QT使用tableWidget显示双排列表
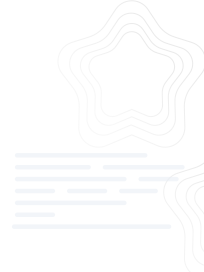


在QT编程中,`QTableWidget` 是一个非常常用的组件,用于展示表格数据。它可以用于创建用户界面,显示和编辑二维数据集。本教程将详细讲解如何使用`QTableWidget`来实现双排列表,并在选中时用红框突出显示。 我们需要了解`QTableWidget`的基本用法。`QTableWidget`继承自`QWidget`,提供了一个二维的格子布局,每个单元格可以包含文本、图像或其他复杂控件。我们可以通过设置列数和行数来初始化`QTableWidget`,并使用`setItem()`方法填充数据。 在创建双排列表时,我们可以设置`QTableWidget`的行数为2,然后在每一行中添加相应的列。例如,我们可以这样创建一个2x2的表格: ```cpp QTableWidget *table = new QTableWidget(); table->setRowCount(2); table->setColumnCount(2); ``` 接着,我们需要填充数据。每行的每个单元格都可以通过`setItem(row, column, item)`方法设置,其中`item`可以是` QTableWidgetItem`的实例。例如: ```cpp for (int i = 0; i < 2; ++i) { for (int j = 0; j < 2; ++j) { QTableWidgetItem *item = new QTableWidgetItem(QString("Row %1, Column %2").arg(i).arg(j)); table->setItem(i, j, item); } } ``` 为了实现选中时用红框圈出来,我们需要重写`QTableWidget`的样式表。`QTableWidget`的选中状态是通过其内部的`QTableWidgetItem`的背景色来体现的。我们可以通过以下方式设置选中项的样式: ```cpp table->setStyleSheet("QTableView::item:selected { background-color: red; border: 2px solid red; }"); ``` 这段代码设置了选中的表格项背景色为红色,并用红色边框包围。这将在用户选择某项时自动应用。 在实际项目中,你可能还需要监听选中事件,例如使用`currentChanged()`信号。当表格项的选中状态改变时,这个信号会被发射。你可以连接这个信号到一个槽函数,以便在用户选择新的项时执行某些操作: ```cpp connect(table, &QTableWidget::currentCellChanged, this, &YourClass::onCurrentCellChanged); ``` 在`onCurrentCellChanged`槽函数中,你可以获取当前选中的行和列,并进行进一步的处理。 别忘了将`QTableWidget`添加到你的布局中,以便它能在界面上正确显示: ```cpp QVBoxLayout *layout = new QVBoxLayout(); layout->addWidget(table); setLayout(layout); ``` 以上就是使用QT的`QTableWidget`创建双排列表,并在选中时用红框突出显示的完整步骤。通过理解`QTableWidget`的基本用法和定制样式,你可以轻松地创建出满足需求的表格界面。在实际开发中,你可能还需要处理更多细节,如数据的动态加载、编辑功能以及更多的自定义样式,但这些都基于上述基础。
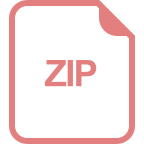
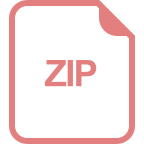
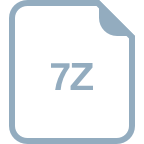
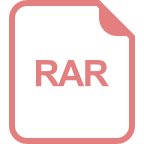
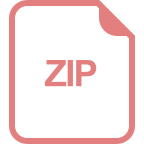
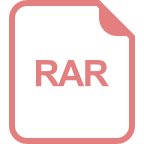
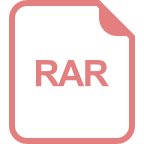
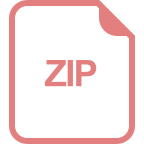
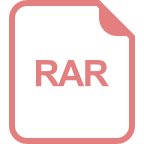
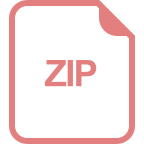
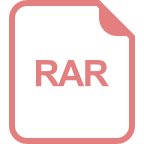
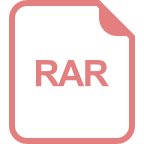
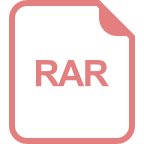
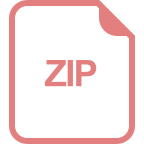
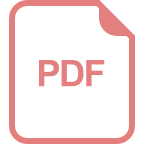
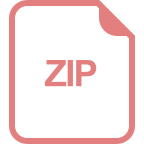
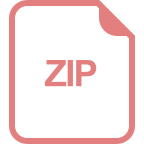
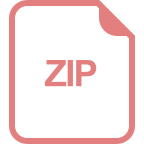
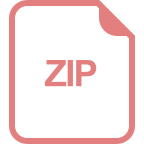




