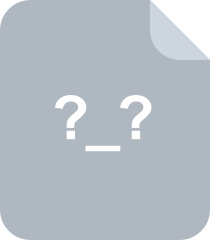
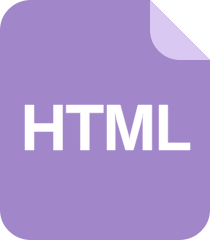
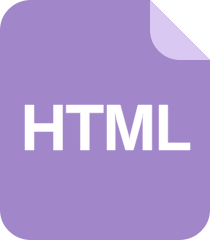
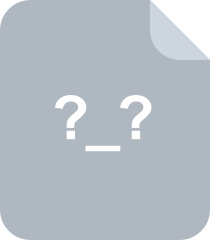
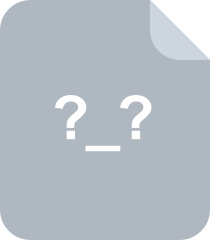
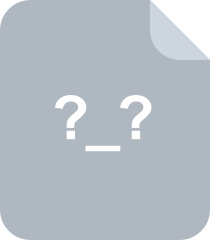
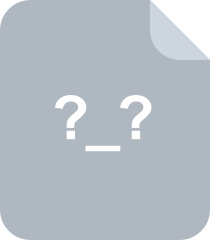
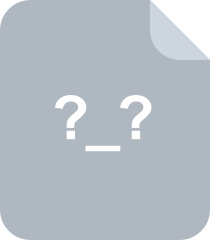
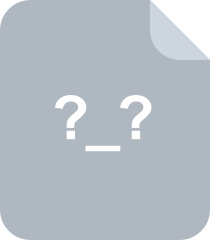
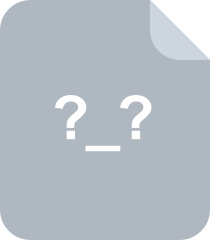
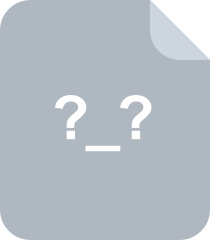
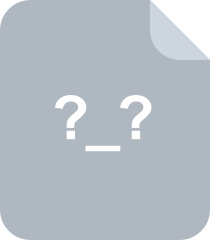
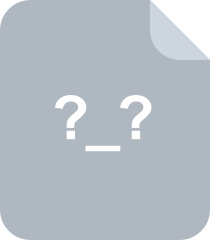
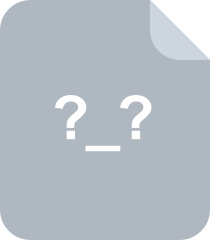
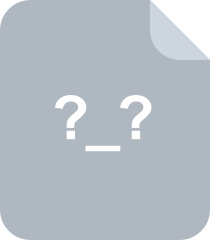
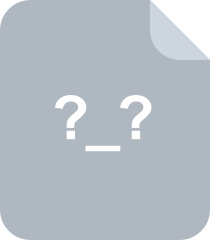
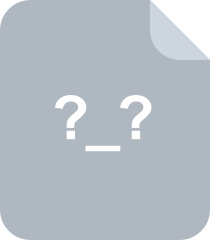
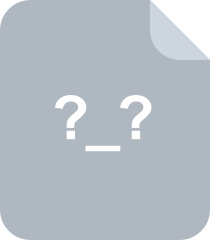
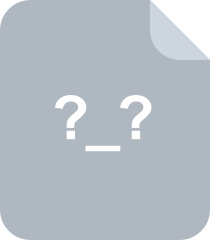
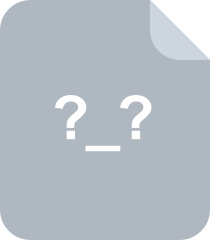
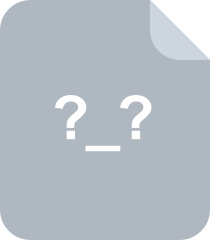
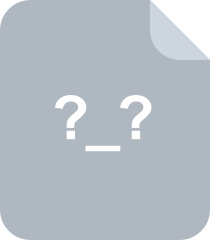
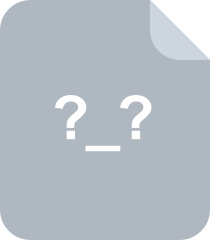
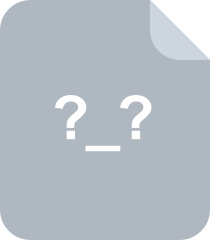
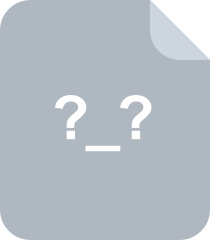
