#include "DctDetect.h"
DctDetect::DctDetect(void)
{
}
DctDetect::DctDetect(Mat& frame )
{
m_frmNum = 0;
m_gridCols = 0;
m_gridRows = 0;
m_inc = 1.0;
m_dec = 0.1;
//m_threshold = 0.50;
m_threshold = sqrtf(3.0)/2.0; // cos(45°)= sqrtf(2.0)/2
m_height = frame.rows;
m_width = frame.cols;
m_rect.x = 0;
m_rect.y = 0;
m_rect.width = 4;
m_rect.height = 4;
m_foreground.create( m_height, m_width, CV_8UC1 );
buildGrid(frame, m_rect);
vector<float> coff;
ELEM _elem;
vector<ELEM> _data;
vector<DATA> v_data;
for ( int i=0; i< m_gridRows; ++i )
{
v_data.clear();
for ( int j=0; j< m_gridCols; ++j )
{
_data.clear();
calcDct(frame(m_grid[i][j]), coff );
_elem.m_data = coff;
_elem.m_weight = m_inc;
_data.push_back( _elem );
v_data.push_back(_data);
}
m_model.push_back(v_data);
}
}
void DctDetect::buildGrid(Mat& frame, Rect& box)
{
int width = box.width;
int height = box.height;
BoundingBox bbox;
vector<BoundingBox> inGrid;
for (int y=1;y<frame.rows-height;y+= height )
{
inGrid.clear();
m_gridCols = 0;
for (int x=1;x<frame.cols-width;x+=width)
{
bbox.x = x;
bbox.y = y;
bbox.width = width;
bbox.height = height;
bbox.status = -1;
bbox.prev_status = 0;
bbox.count = 0;
inGrid.push_back(bbox);
m_gridCols++;
}
m_grid.push_back(inGrid);
m_gridRows++;
}
cout<<" rows = "<< m_gridRows<<endl;
cout<<" cols = "<< m_gridCols<<endl;
}
// 计算DCT系数
void DctDetect::calcDct(Mat& frame, vector<float>& coff)
{
if ( frame.empty() )
return;
Mat temp;
if ( 1 == frame.channels())
frame.copyTo( temp);
else
cvtColor( frame, temp, CV_BGR2GRAY);
Mat tempMat( frame.rows, frame.cols, CV_64FC1);
Mat tempDct( frame.rows, frame.cols, CV_64FC1);
temp.convertTo( tempMat, tempMat.type());
dct( tempMat, tempDct, CV_DXT_FORWARD ); // DCT变换
coff.clear();
coff.push_back((float)tempDct.at<double>(0,1) ); // 取值 ( 0,1 )、( 0,2 )、( 1,0 )、( 1,1 )、( 2,0 )
coff.push_back((float)tempDct.at<double>(0,2) );
coff.push_back((float)tempDct.at<double>(1,0) );
coff.push_back((float)tempDct.at<double>(1,1) );
coff.push_back((float)tempDct.at<double>(2,0) );
if ( !temp.empty())
temp.release();
if ( !tempMat.empty())
tempMat.release();
if ( !tempDct.empty())
tempDct.release();
}
// 计算距离
float DctDetect::calcDist(vector<float>& coff1, vector<float>& coff2)
{
float d1 = norm( coff1 );
float d2 = norm( coff2 );
float d3 = dotProduct( coff1,coff2 );
if ( d2 <0.0001 || d1==0 )
return 1.0;
else
return d3/(d1*d2);
}
// 点积
float DctDetect::dotProduct( vector<float>& coff1, vector<float>& coff2)
{
size_t i = 0, n = coff1.size();
assert(coff1.size() == coff2.size());
float s = 0.0f;
const float *ptr1 = &coff1[0], *ptr2 = &coff2[0];
for( ; i < n; i++ )
s += (float)ptr1[i]*ptr2[i];
return s;
}
// 检测邻域是否有前景,有则返回true
bool DctDetect::checkNeighbor(int r,int c)
{
int count = 0;
if ( (r-1) >=0 && m_grid[r-1][c].prev_status == 1) // 上面patch
count++;
if ( (c+1) < m_gridCols && m_grid[r][c+1].prev_status == 1) // 右边patch
count++;
if ( (r+1) < m_gridRows && m_grid[r+1][c].prev_status == 1) // 下面patch
count++;
if ( (c-1) >= 0 && m_grid[r][c-1].prev_status == 1) // 左边patch
count++;
// cout<<" neighbor num = "<<count<<endl;
if ( count > 0 )
return true;
else
return false;
}
void DctDetect::detect(Mat& frame)
{
