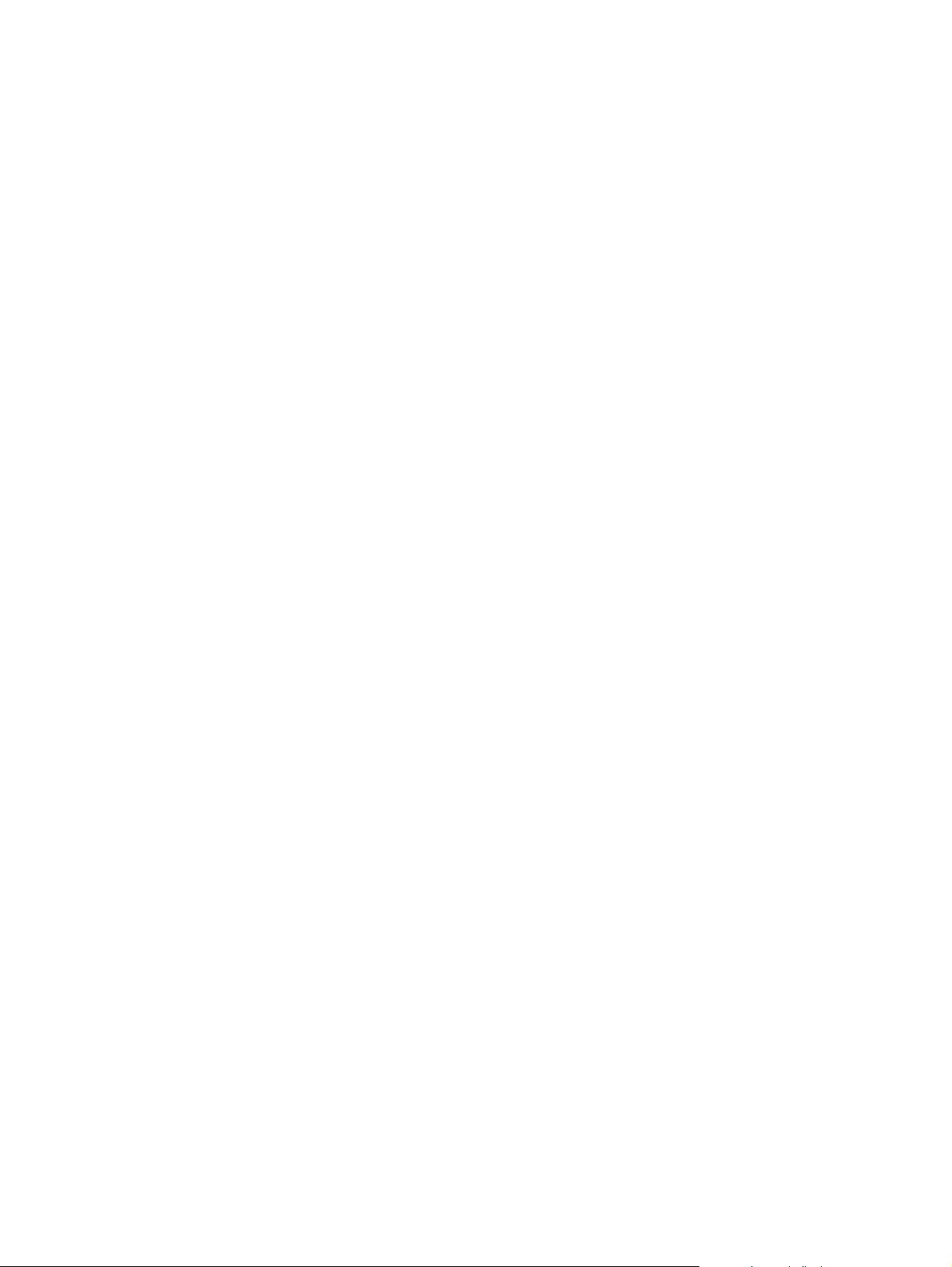
SWIG-2.0 Documentation
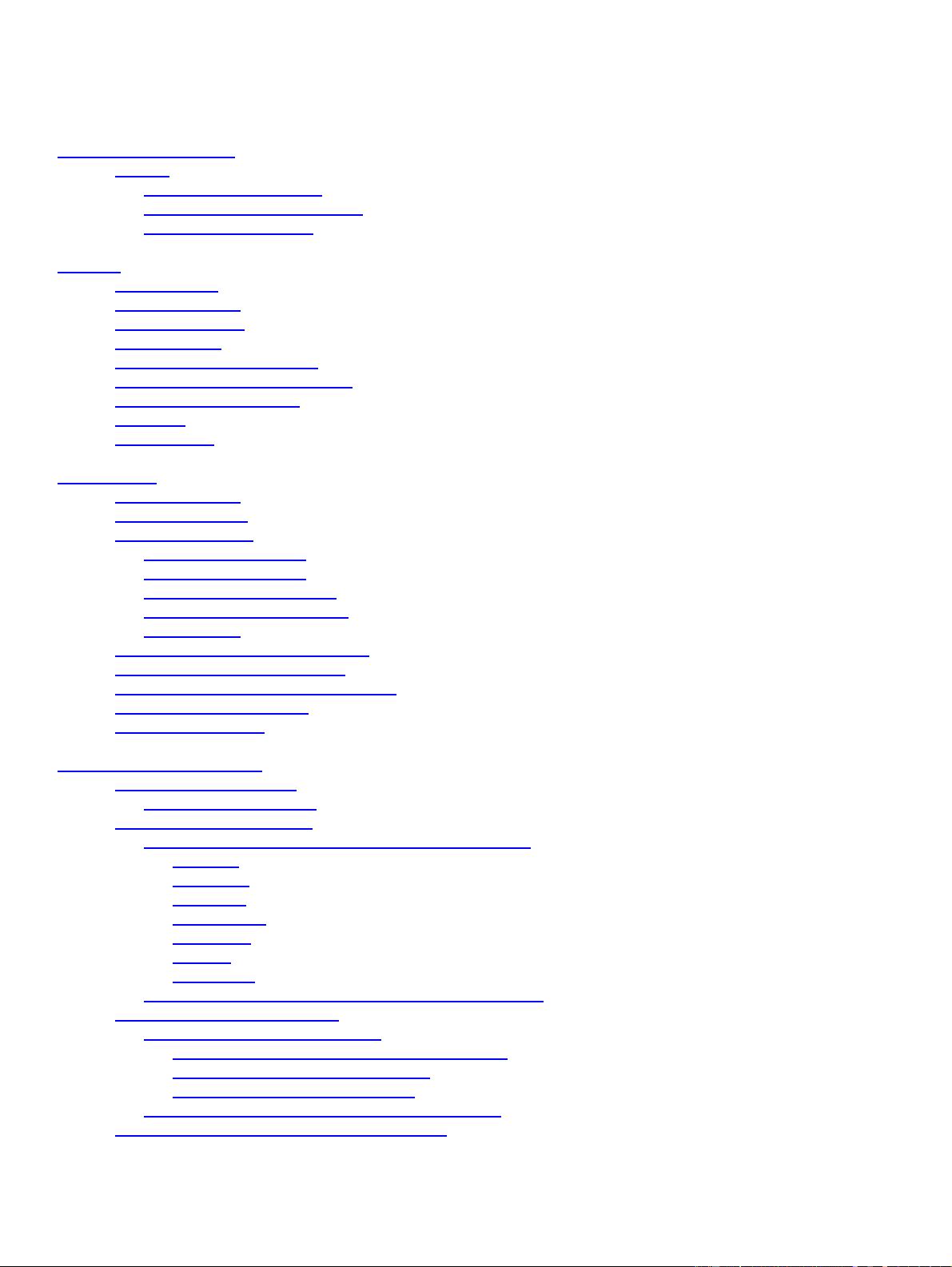
Table of Contents
SWIG-2.0 Documentation..................................................................................................................................................................1
Sections...................................................................................................................................................................................1
SWIG Core Documentation............................................................................................................................................1
Language Module Documentation..................................................................................................................................1
Developer Documentation...............................................................................................................................................1
1 Preface...............................................................................................................................................................................................2
1.1 Introduction.......................................................................................................................................................................2
1.2 SWIG Versions.................................................................................................................................................................2
1.3 SWIG resources................................................................................................................................................................2
1.4 Prerequisites......................................................................................................................................................................3
1.5 Organization of this manual..............................................................................................................................................3
1.6 How to avoid reading the manual.....................................................................................................................................3
1.7 Backwards compatibility..................................................................................................................................................3
1.8 Credits...............................................................................................................................................................................3
1.9 Bug reports........................................................................................................................................................................4
2 Introduction......................................................................................................................................................................................5
2.1 What is SWIG?.................................................................................................................................................................5
2.2 Why use SWIG?...............................................................................................................................................................5
2.3 A SWIG example..............................................................................................................................................................6
2.3.1 SWIG interface file.................................................................................................................................................6
2.3.2 The swig command.................................................................................................................................................7
2.3.3 Building a Perl5 module.........................................................................................................................................7
2.3.4 Building a Python module......................................................................................................................................7
2.3.5 Shortcuts.................................................................................................................................................................8
