#include "stdafx.h"
#include <QtCore/QTimer>
#include <QtCore/QString>
#include <QtCore/QPropertyAnimation>
#include <QtWidgets/QLabel>
#include <QtWidgets/QMenuBar>
#include <QtWidgets/QHBoxLayout>
#include <QtWidgets/QMdiSubWindow>
#include <QtWidgets/QGraphicsOpacityEffect>
#include <QtWidgets/QGraphicsDropShadowEffect>
#include "OperateTip.h"
extern QMainWindow * g_pMainWindow;
static const QString c_szStyleSheet = "QWidget{background-color:%1;\
border:1px solid %2;border-top:0;border-bottom-left-radius:3px;\
border-bottom-right-radius:3px;background-image: url();}\
";
static const char *c_szGMPOperateTip = "GMPOperateTip";
static const char *c_szSuccess = QT_TRANSLATE_NOOP("GMPOperateTip", "操作成功");
static const char *c_szWaring = QT_TRANSLATE_NOOP("GMPOperateTip", "操作失败");
/*!
*@brief 淡出提示框
*@author renl 2017年8月7日
*@param[in] parent:父窗口;strText:提示框内容;nShowTime:显示时长; nAnimationTime:消失动画时长
*@return void
*/
void OperateTip::fadeOut(QWidget * parent /*= nullptr */
, QString strText /*= "" */
, const unsigned int nShowTime /*= 4000 */
, const unsigned int nAnimationTime /*= 500*/)
{
OperateTip * tipWidget = new OperateTip(parent);
tipWidget->setAnimationMode(AM_FADEOUT);
tipWidget->setText(strText);
tipWidget->setStayTime(nShowTime);
tipWidget->setAnimationdDuration(nAnimationTime);
tipWidget->show();
}
/*!
*@brief 自定义飞出方向提示框
*@author renl 2017年8月7日
*@param[in] parent:父窗口;strText:提示框内容;direction:飞出方向;nShowTime:显示时长; nAnimationTime:消失动画时长
*@return void
*/
void OperateTip::flyOut(QWidget * parent /*= nullptr */
, QString strText /*= "" */
, Direction direction /*= D_TOP*/
, const unsigned int nShowTime /*= 4000 */
, const unsigned int nAnimationTime /*= 500 */)
{
OperateTip * tipWidget = new OperateTip(parent);
tipWidget->setAnimationMode(AM_FLYOUT);
tipWidget->setStyleSheet(c_szStyleSheet.arg("#12B33D").arg("#2ABB51"));
tipWidget->setText(strText);
tipWidget->setFlyoutDirection(direction);
tipWidget->setStayTime(nShowTime);
tipWidget->setAnimationdDuration(nAnimationTime);
tipWidget->show();
}
/*!
*@brief 自定义背景色提示框
*@author renl 2017年8月7日
*@param[in] parent:父窗口;strText:提示框内容;color:背景色;animation:动画模式;direction:飞出方向;nShowTime:显示时长; nAnimationTime:消失动画时长
*@return void
*/
void OperateTip::colorize(QWidget * parent /*= nullptr */
, QString strText /*= "" */
, const QColor & color /* = QColor(20, 142, 53) */
, AnimationMode animation /*= AM_FLYOUT */
, Direction direction /*= D_TOP */
, const unsigned int nShowTime /*= 4000 */
, const unsigned int nAnimationTime /*= 500*/)
{
OperateTip * tipWidget = new OperateTip(parent);
tipWidget->setText(strText);
tipWidget->setBackgroundColor(color);
tipWidget->setAnimationMode(animation);
tipWidget->setFlyoutDirection(direction);
tipWidget->setStayTime(nShowTime);
tipWidget->setAnimationdDuration(nAnimationTime);
tipWidget->show();
}
/*!
*@brief 警告提示框
*@author renl 2017年8月7日
*@param[in] parent:父窗口;strText:提示框内容;nShowTime:显示时长; nAnimationTime:消失动画时长
*@return void
*/
void OperateTip::waring(QWidget * parent /*= nullptr */
, QString strText /*= "" */
, const unsigned int nShowTime /*= 4000 */
, const unsigned int nAnimationTime /*= 500*/)
{
OperateTip * tipWidget = new OperateTip(parent);
tipWidget->setAnimationMode(AM_FLYOUT);
tipWidget->setFlyoutDirection(D_TOP);
tipWidget->setIcon(":/tip/warning.png");
tipWidget->setStyleSheet(c_szStyleSheet.arg("#FF9830").arg("#FF9326"));
if (strText.isEmpty())
{
tipWidget->setText(qApp->translate(c_szGMPOperateTip, c_szWaring));
}
else
{
tipWidget->setText(strText);
}
tipWidget->setStayTime(nShowTime);
tipWidget->setAnimationdDuration(nAnimationTime);
tipWidget->show();
}
/*!