m_frmNum++;
m_foreground = 0;
vector<float> coff;
ELEM _elem; // 单个数据
vector<ELEM> _data; // 模型数据
for ( int i=0; i< m_gridRows; ++i )
{
for ( int j=0; j< m_gridCols; ++j )
{
calcDct(frame(m_grid[i][j]), coff );
_data = m_model[i][j];
int mNum = _data.size(); // 模型的个数
float dist = 0.0;
float fmax = FLT_MIN;
int idx = -1;
for ( int k=0; k<mNum; ++k )
{
dist = calcDist( coff, _data[k].m_data );
if ( dist > fmax )
{
fmax = dist;
idx = k;
}
} // 匹配完成
if ( fmax > m_threshold ) // 匹配上
{
for ( int k=0; k<mNum; ++k )
{
if ( idx ==k ) // 匹配上的模型权重增加
m_model[i][j][k].m_weight +=m_inc ;
else
m_model[i][j][k].m_weight -=m_dec;
}
}
else // 如果没有匹配上,则标注为最可能前景
{
if ( m_frmNum == 1)
{
m_grid[i][j].prev_status = 1;
_data = m_model[i][j]; // 加入背景模型
_elem.m_data = coff;
_elem.m_weight = m_inc;
_data.push_back( _elem );
m_model[i][j]= _data;
}
m_grid[i][j].status = 1;
}
} // end for j
} // end for i
cout<<" stage one is finished ( first matched)..."<<endl;
// 这里来进行前景的检测
if ( m_frmNum > 1 )
{
for ( int i=0; i< m_gridRows; ++i )
{
for ( int j=0; j< m_gridCols; ++j )
{
bool isNeighbor = checkNeighbor(i,j);
if ( isNeighbor ) // 如果邻域内有前景,则标注为前景区域
{
m_foreground(m_grid[i][j]) =255;
m_grid[i][j].count +=1;
}
else
{
_data = m_model[i][j]; // 加入背景模型
_elem.m_data = coff;
_elem.m_weight = m_inc;
_data.push_back( _elem );
m_model[i][j]= _data;
}
}
}
}
cout<<" stage two is finished ( second matched)..."<<endl;
// 剔除背景中值为负数的模型
/*for ( int i=0; i< m_gridRows; ++i )
{
for ( int j=0; j< m_gridCols; ++j )
{
vector<ELEM> _temp;
_data = m_model[i][j];
int mNum = _data.size();
for ( int k=0; k<mNum; ++k )
{
if ( _data[k].m_weight<0)
continue;
else
{
if ( _data[k].m_weight>20.0 )
_data[k].m_weight = 20.0;
_temp.push_back( _data[k] );
}
}
_data.clear();
_data.insert( _data.begin(), _temp.begin(), _temp.end());
m_model[i][j]= _data;
}
}*/
cout<<" stage three is finished ( thdir matched)..."<<endl;
chageGridStatus();
cout<<" stage four is finished ( status changes)..."<<endl;
}
void DctDetect::chageGridStatus()
{
for ( int i=0; i<m_gridRows; ++i )
{
for ( int j=0; j<m_gridCols; ++j )
{
m_grid[i][j].prev_status = m_grid[i][j].status ;
m_grid[i][j].status = 0;
}
}
}
DctDetect::~DctDetect(void)
{
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
代码实现的是Real-Time Moving Object Detection for Video Surveillance论文里的算法,这篇文章主要讲采用DCT系数进行背景建模,实现运动目标检测,算法对光照变化和噪声有较强的鲁棒性和适应性。代码中DctDetect类中的detect()函数还有些问题,愿有意之士共同研究探讨。
资源推荐
资源详情
资源评论
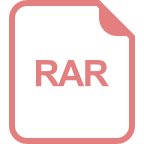
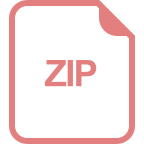
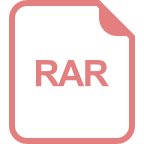
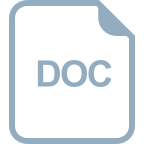
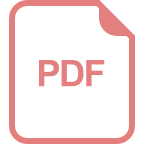
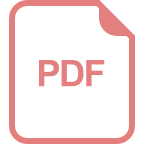
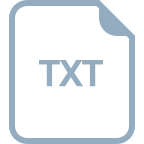
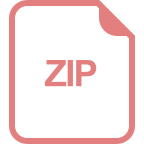
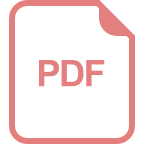
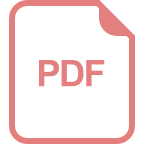
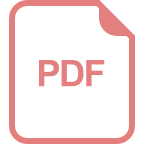
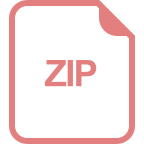
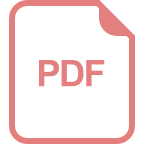
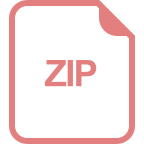
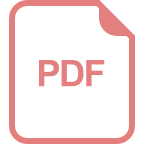
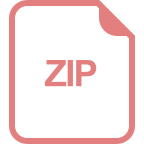
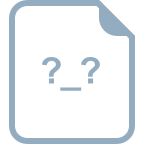
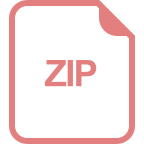
收起资源包目录



