#include "SimpleDPad.h"
using namespace cocos2d;
SimpleDPad::SimpleDPad(void)
{
_delegate = NULL;
}
SimpleDPad::~SimpleDPad(void)
{
}
SimpleDPad* SimpleDPad::dPadWithFile(CCString *fileName, float radius)
{
SimpleDPad *pRet = new SimpleDPad();
if (pRet && pRet->initWithFile(fileName, radius))
{
return pRet;
}
else
{
delete pRet;
pRet = NULL;
return NULL;
}
}
bool SimpleDPad::initWithFile(CCString *filename, float radius)
{
bool bRet = false;
do
{
CC_BREAK_IF(!CCSprite::initWithFile(filename->getCString()));
_radius = radius;
_direction = CCPointZero;
_isHeld = false;
this->scheduleUpdate();
bRet = true;
} while (0);
return bRet;
}
void SimpleDPad::onEnterTransitionDidFinish()
{
CCDirector::sharedDirector()->getTouchDispatcher()->addTargetedDelegate(this, 1, true);
}
void SimpleDPad::onExit()
{
CCDirector::sharedDirector()->getTouchDispatcher()->removeDelegate(this);
}
void SimpleDPad::update(float dt)
{
if (_isHeld)
{
_delegate->isHoldingDirection(this, _direction);
}
}
bool SimpleDPad::ccTouchBegan(CCTouch *pTouch, CCEvent *pEvent)
{
CCPoint location = pTouch->getLocation();
float distanceSQ = ccpDistanceSQ(location, this->getPosition());
if (distanceSQ <= _radius * _radius)
{
this->updateDirectionForTouchLocation(location);
_isHeld = true;
return true;
}
return false;
}
void SimpleDPad::ccTouchMoved(CCTouch *pTouch, CCEvent *pEvent)
{
CCPoint location = pTouch->getLocation();
this->updateDirectionForTouchLocation(location);
}
void SimpleDPad::ccTouchEnded(CCTouch *pTouch, CCEvent *pEvent)
{
_direction = CCPointZero;
_isHeld = false;
_delegate->simpleDPadTouchEnded(this);
}
void SimpleDPad::updateDirectionForTouchLocation(CCPoint location)
{
float radians = ccpToAngle(ccpSub(location, this->getPosition()));
float degrees = -1 * CC_RADIANS_TO_DEGREES(radians);
if (degrees <= 22.5 && degrees >= -22.5)
{
//right
_direction = ccp(1.0, 0.0);
}
else if (degrees > 22.5 && degrees < 67.5)
{
//bottomright
_direction = ccp(1.0, -1.0);
}
else if (degrees >= 67.5 && degrees <= 112.5)
{
//bottom
_direction = ccp(0.0, -1.0);
}
else if (degrees > 112.5 && degrees < 157.5)
{
//bottomleft
_direction = ccp(-1.0, -1.0);
}
else if (degrees >= 157.5 || degrees <= -157.5)
{
//left
_direction = ccp(-1.0, 0.0);
}
else if (degrees < -22.5 && degrees > -67.5)
{
//topright
_direction = ccp(1.0, 1.0);
}
else if (degrees <= -67.5 && degrees >= -112.5)
{
//top
_direction = ccp(0.0, 1.0);
}
else if (degrees < -112.5 && degrees > -157.5)
{
//topleft
_direction = ccp(-1.0, 1.0);
}
_delegate->didChangeDirectionTo(this, _direction);
}
没有合适的资源?快使用搜索试试~ 我知道了~
PompaDroid cocos2d-x
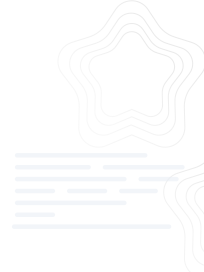
共50个文件
h:10个
dll:9个
cpp:8个


温馨提示
本文实践自 Allen Tan 的文章《How To Make A Side-Scrolling Beat ‘Em Up Game Like Scott Pilgrim with Cocos2D – Part 1》,文中使用Cocos2D,我在这里使用Cocos2D-x 2.0.4进行学习和移植。在这篇文章,将会学习到如何制作一个简单的横版格斗过关游戏。http://blog.csdn.net/akof1314/article/details/8549150
资源推荐
资源详情
资源评论
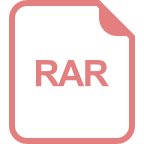
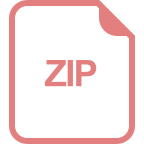
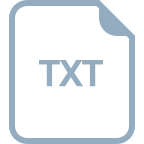
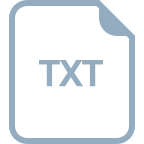
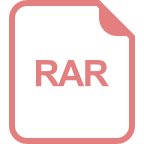
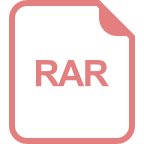
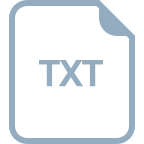
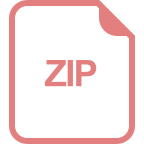
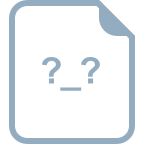
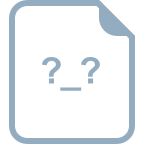
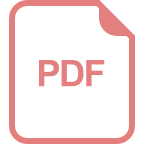
收起资源包目录