2.4 Supported C/C++ language features.................................................................................................................................8
2.5 Non-intrusive interface building.......................................................................................................................................9
2.6 Incorporating SWIG into a build system..........................................................................................................................9
2.7 Hands off code generation................................................................................................................................................9
2.8 SWIG and freedom.........................................................................................................................................................10
3 Getting started on Windows..........................................................................................................................................................11
3.1 Installation on Windows.................................................................................................................................................11
3.1.1 Windows Executable............................................................................................................................................11
3.2 SWIG Windows Examples.............................................................................................................................................11
3.2.1 Instructions for using the Examples with Visual Studio......................................................................................11
3.2.1.1 C#...............................................................................................................................................................12
3.2.1.2 Java.............................................................................................................................................................12
3.2.1.3 Perl.............................................................................................................................................................12
3.2.1.4 Python.........................................................................................................................................................12
3.2.1.5 TCL............................................................................................................................................................12
3.2.1.6 R.................................................................................................................................................................12
3.2.1.7 Ruby...........................................................................................................................................................13
3.2.2 Instructions for using the Examples with other compilers...................................................................................13
3.3 SWIG on Cygwin and MinGW......................................................................................................................................13
3.3.1 Building swig.exe on Windows............................................................................................................................13
3.3.1.1 Building swig.exe using MinGW and MSYS............................................................................................13
3.3.1.2 Building swig.exe using Cygwin...............................................................................................................14
3.3.1.3 Building swig.exe alternatives...................................................................................................................14
3.3.2 Running the examples on Windows using Cygwin..............................................................................................14
3.4 Microsoft extensions and other Windows quirks...........................................................................................................14
SWIG-2.0 Documentation
i
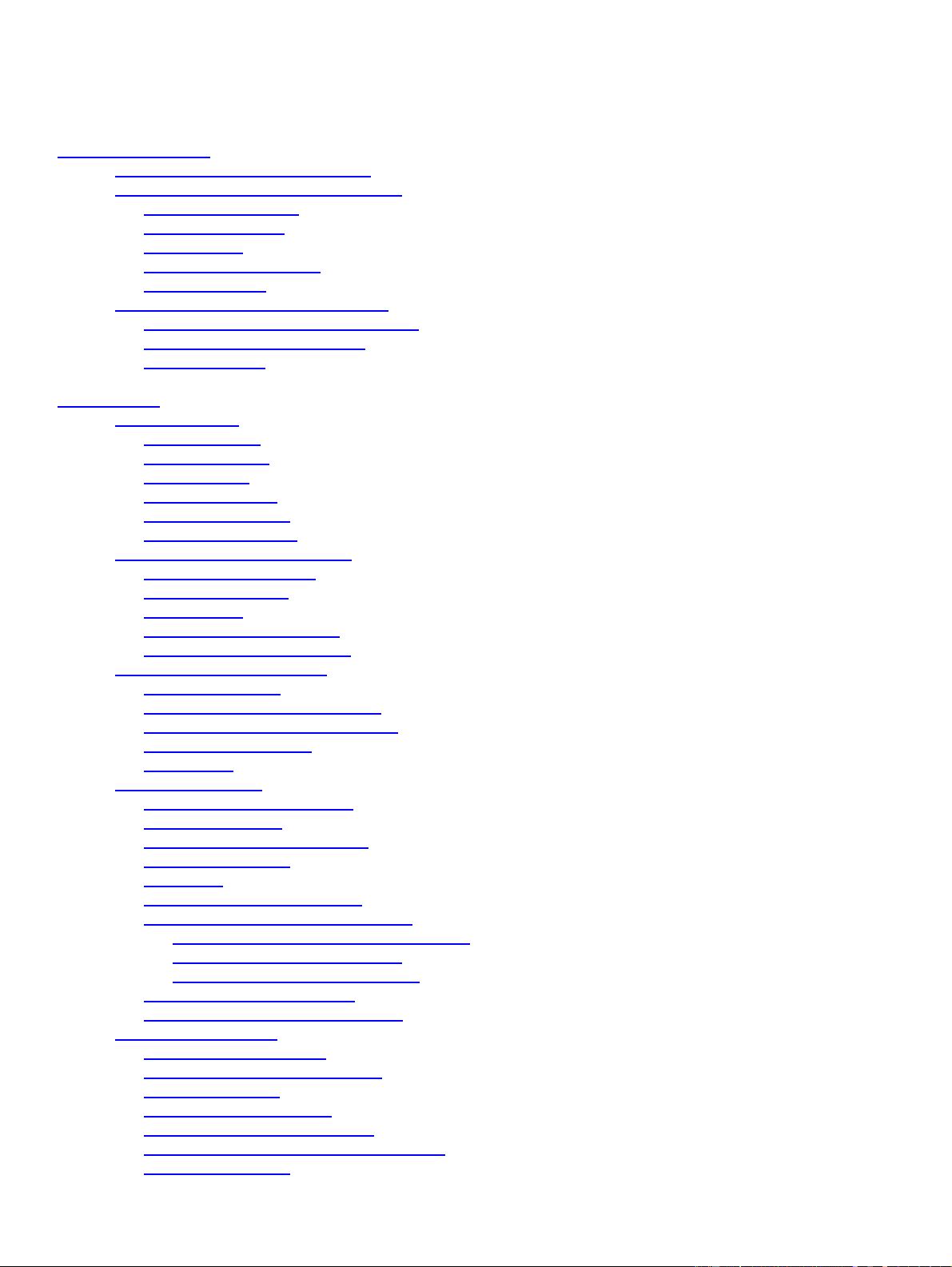
Table of Contents
4 Scripting Languages......................................................................................................................................................................15