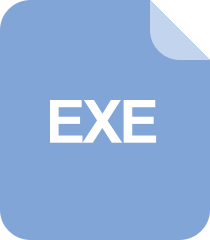
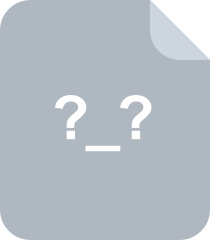
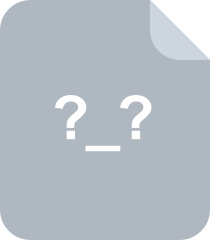
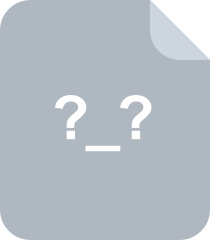
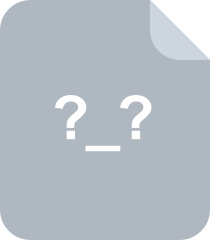


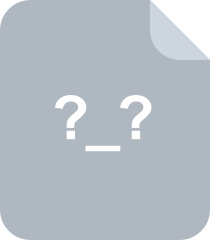
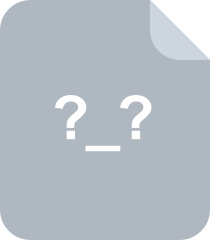
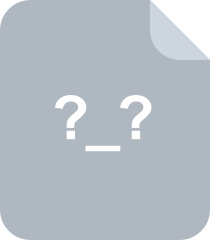
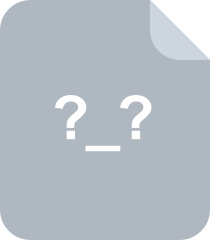
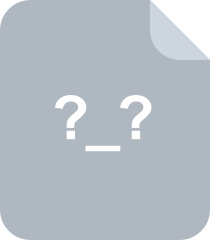
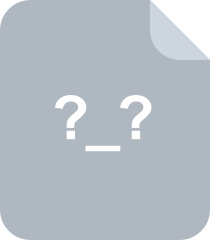
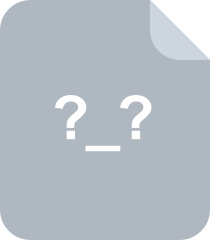
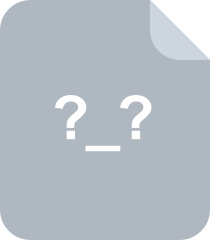
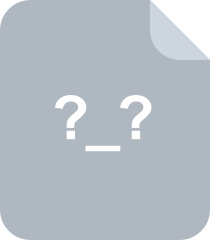
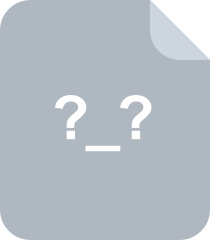
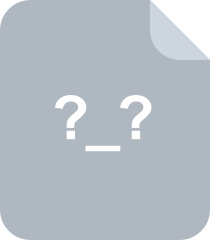
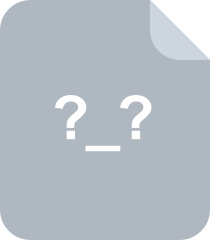
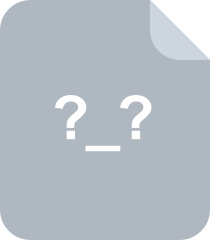