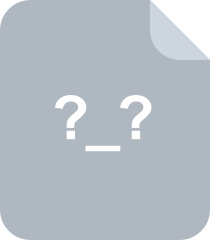

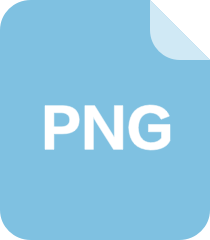
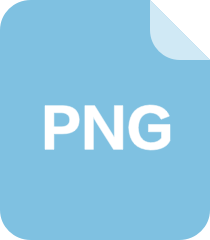
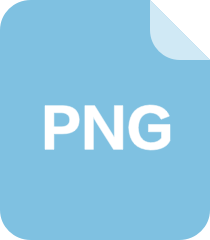
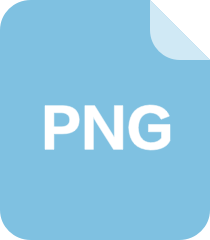
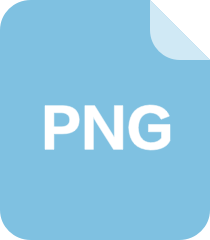
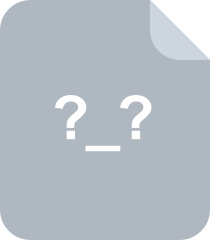
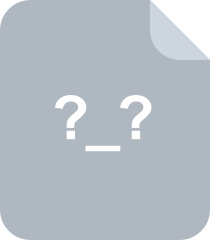

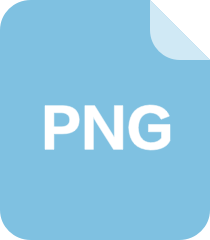
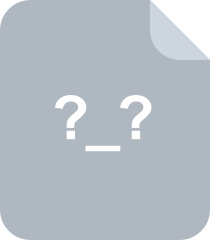
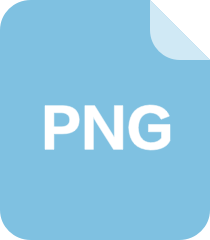


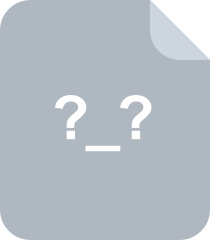
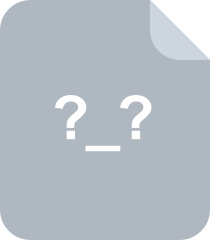

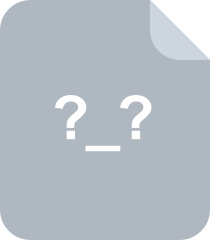
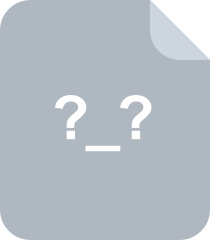
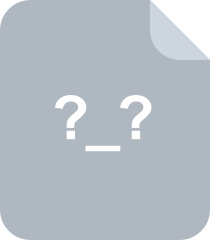