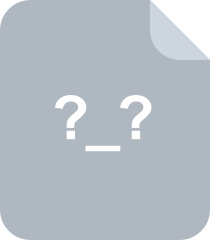
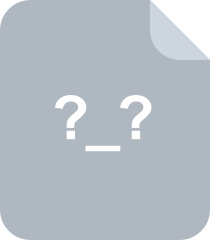
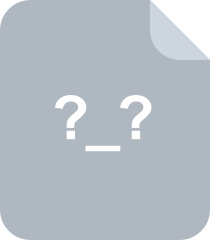
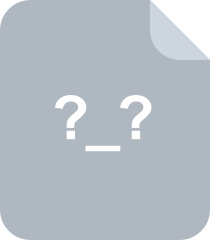
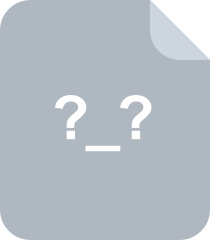
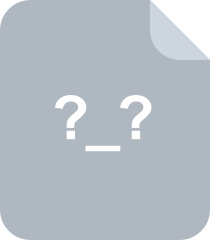
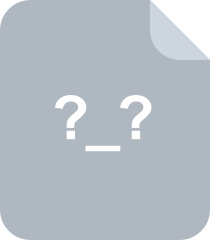
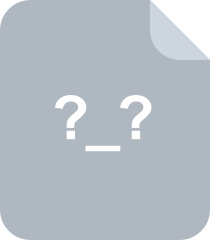
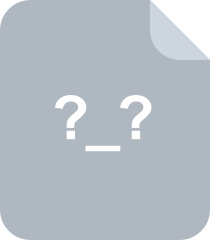
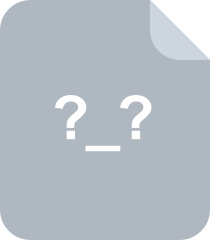
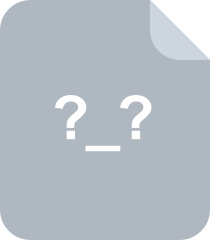
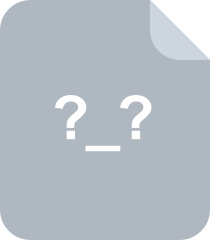
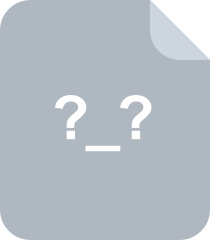
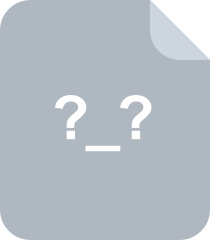
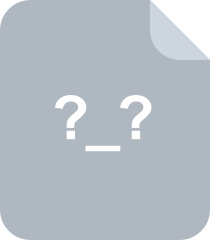
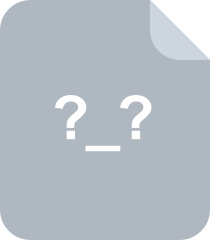
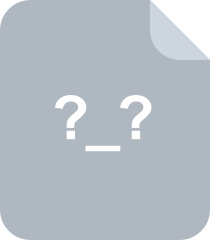
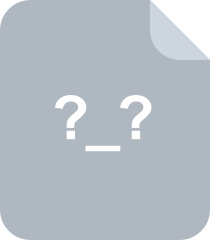
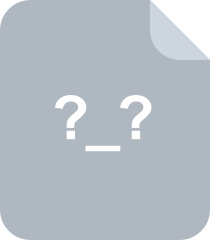
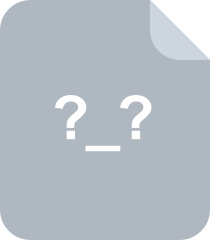
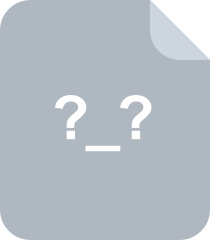
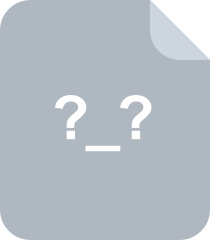
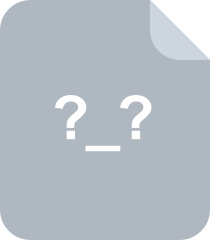
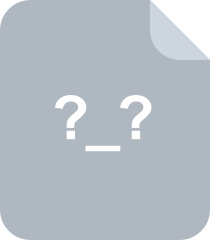
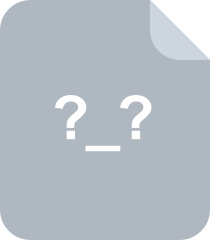