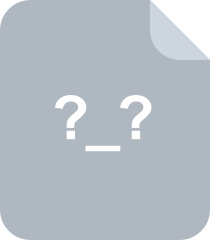

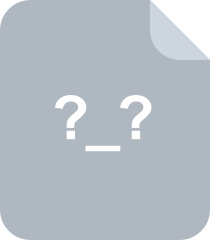
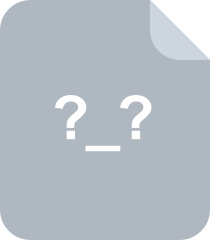

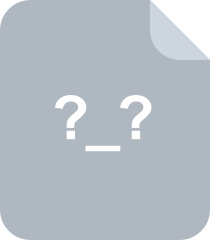

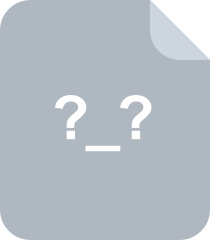
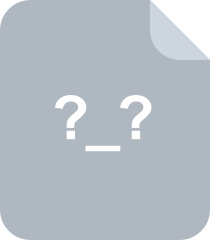
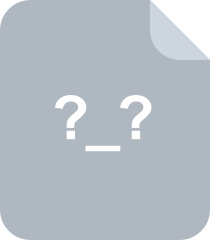
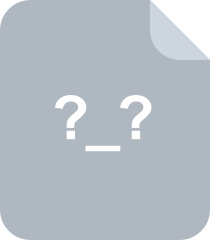

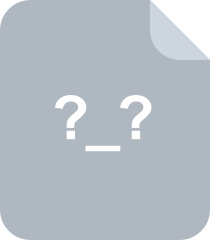
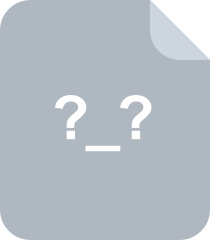
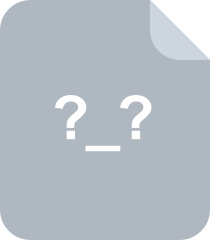
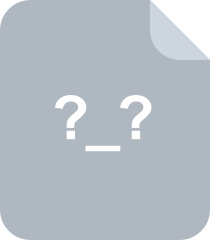
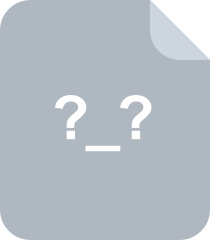
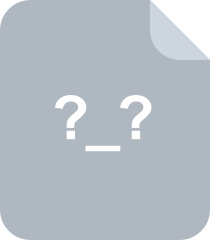
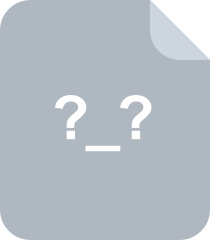
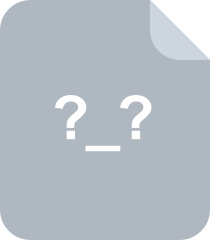
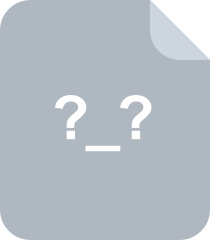
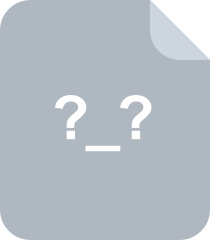
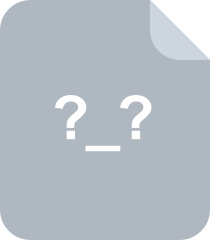
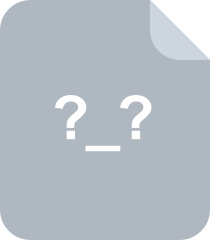
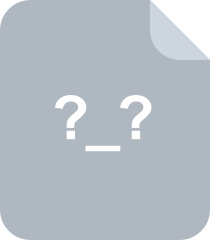
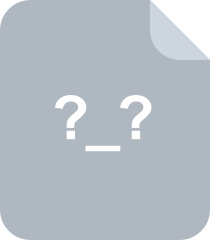
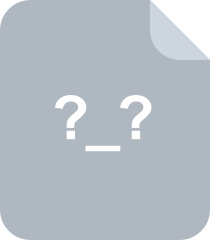