*@brief 成功提示框
*@author renl 2017年8月7日
*@param[in] parent:父窗口;strText:提示框内容;nShowTime:显示时长; nAnimationTime:消失动画时长
*@return void
*/
void OperateTip::success(QWidget * parent /*= nullptr */
, QString strText /*= "" */
, const unsigned int nShowTime /*= 4000 */
, const unsigned int nAnimationTime /*= 500*/)
{
OperateTip * tipWidget = new OperateTip(parent);
tipWidget->setStyleSheet(c_szStyleSheet.arg("#12B33D").arg("#2ABB51"));
tipWidget->setAnimationMode(AM_FLYOUT);
tipWidget->setFlyoutDirection(D_TOP);
if (strText.isEmpty())
{
tipWidget->setText(qApp->translate(c_szGMPOperateTip, c_szSuccess));
}
else
{
tipWidget->setText(strText);
}
tipWidget->setStayTime(nShowTime);
tipWidget->setAnimationdDuration(nAnimationTime);
tipWidget->show();
}
OperateTip::OperateTip(QWidget * parent)
: QWidget(parent)
, m_eMode(AM_FLYOUT)
, m_eDirection(D_TOP)
, m_DurationTime(500)
, m_iStayDuration(4000)
, m_pMessageIcon(nullptr)
, m_pMessage(nullptr)
, m_pMoveWidget(nullptr)
, m_pAnimation(nullptr)
, m_pOpacity(nullptr)
, m_pShadow(nullptr)
{
setAttribute(Qt::WA_DeleteOnClose);
setAttribute(Qt::WA_QuitOnClose, false);
initializeUI();
initializeConnect();
setShadowEnable(true);
m_pMoveWidget->setMinimumWidth(130);
m_StayTimer.setSingleShot(true);
}
OperateTip::~OperateTip()
{
m_pMessageIcon = nullptr;
m_pMessage = nullptr;
m_pMoveWidget = nullptr;
m_pAnimation = nullptr;
m_pOpacity = nullptr;
m_pShadow = nullptr;
}
/*!
*@brief 设置文本内容
*@author renl 2017年8月7日
*@param[in]
*@return void
*/
void OperateTip::setText(const QString & text)
{
if (m_pMessage)
{
m_pMessage->setText(text);
}
}
/*!
*@brief 设置图标
*@author renl 2017年8月7日
*@param[in]
*@return void
*/
void OperateTip::setIcon(const QString & icon)
{
if (m_pMessageIcon)
{
m_pMessageIcon->setPixmap(QPixmap(icon));
}
}
/*!
*@brief 设置背景色
*@author renl 2017年8月7日
*@param[in]
*@return void
*/
void OperateTip::setBackgroundColor(const QColor & color)
{
QColor border = color;
border.setAlpha(255 * 0.1);
QString strBorderRgba = QString("rgba(%1,%2,%3,%4)").arg(border.red()).arg(border.green()).arg(border.blue()).arg(border.alpha());
setStyleSheet(c_szStyleSheet.arg(color.name()).arg(strBorderRgba));
}
/*!
*@brief 消失动画模式
*@author renl 2017年8月7日
*@param[in]
*@return void
*/
void OperateTip::setAnimationMode(AnimationMode mode)
{
if (m_eMode == mode)
{
return;
}
m_eMode = mode;
}
/*!
*@brief 消失动画为飞出时有效 设置飞出方向
*@author renl 2017年8月7日
*@param[in]
*@return void
*/
void OperateTip::setFlyoutDirection(Direction direction)
{
if (m_eDirection == direction)
{
return;
}
m_eDirection = direction;
}
/*!
*@brief 设置是否启用提示框阴影
*@author renl 2017年8月7日
*@param[in]
*@return void
*/
void OperateTip::setShadowEnable(bool enable)
{
if (!m_pShadow)
{
m_pShadow = new QGraphicsDropShadowEffect(this);
m_pShadow->setColor(QColor(0, 0, 0, 70));
m_pShadow->setBlurRadius(10);
m_pShadow->setOffset(4, 4);
}
setGraphicsEffect(enable ? m_pShadow : nullptr);
}
/*!
*@brief 设置提示框消失动画时长
*@author renl 2017年8月7日
*@param[in]
*@return void
*/
void OperateTip::setAnimationdDuration(int duration)
{
m_DurationTime = duration;
}
/*!
*@brief 设置提示框停留时长
*@author renl 2017年8月7日
*@param[in]
*@return void
*/
void OperateTip::setStayTime(int time)
{
m_iStayDuration = time;
}
/*!
*@brief 重写窗口事件函数,当窗口显示时启动定时器
*@author renl 2017年8月7日
*@param[in]
*@return void
*/
bool OperateTip::event(QEvent * e)
{
if (e->type() == QEvent::Show)
{
adjustSize();
int nposx = qrand() % (parentWidg

一窝蜂117
- 粉丝: 604
- 资源: 44
最新资源
- springboot294基于java的火车票订票系统的设计与实现.zip
- springboot296基于个性化定制的智慧校园管理系统设计与开发.zip
- springboot299基于Java的家政服务平台的设计与实现.zip
- springboot297毕业生实习与就业管理系统的设计与实现.zip
- springboot298计算机学院校友网.zip
- stock股票系统使用python进行开发.zip
- statmodels在Python中的统计建模和计量经济学.zip
- Spyder科学Python开发环境的官方存储库.zip
- StreamFramework是一个Python库,它允许你使用Cassandra和Redis构建新闻feed活动流和.zip
- Telegram bot与ChatGPT基于python使用OpenAIs API.zip
- Synapse Matrix用PythonTwisted编写.zip
- Tornado是一个Python web框架和异步网络库,最初由FriendFeed开发.zip
- Theano是一个Python库,它允许您有效地定义、优化和评估涉及多维数组的数学表达式.zip
- TensorRTLLM为用户提供了一个简单易用的Python API来定义大型语言模型llm,并构建包含状态优化的Te.zip
- NI LabVIEW附加工具包激活,第三方组件授权,网络工具包授权,VI激活,全系列支持
- Vim pythonmode PyLint绳Pydoc断点从框.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


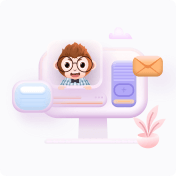
- 1
- 2
前往页