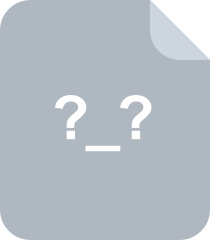
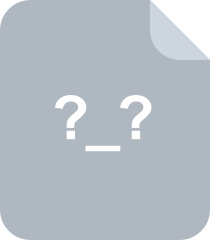
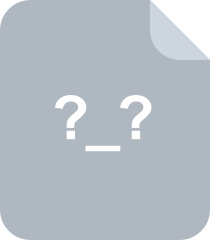
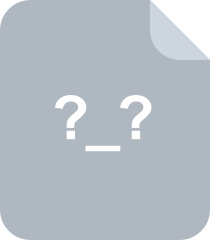
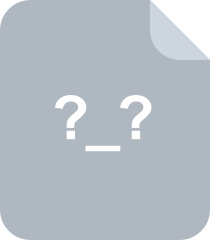
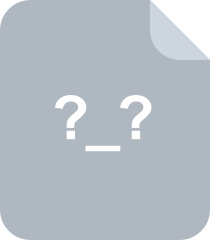
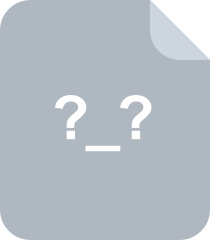
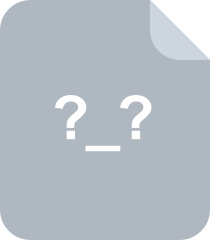
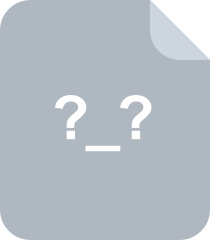
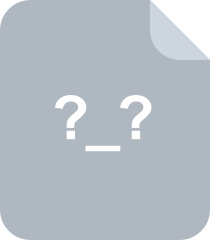
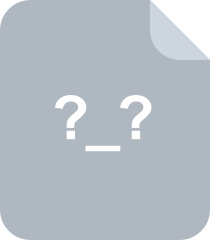
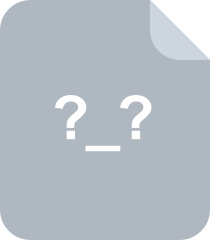
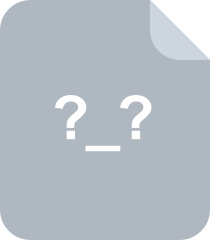
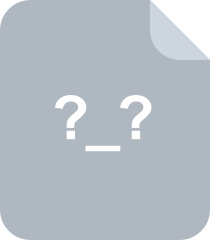
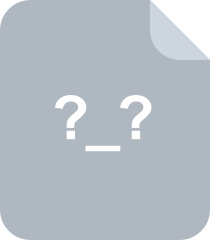
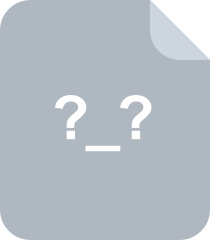
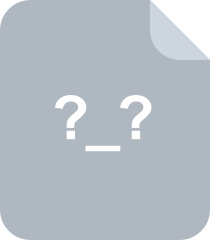
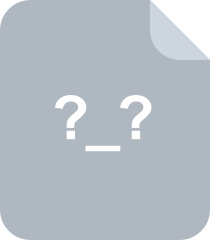
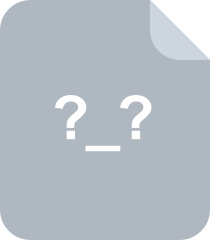
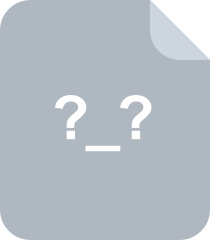
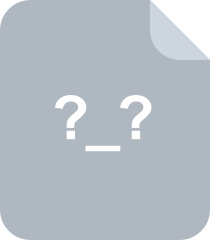
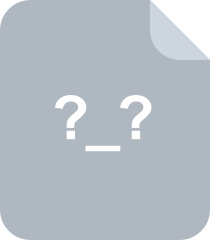
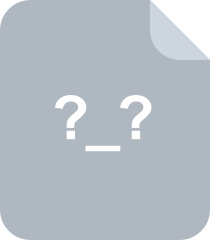
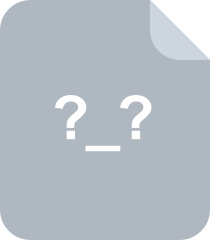