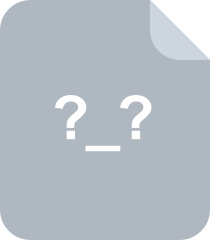
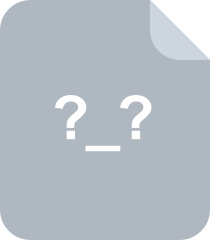
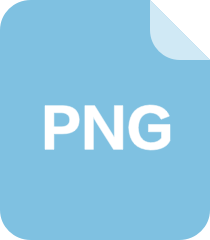
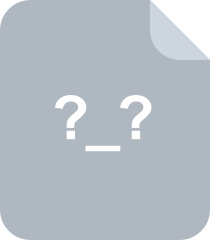
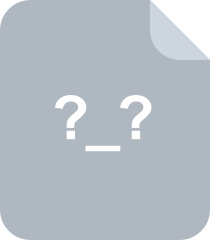
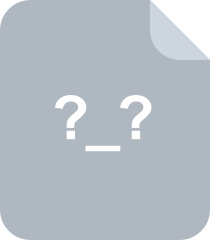
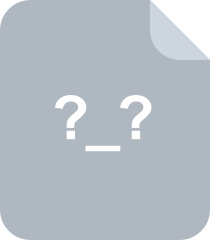
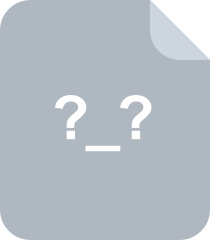
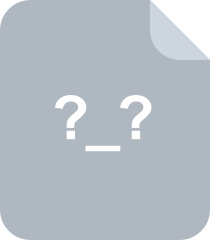
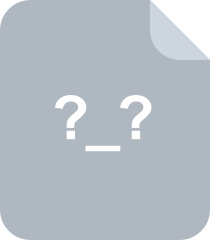
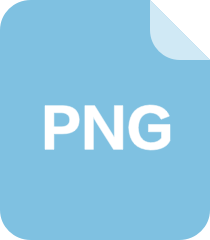

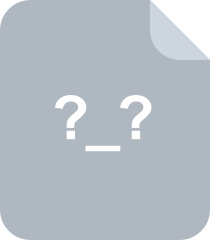
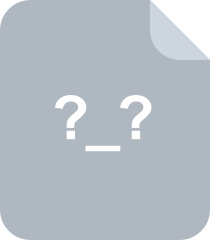
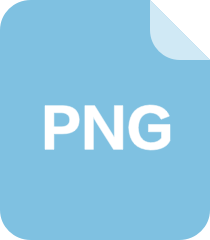
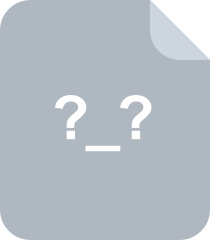
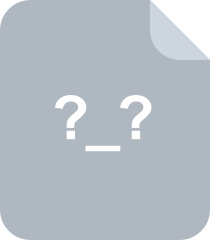
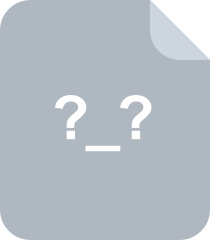
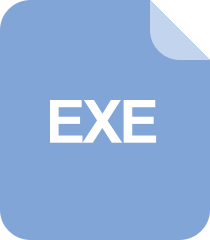
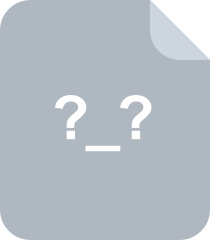
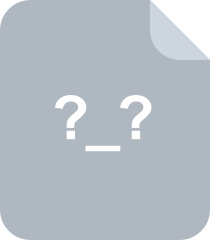
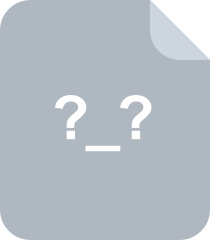
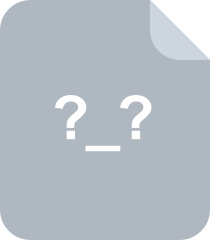
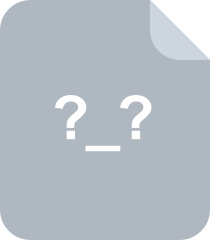
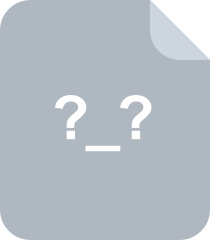
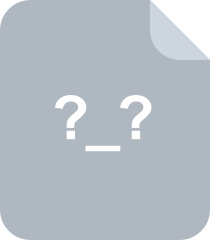
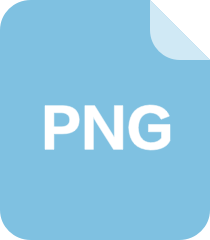
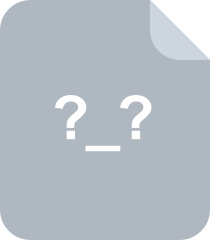
共 50 条
- 1

无幻
- 粉丝: 3547
- 资源: 151
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

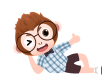
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


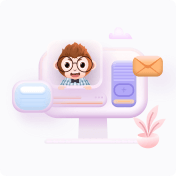
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
- 5
- 6
前往页