4.1 The two language view of the world..............................................................................................................................15
4.2 How does a scripting language talk to C?......................................................................................................................15
4.2.1 Wrapper functions................................................................................................................................................16
4.2.2 Variable linking....................................................................................................................................................16
4.2.3 Constants..............................................................................................................................................................17
4.2.4 Structures and classes...........................................................................................................................................17
4.2.5 Proxy classes........................................................................................................................................................18
4.3 Building scripting language extensions..........................................................................................................................18
4.3.1 Shared libraries and dynamic loading..................................................................................................................18
4.3.2 Linking with shared libraries................................................................................................................................19
4.3.3 Static linking.........................................................................................................................................................19
5 SWIG Basics...................................................................................................................................................................................20
5.1 Running SWIG...............................................................................................................................................................21
5.1.1 Input format..........................................................................................................................................................21
5.1.2 SWIG Output........................................................................................................................................................22
5.1.3 Comments.............................................................................................................................................................22
5.1.4 C Preprocessor......................................................................................................................................................23
5.1.5 SWIG Directives..................................................................................................................................................23
5.1.6 Parser Limitations.................................................................................................................................................23
5.2 Wrapping Simple C Declarations...................................................................................................................................24
5.2.1 Basic Type Handling............................................................................................................................................25
5.2.2 Global Variables...................................................................................................................................................26
5.2.3 Constants..............................................................................................................................................................26
5.2.4 A brief word about const......................................................................................................................................27
5.2.5 A cautionary tale of char *...................................................................................................................................28
5.3 Pointers and complex objects.........................................................................................................................................28