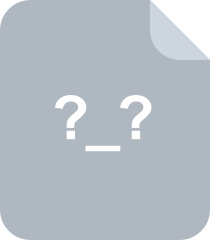
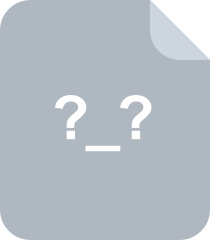
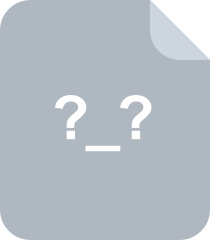
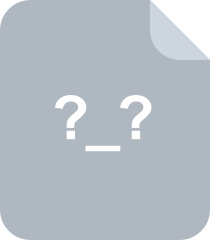
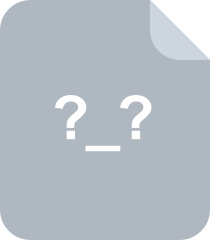
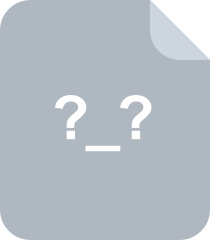
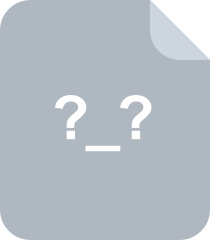
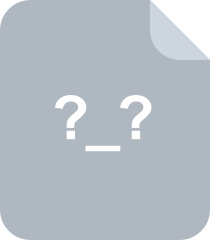
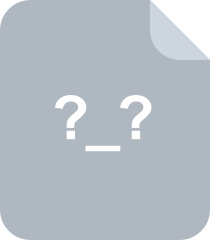
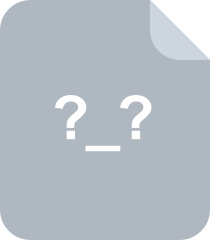
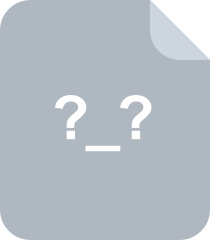
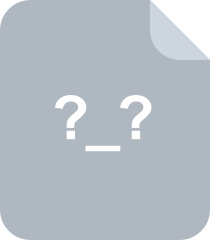
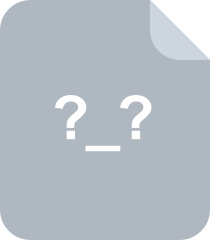
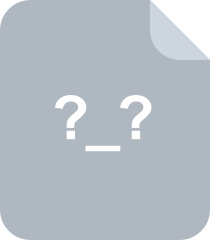
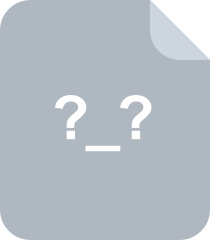
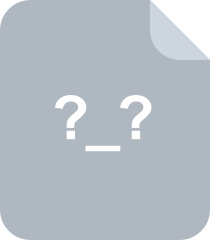
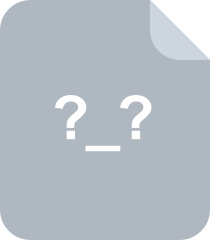
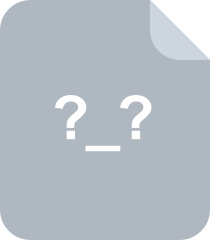
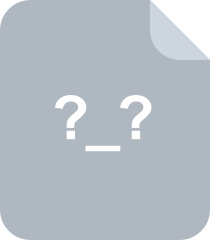
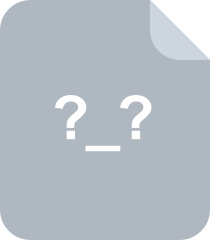
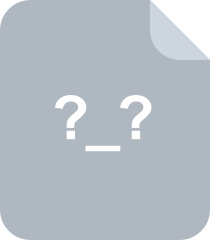
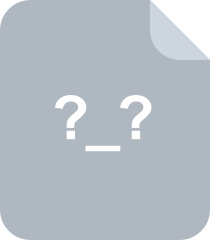
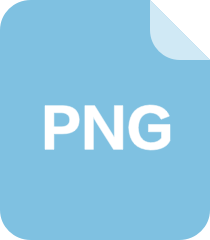
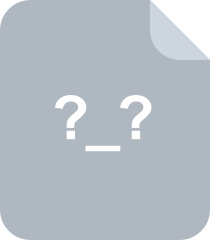
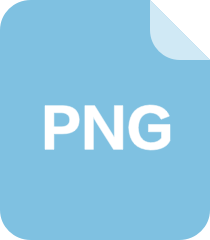
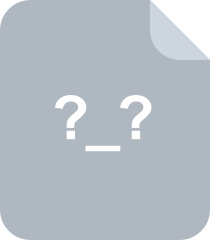
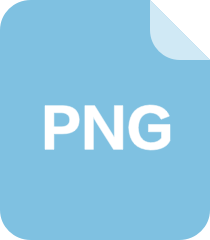
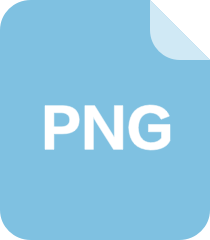
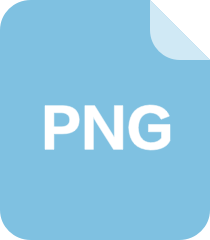
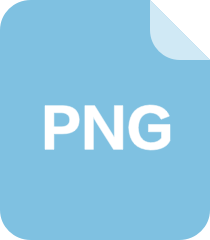