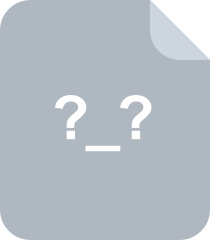
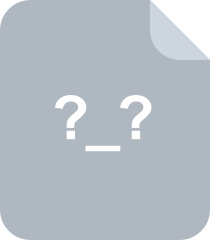
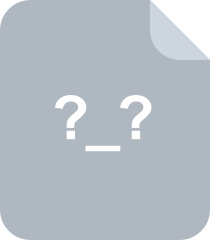
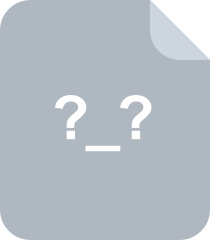
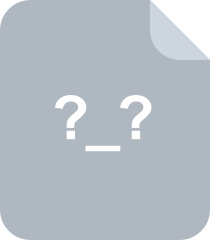
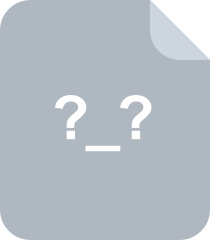
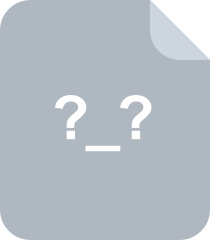
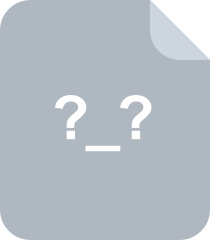
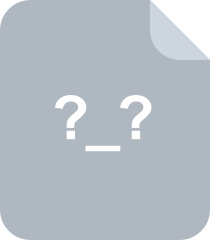
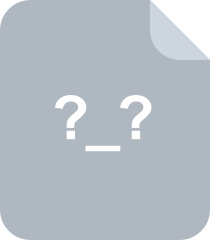
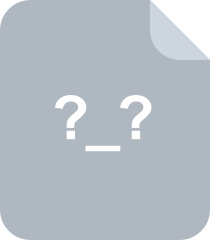
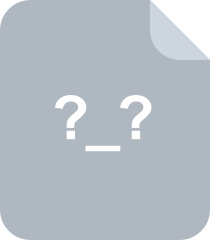
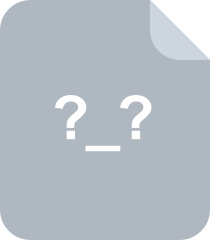
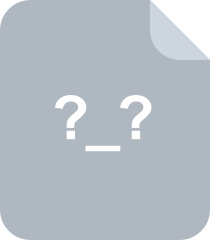
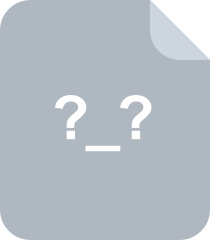
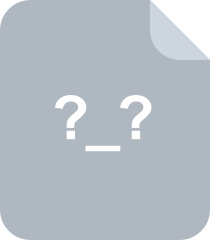
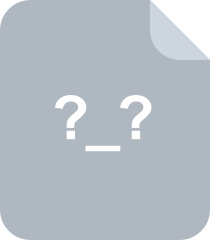
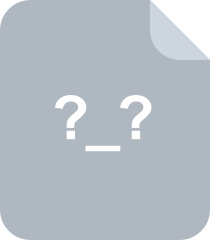
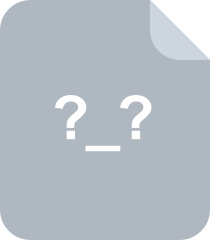
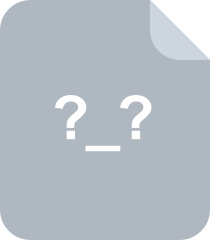
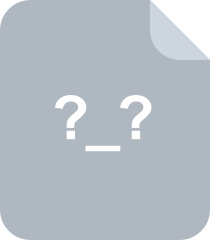
共 445 条
- 1
- 2
- 3
- 4
- 5
资源评论
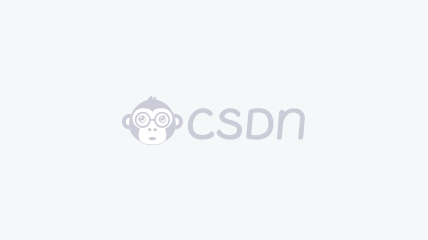

创作小达人
- 粉丝: 2106
- 资源: 598
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