5.3.1 Simple pointers.....................................................................................................................................................29
5.3.2 Run time pointer type checking............................................................................................................................29
5.3.3 Derived types, structs, and classes.......................................................................................................................29
5.3.4 Undefined datatypes.............................................................................................................................................30
5.3.5 Typedef.................................................................................................................................................................31
5.4 Other Practicalities..........................................................................................................................................................31
5.4.1 Passing structures by value...................................................................................................................................31
5.4.2 Return by value.....................................................................................................................................................32
5.4.3 Linking to structure variables...............................................................................................................................32
5.4.4 Linking to char *..................................................................................................................................................33
5.4.5 Arrays...................................................................................................................................................................34
5.4.6 Creating read-only variables.................................................................................................................................35
5.4.7 Renaming and ignoring declarations....................................................................................................................36
5.4.7.1 Simple renaming of specific identifiers.....................................................................................................36
5.4.7.2 Advanced renaming support.......................................................................................................................37
5.4.7.3 Limiting global renaming rules..................................................................................................................39
5.4.8 Default/optional arguments..................................................................................................................................39
5.4.9 Pointers to functions and callbacks......................................................................................................................40
5.5 Structures and unions......................................................................................................................................................41
5.5.1 Typedef and structures.........................................................................................................................................42
5.5.2 Character strings and structures............................................................................................................................43
5.5.3 Array members.....................................................................................................................................................43
5.5.4 Structure data members........................................................................................................................................43
5.5.5 C constructors and destructors..............................................................................................................................44
5.5.6 Adding member functions to C structures............................................................................................................45
5.5.7 Nested structures..................................................................................................................................................48