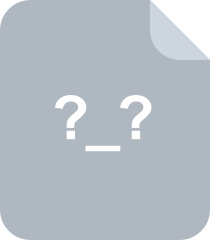
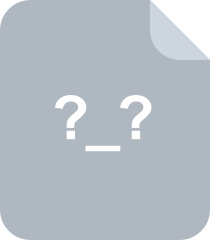
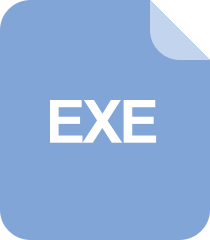
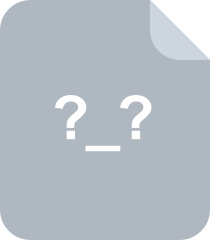
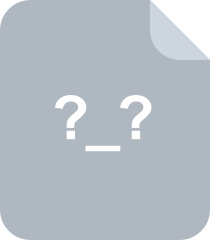
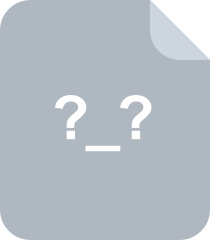
- 1
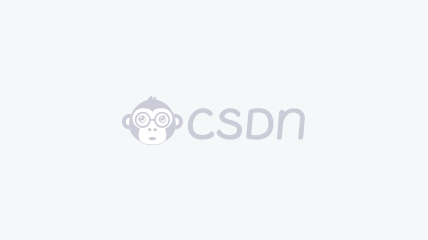
- jing3727694222014-08-14我用的Qt5,导入不进去,也运行不起来呀。

- 粉丝: 1w+
- 资源: 64
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

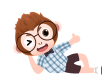
最新资源
- 基于SpringBoot+Vue的校园招聘管理系统(前端代码)
- C++期末大作业-2024-QT仓库商品管理系统,经过老师审定过的,应该能够满足学习、使用需求,如果有需要的话可以放心下载使用
- DH-GSTN5600 剩余电流式电气火灾监控探测器 安装使用说明书
- 天津理工大学信息系统设计实验
- jsp ssm 学校录取查询系统 高校志愿填报录取 项目源码 web java【项目源码+数据库脚本+项目说明+软件工具】毕设
- jsp ssm 网上购物系统 在线购物 在线商城平台 项目源码 web java【项目源码+数据库脚本+项目说明+软件工具】毕设
- 29网课交单平台源码最新修复全开源版本
- jsp ssm 超市网上购物系统 超市管理 超市购物 项目源码 web java【项目源码+数据库脚本+项目说明+软件工具】毕
- 海湾火灾自动报警系统主要设备参数
- C++自制多功能游戏头文件

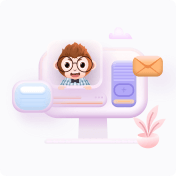