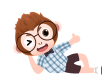
最新资源
- 将电脑屏幕录屏转换成gif
- MATLAB代码:基于非对称纳什谈判的多微网P2P电能交易策略 关键词:纳什谈判 合作博弈 微网 电转气-碳捕集 P2P电能交易交易 参考文档:加好友获取 仿真平台:MATLAB CPLE
- WebSocket长连接实现步骤:基于心跳机制的消息处理与连接管理详解
- MATLAB代码:基于模型预测算法的含储能微网双层能量管理模型 关键词:储能优化 模型预测控制MPC 微网 优化调度 能量管理 参考文档:私 主要内容:代码主要做的是一个微网双层优化调度模型,微网
- “厉行节约从我做起”班会教案课件模板.pptx
- “班主任工作经验交流”中小学老师培训教案课件.pptx
- 电子元件自动整形机(sw16可编辑+工程图)全套技术资料100%好用.zip
- “光盘行动,节约粮食”教案课件.pptx
- 家庭教育“亲子沟通技巧”教案课件.pptx
- 荷花素才“廉政文化”讲座教案课件模板.pptx
- 人工大猩猩部队GTO优化CNN-LSTM用于多变量负荷预测(Matlab) 所有程序经过验证,保证有效运行 2.提出了一种基于CNN-LSTM的多变量电力负荷预测方法,该方法将历史负荷与气象数据作
- 鲜花商城(springboot+vu)
- 1.Matlab实现SSA-CNN-GRU麻雀算法优化卷积门控循环单元时间序列预测; 2.输入数据为单变量时间序列数据,即一维数据; 3.运行环境Matlab2020b及以上,data为数据集,运行主
- 1736388797326.zip
- 在matlab中用蒙特卡洛算法对电动汽车充电负荷进行模拟,可自己修改电动汽车数量,复现 参考lunwen:基于V2G的电动汽车充放电优化调度策略 有注释简单易懂,可随意调整参数
- 研究背景:随着超快激光应用越来越广泛,对超快激光加工过程的材料去除过程就比较关心 研究内容:利用COMSOL仿真软件,构建三维模型,研究电子和晶格温度,引入热焓法对相变过程进行研究 关键词:双温方
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


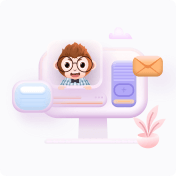
安全验证
文档复制为VIP权益,开通VIP直接复制