SWIG-2.0 Documentation
ii
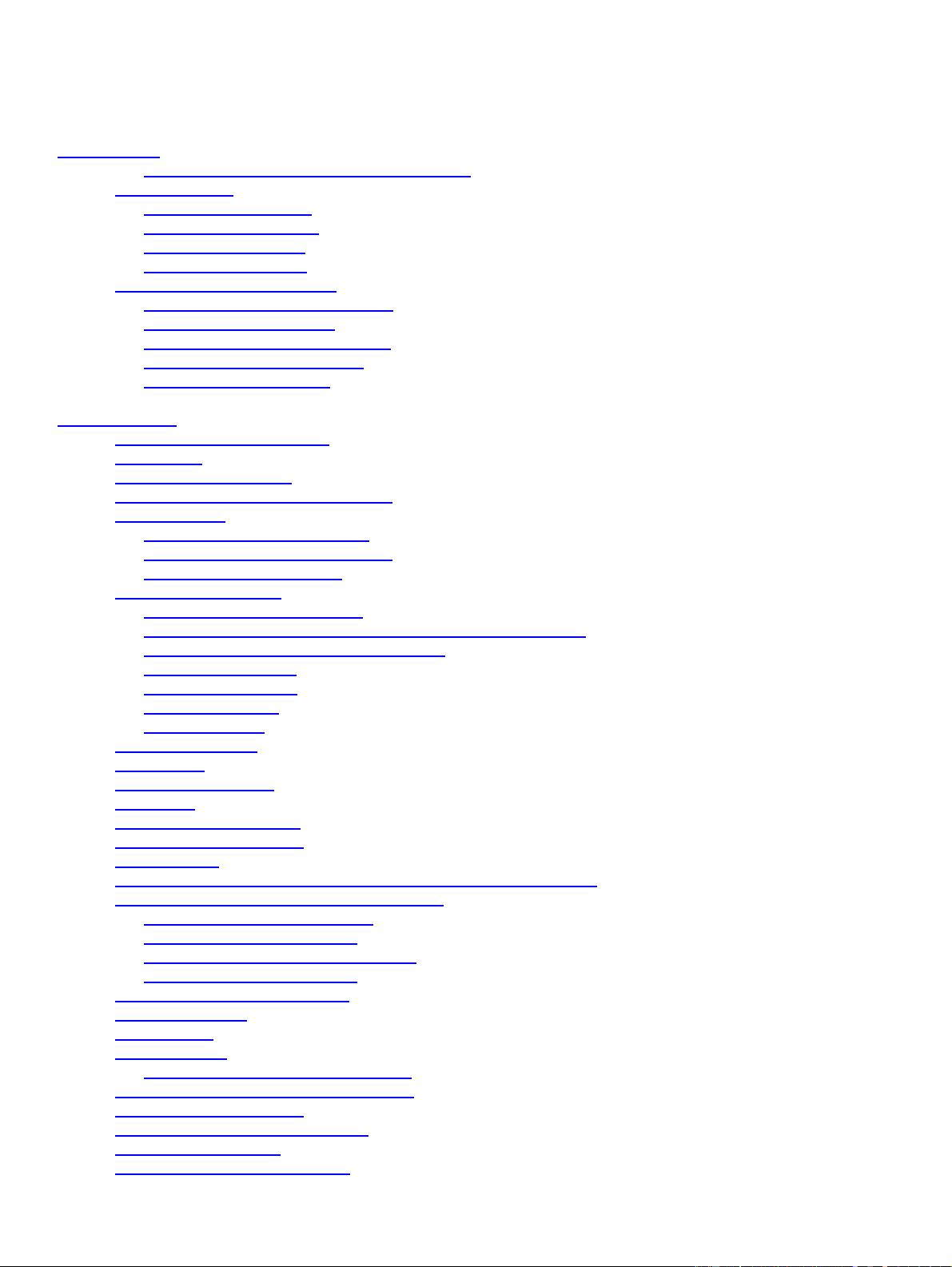
Table of Contents
5 SWIG Basics
5.5.8 Other things to note about structure wrapping.....................................................................................................49
5.6 Code Insertion.................................................................................................................................................................50
5.6.1 The output of SWIG.............................................................................................................................................50
5.6.2 Code insertion blocks...........................................................................................................................................50
5.6.3 Inlined code blocks...............................................................................................................................................51
5.6.4 Initialization blocks..............................................................................................................................................51
5.7 An Interface Building Strategy.......................................................................................................................................51
5.7.1 Preparing a C program for SWIG.........................................................................................................................51
5.7.2 The SWIG interface file.......................................................................................................................................52
5.7.3 Why use separate interface files?.........................................................................................................................53
5.7.4 Getting the right header files................................................................................................................................53
5.7.5 What to do with main().........................................................................................................................................53
6 SWIG and C++...............................................................................................................................................................................54
6.1 Comments on C++ Wrapping.........................................................................................................................................54
6.2 Approach.........................................................................................................................................................................55
6.3 Supported C++ features..................................................................................................................................................55
6.4 Command line options and compilation.........................................................................................................................56
6.5 Proxy classes...................................................................................................................................................................56
6.5.1 Construction of proxy classes...............................................................................................................................56
6.5.2 Resource management in proxies.........................................................................................................................57
6.5.3 Language specific details.....................................................................................................................................59
6.6 Simple C++ wrapping.....................................................................................................................................................59
6.6.1 Constructors and destructors................................................................................................................................59
6.6.2 Default constructors, copy constructors and implicit destructors........................................................................59
6.6.3 When constructor wrappers aren't created............................................................................................................61