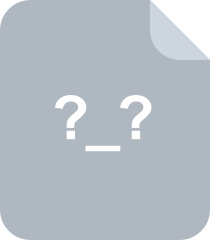
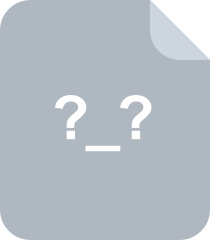
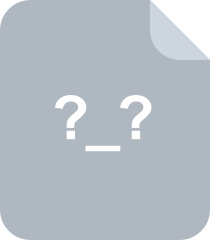
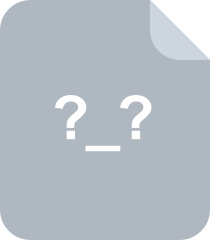
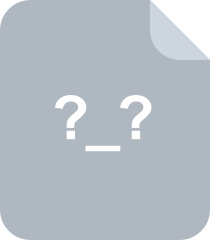
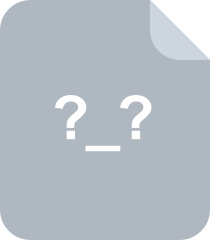
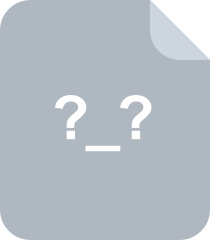

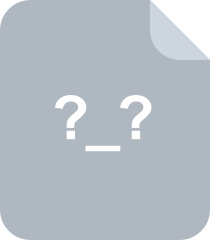
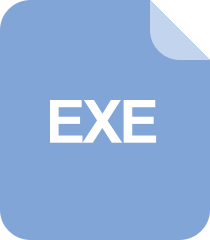
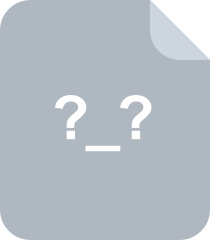
共 28 条
- 1
资源评论
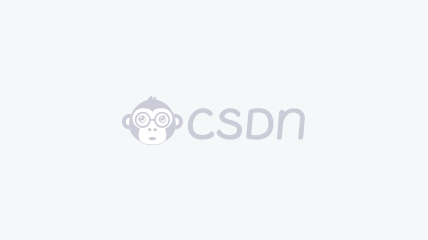
- wanzhaoqu56032017-12-26一般般没什么特别好效果
- 银霜覆秋枫2014-07-09效果比较差~~不太实用~
- 普通网友2013-07-26VC++ ,算法部分使用OpenCV,感谢楼主分享!
- 迷若烟雨2014-06-21该方法对噪声比较敏感,效果有待加强。
- JohnDannl2015-03-04效果很一般,思路可以借鉴一下

Belial_2010
- 粉丝: 1335
- 资源: 46
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

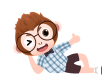
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


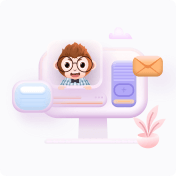
安全验证
文档复制为VIP权益,开通VIP直接复制