6.6.4 Copy constructors.................................................................................................................................................61
6.6.5 Member functions.................................................................................................................................................62
6.6.6 Static members.....................................................................................................................................................63
6.6.7 Member data.........................................................................................................................................................63
6.7 Default arguments...........................................................................................................................................................65
6.8 Protection........................................................................................................................................................................66
6.9 Enums and constants.......................................................................................................................................................66
6.10 Friends..........................................................................................................................................................................66
6.11 References and pointers................................................................................................................................................67
6.12 Pass and return by value...............................................................................................................................................68
6.13 Inheritance....................................................................................................................................................................69
6.14 A brief discussion of multiple inheritance, pointers, and type checking......................................................................70
6.15 Wrapping Overloaded Functions and Methods............................................................................................................72
6.15.1 Dispatch function generation..............................................................................................................................72
6.15.2 Ambiguity in Overloading..................................................................................................................................73
6.15.3 Ambiguity resolution and renaming...................................................................................................................74
6.15.4 Comments on overloading..................................................................................................................................78
6.16 Wrapping overloaded operators....................................................................................................................................78
6.17 Class extension.............................................................................................................................................................80
6.18 Templates......................................................................................................................................................................81
6.19 Namespaces..................................................................................................................................................................89
6.19.1 The nspace feature for namespaces....................................................................................................................93
6.20 Renaming templated types in namespaces...................................................................................................................94
6.21 Exception specifications...............................................................................................................................................95
6.22 Exception handling with %catches...............................................................................................................................96
6.23 Pointers to Members.....................................................................................................................................................96
6.24 Smart pointers and operator->()...................................................................................................................................97
SWIG-2.0 Documentation
iii
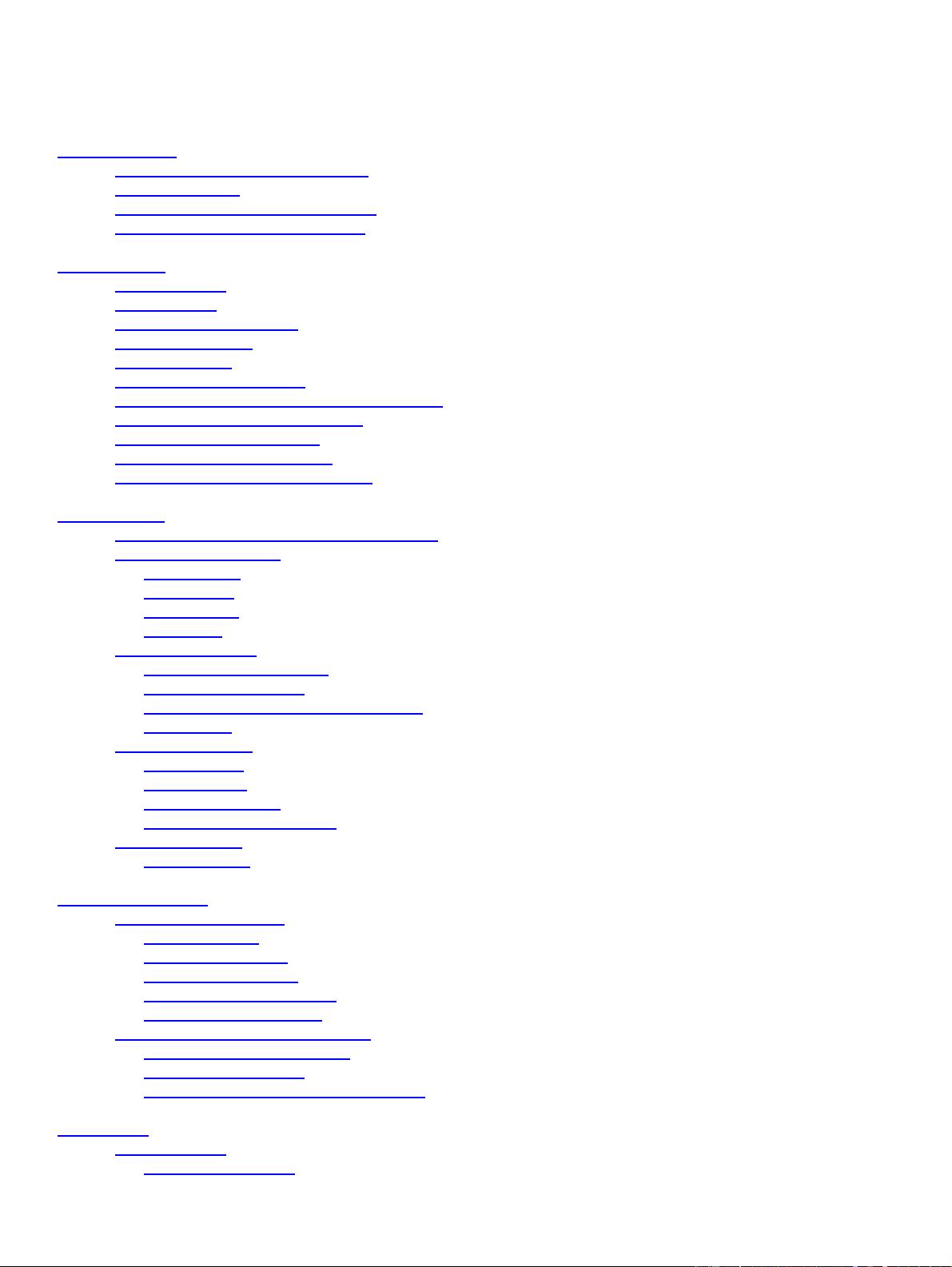
Table of Contents
6 SWIG and C++
6.25 Using declarations and inheritance...............................................................................................................................99
6.26 Nested classes.............................................................................................................................................................100
6.27 A brief rant about const-correctness...........................................................................................................................102
6.28 Where to go for more information..............................................................................................................................103
7 Preprocessing................................................................................................................................................................................104
7.1 File inclusion................................................................................................................................................................104
7.2 File imports...................................................................................................................................................................104
7.3 Conditional Compilation..............................................................................................................................................104
7.4 Macro Expansion..........................................................................................................................................................105
7.5 SWIG Macros...............................................................................................................................................................106
7.6 C99 and GNU Extensions.............................................................................................................................................106
7.7 Preprocessing and %{ ... %} & " ... " delimiters..........................................................................................................107
7.8 Preprocessing and { ... } delimiters..............................................................................................................................107
7.9 Preprocessor and Typemaps.........................................................................................................................................107
7.10 Viewing preprocessor output......................................................................................................................................108
7.11 The #error and #warning directives............................................................................................................................108
8 SWIG library................................................................................................................................................................................109
8.1 The %include directive and library search path...........................................................................................................109
8.2 C Arrays and Pointers...................................................................................................................................................109
8.2.1 cpointer.i.............................................................................................................................................................110
8.2.2 carrays.i..............................................................................................................................................................111
8.2.3 cmalloc.i.............................................................................................................................................................113
8.2.4 cdata.i..................................................................................................................................................................114
8.3 C String Handling.........................................................................................................................................................115
8.3.1 Default string handling.......................................................................................................................................115
8.3.2 Passing binary data.............................................................................................................................................116
8.3.3 Using %newobject to release memory...............................................................................................................116
8.3.4 cstring.i...............................................................................................................................................................116
8.4 STL/C++ Library..........................................................................................................................................................120
8.4.1 std::string............................................................................................................................................................121
8.4.2 std::vector...........................................................................................................................................................121
8.4.3 STL exceptions...................................................................................................................................................123
8.4.4 shared_ptr smart pointer.....................................................................................................................................124
8.5 Utility Libraries............................................................................................................................................................126
8.5.1 exception.i..........................................................................................................................................................126
9 Argument Handling.....................................................................................................................................................................127
9.1 The typemaps.i library..................................................................................................................................................127
9.1.1 Introduction........................................................................................................................................................127
9.1.2 Input parameters.................................................................................................................................................128
9.1.3 Output parameters..............................................................................................................................................129
9.1.4 Input/Output parameters.....................................................................................................................................130
9.1.5 Using different names.........................................................................................................................................130
9.2 Applying constraints to input values............................................................................................................................131
9.2.1 Simple constraint example.................................................................................................................................131
9.2.2 Constraint methods.............................................................................................................................................131
9.2.3 Applying constraints to new datatypes...............................................................................................................131
10 Typemaps....................................................................................................................................................................................133
10.1 Introduction.................................................................................................................................................................134
10.1.1 Type conversion...............................................................................................................................................134
SWIG-2.0 Documentation
iv
- 1
- 2
- 3
前往页